How Can I Retrieve the COM Class Factory for a Component with CLSID?
In the realm of software development, especially when working with Windows applications, developers often encounter complex interactions between different components and libraries. One such challenge arises when trying to access COM (Component Object Model) components through their unique identifiers known as CLSIDs (Class Identifiers). The phrase “retrieving the COM class factory for component with CLSID” encapsulates a common hurdle that many developers face, particularly when integrating legacy systems or third-party components into their applications. Understanding this process is crucial for seamless application performance and functionality, and it opens the door to leveraging powerful features that COM components offer.
When a developer attempts to instantiate a COM object, they must first navigate the intricacies of the COM architecture, which includes registering the component and ensuring that the appropriate permissions and configurations are in place. The CLSID serves as a key to unlock the desired component, but if the system cannot locate the class factory associated with that CLSID, it results in frustrating errors that can halt development. This article delves into the underlying mechanisms of COM, exploring how to effectively retrieve class factories and troubleshoot common pitfalls that may arise during this process.
As we journey through the nuances of COM components and CLSIDs, we will uncover best practices for registration, instantiation, and error handling. By equ
Understanding CLSID and COM Class Factory
To effectively address the issue of retrieving the COM class factory for a component with a specific CLSID, it’s essential to comprehend the significance of CLSIDs (Class Identifiers) and the role of COM (Component Object Model) in Windows applications. A CLSID is a globally unique identifier that identifies a COM class. When an application needs to create an instance of a COM object, it uses the CLSID to locate the appropriate COM class factory, which is responsible for producing instances of that component.
The retrieval process typically involves several key steps:
- Registration: The COM component must be properly registered in the Windows Registry. This registration associates the CLSID with the relevant class factory.
- Accessing the Factory: The application utilizes the `CoCreateInstance` function or similar APIs to request an instance of the COM object.
- Error Handling: If the component is not registered or accessible, the application may encounter errors such as `CLASS_E_CLASSNOTAVAILABLE` or `CO_E_NOTINITIALIZED`.
Common Errors and Troubleshooting
When dealing with COM components, several common errors may arise while attempting to retrieve the class factory. Understanding these errors can help in troubleshooting effectively.
Error Code | Description | Possible Solutions |
---|---|---|
`CLASS_E_CLASSNOTAVAILABLE` | The requested class is not registered or available. | Check registration status in the registry. |
`CO_E_NOTINITIALIZED` | COM library not initialized. | Call `CoInitialize` or `CoInitializeEx`. |
`E_NOINTERFACE` | The requested interface is not supported by the class. | Verify the interface ID and implementation. |
To troubleshoot these issues, consider the following approaches:
- Check Registration: Use tools such as `regedit` to ensure that the CLSID appears under the `HKEY_CLASSES_ROOT\CLSID` key.
- Run as Administrator: Some components may require elevated privileges to access. Running the application as an administrator can resolve permission-related issues.
- 64-bit vs 32-bit: Ensure that the application architecture (32-bit or 64-bit) matches that of the COM component. Mismatched architectures can lead to retrieval failures.
Best Practices for Handling COM Components
To ensure smooth operation when working with COM components, developers should adhere to several best practices:
- Proper Initialization: Always initialize the COM library using `CoInitialize` or `CoInitializeEx` before making any COM calls.
- Release Resources: Use `Release` on COM objects to free resources and avoid memory leaks.
- Error Checking: Implement comprehensive error checking after each COM call to handle potential issues early in the execution flow.
- Threading Models: Understand the threading model of the COM component and ensure that your application’s threading model aligns with it.
By following these guidelines, developers can effectively manage COM components and minimize the likelihood of errors related to CLSID retrieval.
Understanding the COM Class Factory and CLSID
The Component Object Model (COM) is a Microsoft technology that allows software components to communicate. Each COM component is identified by a Class ID (CLSID), which is a unique identifier. The process of retrieving the COM class factory for a component using its CLSID is crucial for instantiating COM objects.
When an application requires a COM object, it utilizes the CLSID to locate the class factory, which is responsible for creating instances of the COM component. This process often involves several steps, including registry access and ensuring that the necessary permissions are in place.
Common Causes of Errors
Errors related to retrieving the COM class factory are often caused by:
- Incorrect CLSID: The provided CLSID does not correspond to a registered component.
- Registration Issues: The COM component might not be properly registered in the Windows registry.
- Permissions: Insufficient privileges to access the COM component or its registry entries.
- Architecture Mismatch: The application trying to access the COM component may be running in a different architecture (32-bit vs. 64-bit) than the component itself.
Steps to Troubleshoot COM Class Factory Retrieval Issues
To address issues when retrieving the COM class factory, consider the following troubleshooting steps:
- Verify CLSID:
- Ensure that the CLSID is correct.
- Use tools like `RegEdit` to check the registry for the corresponding entry.
- Check Registration:
- Open the Windows Registry Editor.
