How Can You Use sapply in R to Replace Else If Statements?
In the world of R programming, efficiency and clarity often go hand in hand. One of the most powerful tools at your disposal is the `sapply` function, which allows you to apply a function over a list or vector, simplifying your code and enhancing readability. However, when it comes to handling conditional logic, many programmers find themselves tangled in the complexities of traditional `if-else` statements. This is where the beauty of `sapply` truly shines, offering a streamlined approach that can replace cumbersome `else if` structures, making your code not only cleaner but also more intuitive.
Using `sapply`, you can seamlessly iterate over elements while applying conditions, transforming your data with elegance. This function allows you to avoid the pitfalls of nested `if-else` statements that can quickly become unwieldy, especially in larger datasets. By leveraging the power of `sapply`, you can create concise, functional code that is easier to maintain and understand. This article will explore how to effectively utilize `sapply` to simplify your conditional logic, providing you with practical examples and insights that will elevate your R programming skills.
As we delve deeper into the topic, we will uncover the nuances of using `sapply` in place of traditional `else if` statements. You’ll learn
sapply Function in R
The `sapply` function in R is a powerful tool that simplifies the process of applying a function to each element of a list or vector. It returns a simplified output, typically a vector or matrix, rather than a list, which is the output type of the `lapply` function. The primary advantage of using `sapply` is its ability to streamline code while maintaining clarity.
Usage of `sapply` can be particularly beneficial when working with data frames and lists, allowing users to perform operations on each column or element efficiently. The basic syntax is as follows:
“`r
sapply(X, FUN, …, simplify = TRUE, USE.NAMES = TRUE)
“`
Where:
- `X` is the input object (list or vector).
- `FUN` is the function to apply.
- `…` allows for additional arguments to be passed to `FUN`.
- `simplify` controls the type of output.
- `USE.NAMES` specifies whether to use names from `X`.
Replacing Else If Statements
In many programming scenarios, especially in data analysis, there may be a need to replace multiple `else if` statements with a more compact solution. R provides several functions, including `sapply`, that can effectively replace these constructs with a more streamlined approach.
For instance, when categorizing values based on conditions, `sapply` can be used to apply a custom function that encapsulates the logic typically contained within multiple `else if` statements. This reduces complexity and enhances readability. Here’s an example:
“`r
data <- c(1, 2, 3, 4, 5)
result <- sapply(data, function(x) {
if (x < 3) {
return("Low")
} else if (x <= 4) {
return("Medium")
} else {
return("High")
}
})
```
This code categorizes numbers into "Low", "Medium", and "High" based on their values.
Alternatively, using vectorized functions such as `ifelse` can be even more efficient:
```r
result <- ifelse(data < 3, "Low", ifelse(data <= 4, "Medium", "High"))
```
Both methods will yield the same output but using `ifelse` can lead to better performance with larger datasets.
Comparison Table
To further illustrate the differences between using `sapply` with `else if` versus using `ifelse`, refer to the following table:
Method | Readability | Performance | Output Type |
---|---|---|---|
sapply with else if | Moderate | Good | Vector |
ifelse | High | Better | Vector |
In summary, while `sapply` provides a useful framework for applying functions across elements in R, utilizing `ifelse` can often yield clearer and more efficient code in scenarios where multiple conditions are evaluated.
Understanding `sapply` in R
The `sapply` function in R is a part of the apply family of functions, which are used to apply a function over a list or vector. It simplifies the output to a vector or matrix when possible. This function is particularly useful when handling repetitive tasks across elements of a data structure.
Syntax of `sapply`
“`R
sapply(X, FUN, …, USE.NAMES = TRUE)
“`
- X: A list or vector to apply the function to.
- FUN: The function to apply.
- …: Additional arguments to pass to the function.
- USE.NAMES: Logical; should the result be named?
Example of `sapply`
“`R
numbers <- 1:5
squared <- sapply(numbers, function(x) x^2)
print(squared)
```
This code snippet squares each element in the vector `numbers`, resulting in a new vector.
Replacing `else if` Constructs
In R, the use of `else if` statements can sometimes lead to lengthy and complex code. When you need to apply conditional logic across elements of a vector or list, `sapply` can be used effectively to replace these constructs with a cleaner approach.
Using `sapply` for Conditional Logic
Instead of using multiple `if` statements, you can utilize `sapply` to evaluate conditions and return corresponding values. This is particularly useful when dealing with categorical data or transforming values based on certain criteria.
