How Can You Use RestSharp to Send a POST Request for Authentication in C#?
In the world of modern web development, seamless communication between client applications and servers is paramount. As APIs become increasingly integral to software solutions, developers often seek efficient ways to handle HTTP requests, especially when it comes to authentication. One popular library that simplifies this process in Cis RestSharp. With its intuitive interface and robust features, RestSharp allows developers to easily send HTTP requests, making it an ideal choice for those looking to implement authentication mechanisms in their applications. In this article, we will explore how to effectively use RestSharp to send POST requests for authentication, guiding you through the essentials to enhance your API interactions.
When it comes to authenticating users in web applications, sending POST requests is a common practice. This method allows developers to securely transmit user credentials to a server, which then verifies the information and grants access. RestSharp streamlines this process with its straightforward syntax, enabling developers to focus on building features rather than wrestling with complex HTTP requests. By leveraging RestSharp, you can implement various authentication strategies, such as token-based or session-based authentication, with ease.
In this article, we will delve into practical examples of how to utilize RestSharp for sending POST requests specifically tailored for authentication. Whether you’re a seasoned developer or just starting out, understanding the nuances of using RestSharp
RestSharp Overview
RestSharp is a popular library for making HTTP requests in .NET applications. It simplifies the process of sending and receiving HTTP requests and responses, making it a go-to choice for developers looking to integrate web services into their applications. RestSharp supports various HTTP methods, including GET, POST, PUT, and DELETE, and is particularly useful for interacting with RESTful APIs.
Sending a POST Request
To send a POST request for authentication using RestSharp, you typically need to set up a client, create a request, and then execute that request. Below is a step-by-step example demonstrating how to achieve this.
Example Code
“`csharp
using RestSharp;
var client = new RestClient(“https://api.example.com/authenticate”);
var request = new RestRequest(Method.POST);
// Setting request body parameters
request.AddJsonBody(new
{
username = “your_username”,
password = “your_password”
});
// Executing the request
var response = client.Execute(request);
// Handling the response
if (response.IsSuccessful)
{
// Process successful authentication
var content = response.Content;
Console.WriteLine(“Authentication successful: ” + content);
}
else
{
// Handle error
Console.WriteLine(“Authentication failed: ” + response.ErrorMessage);
}
“`
In this example:
- A `RestClient` is created with the authentication URL.
- A `RestRequest` is initialized with the HTTP method set to POST.
- The username and password are added as a JSON body to the request.
- The request is executed, and the response is checked for success.
Key Components
When sending a POST request using RestSharp, consider the following key components:
- RestClient: The main class responsible for making requests to the specified API endpoint.
- RestRequest: Represents the request to be sent, including method type and parameters.
- Execute Method: Sends the request and returns the response.
Handling Responses
When handling the response, it is essential to check whether the request was successful. The response object contains various properties that can provide valuable information:
Property | Description |
---|---|
IsSuccessful | Indicates whether the request was successful (status codes 200-299). |
Content | Contains the body of the response as a string. |
ErrorMessage | Provides an error message if the request failed. |
Utilizing these properties allows for effective error handling and response processing, making your authentication logic robust and user-friendly.
By leveraging RestSharp for sending POST requests, developers can efficiently implement authentication mechanisms in their applications. This approach not only streamlines the process but also enhances the overall maintainability of the code.
RestSharp Overview
RestSharp is a popular HTTP client library for .NET that simplifies the process of sending requests and receiving responses from RESTful APIs. It provides a fluent interface for building requests and handling responses, making it an excellent choice for developers working with APIs.
Sending a POST Request for Authentication
To send a POST request using RestSharp for authentication, you generally need to provide the URL of the authentication endpoint along with any required headers and body parameters. Below is an example that demonstrates how to achieve this.
Example Code
The following Ccode snippet illustrates how to send a POST request for authentication using RestSharp:
“`csharp
using RestSharp;
using System;
class Program
{
static void Main(string[] args)
{
var client = new RestClient(“https://api.example.com/authenticate”);
var request = new RestRequest(Method.POST);
request.AddHeader(“Content-Type”, “application/json”);
request.AddJsonBody(new
{
username = “your_username”,
password = “your_password”
});
IRestResponse response = client.Execute(request);
if (response.IsSuccessful)
{
Console.WriteLine(“Authentication successful.”);
Console.WriteLine(response.Content);
}
else
{
Console.WriteLine(“Authentication failed. Status: ” + response.StatusCode);
Console.WriteLine(“Error: ” + response.Content);
}
}
}
“`
Key Components Explained
- RestClient: Initializes the client with the base URL for the API.
- RestRequest: Represents the request, specifying the HTTP method (POST in this case).
- AddHeader: Adds necessary headers to the request, such as `Content-Type`.
