How Can I Run a BAT File in PowerShell?
In the world of Windows scripting, the ability to seamlessly execute batch files can significantly enhance your productivity and streamline your workflows. PowerShell, Microsoft’s powerful task automation framework, offers a robust environment for executing scripts, but many users often wonder how to effectively run traditional batch files (.bat) within this modern shell. Whether you’re looking to automate repetitive tasks, manage system configurations, or simply execute legacy scripts, understanding the nuances of running batch files in PowerShell can unlock a new level of efficiency.
As you delve into the intricacies of running batch files in PowerShell, you’ll discover that the process is not only straightforward but also packed with potential for advanced scripting techniques. PowerShell provides unique features that can complement and enhance the functionality of batch files, allowing you to leverage both tools for optimal results. This article will guide you through the essential steps and considerations, ensuring you can confidently execute your batch scripts within the PowerShell environment.
From understanding the differences between PowerShell and batch scripting to exploring the various methods for execution, this comprehensive overview will equip you with the knowledge needed to integrate batch files into your PowerShell workflows. Whether you are a novice user or an experienced scripter, the insights shared here will pave the way for more efficient and powerful script execution.
Executing a Batch File in PowerShell
To execute a batch file from PowerShell, you can simply call the batch file by its name, ensuring that the path to the file is correct if it’s not located in the current directory. PowerShell treats batch files as executable scripts, allowing you to run them seamlessly.
- Basic Command Structure:
“`powershell
.\YourBatchFile.bat
“`
- Using the Full Path: If the batch file is located in a different directory, specify the full path:
“`powershell
C:\Path\To\YourBatchFile.bat
“`
- Running with Arguments: If your batch file requires arguments, you can pass them directly:
“`powershell
.\YourBatchFile.bat arg1 arg2
“`
Common Issues and Solutions
When running batch files in PowerShell, you may encounter several common issues. Below are some of the issues and their respective solutions.
Issue | Description | Solution |
---|---|---|
Execution Policy | PowerShell may restrict script execution. | Change the policy using `Set-ExecutionPolicy`. |
File Not Found | The specified path to the batch file is incorrect. | Verify the path and file name. |
Permissions | Insufficient permissions to execute the batch file. | Run PowerShell as an administrator. |
Using Start-Process for Batch Files
For more control over the execution of batch files, consider using the `Start-Process` cmdlet. This cmdlet allows you to start a process with various options.
- Basic Syntax:
“`powershell
Start-Process “C:\Path\To\YourBatchFile.bat”
“`
- Running in a New Window: You can also specify that the batch file should run in a new window:
“`powershell
Start-Process “C:\Path\To\YourBatchFile.bat” -NoNewWindow
“`
- Redirecting Output: To capture the output of the batch file, you can redirect it to a file:
“`powershell
Start-Process “C:\Path\To\YourBatchFile.bat” -RedirectStandardOutput “C:\Path\To\Output.txt”
“`
Debugging Batch Files in PowerShell
Debugging a batch file in PowerShell can be more effective by using logging. You can modify the batch file to include echo commands to track progress or errors.
– **Example of Adding Logging**:
“`batch
@echo off
echo Starting Process > C:\Path\To\Log.txt
REM Your commands here
echo Process Finished >> C:\Path\To\Log.txt
“`
This method helps you trace what the batch file is doing and identify any potential points of failure when executed from PowerShell.
Running a BAT File in PowerShell
To execute a BAT file within PowerShell, you can utilize a straightforward command that invokes the script. Below are various methods to achieve this.
Using the Call Operator
The call operator (`&`) can be used to run a BAT file. This is a direct and effective way to execute the script.
“`powershell
& “C:\Path\To\Your\Script.bat”
“`
- Ensure the path to the BAT file is correct.
- The quotes are necessary if the path contains spaces.
Using Start-Process Cmdlet
The `Start-Process` cmdlet is another method for running BAT files. This cmdlet provides additional parameters for enhanced control over the execution.
“`powershell
Start-Process “C:\Path\To\Your\Script.bat”
“`
Parameters:
- `-NoNewWindow`: Run the BAT file in the same window.
- `-Wait`: Wait for the process to complete before proceeding to the next command.
Example:
“`powershell
Start-Process “C:\Path\To\Your\Script.bat” -NoNewWindow -Wait
“`
Using the Invoke-Expression Cmdlet
`Invoke-Expression` can also be used, though it is less common for running BAT files. This cmdlet evaluates a string as a command.
