How Can I Schedule a Cron Job to Run Every Two Days in Spring Boot?
In the fast-paced world of software development, automating tasks is key to enhancing productivity and ensuring efficiency. For Java developers using Spring Boot, the ability to schedule tasks seamlessly is a game changer. One common requirement is to run a cron job every two days, a task that may seem daunting at first but can be easily achieved with the right approach. Whether you’re managing data backups, sending out regular notifications, or performing routine maintenance, mastering the art of scheduling in Spring Boot can elevate your application’s functionality and reliability.
Overview
Spring Boot provides a robust framework for building applications with built-in support for task scheduling. By leveraging the powerful `@Scheduled` annotation, developers can define when and how often tasks should run, including the ability to execute jobs at specified intervals. Understanding the syntax and configuration options available for cron expressions is essential for setting up tasks that run every two days, allowing for precise control over execution timing.
Moreover, integrating cron jobs into your Spring Boot application not only streamlines processes but also enhances the overall user experience. As you delve deeper into the specifics of scheduling tasks, you’ll discover various strategies to optimize your application’s performance, manage resources effectively, and ensure that your scheduled jobs run smoothly without impacting the application’s responsiveness. With the right knowledge,
Using Scheduled Tasks in Spring Boot
To run a cron job every two days in a Spring Boot application, you can leverage the `@Scheduled` annotation along with a cron expression. The `@Scheduled` annotation enables you to schedule tasks at fixed intervals or at specific times.
Cron Expression for Every Two Days
A cron expression consists of six or seven fields that define the schedule. For running a job every two days, the expression can be defined as follows:
“`
0 0 0 */2 * *
“`
This expression breaks down into:
- `0` – The second (0 seconds).
- `0` – The minute (0 minutes).
- `0` – The hour (midnight).
- `*/2` – Every 2 days.
- `*` – Every month.
- `*` – Every day of the week.
Implementing the Scheduled Task
You need to create a service class in your Spring Boot application where you will define the scheduled task. Here is an example:
“`java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
@Service
public class MyScheduledTask {
@Scheduled(cron = “0 0 0 */2 * *”)
public void executeTask() {
// Task logic here
System.out.println(“Executing task every two days”);
}
}
“`
Enabling Scheduling in Spring Boot
To enable scheduling in your Spring Boot application, add the `@EnableScheduling` annotation to your main application class or any configuration class. Here’s how to do it:
“`java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
“`
Additional Considerations
- Time Zone: Cron jobs run based on the server’s time zone. Ensure your server’s time zone is configured correctly or specify a time zone in your cron expression if needed.
- Concurrency: By default, scheduled tasks are executed sequentially. If you need concurrent executions, consider configuring a task scheduler.
Task Scheduler Configuration
You can customize the task scheduler using a configuration class. Here’s an example of how to define a custom task scheduler:
“`java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableAsync;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler;
@Configuration
@EnableScheduling
@EnableAsync
public class SchedulerConfig {
@Bean
public ThreadPoolTaskScheduler taskScheduler() {
ThreadPoolTaskScheduler scheduler = new ThreadPoolTaskScheduler();
scheduler.setPoolSize(5);
scheduler.setThreadNamePrefix(“scheduled-task-“);
return scheduler;
}
}
“`
Summary of Cron Expressions
Frequency | Cron Expression |
---|---|
Every minute | `0 * * * * *` |
Every hour | `0 0 * * * *` |
Every day at midnight | `0 0 0 * * *` |
Every two days | `0 0 0 */2 * *` |
Every week | `0 0 0 * * 0` |
By following the guidelines above, you can effectively schedule a task to run every two days in your Spring Boot application, leveraging the powerful scheduling capabilities provided by the framework.
Configuring Cron Jobs in Spring Boot
To run a cron job every two days in a Spring Boot application, you can utilize the `@Scheduled` annotation provided by the Spring framework. This annotation allows you to define the scheduling of tasks easily.
Using the @Scheduled Annotation
You can set up a scheduled task by annotating a method with `@Scheduled` and specifying the cron expression. The cron expression for running a task every two days can be defined as follows:
“`java
@Scheduled(cron = “0 0 0 */2 * *”)
public void executeTask() {
// Task implementation here
}
“`
Breakdown of the Cron Expression
The cron expression `0 0 0 */2 * *` can be broken down into six fields:
Field | Value | Description |
---|---|---|
Seconds | 0 | At second 0 |
Minutes | 0 | At minute 0 |
Hours | 0 | At midnight |
Day of Month | */2 | Every 2 days |
Month | * | Every month |
Day of Week | * | Every day of the week |
This configuration means that the scheduled task will run at midnight every second day.
Enabling Scheduling in Spring Boot
To enable scheduling in your Spring Boot application, you need to add the `@EnableScheduling` annotation to one of your configuration classes. Here’s how you can do it:
“`java
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableScheduling;
@Configuration
@EnableScheduling
public class SchedulerConfig {
// Configuration settings, if any
}
“`
This annotation instructs Spring to look for methods annotated with `@Scheduled` and manage their execution according to the defined cron expressions.
