How Can You Save API Data to Local Storage in Next.js?
In the fast-paced world of web development, the ability to efficiently manage and store data is crucial for creating seamless user experiences. As applications become increasingly dynamic, developers often seek ways to enhance performance and responsiveness. One powerful technique is leveraging local storage to save API data in a Next.js application. This approach not only optimizes data retrieval but also empowers users with offline capabilities, ensuring that their experience remains uninterrupted regardless of network conditions.
Next.js, a popular React framework, provides a robust foundation for building server-rendered applications. By integrating local storage into your Next.js project, you can significantly reduce the number of API calls, leading to faster load times and a more efficient application. This method allows you to cache data locally, making it readily accessible without the need for constant server communication. Additionally, local storage can enhance user experience by retaining important information even after a page refresh or when the user navigates away from the application.
In this article, we will explore the intricacies of saving API data to local storage in a Next.js environment. From understanding the fundamentals of local storage to implementing effective strategies for data management, we will equip you with the knowledge and tools necessary to elevate your web applications. Whether you’re a seasoned developer looking to refine your skills or a newcomer eager to learn, this
Understanding Local Storage in Next.js
Local storage is a web storage mechanism that allows developers to store key-value pairs in a user’s browser. It provides a simple way to persist data across sessions and is particularly useful for applications built with Next.js, where server-side rendering and client-side interactions often coexist.
Key features of local storage include:
- Synchronous API: Local storage operations are blocking, meaning they can delay UI updates if used improperly.
- Storage Limit: Typically, you can store about 5MB of data, depending on the browser.
- String Storage: Only strings can be stored, necessitating serialization of objects.
Setting Up Local Storage in a Next.js Component
To store API data in local storage within a Next.js component, you first need to fetch the data from the API. After fetching, you can save it to local storage. Here’s an example of how to implement this:
“`javascript
import { useEffect } from ‘react’;
const MyComponent = () => {
useEffect(() => {
const fetchData = async () => {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
localStorage.setItem(‘apiData’, JSON.stringify(data));
};
fetchData();
}, []);
return
;
};
export default MyComponent;
“`
In this example, the `useEffect` hook is utilized to fetch data when the component mounts, ensuring that the API call is made only once.
Retrieving Data from Local Storage
To retrieve data from local storage, you can use the `getItem` method. It’s essential to parse the data back into an object since local storage only stores strings. Below is an example of how to retrieve and display the stored data:
“`javascript
import { useEffect, useState } from ‘react’;
const DisplayData = () => {
const [data, setData] = useState(null);
useEffect(() => {
const storedData = localStorage.getItem(‘apiData’);
if (storedData) {
setData(JSON.parse(storedData));
}
}, []);
return (
{JSON.stringify(data, null, 2)}
: ‘No data available.’}
);
};
export default DisplayData;
“`
This component retrieves the stored data and checks if it exists before attempting to parse and display it.
Data Management Strategies
When working with local storage in Next.js, consider the following strategies to enhance data management:
- Data Expiry: Implement a mechanism to expire data based on specific criteria, such as time or changes in the API.
- Error Handling: Always include error handling for API calls and local storage access to maintain application stability.
- Data Synchronization: If your application can change data, ensure that updates are reflected in both local storage and the server.
Best Practices for Using Local Storage
To effectively utilize local storage in your Next.js applications, adhere to these best practices:
Best Practice | Description |
---|---|
Limit Storage Size | Only store essential data to avoid exceeding storage limits. |
Use JSON Serialization | Always serialize objects before storing and parse them upon retrieval. |
Fallback Mechanisms | Implement fallbacks for environments where local storage may be unavailable. |
Security Considerations | Avoid storing sensitive information in local storage, as it is accessible via JavaScript. |
By following these guidelines, you can effectively manage and utilize local storage in your Next.js applications, enhancing user experience and performance.
Storing API Data in Local Storage with Next.js
Utilizing local storage in Next.js allows developers to enhance user experience by saving API data for offline access or faster retrieval. Here’s how to effectively implement this functionality.
Integrating Local Storage
To save API data in local storage, follow these steps:
- **Fetch Data from API**: Use `useEffect` to make an API call when the component mounts.
- **Store Data in Local Storage**: Once data is fetched, utilize `localStorage.setItem()` to store the data.
- **Retrieve Data**: On subsequent renders, check if data is already in local storage before making a new API call.
Here’s a sample code implementation:
“`javascript
import { useEffect, useState } from ‘react’;
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const localData = localStorage.getItem(‘myData’);
if (localData) {
setData(JSON.parse(localData)); // Use data from local storage if available
} else {
const response = await fetch(‘https://api.example.com/data’);
const result = await response.json();
setData(result);
localStorage.setItem(‘myData’, JSON.stringify(result)); // Save data to local storage
}
};
fetchData();
}, []);
return (
{JSON.stringify(data, null, 2)}
) : (
Loading…
)}
);
};
export default MyComponent;
“`
Handling Local Storage Updates
When working with local storage, consider the following:
- Data Expiration: Implement a mechanism to update or remove stale data from local storage.
