How Can You Run a Python Script from Another Python Script?
In the world of programming, efficiency and modularity are key principles that can significantly enhance productivity and maintainability. Python, with its clean syntax and robust libraries, makes it easy to develop complex applications. However, as projects grow in size and complexity, developers often find themselves needing to execute one Python script from another. This practice not only streamlines workflows but also allows for better organization of code, enabling developers to build upon existing functionalities without reinventing the wheel. In this article, we will explore the various methods available for running a Python script within another Python script, shedding light on the advantages and best practices associated with this approach.
Running a Python script from another script can be accomplished through several techniques, each with its own use cases and benefits. Whether you want to execute a script as a subprocess, import functions and classes from another module, or leverage the versatility of the `exec()` function, Python provides multiple pathways to achieve your goals. Understanding these methods can help you make informed decisions about structuring your projects and enhancing code reusability.
Moreover, the ability to run scripts within scripts opens up a world of possibilities for automation and modular programming. By breaking down larger tasks into smaller, manageable scripts, developers can create a more organized codebase that is easier to debug and maintain
Using the subprocess Module
The `subprocess` module is a powerful tool that allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. This is commonly used to run a Python script from another Python script. You can execute a script as a separate process and manage its execution environment effectively.
To run a Python script using `subprocess`, you can use the following code snippet:
“`python
import subprocess
Specify the script to run
script_name = ‘script_to_run.py’
Run the script
result = subprocess.run([‘python’, script_name], capture_output=True, text=True)
Output the results
print(result.stdout)
print(result.stderr)
“`
In the above example, the `subprocess.run()` function is used, which executes the command specified in a list format. The `capture_output=True` argument captures the standard output and error of the script. The `text=True` argument ensures that the output is returned as a string instead of bytes.
Using exec() Function
Another approach to run a Python script from another script is by using the `exec()` function. This method executes the Python code contained in a script within the context of the calling script. Here’s how to do it:
“`python
script_name = ‘script_to_run.py’
with open(script_name) as f:
exec(f.read())
“`
This will read the content of `script_to_run.py` and execute it as if it were part of the calling script. However, this method does not create a new process, and any variables or functions defined in the executed script will be available in the calling script’s namespace.
Using import Statement
If the script you want to run is structured as a module (i.e., it contains functions or classes), you can simply import it and call its functions directly. Here’s an example:
“`python
import script_to_run
script_to_run.main() Assuming the script has a main() function
“`
This method is advantageous as it allows you to utilize functions and classes defined in the imported script, promoting code reusability.
Comparison of Methods
When deciding which method to use, consider the following table comparing the key attributes of each approach:
Method | Process | Namespace Isolation | Output Capture |
---|---|---|---|
subprocess | New process | Isolated | Yes |
exec() | Current process | Not isolated | No |
import | Current process | Not isolated | No |
Each method has its use cases, and the choice depends on the specific requirements of your project, including the need for output capture, process isolation, and code modularity.
Executing Python Scripts Using `import`
Importing another Python script allows you to use its functions, classes, and variables directly in your current script. This method is efficient for reusing code and maintaining modular design.
- Ensure both scripts are in the same directory or properly configure the Python path.
- Use the following syntax to import:
“`python
import script_name
“`
- You can access functions and classes as follows:
“`python
script_name.function_name()
“`
Using `subprocess` Module
The `subprocess` module provides a powerful interface for spawning new processes. This method is suitable when you need to run a script as a separate process.
- Basic usage:
“`python
import subprocess
subprocess.run([‘python’, ‘script_name.py’])
“`
- To capture output:
“`python
result = subprocess.run([‘python’, ‘script_name.py’], capture_output=True, text=True)
print(result.stdout)
“`
- You can also pass arguments to the script:
“`python
subprocess.run([‘python’, ‘script_name.py’, ‘arg1’, ‘arg2’])
“`
Using `exec()` Function
The `exec()` function can execute Python code dynamically. This approach is less common for running entire scripts but can be useful for executing small snippets.
