How Can I See the Creation Time of a Record in MySQL?
In the world of database management, understanding the lifecycle of data is crucial for maintaining integrity and tracking changes over time. One of the most vital aspects of this lifecycle is the creation time of a record. Whether you’re a seasoned developer or a curious beginner, knowing how to access and utilize this information in MySQL can significantly enhance your ability to manage and analyze your data effectively. In this article, we will explore the methods and best practices for retrieving the creation time of records in MySQL, empowering you to leverage this information for better decision-making and data governance.
MySQL, as one of the most popular relational database management systems, offers various ways to track when a record was created. By default, MySQL does not automatically store the creation timestamp of a record unless explicitly defined. However, with the right strategies and data types, you can easily implement a system that captures this critical information. Understanding how to set up your tables to include a creation timestamp can not only help you maintain a clear history of your data but also facilitate auditing and troubleshooting processes.
In addition to setting up your schema correctly, MySQL provides several functions and queries that can help you retrieve and manipulate this timestamp data. From using the `NOW()` function to automatically populate creation time fields to querying existing records for their creation
Understanding Record Creation Time in MySQL
In MySQL, the creation time of a record can be crucial for tracking changes, auditing, and maintaining the integrity of your data. To effectively capture and view the creation time of a record, you typically use a `DATETIME` or `TIMESTAMP` data type in your table schema. These data types allow you to store date and time information, which can then be automatically populated when a new record is inserted.
Using the TIMESTAMP Data Type
The `TIMESTAMP` data type in MySQL has the ability to automatically record the time of a record’s creation or last update, making it a popular choice for tracking creation times. When defining a table, you can set a column to automatically fill with the current timestamp upon row creation.
Example table definition:
“`sql
CREATE TABLE example_table (
id INT AUTO_INCREMENT PRIMARY KEY,
data VARCHAR(100),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
“`
In this example, the `created_at` column will automatically be populated with the current timestamp when a new record is inserted.
Inserting Records and Viewing Creation Times
When you insert a record into a table with a `TIMESTAMP` column set to `CURRENT_TIMESTAMP`, you don’t need to specify a value for the `created_at` column; it will be automatically filled.
“`sql
INSERT INTO example_table (data) VALUES (‘Sample Data’);
“`
To view the creation time of records, you can execute a simple `SELECT` query:
“`sql
SELECT id, data, created_at FROM example_table;
“`
This query will return a result set that includes the `id`, `data`, and the corresponding `created_at` timestamps.
Using the DATETIME Data Type
Alternatively, you can use the `DATETIME` data type, which stores date and time information but does not automatically update on record modification. You must explicitly set the value when inserting a record.
Example table definition:
“`sql
CREATE TABLE example_table (
id INT AUTO_INCREMENT PRIMARY KEY,
data VARCHAR(100),
created_at DATETIME
);
“`
You would need to insert the current timestamp manually:
“`sql
INSERT INTO example_table (data, created_at) VALUES (‘Sample Data’, NOW());
“`
Comparison of TIMESTAMP and DATETIME
Feature | TIMESTAMP | DATETIME |
---|---|---|
Automatic Update | Yes (on update) | No |
Range | ‘1970-01-01 00:00:01’ to ‘2038-01-19 03:14:07’ | ‘1000-01-01’ to ‘9999-12-31’ |
Time Zone Awareness | Yes (converts to UTC) | No (stores as is) |
Default Value | CURRENT_TIMESTAMP | None (must be set explicitly) |
Retrieving and Formatting Creation Times
You may want to format the creation time for better readability. MySQL provides functions like `DATE_FORMAT()` for this purpose.
“`sql
SELECT id, data, DATE_FORMAT(created_at, ‘%Y-%m-%d %H:%i:%s’) AS formatted_creation_time
FROM example_table;
“`
This will output the creation time in a more human-readable format. By understanding these concepts and utilizing the appropriate data types and functions, you can effectively manage and view the creation times of records in MySQL.
Understanding Creation Timestamps in MySQL
In MySQL, to track the creation time of records, a common approach is to utilize the `TIMESTAMP` or `DATETIME` data types. These types can store date and time information, allowing you to record when a row is inserted into a table.
Creating a Table with a Creation Timestamp
To implement this, you can define a table with a specific column designated to capture the creation time. Here’s an example SQL statement:
“`sql
CREATE TABLE your_table (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
“`
In this example:
- `created_at` is a column that automatically captures the current timestamp when a new record is inserted.
Retrieving Creation Timestamps
To view the creation time of records, you can execute a `SELECT` query. For instance:
“`sql
SELECT id, name, created_at FROM your_table;
“`
This query will return all records along with their creation timestamps.
Updating Records Without Altering Creation Time
If you wish to update a record without changing the `created_at` timestamp, ensure that this column is not included in the `UPDATE` statement. For example:
“`sql
UPDATE your_table SET name = ‘New Name’ WHERE id = 1;
“`
This command updates the `name` field while preserving the `created_at` timestamp.
