How Can You Trace Multi-Line Strings in Rust?
In the realm of programming, effective debugging and logging are crucial for understanding the flow of an application and identifying issues. Rust, known for its performance and safety, offers powerful tools for tracing and logging, making it easier for developers to track the behavior of their code. However, when it comes to logging complex data structures, such as multi-line strings, developers often face unique challenges. In this article, we will explore how Rust handles tracing with multi-line strings, providing you with the insights needed to enhance your logging practices and improve your debugging experience.
Overview
Tracing in Rust involves capturing runtime information about your application, which can be invaluable for diagnosing problems and optimizing performance. Multi-line strings, in particular, present a distinct challenge due to their formatting and potential for cluttering logs. Understanding how to effectively manage and display these strings is essential for maintaining clarity in your logs and ensuring that your tracing output remains readable and informative.
As we delve deeper into the topic, we will discuss various techniques and best practices for logging multi-line strings in Rust. From leveraging built-in features to utilizing external libraries, we will equip you with the tools necessary to make your tracing output more effective. By mastering these techniques, you can elevate your debugging skills and gain deeper insights into your Rust applications
Understanding Tracing in Rust
Tracing in Rust is an essential tool for developers seeking to understand the flow of execution and the state of their applications. The `tracing` crate provides a way to instrument Rust programs to produce structured, contextual, and async-aware diagnostics. This is particularly useful for debugging and performance monitoring.
One of the features of the `tracing` crate is its ability to handle multi-line strings effectively. This is crucial when developers need to log long messages or complex data structures. Here’s how to work with multi-line strings in conjunction with `tracing`.
Logging Multi-Line Strings
In Rust, multi-line strings can be created using triple quotes (`”””`). However, when using `tracing`, the formatting of these strings should be considered to maintain readability and structure in logs.
To log multi-line strings, you can utilize the `info!`, `error!`, or `debug!` macros provided by the `tracing` crate. For instance:
“`rust
use tracing::{info, error};
fn log_multiline() {
let multi_line_message = r”
This is a multi-line log message.
It spans several lines, which can be very useful
for logging detailed information about the application’s state.
“;
info!(“{}”, multi_line_message);
}
“`
This approach ensures that the entire message is captured and formatted correctly in the logs.
Best Practices for Using Multi-Line Strings
When logging multi-line strings, consider the following best practices:
- Use Raw Strings: This prevents the need for escaping quotes or special characters.
- Keep Messages Concise: Even in multi-line logs, aim for clarity and brevity.
- Structured Logging: Consider using structured data formats (like JSON) when logging complex data.
Example of Structured Logging with Multi-Line Strings
Below is a structured logging example using `tracing` with a multi-line string.
“`rust
use tracing::{info, span, Level};
fn log_structured_data() {
let data = r”
{
“event”: “UserLogin”,
“username”: “example_user”,
“timestamp”: “2023-10-05T12:00:00Z”
}
“;
let log_span = span!(Level::INFO, “UserLoginEvent”);
let _enter = log_span.enter();
info!(“Event data: {}”, data);
}
“`
This example shows how to log structured data, which can be particularly useful for tracking events in a more machine-readable format.
Table of Logging Macros
Macro | Description |
---|---|
info! | Logs an informational message. |
error! | Logs an error message. |
debug! | Logs a debug message. |
warn! | Logs a warning message. |
Utilizing the `tracing` crate effectively allows for comprehensive logging practices, which enhance the overall maintainability and debugging capabilities of Rust applications. By adhering to the best practices outlined, developers can ensure their logs remain informative and structured, even when dealing with complex multi-line messages.
Using Tracing in Rust for Multi-Line Strings
When working with Rust, particularly in the context of logging and tracing, multi-line strings can present unique formatting challenges. The `tracing` crate, which is widely used for instrumenting Rust applications, provides a structured way to log messages, including those that span multiple lines.
Defining Multi-Line Strings
In Rust, multi-line strings can be defined using triple quotes `”””`. This allows for better readability and maintenance of string literals that contain line breaks. However, when integrating with the `tracing` library, it’s essential to format these strings appropriately to ensure they are logged correctly.
“`rust
let multi_line_string = r”
This is a multi-line string.
It can contain line breaks and special characters.
“;
“`
Logging Multi-Line Strings with Tracing
To effectively log multi-line strings using the `tracing` library, you can use the `info!`, `error!`, or other logging macros provided by `tracing`. The following example demonstrates how to log a multi-line string:
“`rust
use tracing::{info, error};
fn log_multi_line() {
let multi_line_string = r”
Error occurred while processing:
- Item 1: Failed due to network timeout
- Item 2: Invalid input provided
“;
error!(“{}”, multi_line_string);
}
“`
In this example, the `error!` macro logs the multi-line string, preserving its formatting.