Testing the Scheduled Task
To verify that your scheduled task is running correctly:
- Log Output: Add logging statements within the `executeTask` method to track its execution.
- Mocking Time: Use libraries like `Mockito` or `Awaitility` to simulate and test the execution of tasks based on time.
Handling Time Zones
If your application runs in multiple time zones or if you need to specify a time zone for the cron job, you can include the `zone` attribute in the `@Scheduled` annotation:
“`java
@Scheduled(cron = “0 0 0 */2 * *”, zone = “America/New_York”)
public void executeTask() {
// Task implementation here
}
“`
This ensures that the task runs according to the specified time zone, providing flexibility in global applications.
Considerations for Long-Running Tasks
When implementing scheduled tasks that may take a significant amount of time, consider the following:
- Concurrency: Use the `@Scheduled(fixedDelay = …)` option if the task should not overlap.
- Error Handling: Implement proper exception handling to prevent the scheduler from failing silently.
- Monitoring: Utilize monitoring tools or metrics (like Micrometer) to track the performance and execution of your scheduled tasks.
By following these guidelines, you can effectively set up a cron job that runs every two days in your Spring Boot application, ensuring reliable and efficient task management.
Strategies for Scheduling Cron Jobs in Spring Boot
Jessica Tran (Senior Software Engineer, Cloud Solutions Inc.). “To run a cron job every two days in Spring Boot, you can utilize the `@Scheduled` annotation with a cron expression. The expression ‘0 0 0 */2 * *’ will schedule the task to execute at midnight every two days. This method is efficient and integrates seamlessly with Spring’s scheduling capabilities.”
Michael Chen (DevOps Specialist, Agile Tech Group). “When configuring cron jobs in Spring Boot, it is crucial to ensure that the server time zone aligns with your scheduling needs. Using the `@Scheduled` annotation with the correct cron expression allows for precise control over execution timing. For a two-day interval, the cron expression ‘0 0 0 */2 * *’ is recommended, but testing in a staging environment is advisable to confirm the behavior meets expectations.”
Laura Simmons (Lead Architect, Innovative Software Solutions). “Incorporating cron jobs into your Spring Boot application can enhance task automation. To schedule a job every two days, the cron expression ‘0 0 0 */2 * *’ is effective. However, developers should also consider using Spring’s `TaskScheduler` for more complex scheduling needs, allowing for dynamic adjustments and better resource management in production environments.”
Frequently Asked Questions (FAQs)
How can I schedule a task to run every two days in Spring Boot?
You can use the `@Scheduled` annotation in Spring Boot along with a cron expression. For every two days, the cron expression would be `0 0 0 */2 * *`, which means the task will execute at midnight every second day.
What is the purpose of the `@Scheduled` annotation in Spring Boot?
The `@Scheduled` annotation is used to indicate that a method should be executed on a scheduled basis. It allows for easy scheduling of tasks at fixed intervals or specific times using cron expressions.
Can I configure the scheduling properties in an external configuration file?
Yes, you can define scheduling properties in the `application.properties` or `application.yml` file. You can specify the cron expression as a property and refer to it in the `@Scheduled` annotation.
What dependencies do I need to use scheduling in Spring Boot?
You need to include the `spring-boot-starter` dependency in your `pom.xml` or `build.gradle`. Additionally, ensure that the `@EnableScheduling` annotation is present in your main application class to enable scheduling support.
Is it possible to run multiple scheduled tasks in Spring Boot?
Yes, you can define multiple methods with the `@Scheduled` annotation in the same class or across different classes. Each method can have its own scheduling configuration, including different cron expressions.
How can I handle exceptions in scheduled tasks in Spring Boot?
You can handle exceptions by wrapping the task logic in a try-catch block within the scheduled method. Additionally, consider using a logging framework to log exceptions for monitoring and debugging purposes.
In summary, scheduling a cron job to run every two days in a Spring Boot application can be efficiently achieved using the `@Scheduled` annotation. This annotation allows developers to specify a cron expression that defines the frequency of the task execution. The cron expression for running a job every two days can be formulated as `0 0 0 */2 * ?`, which indicates that the job will execute at midnight every second day.
Implementing this functionality not only automates repetitive tasks but also enhances the overall efficiency of the application. By leveraging Spring Boot’s scheduling capabilities, developers can ensure that essential processes are executed reliably without manual intervention. This is particularly beneficial for tasks such as data cleanup, report generation, or any periodic maintenance work that needs to be performed on a regular basis.
Moreover, it is crucial to consider the implications of running tasks on a schedule, such as resource management and error handling. Proper logging and monitoring should be integrated to track the execution of scheduled tasks and to handle any potential failures gracefully. This proactive approach ensures that the application remains robust and responsive to any issues that may arise during the execution of scheduled jobs.
Author Profile
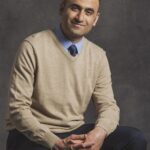
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?