Using Triggers for Advanced Timestamp Management
In scenarios where you need more control over timestamps, consider using triggers. A trigger can automatically set timestamps for both creation and updates. Here’s how to create a trigger for updating a `modified_at` field:
“`sql
CREATE TABLE your_table (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
modified_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
CREATE TRIGGER before_update
BEFORE UPDATE ON your_table
FOR EACH ROW
SET NEW.modified_at = CURRENT_TIMESTAMP;
“`
In this setup:
- `modified_at` records the last modification time.
- The `before_update` trigger updates `modified_at` each time a record is changed.
Querying Records Based on Creation Time
To filter records based on their creation time, you can use a `WHERE` clause. For example:
“`sql
SELECT * FROM your_table WHERE created_at >= ‘2023-01-01 00:00:00’;
“`
This query retrieves all records created on or after January 1, 2023.
Considerations for Time Zones
When dealing with timestamps, it is crucial to consider time zone settings. MySQL timestamps are typically stored in UTC. To ensure accurate time representation:
- Always store timestamps in UTC.
- Use the `CONVERT_TZ()` function when retrieving data to convert it to the desired time zone.
Example:
“`sql
SELECT id, name, CONVERT_TZ(created_at, ‘+00:00’, ‘+02:00’) AS created_at_local FROM your_table;
“`
This converts the UTC timestamp to a local time zone (e.g., UTC+2).
By utilizing these techniques, you can effectively track and manage record creation times in MySQL, ensuring data integrity and facilitating time-based queries.
Understanding Record Creation Time in MySQL
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “To see the creation time of a record in MySQL, you should utilize the `TIMESTAMP` or `DATETIME` data types. By including a column with the `DEFAULT CURRENT_TIMESTAMP` attribute, you can automatically capture the creation time of each record upon insertion.”
Mark Thompson (Senior MySQL Consultant, Data Solutions Group). “It’s crucial to ensure that your table schema includes a timestamp column specifically for tracking creation time. Additionally, using the `ON UPDATE CURRENT_TIMESTAMP` option can help maintain accurate records of when entries are modified.”
Lisa Nguyen (Lead Database Administrator, CloudTech Services). “For existing records, if you haven’t set up a creation timestamp, you can add a new column and populate it with the current timestamp for all existing entries. However, going forward, it’s best practice to always include a creation timestamp in your database design.”
Frequently Asked Questions (FAQs)
How can I see the creation time of a record in MySQL?
To see the creation time of a record in MySQL, you need to include a `TIMESTAMP` or `DATETIME` column in your table that automatically records the creation time. This can be done using the `DEFAULT CURRENT_TIMESTAMP` attribute when creating or altering the table.
Is there a way to retrieve the creation time of existing records?
If existing records do not have a creation timestamp column, you cannot retrieve their creation times. You would need to alter the table to add a timestamp column and then update it for existing records.
Can I use triggers to capture creation timestamps in MySQL?
Yes, you can use triggers in MySQL to automatically set a creation timestamp. Create an `AFTER INSERT` trigger that sets the timestamp column to the current time whenever a new record is inserted.
What data type should I use for storing creation time in MySQL?
You should use either `DATETIME` or `TIMESTAMP` data types for storing creation times. `TIMESTAMP` is often preferred for its ability to automatically adjust for time zones.
How do I modify an existing table to include a creation timestamp?
You can modify an existing table by using the `ALTER TABLE` statement. For example:
“`sql
ALTER TABLE your_table_name ADD COLUMN created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP;
“`
Can I query records based on their creation time?
Yes, you can query records based on their creation time by using a `WHERE` clause in your SQL query. For example:
“`sql
SELECT * FROM your_table_name WHERE created_at > ‘2023-01-01’;
“`
In MySQL, tracking the creation time of a record is essential for various applications, particularly in auditing and data management. To achieve this, developers commonly utilize timestamp or datetime data types in their table schemas. By defining a column that captures the creation time, usually set to the current timestamp upon record insertion, users can easily retrieve this information when querying the database.
MySQL provides the ability to automatically populate a column with the current timestamp using the DEFAULT CURRENT_TIMESTAMP attribute. This feature simplifies the process of recording the creation time, ensuring accuracy and consistency across records. Additionally, it is possible to modify existing tables to include this functionality, allowing for the retroactive tracking of creation times for historical data.
In summary, effectively managing the creation time of records in MySQL involves leveraging the appropriate data types and attributes during table design. This practice not only enhances data integrity but also supports better data analysis and reporting. By implementing these strategies, database administrators and developers can ensure that they have a reliable method for tracking when records were created, which is invaluable for many operational and analytical purposes.
Author Profile
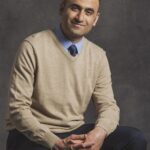
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?