How Can I Use sed to Replace Backslashes with Forward Slashes?
When it comes to text processing and manipulation in Unix-like systems, the `sed` command stands out as a powerful tool for streamlining workflows and automating repetitive tasks. Among its many functionalities, one common requirement is the need to replace characters within strings. A particularly interesting scenario arises when you want to replace backslashes with forward slashes—a task that may seem simple at first glance but can present challenges due to the special nature of these characters in regular expressions. This article delves into the intricacies of using `sed` for this specific replacement, providing you with the knowledge to tackle similar text manipulation tasks with confidence.
Understanding how to effectively use `sed` for replacing backslashes with forward slashes involves grasping both the syntax of the command and the peculiarities of escape characters. Backslashes are often used as escape characters in programming and scripting, which can lead to confusion when trying to manipulate them in text. This article will guide you through the necessary steps to perform this replacement, highlighting the importance of proper escaping and syntax to ensure your commands function as intended.
As we explore the nuances of using `sed` for this task, you’ll discover practical examples and tips that can enhance your text processing skills. Whether you’re a seasoned developer or a newcomer looking to streamline your scripts, mastering
Using `sed` for Replacing Backslashes
The `sed` command in Unix-like operating systems is a powerful stream editor used for parsing and transforming text. When it comes to replacing characters, such as transforming backslashes (`\`) into forward slashes (`/`), it is essential to handle the escaping properly due to the special meaning of backslashes in both the shell and `sed` syntax.
To replace backslashes with forward slashes in a text file, you can use the following `sed` command:
“`bash
sed ‘s/\\/\\//g’ filename
“`
In this command:
- `s` indicates the substitution operation.
- The first `\\` is used to represent a single backslash. In `sed`, the backslash is an escape character, so it must be escaped with another backslash.
- The second `//` indicates that the backslash should be replaced with a forward slash.
- The `g` at the end signifies a global replacement, meaning all occurrences in the line will be replaced.
Example of `sed` Command Usage
Consider a text file named `example.txt` that contains the following lines:
“`
C:\Users\Name\Documents
D:\Projects\Code
“`
To replace the backslashes with forward slashes, execute:
“`bash
sed ‘s/\\/\\//g’ example.txt
“`
The output will be:
“`
C:/Users/Name/Documents
D:/Projects/Code
“`
Alternative Method Using `-i` Flag
If you want to edit the file in place and save the changes directly to `example.txt`, you can use the `-i` option:
“`bash
sed -i ‘s/\\/\\//g’ example.txt
“`
This command modifies the original file without producing a separate output.
Handling Complex Strings
When dealing with complex strings that may contain multiple backslashes or other special characters, it is prudent to use a delimiter other than `/` to avoid confusion. For instance, you can use `|` as a delimiter:
“`bash
sed ‘s|\\|/|g’ filename
“`
This approach simplifies readability, especially in paths that contain multiple slashes.
Common Use Cases for `sed` Replacement
- File Path Normalization: Converting Windows paths to Unix-like paths for compatibility.
- Data Migration: Updating configuration files or scripts that have hard-coded paths.
- Text Processing: Preparing data for processing in tools that require specific path formats.
Original String | Modified String |
---|---|
C:\Program Files\App | C:/Program Files/App |
D:\Data\Backup | D:/Data/Backup |
By utilizing `sed` effectively, you can streamline many text processing tasks involving path transformations and ensure compatibility across different operating systems.
Using sed to Replace Backslashes with Forward Slashes
The `sed` command is a powerful stream editor for filtering and transforming text. When it comes to replacing characters, particularly backslashes (`\`) with forward slashes (`/`), the syntax requires careful handling due to the special meaning of backslashes in regular expressions.
Basic Syntax for Replacement
The basic syntax for using `sed` to replace backslashes with forward slashes is as follows:
“`bash
sed ‘s/\\/\//g’ filename
“`
In this command:
- `s` indicates the substitution operation.
- `\\` is used to represent a single backslash. The first backslash escapes the second one.
- `\/` represents the forward slash, which also needs to be escaped.
- `g` at the end signifies that the substitution should be global, replacing all occurrences in each line.
Examples
Here are a few practical examples illustrating the usage of `sed` for this task.
Example 1: Simple Replacement in a File
To replace backslashes with forward slashes in a file named `example.txt`, run:
“`bash
sed ‘s/\\/\//g’ example.txt
“`
This command reads the content of `example.txt`, applies the substitution, and outputs the result to the terminal.
