How Can You Use sapply in R to Replace Else Statements?
In the world of R programming, efficiency and clarity are paramount, especially when dealing with data manipulation and analysis. One of the most powerful tools at your disposal is the `sapply` function, which simplifies the process of applying functions to elements of lists or vectors. However, a common challenge that many R users face is how to effectively replace elements or handle conditions within this framework. This article delves into the nuances of using `sapply` in R, particularly focusing on strategies for replacing values and exploring alternative approaches when `sapply` may not suffice.
Overview
The `sapply` function is a versatile option for iterating over data structures, allowing users to apply a function to each element and return a simplified output. While it is often praised for its ability to streamline code and enhance readability, there are situations where the need to replace certain values or handle conditional logic arises. Understanding how to implement these replacements effectively can significantly improve the robustness of your data processing tasks.
In cases where `sapply` falls short, R offers a variety of alternatives that can be employed to achieve similar results. Whether you’re looking for more complex conditional replacements or simply want to enhance the performance of your code, exploring these alternatives can open up new avenues for effective data manipulation
sapply Function in R
The `sapply` function in R is a powerful tool for applying a function to each element of a vector or list and simplifying the result. It is particularly useful when you want to perform operations on each element of a data structure without the need for explicit loops, enhancing code readability and efficiency.
The syntax for `sapply` is as follows:
“`R
sapply(X, FUN, …, simplify = TRUE, USE.NAMES = TRUE)
“`
Where:
- `X` is the input vector or list.
- `FUN` is the function to apply.
- `…` allows for additional arguments to be passed to `FUN`.
- `simplify` indicates whether to simplify the result into a vector or matrix.
- `USE.NAMES` determines if the output should be named.
Replacing Elements Using sapply
In situations where you want to replace elements based on a condition while using `sapply`, it can be done through the use of a custom function. Instead of a typical replacement function, you can create a function that incorporates a condition.
Here’s an example where we replace negative values in a numeric vector with zero:
“`R
vec <- c(-1, 2, -3, 4, -5)
result <- sapply(vec, function(x) ifelse(x < 0, 0, x))
print(result)
```
This will output:
```
[1] 0 2 0 4 0
```
The `ifelse` function serves as a vectorized conditional statement, making it efficient for element-wise operations.
Using else in sapply
While the `ifelse` function is efficient for straightforward conditions, you might also want to use a more complex logical flow that includes an `else` statement. In this case, you would typically define a custom function that contains both `if` and `else` clauses. Here’s an example:
“`R
vec <- c(-1, 2, -3, 4, -5)
replace_function <- function(x) {
if (x < 0) {
return(0)
} else {
return(x * 2)
}
}
result <- sapply(vec, replace_function)
print(result)
```
The output will be:
```
[1] 0 4 0 8 0
```
This allows for more complex logic to be applied to each element of the vector.
Table of Common Functions with sapply
The following table summarizes common functions that can be used with `sapply`:
Function | Description |
---|---|
mean() | Calculates the mean of each element. |
sum() | Returns the sum of each element. |
length() | Returns the length of each element. |
max() | Finds the maximum value in each element. |
min() | Finds the minimum value in each element. |
Incorporating `sapply` with custom functions enhances the ability to manipulate and transform data efficiently, catering to various analytical needs in R.
Understanding `sapply` in R
The `sapply` function in R is a powerful tool used for applying a function to each element of a list or vector and simplifying the output. It is particularly useful for dealing with complex datasets where vectorization is beneficial.
Syntax of `sapply`
The basic syntax of the `sapply` function is as follows:
“`r
sapply(X, FUN, …, simplify = TRUE, USE.NAMES = TRUE)
“`
- X: A vector, list, or an expression.
- FUN: The function to be applied.
- …: Additional arguments to `FUN`.
- simplify: Logical; if TRUE, attempts to simplify the result to a vector, matrix, or higher dimensional array.
- USE.NAMES: Logical; if TRUE, the result will be named if X is named.
Example Usage
“`r
numbers <- list(a = 1:5, b = 6:10)
result <- sapply(numbers, sum)
print(result)
```
The above example computes the sum of each vector in the list and returns a named vector.
Replacing `else` with `sapply`
When using `sapply`, you can often replace traditional `if…else` statements to streamline your code. The `sapply` function can handle conditional logic by incorporating custom functions that include conditions.
Implementing Conditional Logic
You can define a function that utilizes conditional statements and then apply it using `sapply`. Here’s an example:
“`r
values <- c(1, 2, 3, 4, 5)
conditional_function <- function(x) {
if (x %% 2 == 0) {
return("Even")
} else {
return("Odd")
}
}
result <- sapply(values, conditional_function)
print(result)
```
This will output a character vector indicating whether each number is "Even" or "Odd".