- Error Handling: Ensure to handle potential errors during API fetch or JSON parsing.
- Sensitive Data: Avoid storing sensitive information in local storage due to security concerns.
Example of Data Expiration Mechanism
Here’s how you can manage data expiration:
“`javascript
const fetchData = async () => {
const localData = localStorage.getItem(‘myData’);
const lastFetch = localStorage.getItem(‘lastFetch’);
const now = Date.now();
if (localData && lastFetch && now – lastFetch < 60000) { // 60 seconds setData(JSON.parse(localData)); } else { const response = await fetch('https://api.example.com/data'); const result = await response.json(); setData(result); localStorage.setItem('myData', JSON.stringify(result)); localStorage.setItem('lastFetch', now); } }; ```
Best Practices for Local Storage in Next.js
Consider implementing the following best practices:
- Data Size: Be mindful of the local storage limit (typically 5MB).
- JSON Stringification: Always stringify objects before storing and parse them upon retrieval.
- Reacting to Changes: Use state management to trigger updates in the UI when local storage changes.
Testing Local Storage Behavior
To ensure consistent behavior across different environments, you can use the following testing strategies:
Strategy | Description |
---|---|
Manual Testing | Check local storage via browser dev tools. |
Unit Tests | Use libraries like Jest to test local storage functions. |
Integration Tests | Test the full component behavior with mocked API responses. |
By following these guidelines, you can efficiently manage API data in local storage within your Next.js application, improving performance and user experience.
Expert Insights on Saving API Data in Local Storage with Next.js
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing local storage in Next.js can significantly enhance the user experience by allowing for faster access to previously fetched API data. It is essential, however, to implement proper data management strategies to avoid stale data issues.”
Michael Chen (Lead Frontend Developer, Web Solutions Group). “When saving API data to local storage in a Next.js application, developers should consider the size limitations and potential security implications. Encrypting sensitive data before storage is a best practice to safeguard user information.”
Sarah Thompson (Full Stack Developer, CodeCraft Labs). “Next.js provides a robust framework for handling API interactions. Leveraging local storage effectively allows for offline capabilities and improved performance, but it is crucial to implement synchronization mechanisms to keep the local data in line with server updates.”
Frequently Asked Questions (FAQs)
How can I save API data to local storage in a Next.js application?
To save API data to local storage in a Next.js application, you can use the `localStorage` API within a `useEffect` hook. Fetch the data from the API, then store it using `localStorage.setItem(‘key’, JSON.stringify(data))`.
Is it safe to store sensitive data in local storage?
Storing sensitive data in local storage is not recommended due to security vulnerabilities. Local storage is accessible via JavaScript, making it susceptible to XSS attacks. Use secure methods, such as server-side storage or encrypted cookies, for sensitive information.
How do I retrieve data from local storage in Next.js?
To retrieve data from local storage in Next.js, use the `localStorage.getItem(‘key’)` method. Ensure you access local storage within a client-side context, such as inside a `useEffect` hook, to avoid issues during server-side rendering.
Can I use local storage in server-side rendered pages in Next.js?
No, local storage is a client-side feature and cannot be accessed during server-side rendering in Next.js. You should handle local storage operations in lifecycle methods or hooks that run on the client side, such as `useEffect`.
What are the limitations of local storage in Next.js?
Local storage has a storage limit of approximately 5-10 MB per origin, depending on the browser. Additionally, it is synchronous and can block the main thread, leading to performance issues if not managed properly.
How can I clear local storage in a Next.js application?
To clear local storage in a Next.js application, use `localStorage.clear()` to remove all items or `localStorage.removeItem(‘key’)` to remove a specific item. This can be executed in response to user actions or during component unmounting.
In the context of Next.js, saving API data to local storage is a practical approach to enhance user experience by allowing offline access and reducing redundant API calls. This process typically involves fetching data from an API, processing it, and then utilizing the browser’s local storage capabilities to store the data for future use. By leveraging local storage, developers can ensure that users have quicker access to previously fetched data, which can significantly improve the performance of web applications.
Implementing local storage in a Next.js application requires a clear understanding of React hooks, particularly the useEffect and useState hooks. These hooks facilitate the fetching of data when the component mounts and manage the state of the application. Additionally, it is essential to handle the retrieval of stored data from local storage effectively, ensuring that the application can seamlessly transition between using fresh API data and cached local data based on availability and relevance.
Moreover, developers should consider the implications of local storage limitations, such as storage size constraints and the need for data synchronization. It is crucial to implement strategies for data expiration and updates to ensure that users are not presented with stale information. By doing so, applications can maintain a balance between performance and data accuracy, ultimately leading to a more robust and user-friendly experience.
Author Profile
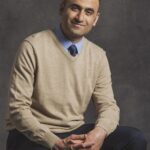
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?