- Example usage:
“`python
with open(‘script_name.py’) as file:
exec(file.read())
“`
- This method does not create a new namespace, so variables from the executed script are accessible afterward.
Utilizing `os.system()`
The `os` module provides a straightforward way to run shell commands, including Python scripts. This method is simple but does not offer advanced control over the execution environment.
- Example usage:
“`python
import os
os.system(‘python script_name.py’)
“`
- It is limited to shell capabilities and does not return output directly.
Considerations When Running Scripts
When deciding on the method to use, consider the following factors:
Method | Advantages | Disadvantages |
---|---|---|
`import` | Direct access to functions and classes | Must be in the same directory |
`subprocess` | Runs in a separate process | Slightly more complex syntax |
`exec()` | Dynamic execution of code | Risk of namespace conflicts |
`os.system()` | Simple and straightforward | Limited control and output handling |
Choosing the right method depends on the specific requirements of your project, including modularity, execution control, and the need for output handling.
Expert Insights on Running Python Scripts from Another Python Script
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To run a Python script from another Python script, the most straightforward approach is to use the `import` statement. This allows you to call functions and classes defined in one script from another, promoting code reusability and modular design.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “For scenarios where you need to execute a script as a separate process, the `subprocess` module is highly effective. It provides the ability to spawn new processes, connect to their input/output/error pipes, and obtain their return codes, which is essential for handling complex workflows.”
Linda Zhao (Python Instructor, Data Science Academy). “Using the `exec()` function can also be a viable option for running a Python script within another script’s context. However, it is crucial to be cautious with this method due to potential security risks associated with executing arbitrary code.”
Frequently Asked Questions (FAQs)
How can I run a Python script from another Python script?
You can use the `import` statement to run functions or classes from another script, or use the `subprocess` module to execute a script as a separate process.
What is the difference between using `import` and `subprocess`?
Using `import` allows you to access functions, classes, and variables directly within the current script, while `subprocess` runs the script in a separate process, which is useful for executing scripts independently.
Can I pass arguments to a Python script when using `subprocess`?
Yes, you can pass arguments by including them in the `subprocess.run()` or `subprocess.call()` function as a list. For example: `subprocess.run([‘python’, ‘script.py’, ‘arg1’, ‘arg2’])`.
Is it possible to capture the output of a script run with `subprocess`?
Yes, you can capture the output by setting the `stdout` parameter to `subprocess.PIPE`. This allows you to retrieve the output for further processing.
What should I consider regarding the working directory when running a script from another script?
Ensure that the working directory is set correctly, especially if the script relies on relative paths for files. You can change the working directory using `os.chdir()` before calling the subprocess.
Are there any performance implications when running a script from another script?
Yes, running a script as a subprocess incurs overhead due to process creation and context switching. For performance-critical applications, consider using `import` to directly access functions instead.
In summary, running a Python script from another Python script is a common practice that can enhance modularity and code reuse. Several methods exist to achieve this, including using the `import` statement, the `subprocess` module, and the `exec()` function. Each method has its own use cases, advantages, and limitations, allowing developers to choose the most appropriate approach based on their specific requirements.
The `import` statement is the most straightforward method, enabling the execution of functions and classes defined in another script without running the entire script. This is particularly useful for maintaining a clean structure and promoting code organization. On the other hand, the `subprocess` module allows for executing scripts as separate processes, which can be beneficial when isolation or parallel execution is needed. The `exec()` function can execute Python code dynamically but should be used with caution due to potential security risks.
Key takeaways include the importance of understanding the context in which each method is applicable. For instance, if you need to share functions or classes, importing is ideal. However, if you require a completely separate execution environment, the `subprocess` module is preferable. Additionally, developers should consider the implications of each method on performance, error handling, and maintainability
Author Profile
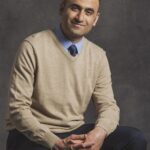
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?