How Can I Run a Batch Script from PowerShell?
In the world of Windows automation, PowerShell stands out as a powerful tool that allows users to streamline tasks and enhance productivity. However, many users may not realize that they can also leverage the capabilities of traditional batch scripts within PowerShell. Whether you’re a seasoned IT professional or a curious beginner, understanding how to run batch scripts from PowerShell can open up a realm of possibilities for automating repetitive tasks, managing system configurations, and executing complex workflows with ease.
Running batch scripts from PowerShell is not only a practical skill but also a gateway to integrating legacy scripts into modern workflows. This process allows users to harness the simplicity of batch commands while benefiting from PowerShell’s advanced features. By combining these two scripting environments, you can create more robust and flexible automation solutions that cater to a variety of needs, from simple file manipulations to intricate system management tasks.
As you delve deeper into this topic, you’ll uncover the nuances of executing batch files, understanding the command syntax, and troubleshooting common issues that may arise. Whether you’re looking to enhance your scripting repertoire or seeking to optimize existing processes, mastering the art of running batch scripts from PowerShell is an essential step towards achieving greater efficiency and control in your computing environment.
Running a Batch Script from PowerShell
To execute a batch script from PowerShell, you can utilize the `Start-Process` cmdlet or simply call the batch file directly. Both methods are straightforward and allow for various options to customize the execution.
Using Start-Process Cmdlet
The `Start-Process` cmdlet is beneficial when you want to run the batch script in a separate window or control its execution options. Here’s how to use it:
“`powershell
Start-Process “C:\Path\To\YourScript.bat”
“`
This command initiates the batch script in its own command prompt window. You can also pass additional parameters such as:
- -ArgumentList: To provide arguments to the batch file.
- -NoNewWindow: To run the script in the current PowerShell session without opening a new window.
- -Wait: To make PowerShell wait until the batch script has completed before moving on to the next command.
Example of using these parameters:
“`powershell
Start-Process “C:\Path\To\YourScript.bat” -ArgumentList “arg1”, “arg2” -NoNewWindow -Wait
“`
Calling the Batch File Directly
Another method is simply to call the batch file directly from the PowerShell command line. This is often the simplest approach:
“`powershell
& “C:\Path\To\YourScript.bat”
“`
The ampersand (`&`) is the call operator in PowerShell, which allows you to run commands or scripts that are not cmdlets. This method will run the script in the current session and is useful for quick executions without additional parameters.
Considerations When Running Scripts
When executing batch scripts, there are several considerations to keep in mind:
- Execution Policy: Ensure that the PowerShell execution policy allows script execution. You may need to set it using:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
- Path: Make sure that the path to the batch file is correct. If the path includes spaces, enclose it in quotes.
- Permissions: Verify that you have the necessary permissions to execute the script.
- Environment Variables: If the batch script relies on certain environment variables, ensure they are set appropriately in the PowerShell session.
Example Table of Commands
Command | Description |
---|---|
Start-Process “C:\Path\To\YourScript.bat” | Runs the batch script in a new window. |
Start-Process “C:\Path\To\YourScript.bat” -NoNewWindow | Runs the script in the current PowerShell window. |
& “C:\Path\To\YourScript.bat” | Calls the batch script directly in the current session. |
By understanding these methods and considerations, you can effectively run batch scripts from PowerShell, making it a powerful tool for automation and scripting tasks.
Running a Batch Script in PowerShell
To execute a batch script from PowerShell, use the `Start-Process` cmdlet or simply call the script directly. Here are the two main methods for running a batch file.
Using Start-Process Cmdlet
The `Start-Process` cmdlet provides a straightforward way to run a batch file while allowing for more options, such as running it as a different user or specifying the working directory.
Syntax:
“`powershell
Start-Process -FilePath “C:\Path\To\YourScript.bat”
“`
Example:
“`powershell
Start-Process -FilePath “C:\Scripts\example.bat”
“`
Parameters:
- `-FilePath`: Specifies the path to the batch file.
- `-WorkingDirectory`: Sets the working directory for the process.
- `-ArgumentList`: Passes arguments to the batch file if needed.
Directly Calling the Batch Script
You can also run a batch script directly by specifying its path. This method is simpler but does not offer the same level of control as `Start-Process`.
Syntax:
“`powershell
& “C:\Path\To\YourScript.bat”
“`
Example:
“`powershell
& “C:\Scripts\example.bat”
“`
Handling Arguments
If your batch script requires arguments, you can pass them using either method.
