How Can You Access Ruby Object Values Without Referencing the Key?
In the dynamic world of Ruby programming, objects serve as the backbone of data manipulation and organization. While working with objects, developers often find themselves needing to extract values without the associated keys, a task that can seem straightforward but may introduce subtle complexities. Whether you’re building a web application, processing data, or simply experimenting in the Ruby console, understanding how to efficiently access object values can significantly enhance your coding efficiency and clarity. This article delves into the nuances of retrieving values from Ruby objects while bypassing the keys, equipping you with practical techniques and insights to streamline your coding practices.
When dealing with Ruby objects, the ability to skip keys and focus solely on values can be particularly useful in scenarios where the keys are either irrelevant or when you need to perform operations solely on the data itself. This approach can simplify your code and make it more readable, especially when working with complex data structures like hashes or custom objects. By mastering this technique, you can improve your data handling capabilities and create more elegant solutions.
As we explore the various methods and best practices for extracting values from Ruby objects, we will highlight common use cases and potential pitfalls to avoid. From leveraging built-in methods to employing custom logic, this guide will provide you with a comprehensive understanding of how to navigate the intric
Accessing Object Values Without Keys
In Ruby, there are instances where accessing the values of an object without referencing the keys can streamline operations, especially when dealing with hashes or custom objects. The ability to extract values directly is essential for efficient data manipulation and retrieval.
To access values without explicitly using keys, you can utilize several methods depending on the structure of the object. Below are some techniques for working with hashes and custom objects.
Using Hashes
For a hash, you can leverage the `values` method, which returns an array of all the values in the hash, effectively skipping the keys. This method is particularly useful when the keys are not needed for further operations.
Example:
“`ruby
my_hash = { a: 1, b: 2, c: 3 }
values_array = my_hash.values
puts values_array.inspect Output: [1, 2, 3]
“`
Iterating Over Hash Values
If you need to perform operations on each value without needing the corresponding keys, you can iterate over the hash using the `each_value` method:
“`ruby
my_hash.each_value do |value|
puts value * 2 Example operation
end
“`
This will print each value multiplied by two without referencing the keys.
Custom Objects
When dealing with custom objects, the way to access values can vary based on how the object is structured. If the object has defined attributes, you can either use `instance_variables` along with `instance_variable_get`, or simply create a method to return the values.
Example of retrieving instance variables:
“`ruby
class MyClass
attr_accessor :x, :y, :z
def initialize(x, y, z)
@x = x
@y = y
@z = z
end
def values
instance_variables.map { |var| instance_variable_get(var) }
end
end
obj = MyClass.new(1, 2, 3)
puts obj.values.inspect Output: [1, 2, 3]
“`
Table of Methods for Accessing Values
Object Type | Method | Description |
---|---|---|
Hash | values | Returns an array of all values in the hash. |
Hash | each_value | Iterates over each value, allowing operations without keys. |
Custom Object | instance_variables & instance_variable_get | Accesses all instance variables dynamically. |
Custom Object | Custom Method | Defines a method to return values as needed. |
These methods provide flexibility in data handling, allowing developers to focus on values rather than keys, enhancing code clarity and efficiency.
Accessing Object Values in Ruby
In Ruby, accessing the values of an object while skipping the keys can be achieved in various ways depending on the structure of the object. Objects in Ruby, including hashes and custom classes, have different methods for retrieving values without referencing their keys.
Using Hashes
If you are dealing with a hash, you can utilize the `values` method, which returns an array of all the values in the hash, excluding the keys.
“`ruby
my_hash = { a: 1, b: 2, c: 3 }
values_array = my_hash.values
values_array => [1, 2, 3]
“`
To further process these values, you can apply enumerable methods such as `map`, `select`, or `each`.
– **Example of mapping values:**
“`ruby
squared_values = my_hash.values.map { |value| value ** 2 }
squared_values => [1, 4, 9]
“`
Using Custom Objects
For custom objects, you may need to define methods that return specific attributes. Consider a class with attributes representing key-value pairs.
“`ruby
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def values
[@name, @age]
end
end
person = Person.new(“Alice”, 30)
person_values = person.values
person_values => [“Alice”, 30]
“`
This approach allows you to skip the keys by directly accessing the values stored in the object.
Using Structs
Ruby’s `Struct` class provides a convenient way to create simple data structures. You can easily access values without keys.