Best Practices for Formatting
When logging multi-line strings, consider the following best practices to enhance clarity and maintainability:
- Use Raw String Literals: Utilize `r””` for raw strings to avoid escaping characters.
- Consistent Indentation: Maintain consistent indentation to improve readability.
- Structured Logging: If the multi-line string contains structured data, consider using JSON format for clarity.
Example of Structured Logging
For applications that require structured logging, converting multi-line strings to JSON can be beneficial. Here is an example of how to log a structured format:
“`rust
use serde_json::json;
use tracing::info;
fn log_as_json() {
let log_data = json!({
“error”: “Processing Error”,
“details”: [
“Item 1: Failed due to network timeout”,
“Item 2: Invalid input provided”
]
});
info!(“{}”, log_data);
}
“`
This approach allows for better parsing and filtering in log management tools.
Conclusion on Multi-Line String Logging
Incorporating multi-line strings into your logging strategy using Rust’s `tracing` library can improve the clarity of logs. By following structured logging practices and utilizing raw string literals, developers can ensure that their logs are both informative and easy to read.
Expert Insights on Rust Tracing for Multi-Line Strings
Dr. Emily Carter (Senior Software Engineer, Rustacean Insights). “In Rust, tracing multi-line strings can be efficiently managed by utilizing the `tracing` crate, which allows developers to create structured logs. By leveraging the `info!` macro, you can easily format and output multi-line strings, ensuring that your logs remain readable and informative.”
Michael Chen (Lead Developer, Open Source Projects). “When dealing with multi-line strings in Rust, it is crucial to remember that the `tracing` crate supports rich contextual information. By using the `span!` macro, you can capture the state of your application at various points, which is particularly useful when logging complex multi-line data.”
Sarah Patel (Technical Writer, Rust Programming Journal). “Effective logging of multi-line strings in Rust can significantly enhance debugging. The `tracing` framework allows for dynamic log levels and structured data, making it easier to trace the flow of multi-line string data through your application. This is essential for maintaining code clarity and performance.”
Frequently Asked Questions (FAQs)
What is the purpose of tracing in Rust?
Tracing in Rust is used for instrumenting applications, allowing developers to collect structured, contextual information about program execution. This facilitates debugging, performance monitoring, and understanding application behavior.
How do I create a multi-line string in Rust?
In Rust, a multi-line string can be created using triple quotes (`”””`), or by concatenating multiple string literals with the `+` operator or using the `format!` macro. The `r` prefix can also be used for raw strings to avoid escaping.
Can I include tracing information in multi-line strings?
Yes, you can include tracing information in multi-line strings by formatting the string with tracing data. Use the `format!` macro to embed dynamic tracing values within the string.
What libraries are commonly used for tracing in Rust?
Common libraries for tracing in Rust include `tracing`, `log`, and `slog`. The `tracing` library is particularly popular for its structured logging capabilities and support for asynchronous operations.
How can I visualize tracing data in Rust?
Tracing data in Rust can be visualized using tools like `tokio-console` or `tracing-subscriber`. These tools help in rendering the collected tracing information in a user-friendly format, making it easier to analyze and debug applications.
Is it possible to filter tracing output based on severity levels?
Yes, tracing output can be filtered based on severity levels such as `INFO`, `DEBUG`, `ERROR`, etc. This can be configured using the `tracing-subscriber` crate, allowing developers to focus on relevant logs during development and production.
In Rust, tracing multi-line strings can be effectively managed using the built-in capabilities of the language. The `tracing` crate offers a robust framework for instrumenting Rust applications, allowing developers to capture and log structured, contextual information about their code execution. When dealing with multi-line strings, proper formatting and handling are essential to ensure clarity and maintainability in logs.
One of the key aspects of tracing multi-line strings is the use of raw string literals, which allow for easier inclusion of line breaks and special characters without the need for escaping. This feature simplifies the process of logging complex messages or structured data, making it more readable. Additionally, developers can leverage formatting macros such as `format!` and `println!` to create well-structured log entries that can span multiple lines.
Moreover, utilizing the `tracing` crate’s features, such as spans and events, can enhance the logging of multi-line strings by providing context around the logged data. This context is crucial for debugging and performance monitoring, as it allows developers to trace the flow of execution and understand the state of the application at any given point. Overall, effective tracing of multi-line strings in Rust not only improves the quality of logs but also contributes to better application
Author Profile
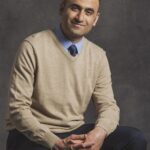
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?