Using Start-Process with Arguments:
“`powershell
Start-Process -FilePath “C:\Scripts\example.bat” -ArgumentList “arg1”, “arg2”
“`
Direct Call with Arguments:
“`powershell
& “C:\Scripts\example.bat” “arg1” “arg2”
“`
Running Scripts in the Background
To run a batch file in the background, you can utilize the `-NoNewWindow` parameter with `Start-Process`. This allows the script to execute without opening a new command prompt window.
Example:
“`powershell
Start-Process -FilePath “C:\Scripts\example.bat” -NoNewWindow
“`
Examples of Use Cases
Use Case | Command Example |
---|---|
Run a simple batch script | `& “C:\Scripts\example.bat”` |
Run with arguments | `& “C:\Scripts\example.bat” “arg1” “arg2″` |
Run in background | `Start-Process -FilePath “C:\Scripts\example.bat” -NoNewWindow` |
Set working directory | `Start-Process -FilePath “C:\Scripts\example.bat” -WorkingDirectory “C:\Scripts”` |
Error Handling
To capture errors when executing batch scripts, you can use `try-catch` blocks in PowerShell. This method helps you manage exceptions and take action based on the error encountered.
Example:
“`powershell
try {
& “C:\Scripts\example.bat”
} catch {
Write-Host “An error occurred: $_”
}
“`
Utilizing PowerShell to execute batch scripts enhances automation capabilities within Windows environments. Whether using `Start-Process` for more control or calling the script directly, both methods offer flexibility for managing batch operations effectively.
Expert Insights on Running Batch Scripts from PowerShell
Dr. Emily Carter (Senior Systems Engineer, Tech Innovations Inc.). “Running batch scripts from PowerShell is a powerful way to leverage the capabilities of both environments. It allows users to automate complex tasks while maintaining the flexibility of PowerShell’s advanced scripting features. Properly structuring the command ensures smooth execution and error handling.”
Michael Chen (IT Automation Specialist, Cloud Solutions Group). “To effectively run batch scripts from PowerShell, one must understand the nuances of command execution. Utilizing the `Start-Process` cmdlet can provide better control over the execution environment, allowing for parameters and working directories to be specified, which enhances script reliability.”
Lisa Thompson (Windows Scripting Expert, Scripting World Magazine). “Integrating batch scripts within PowerShell scripts can significantly streamline workflows. It is essential to consider the context in which the batch script is executed, especially regarding permissions and environment variables, to avoid common pitfalls.”
Frequently Asked Questions (FAQs)
How do I run a batch script from PowerShell?
To run a batch script from PowerShell, use the command `& “C:\path\to\your\script.bat”`. Make sure to replace the path with the actual location of your batch file.
Can I pass arguments to a batch script when running it from PowerShell?
Yes, you can pass arguments by appending them after the script path, like this: `& “C:\path\to\your\script.bat” arg1 arg2`.
What should I do if my batch script requires administrative privileges?
If your batch script requires administrative privileges, you can run PowerShell as an administrator by right-clicking the PowerShell icon and selecting “Run as administrator” before executing the script.
Are there any differences in how PowerShell handles batch scripts compared to Command Prompt?
Yes, PowerShell may handle certain commands and environment variables differently than Command Prompt. It is advisable to test scripts in both environments if compatibility is a concern.
Can I schedule a batch script to run from PowerShell?
Yes, you can schedule a batch script using the Task Scheduler in Windows. Create a new task and set the action to run PowerShell with the command to execute your batch script.
What should I do if my batch script does not execute properly from PowerShell?
If the batch script does not execute properly, check for syntax errors, ensure the script path is correct, and verify that all required permissions are granted. Additionally, consider running the script directly in Command Prompt for troubleshooting.
running a batch script from PowerShell is a straightforward process that can enhance automation and streamline workflows. Users can execute batch files by using the `Start-Process` cmdlet or simply calling the batch file directly within the PowerShell environment. This flexibility allows for seamless integration of legacy batch scripts with modern PowerShell capabilities, enabling users to leverage both scripting languages effectively.
Additionally, it is important to consider the execution policy settings in PowerShell, as these may affect the ability to run scripts. Users should ensure that the appropriate permissions and policies are in place to avoid any interruptions. Furthermore, understanding the differences between PowerShell and batch scripting can help users optimize their scripts for better performance and reliability.
Ultimately, mastering the execution of batch scripts from PowerShell not only enhances productivity but also empowers users to create more complex and efficient automation solutions. By combining the strengths of both scripting languages, users can achieve greater control over their computing environments and streamline repetitive tasks.
Author Profile
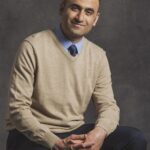
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?