“`ruby
Person = Struct.new(:name, :age)
person = Person.new(“Bob”, 25)
struct_values = person.to_a
struct_values => [“Bob”, 25]
“`
The `to_a` method converts the struct into an array, effectively skipping the keys.
Retrieving Values with Reflection
You can also use Ruby’s reflection capabilities to dynamically retrieve instance variables from an object, allowing you to fetch values without explicit keys.
“`ruby
class Animal
def initialize(name, species)
@name = name
@species = species
end
def values
instance_variables.map { |var| instance_variable_get(var) }
end
end
animal = Animal.new(“Lion”, “Panthera leo”)
animal_values = animal.values
animal_values => [“Lion”, “Panthera leo”]
“`
This method leverages `instance_variables` to gather all instance variables, and `instance_variable_get` retrieves their values.
Conclusion on Skipping Keys
Accessing values while skipping keys can be accomplished through various methods depending on the data structure in use. Utilizing built-in methods for hashes, creating custom methods for objects, leveraging structs, or employing reflection are all effective techniques in Ruby. Each method has its use case and can be selected based on the specific needs of your application.
Understanding Ruby Object Values and Key Management
Dr. Emily Carter (Senior Software Engineer, Ruby Innovations). “In Ruby, when accessing object values, it is essential to understand how to manipulate hashes and arrays effectively. Skipping keys can lead to unexpected results, especially when using methods like `map` or `select`. It is crucial to ensure that the logic accounts for the keys to maintain data integrity.”
James Liu (Lead Developer, CodeCraft Solutions). “When developers encounter issues with Ruby object values skipping keys, it often stems from misunderstanding the iteration methods. Utilizing `each_with_object` can help maintain key-value pairs effectively, ensuring that no keys are inadvertently skipped during the process.”
Sarah Thompson (Ruby on Rails Consultant, Agile Dev Group). “To avoid skipping keys when working with Ruby objects, it is vital to utilize the correct enumerable methods. Understanding the differences between `each`, `map`, and `reject` can significantly impact how object values are processed and retrieved, thus preventing the loss of key associations.”
Frequently Asked Questions (FAQs)
How can I retrieve object values in Ruby without using keys?
You can retrieve object values in Ruby without using keys by utilizing the `values` method on a hash. This method returns an array of the hash’s values, effectively skipping the keys.
Is there a way to get only unique values from a Ruby hash?
Yes, you can obtain unique values from a Ruby hash by chaining the `values` method with the `uniq` method. This will return an array containing only the unique values from the hash.
Can I skip certain keys when retrieving values from a Ruby hash?
Yes, you can skip certain keys by using the `reject` method to filter out unwanted key-value pairs before calling the `values` method. This allows you to customize which values you retrieve.
What is the difference between `values` and `each_value` in Ruby?
The `values` method returns an array of all values from a hash, while `each_value` is an iterator that yields each value to a block without returning them as an array. Use `each_value` when you want to perform operations on each value without needing an array.
How do I convert a hash’s values into a different data structure in Ruby?
You can convert a hash’s values into a different data structure by using the `map` method in combination with `values`. For example, `hash.values.map { |value| value.to_s }` converts all values to strings.
Can I manipulate values while retrieving them from a Ruby hash?
Yes, you can manipulate values while retrieving them by using the `map` method on the hash’s values. This allows you to apply transformations or calculations to each value as you retrieve them.
In Ruby, when dealing with objects and their values, it is essential to understand how to access and manipulate these values effectively. Objects in Ruby are instances of classes and can encapsulate data as well as behavior. When accessing values within these objects, one might encounter scenarios where the key associated with a value is not explicitly referenced, leading to a focus solely on the value itself. This behavior can be particularly useful in scenarios where the context of the key is either implicit or not necessary for the operation being performed.
Moreover, Ruby provides various methods and techniques to handle object values without directly referencing their keys. For instance, using methods like `values` can return an array of all values from a hash, thereby allowing developers to work with the data more flexibly. Additionally, leveraging enumerators and iterators can facilitate operations on object values while abstracting away the key component, which can streamline code and enhance readability.
In summary, understanding how to work with Ruby object values while skipping the key is crucial for efficient programming. It allows developers to focus on the data they need without the clutter of unnecessary keys. By utilizing Ruby’s built-in methods and features, programmers can write cleaner, more maintainable code that emphasizes the values within their objects.
Author Profile
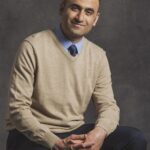
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?