“`powershell
Invoke-Expression “C:\Path\To\Your\Script.bat”
“`
Setting Execution Policies
In some cases, you may encounter restrictions due to execution policies. To check the current policy, use:
“`powershell
Get-ExecutionPolicy
“`
To temporarily allow script execution, you can set the policy:
“`powershell
Set-ExecutionPolicy -ExecutionPolicy Bypass -Scope Process
“`
This command allows you to bypass restrictions for the current session only.
Common Issues and Troubleshooting
Here are potential issues you might face while executing a BAT file and their solutions:
Issue | Solution |
---|---|
File not found | Verify the file path and ensure the file exists. |
Execution policy error | Adjust the execution policy as described above. |
Permission denied | Run PowerShell as an administrator if needed. |
Command not recognized | Ensure the BAT file is correctly formatted and accessible. |
Example Script
Consider a simple BAT file named `example.bat` with the following content:
“`bat
@echo off
echo Hello, World!
pause
“`
To run this file from PowerShell, you would use:
“`powershell
& “C:\Path\To\example.bat”
“`
This command will execute the BAT file and display “Hello, World!” in the PowerShell window.
Executing BAT files in PowerShell can enhance automation and scripting efficiency. The methods outlined above offer flexibility depending on your needs and specific scenarios.
Expert Insights on Running BAT Files in PowerShell
Dr. Emily Carter (Systems Administrator, Tech Solutions Inc.). “Running a BAT file in PowerShell is straightforward, but it is crucial to understand the context in which you are executing the script. PowerShell can run BAT files directly by calling the file’s path, but you may need to adjust execution policies depending on your system’s security settings.”
James Liu (Senior Software Engineer, CodeCraft Labs). “When invoking a BAT file from PowerShell, it is essential to use the correct syntax. The command should be prefixed with `&` to ensure that PowerShell recognizes it as a command rather than a string. This small detail can save you from unnecessary troubleshooting.”
Maria Gonzalez (IT Security Consultant, SecureTech Group). “While running BAT files in PowerShell can be efficient, it is important to be aware of potential security risks. Always verify the source of the BAT file and consider running it in a controlled environment to mitigate any threats that may arise from executing unknown scripts.”
Frequently Asked Questions (FAQs)
How do I run a .bat file in PowerShell?
To run a .bat file in PowerShell, use the command `& “C:\path\to\your\file.bat”`, replacing the path with the actual location of your .bat file.
Can I run a .bat file with administrative privileges in PowerShell?
Yes, you can run a .bat file with administrative privileges by opening PowerShell as an administrator and then executing the command to run the .bat file.
What happens if I run a .bat file in PowerShell?
When you run a .bat file in PowerShell, it executes the commands contained within the batch file, just as it would in the Command Prompt.
Are there any differences between running a .bat file in Command Prompt and PowerShell?
Yes, while both environments can execute .bat files, PowerShell has additional features and cmdlets that may not be available in Command Prompt, affecting how certain commands are processed.
Can I pass arguments to a .bat file when running it in PowerShell?
Yes, you can pass arguments to a .bat file in PowerShell by including them after the file path, like this: `& “C:\path\to\your\file.bat” arg1 arg2`.
What if the .bat file fails to run in PowerShell?
If a .bat file fails to run in PowerShell, check for syntax errors in the script, ensure that the file path is correct, and verify that you have the necessary permissions to execute the file.
Running a batch file in PowerShell is a straightforward process that allows users to execute commands and scripts written in the traditional batch file format. PowerShell, being a more advanced command-line interface, provides enhanced capabilities compared to the Command Prompt. Users can invoke a batch file by simply calling its path, ensuring that the execution context is correctly set up to handle the commands contained within the file.
One important aspect to consider is the execution policy in PowerShell, which may affect the ability to run scripts and batch files. Users should ensure that their execution policy allows for script execution, which can be checked and modified using the `Get-ExecutionPolicy` and `Set-ExecutionPolicy` cmdlets. Additionally, it is essential to use the correct syntax when calling the batch file, which typically involves using the `&` operator or invoking it directly by its path.
In summary, running a batch file in PowerShell is a valuable skill that can enhance productivity by leveraging existing scripts within a more powerful environment. Understanding the nuances of execution policies and syntax will empower users to effectively integrate batch files into their PowerShell workflows. This knowledge not only facilitates seamless execution but also opens the door to more complex automation tasks that can be accomplished through the combination of
Author Profile
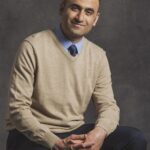
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?