Example of Conditional Logic with `sapply`
“`R
grades <- c(85, 70, 90, 60, 75)
grade_category <- sapply(grades, function(x) {
if (x >= 85) {
return(“A”)
} else if (x >= 75) {
return(“B”)
} else if (x >= 65) {
return(“C”)
} else {
return(“D”)
}
})
print(grade_category)
“`
Simplifying with `sapply` and `ifelse`
For simpler conditions, the `ifelse` function can be a more concise alternative to `sapply` combined with `else if`. This function allows for vectorized operations and is efficient for binary conditions.
Example with `ifelse`
“`R
grades <- c(85, 70, 90, 60, 75)
grade_category <- ifelse(grades >= 85, “A”,
ifelse(grades >= 75, “B”,
ifelse(grades >= 65, “C”, “D”)))
print(grade_category)
“`
Comparing `sapply` and `ifelse`
Feature | `sapply` | `ifelse` |
---|---|---|
Structure | More flexible for complex logic | Best for binary conditions |
Readability | Can become verbose with multiple conditions | More concise for straightforward conditions |
Output Type | Can return different types | Returns a vector of the same type |
Performance | May be slower for large datasets | Generally faster for large vectors |
Using `sapply` enables a more functional programming style, while `ifelse` is great for quick conditional assignments. The choice between the two often depends on the complexity of the conditions being evaluated and personal coding preferences.
Expert Insights on Using `sapply` in R for Conditional Replacement
Dr. Emily Chen (Data Scientist, Analytics Innovations). “The `sapply` function in R is a powerful tool for applying a function over a list or vector. When it comes to replacing values conditionally, using `ifelse` within `sapply` can streamline your code, making it easier to read and maintain compared to a series of `else if` statements.”
Michael Thompson (R Programming Instructor, Data Science Academy). “Incorporating `sapply` with `ifelse` is often preferable for vectorized operations. It enhances performance and allows for concise code. While `else if` can be used, it tends to complicate the logic and can lead to slower execution times when dealing with large datasets.”
Sarah Patel (Quantitative Analyst, Financial Analytics Group). “When using `sapply`, replacing values conditionally should ideally leverage vectorized functions like `ifelse` for clarity and efficiency. This approach minimizes the need for nested `else if` statements, which can obfuscate the intent of the code and hinder debugging.”
Frequently Asked Questions (FAQs)
What is the purpose of the `sapply` function in R?
The `sapply` function in R is used to apply a function over a list or vector and simplify the result into a vector or matrix. It is particularly useful for performing operations on each element of a collection without the need for explicit loops.
Can I use `sapply` to replace the `else if` statements in R?
Yes, `sapply` can often be used to replace `else if` statements by applying a function that evaluates conditions for each element in a vector. This allows for more concise and vectorized code, improving readability and performance.
How do I implement conditional logic using `sapply`?
You can implement conditional logic within `sapply` by using a custom function that includes conditions (e.g., `ifelse` or `switch`) to evaluate each element. This enables you to return different values based on the conditions specified.
What are the advantages of using `sapply` over traditional loops?
The advantages of using `sapply` include increased code efficiency, reduced complexity, and enhanced readability. It allows for vectorized operations, which can lead to faster execution compared to traditional `for` loops.
Are there any limitations to using `sapply`?
Yes, `sapply` may not always return the expected output type, especially when the results vary in length or type. In such cases, `lapply` or `vapply` may be more appropriate as they provide more control over the output structure.
Can `sapply` handle multiple conditions like `else if`?
Yes, `sapply` can handle multiple conditions by using vectorized functions such as `ifelse`. By nesting multiple `ifelse` statements, you can effectively replicate the behavior of `else if` constructs within the `sapply` framework.
The use of the `sapply` function in R is a powerful tool for applying a function over a list or vector and simplifying the output. It is particularly useful when dealing with data frames or lists where you need to perform operations on each element. While `sapply` is efficient for many tasks, it may not always be the best choice when complex conditional logic is required, such as when using multiple `else if` statements. In such cases, alternative approaches like `lapply` or vectorized functions may provide clearer and more efficient solutions.
When replacing `else if` constructs, it is essential to consider the readability and maintainability of the code. Using vectorized operations or the `ifelse` function can streamline the process, making the code more concise and easier to understand. This is particularly important in collaborative environments where multiple individuals may work on the same codebase. Clear and efficient code enhances collaboration and reduces the likelihood of errors.
In summary, while `sapply` is a valuable function in R for applying operations across elements, it is crucial to evaluate the complexity of the logic being implemented. For scenarios involving multiple conditional checks, exploring alternatives such as `ifelse`, `lapply`, or even custom functions can lead
Author Profile
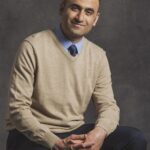
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?