How Can You Search for Text Within a Stored Procedure in SQL Server?
In the world of database management, stored procedures serve as powerful tools that encapsulate complex operations and streamline data manipulation. However, as databases grow in size and complexity, so does the challenge of maintaining and navigating through these stored procedures. One common task that developers and database administrators often face is the need to search for specific text or keywords within these procedures. Whether it’s for debugging, optimization, or simply understanding the flow of logic, knowing how to efficiently locate text within stored procedures can save valuable time and enhance productivity.
Searching for text in stored procedures in SQL Server is not just about finding a needle in a haystack; it’s about harnessing the right tools and techniques to make the process seamless. SQL Server offers various methods to query and analyze stored procedures, enabling users to pinpoint exact locations of interest. From using built-in system views to leveraging dynamic management views, understanding these approaches can significantly improve your workflow and ensure that you maintain a clear overview of your database’s functionality.
As we delve deeper into the methods and best practices for searching text in stored procedures, we’ll explore the intricacies of SQL Server’s querying capabilities. By the end of this article, you’ll be equipped with the knowledge to efficiently navigate your stored procedures, making your database management tasks not only easier but also more effective.
Methods to Search Text in Stored Procedures
Searching for specific text or keywords within stored procedures in SQL Server can be accomplished through various methods. Each method has its advantages depending on the context and requirements of your search.
Using System Views
SQL Server provides system views that can be queried to find the definitions of stored procedures. The `sys.sql_modules` view contains the definition of the objects, including stored procedures, while the `sys.objects` view provides information about the objects themselves.
To search for specific text within stored procedures, you can use the following SQL query:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules AS m
JOIN
sys.objects AS o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — P denotes stored procedures
AND m.definition LIKE ‘%search_text%’
“`
Replace `search_text` with the text you want to search for. This query will return the names and definitions of stored procedures that contain the specified text.
Using SQL Server Management Studio (SSMS)
SQL Server Management Studio (SSMS) provides a user-friendly interface for searching within stored procedures. You can leverage the “Find” feature to locate specific text. Here’s how:
- Open SSMS.
- Connect to your database instance.
- In the Object Explorer, right-click on the database.
- Select “Tasks” > “Generate Scripts”.
- Choose “Select specific database objects”.
- Check “Stored Procedures” and click “Next”.
- On the “Set Scripting Options” page, click “Advanced”.
- In the “General” section, find the “Script Data” option and set it to “”.
- Complete the wizard and open the generated script.
- Use `Ctrl + F` to search for your text within the script.
Using Third-Party Tools
Several third-party tools can facilitate text searches across stored procedures. These tools often provide advanced features, such as:
- Searching across multiple databases.
- Regular expression support.
- More user-friendly interfaces.
Popular tools include:
- Redgate SQL Search
- ApexSQL Search
Performance Considerations
When searching for text in stored procedures, consider the following performance aspects:
- Database Size: Larger databases may take longer to search.
- Number of Procedures: A high number of stored procedures can slow down the search.
- Execution Time: Queries against system views might impact performance if run on production servers.
Method | Pros | Cons |
---|---|---|
System Views | Direct access, no additional tools needed. | Requires SQL knowledge, may not be user-friendly. |
SSMS | Built-in feature, easy to use. | Limited to one database at a time. |
Third-Party Tools | Advanced features, supports multiple databases. | Cost associated, may require installation. |
By utilizing these methods, you can efficiently search for text within stored procedures, streamlining your development and debugging processes.
Methods to Search Text in Stored Procedures
To locate specific text within stored procedures in SQL Server, several methods can be employed. Below are the most common techniques:
Using SQL Server Management Studio (SSMS)
- **Object Explorer**:
- Open SQL Server Management Studio.
- Navigate to your database and expand the **Programmability** folder.
- Expand the **Stored Procedures** folder.
- Right-click on a specific stored procedure and select **Modify**.
- Use the **Find** feature (Ctrl + F) to search for the desired text within the procedure.
- **Filter Option**:
- In Object Explorer, right-click on the **Stored Procedures** folder.
- Choose **Filter** > Filter Settings.
- Set the filter criteria to narrow down the stored procedures based on names or other attributes.
Using T-SQL Queries
A more efficient approach for searching through multiple stored procedures is executing a T-SQL query. The following query can be utilized to find a specific text string:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%your_search_text%’
“`
- Replace `your_search_text` with the actual text you want to find.
- This query will return all stored procedures containing the specified text.
