How Can I Rewrite an Absolute Path to a Relative Path?
In the world of web development and file management, the distinction between absolute and relative paths is crucial for ensuring seamless navigation and functionality. Whether you’re building a website, organizing files, or collaborating on a project, understanding how to rewrite absolute paths into relative paths can significantly enhance your workflow. This process not only streamlines your code but also makes it more adaptable and portable across different environments. If you’ve ever encountered broken links or struggled with file references after moving your project, mastering this skill is essential.
At its core, an absolute path specifies a file’s location in relation to the root directory, providing a complete address that remains constant regardless of the current working directory. In contrast, a relative path offers a more flexible approach, allowing you to reference files in relation to the current directory, which can be particularly useful in collaborative projects or when deploying applications across various servers. By rewriting absolute paths to relative paths, developers can create more resilient code that is less prone to errors when files are relocated or when the project structure changes.
This article will delve into the practical techniques for converting absolute paths to relative paths, exploring the benefits of this approach and providing insights into best practices. Whether you’re a seasoned developer or just starting your journey in coding, understanding how to effectively manage file paths can elevate your projects
Understanding Absolute and Relative Paths
Absolute paths provide a complete address to a resource in a file system, detailing the exact location from the root directory. For example, an absolute path on a Unix-based system might look like `/home/user/documents/file.txt`. This path is unambiguous and always points to the same file, regardless of the current working directory.
In contrast, relative paths are dependent on the current working directory. They provide a more flexible way to reference files by indicating the location relative to the current directory. For instance, if the current directory is `/home/user`, a relative path to the same file would simply be `documents/file.txt`. The primary advantage of using relative paths is their portability; they remain valid as long as the directory structure is maintained.
Converting Absolute Paths to Relative Paths
To rewrite an absolute path as a relative path, follow these steps:
- Identify the Common Base: Determine the directory where both the absolute path and the current directory intersect.
- Navigate Upwards: Count how many directories you need to traverse upwards from the current directory to reach the common base. Use `..` to represent each upward move.
- Add the Remaining Path: Append the remaining path from the common base to the absolute path.
For example, if you are currently in `/home/user` and want to convert the absolute path `/home/user/documents/file.txt` to a relative path, you would follow these steps:
- The common base is `/home/user`.
- You do not need to move up any directories since you are already in the base.
- The remaining path is `documents/file.txt`.
Thus, the relative path is simply `documents/file.txt`.
Practical Examples of Path Conversion
Here are some examples illustrating the conversion process from absolute to relative paths:
Current Directory | Absolute Path | Relative Path |
---|---|---|
`/home/user` | `/home/user/documents/file.txt` | `documents/file.txt` |
`/home/user/documents` | `/home/user/documents/reports/file.docx` | `reports/file.docx` |
`/var/www/html` | `/var/www/html/images/photo.jpg` | `images/photo.jpg` |
`/usr/local/bin` | `/usr/local/share/documents/manual.pdf` | `../share/documents/manual.pdf` |
Considerations When Using Relative Paths
While relative paths are beneficial for portability and flexibility, there are some considerations to keep in mind:
- Context Sensitivity: Relative paths depend on the current working directory. Changing the directory may break the link to the intended resource.
- Complex Structures: In complex directory structures, relative paths can become lengthy and harder to manage.
- Cross-Platform Compatibility: Different operating systems use different path separators (e.g., `/` for Unix-based systems and `\` for Windows), which may necessitate additional handling when working in cross-platform environments.
By understanding the nuances of absolute and relative paths, developers can create more robust and adaptable file referencing systems within their applications.
Understanding Absolute and Relative Paths
Absolute paths provide the complete location to a file or directory in the file system, starting from the root directory. In contrast, relative paths define a location in relation to the current working directory. Understanding the difference is crucial for effective file management and navigation in various environments.
Characteristics of Absolute Paths:
- Start from the root directory (e.g., `/home/user/documents/file.txt`).
- Provide a complete address to a resource, ensuring no ambiguity.
- Useful for scripts and configurations where the exact file location is necessary.
Characteristics of Relative Paths:
- Start from the current directory (e.g., `documents/file.txt`).
- Offer flexibility, particularly when moving projects across different environments.
- Simplify path management in collaborative settings.
Converting Absolute Paths to Relative Paths
To convert an absolute path to a relative path, follow these steps:
- Identify the Current Directory: Determine the directory from which you want to create the relative path.
- Locate the Absolute Path: Note the absolute path of the target file or directory.
