How Can You Search Text Within a SQL Stored Procedure?
In the world of database management, SQL stored procedures stand as powerful tools that streamline complex operations and enhance performance. However, as systems grow and evolve, so does the need to maintain and optimize these procedures. One common challenge that developers and database administrators face is the ability to efficiently search for specific text within these stored procedures. Whether you’re troubleshooting an issue, refactoring code, or simply trying to understand the logic behind a procedure, knowing how to effectively search through the text can save you valuable time and effort.
Searching for text in SQL stored procedures is not just about finding keywords; it’s about navigating through layers of logic and functionality embedded within the code. With a variety of methods available—from using built-in SQL Server functions to employing third-party tools—understanding the best approaches can significantly enhance your workflow. The ability to pinpoint specific sections of code allows you to make informed decisions, ensuring that your database operates smoothly and efficiently.
As we delve deeper into this topic, we’ll explore the various techniques and tools available for searching text in SQL stored procedures. From leveraging SQL queries to utilizing integrated development environments (IDEs), you’ll gain insights into how to streamline your search process and improve your overall database management practices. Whether you’re a seasoned developer or new to SQL, mastering these search techniques will empower you to
Searching Text in SQL Stored Procedures
To search for specific text within SQL stored procedures, you can utilize system catalog views that contain metadata about procedures, such as `sys.sql_modules` and `sys.procedures`. These views allow you to query the definitions of stored procedures stored in the SQL Server database.
The basic approach to searching for text involves executing a SQL query that checks the `definition` column of the `sys.sql_modules` view for the desired string. Here’s an example query that searches for a specific text string within all stored procedures:
“`sql
SELECT
OBJECT_NAME(m.object_id) AS ProcedureName,
m.definition
FROM
sys.sql_modules AS m
JOIN
sys.procedures AS p ON m.object_id = p.object_id
WHERE
m.definition LIKE ‘%your_search_text%’
ORDER BY
ProcedureName;
“`
This query retrieves the names of stored procedures and their definitions containing `your_search_text`. It is crucial to replace `your_search_text` with the actual string you are looking for.
Understanding the Query Components
The components of the query can be broken down as follows:
- OBJECT_NAME(m.object_id): Returns the name of the stored procedure based on its object ID.
- m.definition: Contains the actual SQL code of the stored procedure.
- JOIN sys.procedures: Ensures that only procedures are included in the results.
- WHERE m.definition LIKE ‘%your_search_text%’: Filters the results to only those procedures that include the specified text.
Considerations When Searching
When performing text searches in stored procedures, consider the following:
- Case Sensitivity: The search may be case-sensitive depending on the collation settings of your SQL Server database.
- Performance: Searching large databases can be resource-intensive. It may be beneficial to limit searches to specific schemas or use additional filtering criteria.
- Output Management: The result set can be large; consider applying pagination or storing results in a temporary table for further analysis.
Example Output Table
The output from the query can be structured in a table format for better readability. Below is an example of what the output might look like:
Procedure Name | Definition |
---|---|
usp_GetCustomerData | CREATE PROCEDURE usp_GetCustomerData AS SELECT * FROM Customers WHERE Active = 1 |
usp_UpdateOrderStatus | CREATE PROCEDURE usp_UpdateOrderStatus AS UPDATE Orders SET Status = ‘Shipped’ WHERE OrderID = @OrderID |
Utilizing these techniques will enhance your ability to effectively search and manage SQL stored procedures, ensuring that you can maintain and optimize your database systems efficiently.
Searching for Text in SQL Stored Procedures
When dealing with SQL Server, it may become necessary to search for specific text within stored procedures. This is particularly useful for developers and database administrators who need to identify and modify code or understand dependencies.
Using SQL Server Management Studio (SSMS)
SQL Server Management Studio provides a user-friendly interface for searching through stored procedures.
– **Open Object Explorer**: Navigate to the database containing the stored procedures.
– **Use the ‘Search’ Feature**:
- Right-click on the database.
- Select “View” > “Object Explorer Details”.
- In the Object Explorer Details window, use the search box to filter stored procedures by name or text.
– **Script Out Procedures**:
- Right-click on the stored procedure.
- Choose “Script Stored Procedure as” > “CREATE To” > “New Query Editor Window”.
- Use `CTRL + F` to search for the desired text within the script.
Querying System Catalog Views
For more advanced searching, you can query system catalog views to find specific text within stored procedure definitions.