Using the sys.sql_modules System View
An alternative method is to query the `sys.sql_modules` view, which contains the definitions of SQL Server objects including stored procedures:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%your_search_text%’
“`
- This command provides a list of stored procedures with their definitions that match the search text.
Using SQL Server Data Tools (SSDT)
If you are using SSDT, you can perform a search across the entire project. The steps are as follows:
- Open your SSDT project.
- Press Ctrl + Shift + F to open the Find in Files dialog.
- Enter the text you want to search for and select the search scope.
- Review the results displayed in the output window.
Using Third-Party Tools
Several third-party tools can facilitate searching through stored procedures, providing advanced features:
- Redgate SQL Search: Integrates with SSMS, allowing for quick searches across database objects.
- ApexSQL Search: A free tool that provides comprehensive search capabilities for SQL Server databases.
Tool | Features |
---|---|
Redgate SQL Search | Quick search, integrates with SSMS, filters results |
ApexSQL Search | Free tool, advanced search options, results filtering |
Utilizing these methods will enable you to efficiently search for text within stored procedures in SQL Server, whether through SSMS, T-SQL queries, SSDT, or third-party tools. Each approach has its advantages depending on the specific needs and environment.
Expert Insights on Searching Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When searching for text within stored procedures in SQL Server, utilizing the `sys.sql_modules` system view is essential. This view contains the definitions of all SQL objects, allowing developers to filter and locate specific text patterns efficiently.”
Michael Thompson (Senior SQL Developer, Data Dynamics). “Employing the `LIKE` operator in conjunction with the `OBJECT_DEFINITION` function can be an effective strategy for searching text in stored procedures. This method provides flexibility in pattern matching, enabling developers to find exact or partial matches within the procedure definitions.”
Linda Zhang (Database Management Consultant, SQL Insights). “For comprehensive text searches, I recommend creating a script that iterates through the `sys.sql_modules` and `sys.objects` tables. This approach allows for a more granular search, accommodating various conditions and improving overall efficiency in locating specific text within stored procedures.”
Frequently Asked Questions (FAQs)
How can I search for specific text within a stored procedure in SQL Server?
You can search for specific text within a stored procedure by querying the `sys.sql_modules` system view. Use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to find all stored procedures that contain a certain keyword?
Yes, you can find all stored procedures containing a specific keyword by executing a query against the `sys.sql_modules` view, similar to the previous example, replacing `your_search_text` with your desired keyword.
Can I use SQL Server Management Studio (SSMS) to search for text in stored procedures?
Yes, in SSMS, you can use the “Find” feature. Press `Ctrl + F`, select “Current Project” or “Entire Solution,” and enter the text you want to search for. This will display all occurrences of the text in your stored procedures.
What should I do if I need to search for text in a large number of stored procedures?
For large numbers of stored procedures, use a script that queries `sys.sql_modules` or consider using third-party tools designed for database management that offer advanced search capabilities.
Are there any limitations to searching text in stored procedures using SQL queries?
Yes, limitations include the possibility of missing text in comments or dynamic SQL, as these may not be fully captured in the `definition` column. Additionally, performance may be affected when searching through a large number of objects.
Can I automate the search for text in stored procedures?
Yes, you can automate the search by creating a SQL Server job that runs a script at scheduled intervals, or by using PowerShell scripts to query the database and log results based on your search criteria.
Searching for text within stored procedures in SQL Server is a common requirement for database administrators and developers. This process typically involves querying the system catalog views, such as `sys.sql_modules` and `sys.objects`, to locate the definition of stored procedures that contain specific keywords or phrases. By utilizing the `LIKE` operator in conjunction with these catalog views, users can effectively filter and retrieve the stored procedures that match their search criteria.
One effective method for performing this search is to execute a SQL query that targets the `definition` column of the `sys.sql_modules` view. This approach allows for a direct examination of the text within stored procedures. Additionally, incorporating the `sys.objects` view can help to further refine the search by filtering results based on the type of object, ensuring that only stored procedures are considered in the results.
It is also important to note that while searching for text in stored procedures can be straightforward, it may present challenges if the stored procedures are extensive or poorly documented. Regular maintenance of stored procedures, including clear naming conventions and comments, can significantly enhance the ease of searching and understanding the code. Overall, leveraging SQL Server’s system catalog views provides a powerful means to efficiently locate and manage stored procedures based on their content.
Author Profile
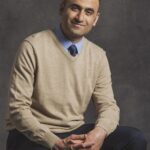
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?