How Does RestSharp Authenticate When Working with Services and Interfaces in C#?
In today’s digital landscape, seamless communication between applications is vital for delivering robust services and enhancing user experiences. As developers strive to build efficient and secure applications, the integration of authentication mechanisms becomes paramount. RestSharp, a popular HTTP client for .NET, offers a powerful toolkit for making API calls and handling authentication with ease. In this article, we will explore how to effectively authenticate with services using RestSharp while leveraging interfaces in C. Whether you’re building a new application or enhancing an existing one, understanding these concepts will empower you to create secure and efficient connections to external services.
When working with RestSharp, the ability to authenticate against various services is crucial for ensuring that your application can access protected resources. This involves not only understanding the different authentication methods available—such as OAuth, API keys, and basic authentication—but also implementing them in a way that aligns with best practices. By utilizing interfaces in C, developers can create more flexible and maintainable code, allowing for easier testing and integration of different authentication strategies. This approach enables you to abstract the authentication process, making it easier to switch between different services or modify your authentication logic without significant code changes.
In this article, we will delve into the practical aspects of using RestSharp for authentication, including how to set up your client,
Understanding Authentication with RestSharp
When working with RestSharp to authenticate against a service, it’s essential to understand the various authentication mechanisms it supports. RestSharp is a popular HTTP client library for .NET that simplifies REST API calls. It provides built-in support for several authentication methods, including Basic, OAuth, and API Key authentication.
To authenticate using RestSharp, you typically need to set the appropriate headers or parameters in your request. Below are some common authentication methods and how to implement them in RestSharp:
- Basic Authentication: This method involves sending a username and password encoded in Base64.
- OAuth: This is used for more complex scenarios, where you need to obtain an access token to authenticate requests.
- API Key: This involves sending a unique key in the header or as a query parameter.
Implementing an Interface for Service Calls
When designing a service layer that interacts with external APIs, it is beneficial to define an interface. This promotes loose coupling and makes it easier to implement unit tests. Here’s a sample interface definition in C:
“`csharp
public interface IApiService
{
Task
Task
}
“`
By using this interface, you can create a concrete implementation that utilizes RestSharp for making HTTP calls:
“`csharp
public class RestApiService : IApiService
{
private readonly RestClient _client;
public RestApiService(string baseUrl)
{
_client = new RestClient(baseUrl);
}
public async Task
{
var request = new RestRequest(resource, Method.GET);
var response = await _client.ExecuteAsync
return response.Data;
}
public async Task
{
var request = new RestRequest(resource, Method.POST);
request.AddJsonBody(body);
var response = await _client.ExecuteAsync
return response.Data;
}
}
“`
Configuring Authentication in RestSharp
To configure authentication in RestSharp, you can use the `AddHeader` method for API Key or Basic Authentication. For OAuth, you might need to handle token retrieval and refresh cycles. Below is a table summarizing how to set up different authentication methods:
Authentication Type | Implementation |
---|---|
Basic Authentication | request.AddHeader(“Authorization”, “Basic ” + Convert.ToBase64String(Encoding.ASCII.GetBytes(“username:password”))); |
API Key | request.AddHeader(“x-api-key”, “your_api_key_here”); |
OAuth | request.AddHeader(“Authorization”, “Bearer ” + accessToken); |
By structuring your service interactions with an interface and implementing various authentication methods, you can create a robust and flexible application that easily integrates with different RESTful services. Properly managing authentication will enhance security and ensure reliable communication with external APIs.
Understanding RestSharp for Authentication
RestSharp is a popular library in .NET for consuming RESTful APIs. When working with services that require authentication, it’s essential to understand how to implement authentication mechanisms effectively using RestSharp.
Common Authentication Mechanisms
When working with RestSharp, several authentication methods can be utilized, including:
- Basic Authentication: Simple and widely supported, where the username and password are encoded in base64.
- Bearer Token Authentication: Common in OAuth2, where a token is passed in the Authorization header.
- API Key Authentication: Using a key as a query parameter or in headers.
Implementing Basic Authentication
To implement basic authentication with RestSharp, follow these steps:
“`csharp
var client = new RestClient(“https://api.example.com”);
var request = new RestRequest(“endpoint”, Method.GET);
request.AddHeader(“Authorization”, “Basic ” + Convert.ToBase64String(Encoding.ASCII.GetBytes(“username:password”)));
var response = client.Execute(request);
“`
This code snippet demonstrates how to set up a RestSharp client and request while adding the necessary headers for basic authentication.
Using Bearer Tokens
For APIs using bearer token authentication, here is an example implementation:
“`csharp
var client = new RestClient(“https://api.example.com”);
var request = new RestRequest(“endpoint”, Method.GET);
request.AddHeader(“Authorization”, “Bearer YOUR_ACCESS_TOKEN”);
var response = client.Execute(request);
“`
Replace `YOUR_ACCESS_TOKEN` with the actual token received from the authentication server.