Example 2: In-Place Editing of a File
If you want to modify the file directly without creating a new output, use the `-i` option:
“`bash
sed -i ‘s/\\/\//g’ example.txt
“`
This command overwrites `example.txt` with the changes.
Example 3: Processing Command Output
You can also pipe the output of another command through `sed`. For instance, if you have a command that generates paths with backslashes:
“`bash
echo “C:\Program Files\MyApp” | sed ‘s/\\/\//g’
“`
This will display:
“`
C:/Program Files/MyApp
“`
Handling Special Cases
When dealing with more complex strings or files that might contain multiple special characters, additional care may be required.
- Multiple Backslashes: If the text contains sequences of backslashes, adjust the pattern accordingly. For example, to replace two backslashes with a single forward slash:
“`bash
sed ‘s/\\\\/\//g’ filename
“`
- Escaping Other Characters: If your text includes other characters that need escaping, ensure to manage those as well. For instance, if replacing backslashes in URLs or paths, consider the implications of other special characters.
Using `sed` for replacing backslashes with forward slashes is efficient and straightforward. With the right syntax and understanding of escaping characters, you can manipulate text effectively for various applications, including configuration files, scripts, and data processing tasks.
Expert Insights on Using sed to Replace Backslashes with Forward Slashes
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When utilizing sed for text manipulation, it is crucial to escape the backslash properly. The command ‘sed ‘s/\\\\/\\//g’ effectively replaces all backslashes with forward slashes, ensuring that your file paths are compatible with Unix-based systems.”
Michael Chen (DevOps Specialist, CloudTech Innovations). “In scripting environments, replacing backslashes with forward slashes can prevent issues with path interpretation. Using sed in this manner not only simplifies path management but also enhances script portability across different operating systems.”
Laura Simmons (Linux System Administrator, OpenSource Experts). “The sed command is a powerful tool for text processing. When replacing backslashes, remember that each backslash must be escaped, leading to the syntax ‘s/\\\\/\\//g’. This is essential for maintaining the integrity of your scripts and avoiding unexpected errors.”
Frequently Asked Questions (FAQs)
How can I use sed to replace backslashes with forward slashes?
You can use the following command: `sed ‘s/\\/\\//g’ filename`. This command replaces all instances of backslashes (`\`) with forward slashes (`/`) in the specified file.
Why do I need to escape backslashes in sed?
Backslashes are special characters in sed, used for escaping other characters. To match a literal backslash, you must escape it with another backslash, resulting in `\\`.
Can I use sed to replace backslashes in a variable instead of a file?
Yes, you can use echo to pass a variable to sed. For example: `echo “$variable” | sed ‘s/\\/\\//g’` will replace backslashes in the content of the variable.
What if I want to replace backslashes only at the beginning of a line?
You can modify the sed command to target only the beginning of a line: `sed ‘s/^\\//g’ filename`. This will replace a backslash at the start of each line with a forward slash.
Is it possible to replace backslashes with forward slashes in a specific context using sed?
Yes, you can use regular expressions to specify the context. For example, `sed ‘s/context\\context/forward_slash/g’ filename` allows you to replace backslashes only within a specific context.
What are some common errors when using sed for this replacement?
Common errors include forgetting to escape the backslash, using incorrect syntax, or not specifying the global flag (`g`) if multiple replacements are needed within a line.
In summary, the process of replacing backslashes with forward slashes using the `sed` command in Unix-based systems is a straightforward yet powerful technique. The `sed` command, which stands for stream editor, allows users to perform basic text transformations on an input stream (a file or input from a pipeline). By utilizing specific syntax, users can effectively substitute characters in text files or command outputs, enhancing the flexibility of text manipulation tasks.
When executing this replacement, it is essential to properly escape the backslash character due to its special meaning in regular expressions. The correct syntax for this operation typically involves using double backslashes to represent a single backslash in the `sed` command. For instance, the command `sed ‘s/\\/\\//g’` effectively replaces all occurrences of backslashes with forward slashes across the specified input.
Key takeaways from this discussion include the importance of understanding character escaping in regular expressions, as well as the utility of `sed` for batch processing text files. Mastering these skills can significantly streamline workflows, particularly in programming and data processing tasks where path formats may need to be standardized. Overall, the ability to manipulate text with precision is a valuable asset in many technical fields.
Author Profile
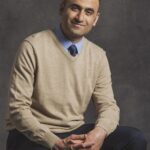
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?