Why Does My Response to Preflight Request Fail Access Control Checks?
In the ever-evolving landscape of web development, the interaction between browsers and servers is governed by a complex set of rules designed to ensure security and functionality. One of the most critical aspects of this interaction is the Cross-Origin Resource Sharing (CORS) protocol, which allows or restricts resources requested from another domain outside the domain from which the first resource was served. However, developers often encounter a frustrating hurdle known as the “response to preflight request doesn’t pass access control check.” This error can halt progress and leave even seasoned programmers scratching their heads, as it signifies a breakdown in the communication between client and server.
At its core, this error arises during the preflight request phase of a CORS operation, where the browser sends an initial request to determine if the actual request is safe to send. If the server’s response does not meet the required access control criteria, the browser blocks the request, leading to the dreaded error message. Understanding the nuances of this process is essential for developers who wish to create seamless web applications that communicate across different domains without running afoul of security protocols.
Navigating the intricacies of CORS and the preflight request mechanism can be daunting, but it is crucial for ensuring that web applications function as intended. This article will delve into the common causes
Understanding Preflight Requests
Preflight requests are an essential part of the Cross-Origin Resource Sharing (CORS) protocol, which is a security feature implemented in web browsers. When a web application makes a request to a different origin (domain, protocol, or port), the browser sends an initial “preflight” request using the OPTIONS method to determine whether the actual request is safe to send. This preflight request checks the server’s CORS policy, which dictates whether the requested resource can be accessed by the calling domain.
Key aspects of preflight requests include:
- Method Verification: The server must allow the HTTP method used in the actual request (e.g., POST, PUT).
- Header Check: The server needs to specify which headers can be included in the actual request.
- Origin Validation: The server must confirm whether the requesting origin is permitted to access the resource.
Common Causes of Preflight Failures
When a preflight request fails, it can lead to the error message “response to preflight request doesn’t pass access control check.” This typically occurs due to several reasons:
- Missing CORS Headers: The server does not include necessary CORS headers in its response.
- Invalid HTTP Method: The requested method is not permitted by the server’s CORS policy.
- Restricted Headers: The server does not allow certain headers in the actual request.
- Origin Mismatch: The requesting origin is not included in the server’s allowed origins.
Resolving CORS Issues
To resolve issues related to preflight request failures, developers can implement several strategies:
- Configure Server CORS Policies: Ensure that the server is correctly configured to respond to OPTIONS requests with the appropriate headers.
- Add Allowed Origins: Include the requesting domain in the server’s CORS configuration.
- Permit Required Methods: Update the server settings to allow the necessary HTTP methods for incoming requests.
- Handle Preflight Requests Properly: Make sure the server responds to OPTIONS requests with a 200 status code and the correct CORS headers.
Example CORS Configuration
The following table outlines an example CORS configuration for a server to handle preflight requests correctly:
Header | Value |
---|---|
Access-Control-Allow-Origin | https://example.com |
Access-Control-Allow-Methods | GET, POST, OPTIONS |
Access-Control-Allow-Headers | Content-Type, Authorization |
Access-Control-Max-Age | 86400 |
By following best practices and ensuring proper server configuration, developers can effectively address and resolve CORS-related issues, ensuring smooth interactions between web applications and APIs.
Understanding Preflight Requests
Preflight requests are part of the Cross-Origin Resource Sharing (CORS) protocol, which allows web applications running at one origin to request resources from a different origin. This mechanism is crucial for ensuring secure communication between different domains.
- What is a Preflight Request?
- A preflight request is an HTTP OPTIONS request sent by the browser before a cross-origin request. It checks whether the actual request is safe to send.
- This request includes headers that provide information about the actual request method and headers that will be used in the subsequent request.
- When Does a Preflight Request Occur?
- It occurs when:
- The request method is not a simple method (e.g., GET, POST).
- The request includes custom headers.
- The request’s content type is not one of the simple types (application/x-www-form-urlencoded, multipart/form-data, or text/plain).
Common Reasons for CORS Errors
When encountering the error “response to preflight request doesn’t pass access control check,” several potential issues could be at play. Understanding these can help in troubleshooting.
- Missing CORS Headers
- The server must include the `Access-Control-Allow-Origin` header in its response.
- Example: `Access-Control-Allow-Origin: https://example.com`
- Incorrect Method or Header
- The server must allow the specific HTTP method used in the request (e.g., POST, PUT).
- The response must also specify allowed headers using `Access-Control-Allow-Headers`.
- Preflight Response Issues
- The preflight request must return a 200 OK status.
- Any non-200 responses will lead to CORS failures.
Configuring CORS on the Server
To resolve the CORS errors related to preflight requests, proper server configuration is essential. Below are guidelines for configuring CORS headers.
