How Can I Search for Text Within a Stored Procedure in SQL Server?
In the world of database management, SQL Server stands out as a powerful tool for developers and data analysts alike. Among its many features, stored procedures play a crucial role in encapsulating complex logic and streamlining database operations. However, as systems grow and evolve, the need to search for specific text within these stored procedures becomes increasingly important. Whether you’re troubleshooting an issue, optimizing performance, or simply trying to understand existing code, knowing how to effectively search for text in stored procedures can save you time and enhance your productivity.
Searching for text within stored procedures in SQL Server is not just about finding a needle in a haystack; it’s about navigating through layers of code to uncover insights that can lead to better database management practices. With the right techniques, you can quickly locate specific commands, parameters, or even comments that might hold the key to understanding complex logic. This capability is especially vital in environments where multiple developers contribute to the same codebase, making it essential to maintain clarity and coherence in your procedures.
In this article, we will explore various methods and tools available for searching text within stored procedures in SQL Server. From leveraging built-in system views to utilizing third-party solutions, we will guide you through the most effective strategies to streamline your search process. By the end, you will be equipped
Searching for Text in Stored Procedures
To search for specific text within stored procedures in SQL Server, you can use system views that provide metadata about the procedures. The primary view used for this purpose is `sys.sql_modules`, which contains the definition of all SQL Server objects, including stored procedures.
You can execute a query that filters stored procedures based on the text you are searching for. The following SQL query demonstrates how to find stored procedures containing a specific keyword:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%YourSearchText%’
AND
OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
In this query:
- `OBJECT_NAME(object_id)` retrieves the name of the stored procedure.
- `definition` contains the actual SQL code of the stored procedure.
- The `LIKE` operator is used to search for the specified text within the procedure’s definition.
- `OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1` ensures that only stored procedures are included in the results.
Example of Searching for Text
Suppose you want to find all stored procedures that contain the word “UPDATE”. You would modify the SQL query as follows:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%UPDATE%’
AND
OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
This will return a list of all stored procedures that contain the term “UPDATE” along with their definitions, making it easier for developers to locate specific logic or commands within their codebase.
Performance Considerations
Searching through the definitions of stored procedures can be resource-intensive, especially in databases with a large number of objects. To optimize performance, consider the following strategies:
- Limit Search Scope: If you know the schema or specific procedures to search within, add additional conditions to the `WHERE` clause.
- Use Full-Text Search: For larger databases, consider implementing Full-Text Search capabilities to enhance search performance and functionality.
Sample Result Table
When you execute the search query, the result might look like this:
ProcedureName | Definition |
---|---|
usp_UpdateCustomer | CREATE PROCEDURE usp_UpdateCustomer AS BEGIN UPDATE Customers SET … |
usp_GetCustomerDetails | CREATE PROCEDURE usp_GetCustomerDetails AS BEGIN SELECT * FROM Customers WHERE … |
This table format provides a clear view of each procedure’s name and its corresponding definition, facilitating easier navigation and understanding of the stored procedures in question.
Searching for Text in SQL Server Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize system views that store metadata about the procedures. The primary view for this purpose is `sys.sql_modules`, which contains definitions of SQL Server objects, including stored procedures.
Using SQL Queries for Text Search
You can execute a simple SQL query to find stored procedures containing a specified text string. Below is a sample query to achieve this:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_TYPE,
OBJECT_DEFINITION(OBJECT_ID(ROUTINE_NAME)) AS [Definition]
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
AND ROUTINE_TYPE = ‘PROCEDURE’;
“`
Key Components of the Query
- ROUTINE_NAME: The name of the stored procedure.
- ROUTINE_TYPE: Type of the routine, which should be ‘PROCEDURE’.
- OBJECT_DEFINITION: Retrieves the SQL definition of the procedure.
Modifying Search Criteria
You can adjust the `LIKE` clause to refine your search:
- Use `%` as a wildcard to match any sequence of characters.
- Use `_` as a wildcard for a single character.
Using the sys.sql_modules View
Alternatively, you can query the `sys.sql_modules` view directly:
“`sql
SELECT
OBJECT_NAME(object_id) AS [Procedure Name],
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%YourSearchText%’;
“`
Explanation of the Query
- OBJECT_NAME(object_id): Converts the object ID into the name of the procedure.
- definition: The actual SQL code of the stored procedure.
Performance Considerations
Searching through the definitions of stored procedures can be resource-intensive, especially in databases with numerous objects. To optimize your search:
- Limit searches to specific schemas if possible:
“`sql
AND SCHEMA_NAME(schema_id) = ‘YourSchemaName’
“`
- Execute the search during off-peak hours to reduce impact on database performance.
