Are You Curious About How to Monitor File Changes in a Sandbox Environment?
In the ever-evolving landscape of software development, efficiency and responsiveness are paramount. Developers often find themselves in a race against time, striving to deliver high-quality code while adapting to changing requirements. One of the most powerful tools at their disposal is the ability to monitor file changes in real-time, a feature that can significantly streamline workflows and enhance productivity. Enter the concept of a “sandbox” environment—an isolated space where developers can experiment, test, and refine their code without the fear of disrupting live applications. But what happens when you need to keep an eye on those file changes? This article delves into the intricacies of watching for file changes within a sandbox, exploring its importance, implementation, and the myriad benefits it brings to modern development practices.
At its core, watching for file changes is about creating a responsive development environment that reacts to modifications in real-time. This functionality allows developers to see the impact of their changes immediately, fostering a more iterative and agile approach to coding. Whether you’re working on a small project or a large-scale application, the ability to track file changes can lead to faster debugging, enhanced collaboration, and a more streamlined development process. As we explore this topic, we will touch upon the various tools and techniques available to implement file watching, as well as the
Understanding File Change Monitoring
File change monitoring is a critical feature in many development environments. It allows developers to automatically track changes in their files and trigger specific actions in response. This capability is especially useful in workflows that involve compilation, testing, or deployment. The process generally involves watching a directory for any modifications, additions, or deletions to files.
Key functionalities of file change monitoring include:
- Real-time Updates: Instant feedback on changes made to code or configuration files.
- Automation: Reducing the need for manual checks or commands, thus increasing efficiency.
- Error Reduction: Immediate identification of issues arising from code changes.
Common Tools for Watching File Changes
Various tools and libraries are available that facilitate file change monitoring. These tools can be integrated into development environments or used as standalone utilities. Some popular options include:
- Webpack: A module bundler that watches for changes in files and automatically recompiles them.
- Gulp: A task runner that can monitor file changes and execute predefined tasks.
- Nodemon: A utility that monitors for changes in Node.js applications and automatically restarts the server.
- File Watcher: A lightweight tool that triggers actions on file changes.
Configuration of File Watching
Configuring file watching can vary based on the tools being used. Below is a simple example of how to set up file watching using Gulp:
“`javascript
const gulp = require(‘gulp’);
gulp.task(‘watch’, function() {
gulp.watch(‘src/**/*.js’, gulp.series(‘scripts’));
});
“`
This configuration will monitor all JavaScript files in the `src` directory and execute the `scripts` task whenever a change is detected.
Performance Considerations
When implementing file watching, performance can be a concern, particularly in large projects with many files. Here are some considerations to ensure optimal performance:
- Limit Watched Files: Only watch files that are necessary for the current task or project.
- Debounce Changes: Implement a debounce mechanism to prevent multiple triggers from rapid changes.
- Use Efficient Libraries: Choose libraries known for their performance and reliability in monitoring file changes.
Tool | Language | Key Features |
---|---|---|
Webpack | JavaScript | Module bundling, hot reloading |
Gulp | JavaScript | Task automation, streaming builds |
Nodemon | JavaScript | Automatic server restarts |
File Watcher | Multiple | Lightweight, easy to configure |
By understanding these aspects of file change monitoring, developers can implement efficient workflows that enhance productivity and streamline their development processes.
Understanding File Change Monitoring in Sandbox Environments
Sandbox environments are designed to allow developers to test and experiment without affecting the main system. One of the essential features of these environments is the ability to monitor file changes, which can significantly enhance the development workflow.
File change monitoring typically involves the following components:
- File System Watchers: These are tools or libraries that monitor changes to files and directories. They alert developers when files are created, modified, or deleted.
- Event-Driven Architecture: Many sandbox environments use an event-driven model, where changes in the file system trigger events that can be handled by the application.
Common Tools for Monitoring File Changes
Several tools facilitate file change monitoring in sandbox environments. Below is a list of some of the most commonly used options:
- Chokidar: A Node.js library that provides a simple API for watching file changes in real-time.
- fsnotify: A Go library that offers file system notification capabilities.
- Watchman: Developed by Facebook, this tool watches for changes in files and can trigger actions based on those changes.
- inotify-tools: A set of command-line programs for Linux that utilize the inotify subsystem to monitor file system events.
Configuration and Setup
Setting up file change monitoring in a sandbox environment typically involves configuring the chosen tool to watch specific directories or files. The following steps outline a general approach:
- Install the Monitoring Tool: Use package managers such as npm for JavaScript tools or apt for Linux-based tools.
- Specify the Paths: Configure the paths that need to be monitored. This can often be done in a configuration file or via command-line arguments.
- Define Event Handlers: Set up event handlers to define what actions to take when a file change is detected. This could include recompiling code, running tests, or refreshing the application.
- Start the Monitoring Process: Launch the monitoring tool, ensuring it runs in the background to continuously watch for changes.