API Key Authentication Example
API key authentication can be done by adding the key directly to the headers or as a query parameter:
Header Method:
“`csharp
var client = new RestClient(“https://api.example.com”);
var request = new RestRequest(“endpoint”, Method.GET);
request.AddHeader(“x-api-key”, “YOUR_API_KEY”);
var response = client.Execute(request);
“`
Query Parameter Method:
“`csharp
var client = new RestClient(“https://api.example.com”);
var request = new RestRequest(“endpoint?api_key=YOUR_API_KEY”, Method.GET);
var response = client.Execute(request);
“`
Working with Interfaces
To enhance maintainability and testability, it is advisable to use interfaces when interacting with APIs. Define an interface for your service:
“`csharp
public interface IApiService
{
IRestResponse GetData(string endpoint);
}
“`
Then, implement this interface in a class:
“`csharp
public class ApiService : IApiService
{
private readonly RestClient _client;
public ApiService(string baseUrl)
{
_client = new RestClient(baseUrl);
}
public IRestResponse GetData(string endpoint)
{
var request = new RestRequest(endpoint, Method.GET);
// Configure authentication here as needed
return _client.Execute(request);
}
}
“`
This structure allows for easier unit testing and future enhancements.
Dependency Injection Example
When using dependency injection, you can register your service in a .NET Core application as follows:
“`csharp
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped
}
“`
This registration enables you to inject `IApiService` where needed, promoting a clean architecture.
Handling Responses
After executing a request, handling the response is crucial:
“`csharp
var response = client.Execute(request);
if (response.IsSuccessful)
{
var content = response.Content; // Process content
}
else
{
// Handle error
var errorMessage = response.ErrorMessage;
}
“`
This approach ensures that you manage both successful and erroneous responses effectively.
Conclusion on Best Practices
Adhering to best practices when using RestSharp for authentication involves:
- Selecting the appropriate authentication method based on API requirements.
- Structuring your code using interfaces for better maintainability.
- Utilizing dependency injection to manage service lifetimes.
- Properly handling API responses to ensure robust applications.
Expert Insights on Using RestSharp for Authentication in CServices
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). RestSharp provides a robust framework for making HTTP requests in C. When implementing authentication with services and interfaces, it is crucial to understand the underlying authentication mechanisms, such as OAuth or API keys, to ensure secure communication between the client and server.
Michael Thompson (Lead Developer, Cloud Solutions Group). Utilizing RestSharp for authentication in Crequires a clear understanding of how to configure the client with the necessary headers and parameters. By encapsulating authentication logic in a dedicated service interface, developers can promote code reusability and maintainability while ensuring that authentication is consistently applied across various service calls.
Sarah Lee (Principal Consultant, SecureCode Practices). When working with RestSharp in C, it is essential to implement proper error handling and logging during the authentication process. This not only aids in debugging but also enhances the security posture by allowing developers to monitor unauthorized access attempts and respond accordingly.
Frequently Asked Questions (FAQs)
How does RestSharp handle authentication with a service?
RestSharp supports various authentication methods, including Basic, OAuth1, and OAuth2. You can configure authentication by setting the appropriate properties on the RestRequest object or by using the RestClient’s authentication methods.
Can I use RestSharp to authenticate with a web API that requires token-based authentication?
Yes, RestSharp can be used to authenticate with token-based APIs. You can add the token to the request headers using the `AddHeader` method of the RestRequest object, ensuring that the token is included in each request.
What is the best way to implement an interface for service authentication in Cusing RestSharp?
To implement an interface for service authentication, define an interface that includes methods for authentication. Implement this interface in a class that uses RestSharp to handle the authentication logic, such as acquiring and refreshing tokens.
Is it possible to manage multiple authentication types in a single RestSharp client?
Yes, you can manage multiple authentication types by configuring the RestClient or RestRequest dynamically based on the requirements of each API endpoint. This involves setting the appropriate authentication method before making requests.
How can I handle expired tokens when using RestSharp for authentication?
To handle expired tokens, you should implement a mechanism to check the token’s validity before making requests. If the token is expired, use your authentication logic to refresh the token and then retry the original request with the new token.
Can RestSharp be used with dependency injection for service interfaces in C?
Yes, RestSharp can be integrated with dependency injection in C. You can register your RestClient and any service interfaces in the DI container, allowing for better management of service instances and promoting loose coupling in your application architecture.
RestSharp is a popular library in Cthat simplifies the process of making HTTP requests to RESTful services. When integrating authentication mechanisms with RestSharp, developers can leverage various authentication methods, such as Basic, OAuth, and API keys, to ensure secure communication with services. Understanding how to implement these authentication strategies is crucial for maintaining the integrity and confidentiality of data exchanged between clients and servers.
Working with interfaces in Cenhances the flexibility and maintainability of the code. By defining service interfaces, developers can create abstractions that allow for easier testing and swapping of implementations without affecting the overall application architecture. This approach aligns well with RestSharp, as it enables developers to define clear contracts for service interactions while providing the capability to mock these services during unit testing.
effectively utilizing RestSharp for authentication in conjunction with service interfaces in Cleads to a robust and secure application design. It allows developers to implement various authentication strategies while promoting code reusability and testability through the use of interfaces. By adopting these practices, developers can ensure that their applications are not only functional but also secure and maintainable in the long term.
Author Profile
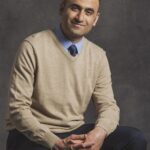
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?