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%search_text%’
AND OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
This query retrieves the names and definitions of all stored procedures containing the specified `search_text`.
Using Information Schema
An alternative method involves using the `INFORMATION_SCHEMA.ROUTINES` view:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%search_text%’;
“`
This provides a list of stored procedures where the `ROUTINE_DEFINITION` contains the specified text.
Considerations for Searching
When performing text searches in stored procedures, keep the following in mind:
- Case Sensitivity: SQL Server’s default collation is case-insensitive. However, this can vary based on your database collation settings.
- Performance: Searching through large numbers of stored procedures can be resource-intensive. Limit your search to specific schemas if necessary.
- Stored Procedures with Encryption: Encrypted stored procedures will not return their definitions through these queries. You will need to decrypt them before searching.
Using Third-Party Tools
Several third-party tools can enhance your ability to search and manage SQL Server stored procedures. These tools often provide more advanced features, such as:
- Full-text search capabilities.
- Code analysis tools for identifying unused procedures.
- Dependency tracking to understand which procedures call others.
Tools such as Redgate SQL Search, ApexSQL Search, and dbForge Studio are popular among SQL Server professionals.
Expert Insights on Searching Text in SQL Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Searching for text within SQL stored procedures can be a challenging task due to the complexity of procedural code. Utilizing system catalog views such as `INFORMATION_SCHEMA.ROUTINES` or querying the `sys.sql_modules` table allows developers to extract the definition of stored procedures and apply pattern matching techniques to locate specific text.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “When attempting to search for text in SQL stored procedures, I recommend leveraging the SQL Server Management Studio’s built-in search functionality. This tool provides an efficient way to locate specific keywords or phrases across multiple stored procedures, enhancing productivity and reducing manual inspection time.”
Linda Chen (Data Analyst, Analytics & Insights Ltd.). “Incorporating dynamic SQL can be a powerful approach when searching for text within stored procedures. By dynamically constructing queries that filter the stored procedure definitions, analysts can effectively identify and analyze the usage of specific terms or patterns, which is crucial for maintaining code quality and optimizing performance.”
Frequently Asked Questions (FAQs)
How can I search for text within a SQL stored procedure?
You can search for text within a SQL stored procedure by querying the `sys.sql_modules` or `sys.procedures` system views. Use a `LIKE` clause to filter the `definition` column for the desired text.
What SQL command is used to find specific text in stored procedures?
The SQL command typically used is `SELECT`. For example, you can execute:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Can I search for text in multiple stored procedures at once?
Yes, you can search across multiple stored procedures by using the same `SELECT` statement with the `LIKE` clause. This will return all procedures containing the specified text.
Is it possible to search for text in stored procedures using SQL Server Management Studio (SSMS)?
Yes, you can use the “Find in Files” feature in SSMS. Access it via the Edit menu, then select “Find and Replace” and choose “Find in Files.” Input your search text and specify the scope to include stored procedures.
Are there any limitations when searching for text in stored procedures?
Yes, limitations may include not being able to search for text in encrypted stored procedures. Additionally, performance may be impacted if searching large databases without proper indexing.
What should I do if I cannot find the text I’m searching for in a stored procedure?
If you cannot find the text, ensure that you are searching with the correct syntax and that the text actually exists in the procedure. Additionally, check for variations in text or consider examining related procedures.
Searching for text within SQL stored procedures is a crucial task for database administrators and developers who need to analyze, maintain, or optimize their database code. SQL Server Management Studio (SSMS) provides built-in functionalities that allow users to search through stored procedures, functions, and other database objects. This capability is essential for identifying specific logic, debugging issues, or ensuring compliance with coding standards across the database environment.
One effective approach to search text in SQL stored procedures is to utilize the system views or information schema. For example, querying the `sys.sql_modules` or `INFORMATION_SCHEMA.ROUTINES` can reveal the definitions of stored procedures, allowing users to filter results based on specific keywords or phrases. Additionally, using text-based search functions, such as `LIKE`, can further refine the search process within the procedure definitions.
Moreover, leveraging third-party tools or dedicated SQL search utilities can enhance the efficiency of searching through extensive codebases. These tools often provide more advanced search capabilities, including regular expressions, which can significantly streamline the process of locating specific patterns or keywords in stored procedures. Overall, understanding how to effectively search within SQL stored procedures is vital for maintaining code quality and ensuring the smooth operation of database systems.
Author Profile
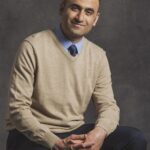
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?