- Break Down the Paths:
- Count the levels from the current directory to the common base directory in both paths.
- Identify the remaining path from the base directory to the target.
Example Conversion:
- Absolute Path: `/home/user/documents/project/report.txt`
- Current Directory: `/home/user/documents/`
Steps:
- The common base is `/home/user/documents/`.
- Move to the base using `..` for each level, then specify the target file:
- `..` (goes to `/home/user`) + `project/report.txt`
Relative Path: `project/report.txt`
Practical Applications of Relative Paths
Relative paths are particularly beneficial in various scenarios:
- Web Development:
- Linking to CSS, JavaScript, and image files using relative links enhances portability across environments.
- Version Control Systems:
- Keeping paths relative allows for easier collaboration and merging of code from different developers.
- Scripting and Automation:
- Scripts that utilize relative paths can be run from any directory without modification.
Common Pitfalls When Using Relative Paths
When working with relative paths, be cautious of the following:
- Changing Directories: If the current working directory changes, the relative path may no longer point to the correct location.
- Nested Structures: In deeply nested directories, long relative paths can become complex and error-prone.
- Cross-Platform Compatibility: Different operating systems may interpret paths differently (e.g., Windows uses backslashes).
Tools and Techniques for Conversion
Several tools and techniques can facilitate the conversion from absolute to relative paths:
Tool/Technique | Description |
---|---|
Command Line Tools | Use commands like `pwd`, `cd`, and `realpath` in UNIX/Linux. |
Code Editors | Many IDEs and text editors provide built-in features for path conversion. |
Online Converters | Various websites allow you to input absolute paths to receive relative ones. |
Utilizing these tools can simplify the process and reduce the potential for errors.
Transforming Absolute Paths to Relative Paths: Expert Insights
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “Converting absolute paths to relative paths is essential for enhancing website portability and maintainability. It allows developers to move projects between environments without breaking links, which is particularly crucial in collaborative settings.”
James Liu (Software Architect, CodeCraft Solutions). “Utilizing relative paths not only simplifies the directory structure but also improves loading times. When assets are referenced relatively, the browser can resolve them more efficiently, leading to a better user experience.”
Sarah Thompson (Systems Analyst, Digital Pathways). “Incorporating relative paths into your coding practices reduces the risk of errors during deployment. It is a best practice that ensures your application remains functional regardless of the server or hosting environment.”
Frequently Asked Questions (FAQs)
What is the difference between an absolute path and a relative path?
An absolute path provides the complete location of a file or directory from the root of the file system, while a relative path specifies the location in relation to the current working directory.
How can I convert an absolute path to a relative path in a programming context?
To convert an absolute path to a relative path, you can use functions provided by programming languages, such as `os.path.relpath()` in Python, which calculates the relative path from one directory to another.
What tools can assist in rewriting absolute paths to relative paths?
Various tools and libraries, such as command-line utilities like `realpath`, or version control systems like Git, can assist in converting absolute paths to relative paths.
Are there any potential issues when using relative paths?
Yes, relative paths can lead to issues if the working directory changes or if the file structure is modified, potentially causing broken links or inaccessible files.
Can I manually rewrite an absolute path to a relative path?
Yes, you can manually rewrite an absolute path to a relative path by determining the common base directory and appending the necessary subdirectories to navigate to the target file or directory.
Is it possible to automate the process of converting paths in a project?
Yes, automation can be achieved through scripts or build tools that systematically convert absolute paths to relative paths, ensuring consistency across the project.
In summary, rewriting an absolute path to a relative path is a crucial skill in various fields such as web development, software engineering, and data management. An absolute path specifies the complete location of a file or directory in relation to the root directory, while a relative path defines the location in relation to the current working directory. Understanding the distinction between these two types of paths is essential for effective file management and navigation within systems.
One of the key insights from the discussion is the practicality of using relative paths for portability and flexibility. Relative paths allow for easier movement of projects across different environments without the need to modify file references. This is particularly beneficial in collaborative projects, where team members may have different directory structures on their local machines. By adopting relative paths, developers can ensure that their code remains functional regardless of the specific file system layout.
Moreover, the process of converting absolute paths to relative paths involves understanding the directory structure and the current location of the working directory. This conversion not only enhances the maintainability of code but also improves the overall user experience by reducing potential errors related to file access. As such, mastering this skill can significantly streamline workflows and contribute to more efficient project management.
Author Profile
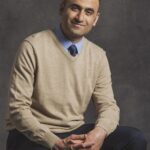
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?