- Basic Configuration:
- For a Node.js/Express server:
“`javascript
const cors = require(‘cors’);
app.use(cors({
origin: ‘https://example.com’, // specify allowed origin
methods: [‘GET’, ‘POST’, ‘PUT’, ‘DELETE’], // specify allowed methods
allowedHeaders: [‘Content-Type’, ‘Authorization’] // specify allowed headers
}));
“`
- Apache Configuration:
“`apache
Header set Access-Control-Allow-Origin “https://example.com”
Header set Access-Control-Allow-Methods “GET, POST, OPTIONS”
Header set Access-Control-Allow-Headers “Content-Type, Authorization”
“`
Testing and Debugging CORS Issues
Testing and debugging CORS-related issues can be challenging. Here are some effective strategies:
- Browser Developer Tools
- Use the Network tab to inspect requests and responses for CORS headers.
- Check the preflight request and its response status.
- CORS Proxy
- Utilize a CORS proxy to bypass restrictions temporarily during development.
- Error Logs
- Review server logs to identify any issues with handling OPTIONS requests or missing headers.
- Online Tools
- Tools like Postman can simulate preflight requests to test server responses without a browser.
Addressing CORS errors requires a thorough understanding of preflight requests and server configurations. By systematically checking headers, methods, and server responses, developers can effectively resolve these issues and ensure seamless cross-origin interactions.
Understanding CORS Issues in Web Development
Dr. Emily Carter (Web Security Analyst, CyberSafe Solutions). “The error message ‘response to preflight request doesn’t pass access control check’ typically indicates that the server is not configured to allow cross-origin requests from the client. This is a common issue in web applications that need to communicate with APIs hosted on different domains. Ensuring that the server sets the appropriate CORS headers is essential for resolving this issue.”
Michael Thompson (Lead Software Engineer, Tech Innovations Inc.). “When dealing with preflight requests, it is crucial to understand the role of the OPTIONS HTTP method in CORS. If the server fails to respond correctly to the preflight request, the browser will block the actual request. Developers should meticulously check the server’s response headers, particularly ‘Access-Control-Allow-Origin’, to ensure they match the requesting origin.”
Sarah Kim (Frontend Developer, CodeCraft Agency). “In my experience, the ‘response to preflight request doesn’t pass access control check’ error often arises from misconfigurations in the server settings. It is vital to not only allow the correct origins but also to specify allowed methods and headers. Testing with tools like Postman can help identify and resolve these issues before deploying the application.”
Frequently Asked Questions (FAQs)
What does the error “response to preflight request doesn’t pass access control check” mean?
This error indicates that a preflight request, which is part of the CORS (Cross-Origin Resource Sharing) protocol, has failed. The browser is blocking the request because the server’s response does not meet the required access control criteria.
What is a preflight request in the context of CORS?
A preflight request is an HTTP OPTIONS request sent by the browser to determine if the actual request is safe to send. It checks if the server allows the requested method and headers from the origin of the request.
How can I resolve the “response to preflight request doesn’t pass access control check” error?
To resolve this error, ensure that your server is configured to respond correctly to preflight requests. This includes setting appropriate CORS headers, such as `Access-Control-Allow-Origin`, `Access-Control-Allow-Methods`, and `Access-Control-Allow-Headers`.
What headers should be included in the server response to a preflight request?
The server response should include `Access-Control-Allow-Origin` to specify allowed origins, `Access-Control-Allow-Methods` to list permitted HTTP methods, and `Access-Control-Allow-Headers` to indicate which headers can be used in the actual request.
Can this error occur due to incorrect server configuration?
Yes, incorrect server configuration is a common cause of this error. If the server does not explicitly allow the origin or the methods being requested, the browser will block the request.
Are there any tools to help diagnose CORS issues?
Yes, tools like browser developer tools (F12), Postman, or various CORS debugging extensions can help diagnose CORS issues by inspecting request and response headers and identifying where the configuration may be incorrect.
The error message “response to preflight request doesn’t pass access control check” typically arises in the context of web applications that utilize Cross-Origin Resource Sharing (CORS). This issue occurs when a web application attempts to make a request to a different origin (domain, protocol, or port) than the one that served the web page. The browser sends a preflight request to the server to determine if the actual request is safe to send. If the server does not respond with the appropriate headers or fails to acknowledge the request, the browser will block the request, resulting in this error.
Understanding the CORS mechanism is crucial for developers to effectively troubleshoot and resolve this issue. The server must explicitly allow the requesting origin by including the `Access-Control-Allow-Origin` header in its response. Additionally, it may need to handle other headers such as `Access-Control-Allow-Methods` and `Access-Control-Allow-Headers` to ensure that the preflight request is accepted. Failure to configure these headers correctly can lead to the blocking of legitimate requests, which can severely impact the functionality of web applications.
In summary, addressing the “response to preflight request doesn’t pass access control check” error involves ensuring that the server is properly configured to handle CORS requests.
Author Profile
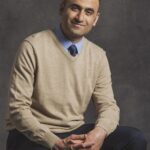
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?