Alternative: Using `ifelse`
For simpler cases, where you have a binary condition, `ifelse` can be a more concise alternative:
```r
result <- ifelse(values %% 2 == 0, "Even", "Odd")
print(result)
```
Performance Considerations
- Efficiency: `sapply` can be slower than vectorized operations like `ifelse`, especially on larger datasets. Therefore, consider the size of your data when choosing between these functions.
- Readability: Using `sapply` with a defined function can enhance readability when applying complex logic, while `ifelse` provides a compact solution for straightforward conditions.
Comparison Table: `sapply` vs `ifelse`
Feature | `sapply` | `ifelse` |
---|---|---|
Function Type | General-purpose function | Vectorized conditional function |
Syntax Complexity | More complex, requires function | Simple, one-liner |
Performance | Slower for large datasets | Faster with vectorized operations |
Use Case | Complex logic with multiple cases | Simple binary conditions |
By strategically using `sapply` or `ifelse`, you can write more efficient and cleaner R code to handle conditional logic across your data structures.
Expert Insights on Using `sapply` in R for Conditional Replacement
Dr. Emily Chen (Data Scientist, StatTech Solutions). “When using `sapply` in R, it is essential to understand that while it simplifies the application of functions over lists or vectors, it may not always be the best choice for conditional replacements. In cases where complex conditions are involved, leveraging `ifelse` or the `dplyr` package can provide clearer and more efficient alternatives.”
Michael Thompson (Senior R Programmer, Analytics Pro). “In my experience, `sapply` can be quite powerful for vectorized operations, but it can lead to unexpected results when replacing values conditionally. I recommend using `lapply` in conjunction with `unlist` for more control, especially when dealing with nested lists or when the output structure is crucial.”
Sarah Patel (Statistical Consultant, DataWise Inc.). “While `sapply` is a convenient function for applying operations, it is important to consider readability and maintainability of your code. For conditional replacements, using `dplyr::mutate` with `case_when` can enhance clarity and performance, especially in larger datasets.”
Frequently Asked Questions (FAQs)
What is the purpose of the `sapply` function in R?
The `sapply` function in R is used to apply a function over a list or vector and simplify the result into a vector or matrix. It is particularly useful for performing operations on each element of a collection without the need for explicit loops.
How does `sapply` handle different data types?
`sapply` attempts to simplify the output based on the data types of the results. If all results are of the same type, it returns a vector; if they are of different types, it returns a list. This behavior allows for flexible data manipulation.
Can `sapply` be used to replace values in a vector?
Yes, `sapply` can be utilized to replace values in a vector by applying a function that defines the replacement logic. The function can check conditions and return the new value or the original value based on those conditions.
What is an alternative to `sapply` for more complex replacements?
For more complex replacements, the `dplyr` package offers functions like `mutate` and `if_else`, which provide clearer syntax and better handling of conditions, especially when working with data frames.
Is it possible to use `sapply` with a custom function for replacements?
Yes, you can define a custom function that specifies the replacement logic and pass it to `sapply`. This allows for tailored operations based on specific criteria for each element in the vector or list.
What should I consider when using `sapply` for large datasets?
When using `sapply` on large datasets, consider performance implications, as it may not be the most efficient option. In such cases, using vectorized operations or the `data.table` package can yield better performance and speed.
The `sapply` function in R is a powerful tool for applying a function to each element of a list or vector and simplifying the result into a vector or matrix. It is particularly useful for performing operations on data structures without the need for explicit loops, thus enhancing code efficiency and readability. The function automatically simplifies the output, which can be advantageous when dealing with large datasets or when the desired output is a uniform structure. However, there are scenarios where the default behavior of `sapply` may not be suitable, prompting users to explore alternative approaches.
When `sapply` does not meet specific needs, particularly in cases where more complex output structures are required, alternatives such as `lapply` or `vapply` can be employed. `lapply` returns a list regardless of the input type, which can be useful when the output varies in structure. On the other hand, `vapply` allows for more control by specifying the expected output type, thereby preventing unexpected results. Understanding the distinctions between these functions is crucial for effective data manipulation and analysis in R.
In summary, while `sapply` is a valuable function for simplifying operations on data structures in R, users should be aware of its limitations and consider other functions like
Author Profile
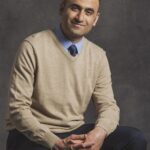
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?