Identifying Related Objects
While searching for text in stored procedures, it may also be useful to identify any related functions, triggers, or views. You can extend your search to include these objects by modifying the queries as follows:
“`sql
SELECT
OBJECT_NAME(object_id) AS [Object Name],
type_desc
FROM
sys.objects
WHERE
OBJECT_DEFINITION(object_id) LIKE ‘%YourSearchText%’
AND type_desc IN (‘SQL_STORED_PROCEDURE’, ‘SQL_SCALAR_FUNCTION’, ‘SQL_TABLE_VALUED_FUNCTION’, ‘SQL_TRIGGER’);
“`
Object Types Included
- SQL_STORED_PROCEDURE: Standard stored procedures.
- SQL_SCALAR_FUNCTION: Functions returning a single value.
- SQL_TABLE_VALUED_FUNCTION: Functions returning a table.
- SQL_TRIGGER: Triggers associated with tables.
Using Third-Party Tools
For more complex searches or to enhance productivity, consider utilizing third-party SQL management tools, which often provide built-in functionality for searching through code. These tools can offer features such as:
- Syntax highlighting
- Code navigation
- Advanced filtering options
Utilizing these tools can significantly streamline the process of managing and searching through stored procedures and other database objects.
Expert Insights on Searching Text in SQL Server Stored Procedures
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “To effectively search for text within stored procedures in SQL Server, one can utilize the system views such as sys.sql_modules or sys.objects. These views allow you to query the definition of stored procedures and filter results based on specific text patterns using the LIKE operator.”
Mark Thompson (Senior SQL Server Consultant, Data Solutions Group). “Implementing a script that leverages the OBJECT_DEFINITION function can streamline the process of searching for specific text in stored procedures. This function retrieves the definition of the specified object, enabling developers to identify occurrences of particular keywords or phrases efficiently.”
Lisa Patel (Lead Database Administrator, Enterprise Data Management). “For comprehensive text searches within stored procedures, I recommend creating a custom stored procedure that iterates through all stored procedures in the database. This approach allows for more flexibility and can handle complex search criteria beyond simple text matching.”
Frequently Asked Questions (FAQs)
How can I search for a specific text in a stored procedure in SQL Server?
You can search for specific text in stored procedures by querying the `sys.sql_modules` system view or using the `OBJECT_DEFINITION` function. For example:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%YourSearchText%’;
“`
Is there a way to find all stored procedures containing a certain keyword?
Yes, you can use the same `sys.sql_modules` view to find all stored procedures that contain a specific keyword. Modify the query to include the keyword in the `LIKE` clause.
Can I use SQL Server Management Studio (SSMS) to search for text in stored procedures?
Yes, in SSMS, you can use the “Find” feature. Press `Ctrl + F`, select “Current Project” or “Entire Solution,” and enter the text you want to search for. This will return all occurrences of the text within stored procedures.
What permissions are required to search for text in stored procedures?
To search text in stored procedures, you need at least `VIEW DEFINITION` permission on the database. This allows you to access the definitions of the stored procedures.
Are there any third-party tools available for searching text in SQL Server stored procedures?
Yes, several third-party tools, such as Redgate SQL Search and ApexSQL Search, provide enhanced capabilities for searching text within stored procedures and other database objects.
Can I automate the process of searching for text in stored procedures?
Yes, you can automate the search process using SQL Server Agent jobs or scripts that run periodically. This can help in monitoring changes or finding specific patterns in stored procedure definitions.
Searching for text within stored procedures in SQL Server is an essential task for database administrators and developers. This process often involves querying the system catalog views, particularly `sys.sql_modules` and `sys.objects`, to locate specific keywords or phrases within the body of stored procedures. By executing a well-crafted SQL query, users can efficiently identify the stored procedures that contain the desired text, which is crucial for code maintenance, debugging, and understanding dependencies.
One effective method to perform this search is by using the `LIKE` operator in conjunction with the `OBJECT_DEFINITION` function. This approach allows for a flexible search, accommodating partial matches and case sensitivity as needed. Additionally, leveraging tools such as SQL Server Management Studio (SSMS) can enhance the user experience by providing graphical interfaces for searching through stored procedures, making the process more intuitive.
In summary, the ability to search for text within stored procedures is a vital skill for managing SQL Server databases. Understanding how to utilize system catalog views and SQL functions empowers users to maintain their codebase effectively. Moreover, integrating these techniques into regular database management practices can lead to improved performance and more streamlined development workflows.
Author Profile
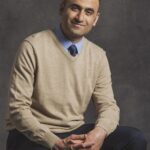
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?