Example Configuration Using Chokidar
Here’s a sample configuration for using Chokidar in a Node.js project:
“`javascript
const chokidar = require(‘chokidar’);
// Initialize watcher
const watcher = chokidar.watch(‘src/**/*.js’, {
persistent: true
});
// Event listeners
watcher.on(‘add’, path => console.log(`File ${path} has been added`))
.on(‘change’, path => console.log(`File ${path} has been changed`))
.on(‘unlink’, path => console.log(`File ${path} has been removed`));
“`
In this example, Chokidar monitors all JavaScript files in the `src` directory. When a file is added, changed, or removed, it logs the event to the console.
Performance Considerations
When implementing file change monitoring, it’s crucial to consider the following performance aspects:
- Resource Utilization: Continuous monitoring can consume CPU and memory. Choose tools that are efficient and optimize for performance.
- Debouncing Events: Implement debouncing mechanisms to prevent multiple rapid events from triggering excessive actions. This is especially important in cases where multiple changes occur in quick succession.
- Scalability: Ensure that the monitoring solution can handle the scale of your project, especially as the number of files and directories increases.
Troubleshooting Common Issues
Developers may encounter several common issues when setting up file change monitoring:
Issue | Possible Causes | Solutions |
---|---|---|
Events not triggering | Incorrect path configuration | Verify and correct paths |
High resource consumption | Too many watched files | Limit the number of watched files |
Missing events | File system limitations | Switch to a more robust monitoring tool |
By addressing these common issues and optimizing configuration settings, developers can effectively utilize file change monitoring to enhance productivity in sandbox environments.
Expert Perspectives on Monitoring File Changes in Sandboxes
Dr. Emily Carter (Software Development Specialist, Tech Innovations Inc.). “Monitoring file changes in a sandbox environment is crucial for maintaining the integrity of development workflows. By implementing robust file change detection mechanisms, developers can ensure that unauthorized modifications are flagged immediately, thereby enhancing security and reducing the risk of errors.”
Mark Thompson (Cybersecurity Analyst, SecureTech Solutions). “In the realm of cybersecurity, watching for file changes in sandboxed environments is a fundamental practice. It allows organizations to detect potential breaches or malicious activities in real-time, which is essential for proactive threat management and incident response.”
Lisa Nguyen (DevOps Engineer, Agile Systems Group). “Effective file change monitoring in sandboxes not only streamlines the development process but also fosters collaboration among teams. By utilizing automated tools to track changes, teams can maintain a clear audit trail, facilitating easier debugging and version control.”
Frequently Asked Questions (FAQs)
What does it mean when a sandbox is watching for file changes?
A sandbox watching for file changes refers to a development environment that monitors specific files or directories for modifications. This allows developers to see real-time updates and test changes without needing to restart the application.
How can I enable file change watching in my sandbox environment?
To enable file change watching, you typically need to configure your development tools or frameworks to monitor specific directories. This can often be done through settings in your development environment or by using command-line options.
What are the benefits of using file change watching in a sandbox?
The benefits include increased productivity, as developers receive immediate feedback on changes, and a more efficient workflow, as it reduces the need for manual refreshes or restarts of the application.
Are there any performance drawbacks to file change watching?
Yes, file change watching can consume additional system resources, particularly if monitoring a large number of files or directories. This may lead to slower performance in some cases, especially on less powerful machines.
Can I customize the file change watching behavior in my sandbox?
Most development environments allow customization of file change watching behavior. You can specify which files to watch, set debounce times to limit the frequency of updates, and even exclude certain files or directories from monitoring.
What should I do if file change watching is not functioning correctly?
If file change watching is not functioning as expected, check the configuration settings, ensure that the necessary permissions are granted, and verify that the file system supports change notifications. Restarting the development server or the sandbox environment may also resolve the issue.
The concept of a “sandbox” in computing generally refers to a testing environment that isolates changes from the main system. This isolation is crucial when monitoring file changes, as it allows developers to experiment without risking the stability of the primary environment. When a sandbox is actively watching for file changes, it can trigger specific actions or alerts, enabling developers to understand the impact of their modifications in real-time. This functionality is particularly beneficial in development and testing phases, where rapid iterations and feedback loops are essential for productivity.
Furthermore, the ability to monitor file changes within a sandbox environment enhances security and stability. By isolating potentially harmful changes, developers can identify issues early in the development cycle, reducing the risk of introducing bugs or vulnerabilities into the main application. This proactive approach not only streamlines the development process but also fosters a culture of safe experimentation, where developers can innovate without fear of negative repercussions on the live system.
utilizing a sandbox that actively watches for file changes is a best practice in modern software development. It promotes a more efficient workflow, encourages safe experimentation, and ultimately leads to higher quality software products. Developers should leverage these environments to enhance their productivity and ensure the integrity of their applications during the development lifecycle.
Author Profile
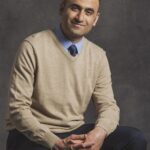
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?