- AddJsonBody: Sends the authentication credentials as a JSON object in the request body.
- Execute: Sends the request and returns the response.
Handling the Response
The response from the API can be examined to determine if the authentication was successful. Use the following properties for effective response handling:
Property | Description |
---|---|
IsSuccessful | Indicates if the request was successful. |
StatusCode | Contains the HTTP status code of the response. |
Content | Holds the response body returned by the server. |
ErrorMessage | Provides error details if the request failed. |
Common Authentication Scenarios
When working with APIs, the authentication method may vary. Below are common scenarios:
- Basic Authentication: Include the `Authorization` header with Base64 encoded credentials.
- Bearer Token Authentication: Include the `Authorization` header with a token obtained from a previous login request.
- OAuth2: Follow the OAuth2 flow to obtain an access token and use it in the request headers.
Best Practices
- Always handle exceptions and errors gracefully.
- Secure sensitive information like passwords and tokens.
- Log responses and errors for troubleshooting purposes.
- Use HTTPS to encrypt data in transit.
By following the above guidelines and utilizing the provided example, developers can efficiently implement authentication mechanisms in their applications using RestSharp.
Expert Insights on Sending POST Requests for Authentication with RestSharp
Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Using RestSharp for sending POST requests is an efficient way to handle authentication in .NET applications. The library simplifies the process by allowing developers to easily configure headers and body content, making it ideal for RESTful APIs that require token-based authentication.”
Michael Chen (API Integration Specialist, CodeCraft Solutions). “When implementing authentication with RestSharp, it is crucial to ensure that the request is properly formatted. This includes setting the correct content type and including any necessary authentication tokens in the headers. A well-structured POST request can significantly enhance security and user experience.”
Sarah Thompson (Lead Developer, SecureApp Technologies). “RestSharp provides a robust framework for managing authentication requests. By leveraging its asynchronous capabilities, developers can improve application performance while maintaining secure communication with authentication servers. This approach is essential for modern applications that demand speed and security.”
Frequently Asked Questions (FAQs)
What is RestSharp?
RestSharp is a popular open-source library for .NET that simplifies the process of making HTTP requests to RESTful APIs. It provides a straightforward way to send requests and handle responses.
How do I send a POST request using RestSharp in C?
To send a POST request using RestSharp, create a `RestClient` instance, build a `RestRequest` with the desired endpoint and method, and then use the `Execute` method to send the request. For example:
“`csharp
var client = new RestClient(“https://api.example.com”);
var request = new RestRequest(“auth”, Method.POST);
request.AddJsonBody(new { username = “user”, password = “pass” });
var response = client.Execute(request);
“`
How can I handle authentication with RestSharp?
Authentication can be handled by including the necessary credentials in the request headers or body. For example, for Basic Authentication, you can set the `Authorization` header:
“`csharp
request.AddHeader(“Authorization”, “Basic ” + Convert.ToBase64String(Encoding.ASCII.GetBytes(“username:password”)));
“`
What types of authentication does RestSharp support?
RestSharp supports various authentication methods, including Basic, OAuth1, OAuth2, and custom token-based authentication. You can configure these methods using the appropriate headers or request parameters.
How do I check the response status of a POST request in RestSharp?
After executing a request, you can check the response status by examining the `StatusCode` property of the response object. For example:
“`csharp
if (response.StatusCode == HttpStatusCode.OK) {
// Handle success
} else {
// Handle error
}
“`
Can I send JSON data in a POST request using RestSharp?
Yes, you can send JSON data in a POST request by using the `AddJsonBody` method to serialize your object into JSON format automatically. This ensures that the content type is set to `application/json`.
RestSharp is a popular library in Cused for making HTTP requests, particularly for RESTful APIs. When it comes to sending a POST request for authentication, RestSharp simplifies the process significantly. The library allows developers to create requests easily, handle responses, and manage serialization and deserialization of data seamlessly. This is particularly useful when dealing with APIs that require authentication tokens or credentials to access secured resources.
In a typical authentication scenario using RestSharp, developers initiate a new RestClient instance, specify the endpoint URL, and create a RestRequest object with the appropriate HTTP method set to POST. The necessary authentication parameters, such as username and password, can be added to the request body or as query parameters, depending on the API’s requirements. Once the request is configured, it can be executed, and the response can be processed to extract authentication tokens or error messages as needed.
Key takeaways from using RestSharp for authentication include its ease of use and flexibility. The library supports various content types, making it adaptable to different API specifications. Furthermore, the ability to handle asynchronous requests allows for more responsive applications. Overall, RestSharp is a valuable tool for developers looking to integrate authentication mechanisms into their applications efficiently.
Author Profile
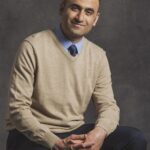
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?