- Navigate to `HKEY_CLASSES_ROOT\CLSID\{Your-CLSID}`.
- Confirm that the component is listed and has the correct subkeys, such as `InprocServer32` or `LocalServer32`.
- Confirm Permissions:
- Right-click the CLSID key in the registry.
- Select “Permissions” and ensure that your user has the necessary access rights.
- Match Application Architecture:
- If your application is 32-bit, ensure that you are accessing a 32-bit COM component.
- Similarly, for 64-bit applications, ensure that the components are registered under the appropriate registry view.
Using Code to Access COM Components
Implementing code to retrieve a COM object involves specific programming techniques depending on the language used. Here is an example using C:
“`csharp
using System;
using System.Runtime.InteropServices;
class Program
{
static void Main()
{
Type comType = Type.GetTypeFromProgID(“Your.ProgID”);
if (comType != null)
{
object comObject = Activator.CreateInstance(comType);
// Use the comObject as needed
}
else
{
Console.WriteLine(“Unable to retrieve the COM class.”);
}
}
}
“`
In this code snippet, replace `”Your.ProgID”` with the appropriate Programmatic Identifier for the desired COM component. This method leverages the `Activator.CreateInstance` method to instantiate the COM object dynamically.
Conclusion of Key Points
When retrieving the COM class factory for a component with CLSID, it is essential to:
- Validate the CLSID and its registration.
- Ensure correct permissions are set.
- Match the architecture of the application and the COM component.
- Utilize appropriate coding techniques to access the COM object successfully.
By following these guidelines, developers can effectively troubleshoot and resolve issues related to COM component instantiation.
Understanding COM Class Factory Issues in Software Development
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “Retrieving the COM class factory for a component with CLSID often indicates issues with registration or compatibility. It’s crucial to ensure that the component is properly registered in the Windows Registry and that the application has the necessary permissions to access it.”
Mark Thompson (Lead Developer, Legacy Systems Solutions). “When encountering the error related to retrieving the COM class factory, developers should first verify the CLSID in the system registry. Misconfigured or missing entries can lead to this problem, and a thorough check can often reveal the underlying issue.”
Lisa Chen (Technical Consultant, Software Reliability Experts). “In many cases, this error can arise from version mismatches between the COM component and the application. Ensuring that both are compatible and that the correct version of the component is registered can resolve these types of issues effectively.”
Frequently Asked Questions (FAQs)
What does “retrieving the COM class factory for component with CLSID” mean?
This phrase refers to an error encountered when a system is unable to create an instance of a COM (Component Object Model) object identified by its CLSID (Class Identifier). It indicates a failure in accessing the required component due to issues such as registration problems or missing dependencies.
What are common causes of this error?
Common causes include improper registration of the COM component, missing or corrupted files, insufficient permissions, or the component not being installed on the system. Additionally, it may arise from compatibility issues between the component and the operating system.
How can I resolve this error?
To resolve this error, ensure that the COM component is properly registered using tools like `regsvr32`. Check for missing dependencies, verify user permissions, and ensure that the component is compatible with your operating system. Reinstalling the component may also help.
What tools can I use to troubleshoot this issue?
You can use tools such as the Windows Event Viewer to check for related error logs, Dependency Walker to identify missing dependencies, and the Registry Editor to verify the registration of the CLSID. Additionally, application-specific logs may provide more context.
Is this error specific to certain programming languages or environments?
While the error can occur in various programming environments that utilize COM components, it is most commonly encountered in languages such as C, VB.NET, and unmanaged C++. The specific context of the error may vary based on the application and the COM component being used.
Can this error occur on both 32-bit and 64-bit systems?
Yes, this error can occur on both 32-bit and 64-bit systems. However, it is important to ensure that the correct version of the COM component is registered for the architecture of the application attempting to access it. Compatibility between the component and the application architecture is crucial.
Retrieving the COM class factory for a component with a specific CLSID (Class Identifier) is a fundamental task in Windows programming, particularly when working with Component Object Model (COM) components. This process involves using the `CoGetClassObject` function, which allows developers to obtain a pointer to the class factory of a specified COM class. The CLSID serves as a unique identifier for the COM object, ensuring that the correct component is instantiated and utilized within the application.
One of the critical aspects of this process is understanding the registration of COM components. A COM class must be properly registered in the Windows Registry to be accessible through its CLSID. This registration includes details about the class, such as its ProgID (Programmatic Identifier), threading model, and the location of the associated DLL or executable. Without proper registration, attempts to retrieve the class factory will result in errors, hindering the functionality of applications that rely on these components.
Another important takeaway is the handling of potential errors during the retrieval process. Developers should implement robust error-checking mechanisms to manage scenarios where the CLSID is not found or when the class factory cannot be instantiated. This includes understanding and interpreting the HRESULT values returned by the COM functions, which can provide insight
Author Profile
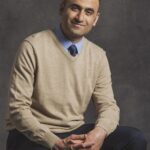
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?