Why Did My Select Method of Range Class Fail?
In the realm of programming and data manipulation, encountering errors can be a frustrating yet enlightening experience. One such error that developers may come across is the “select method of range class failed.” This cryptic message can halt productivity and leave even seasoned programmers scratching their heads. Understanding the nuances of this error is crucial for anyone working with spreadsheets, particularly in environments like Microsoft Excel or its automation through programming languages such as VBA.
This article delves into the intricacies of the “select method of range class failed” error, exploring its common causes and the contexts in which it arises. Whether you’re automating tasks in Excel or manipulating data through scripts, this error can disrupt your workflow, but it also presents an opportunity to enhance your coding skills and deepen your understanding of object-oriented programming principles.
As we navigate through the potential pitfalls and solutions, you’ll gain insights into best practices for working with ranges in your code, ensuring that you can avoid this error in the future. By the end of this exploration, you’ll be equipped with the knowledge to troubleshoot effectively and enhance your programming prowess, turning a moment of frustration into a stepping stone for growth.
Understanding the Error
The error message “select method of range class failed” typically occurs in Microsoft Excel or other applications that utilize VBA (Visual Basic for Applications). It signifies that a specified range could not be selected or manipulated, often due to various underlying issues. Understanding the reasons for this error is crucial for effective troubleshooting.
Common causes include:
- Invalid Range Reference: The specified range may not exist or is incorrectly defined.
- Protected Worksheet: The worksheet may be protected, preventing selection.
- Outdated Excel Version: Using an outdated or unsupported version of Excel can lead to compatibility issues.
- Active Sheet Context: The code may be running on a different sheet than intended, leading to range selection failures.
Troubleshooting Steps
To resolve the “select method of range class failed” error, the following steps can be taken:
- Verify Range Reference: Ensure that the range you are trying to select is valid and exists in the active workbook.
- Check Worksheet Protection: Unprotect the worksheet if it is protected, or modify the code to bypass this limitation.
- Update Excel: Check for updates to your Excel application and install the latest version to avoid compatibility issues.
- Activate the Correct Sheet: Before selecting the range, ensure that the correct worksheet is activated using `Worksheets(“SheetName”).Activate`.
Sample Code for Reference
The following VBA code demonstrates how to handle range selection and avoid the “select method of range class failed” error:
“`vba
Sub SelectRange()
On Error Resume Next ‘ Prevents runtime errors from stopping code execution
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(“Sheet1”) ‘ Specify your sheet name here
ws.Activate
ws.Range(“A1:B2”).Select ‘ Adjust the range as needed
If Err.Number <> 0 Then
MsgBox “Error selecting range: ” & Err.Description
End If
On Error GoTo 0 ‘ Reset error handling
End Sub
“`
Best Practices
To prevent encountering this error in the future, consider implementing the following best practices:
- Avoid Selecting Ranges: Instead of selecting ranges, directly manipulate them. For example, instead of `Range(“A1”).Select`, use `Range(“A1”).Value = “New Value”`.
- Use Fully Qualified References: Always use fully qualified references to avoid ambiguity in your code.
- Regularly Update Software: Keep your software up to date to reduce compatibility issues.
Summary of Common Causes
Cause | Description |
---|---|
Invalid Range Reference | The specified range does not exist or is incorrectly defined. |
Protected Worksheet | The worksheet is protected, preventing range selection. |
Outdated Excel Version | An outdated version can lead to compatibility issues. |
Active Sheet Context | The code may be executing in a different worksheet context. |
Implementing these strategies can significantly reduce the likelihood of encountering the “select method of range class failed” error while enhancing the robustness of your VBA projects.
Understanding the Range Class in Python
The `range` class in Python is a built-in sequence type that generates a sequence of numbers. It is commonly used in loops for iteration, especially in `for` loops. The `range` function can take one to three parameters: `start`, `stop`, and `step`.
- start: The starting point of the sequence (inclusive). Defaults to 0.
- stop: The endpoint of the sequence (exclusive). Required.
- step: The increment between each number in the sequence. Defaults to 1.
Here’s a simple example of how to create a range object:
“`python
my_range = range(1, 10, 2) Generates numbers 1, 3, 5, 7, 9
“`
Common Causes of the “Select Method of Range Class Failed” Error
The error message “select method of range class failed” typically indicates an issue with how the `range` class is being utilized or invoked in your code. Below are common causes for this error:
- Incorrect Usage of Select Method: The `select` method is not a standard method for the `range` class. Ensure that you are not mistakenly trying to call it on a range object.
- Data Type Mismatch: If the `select` method is being used in conjunction with another data type (for instance, a list or a string) rather than a range object, it may lead to this error.
- Exceeding Memory Limits: When working with very large ranges, the system may run out of memory, causing the select method to fail.
- Environment Issues: Certain environments or IDEs may not fully support the operations being performed, leading to unexpected errors.
Debugging Steps
To resolve the “select method of range class failed” error, consider the following debugging steps:
- Check Method Calls: Verify that you are not invoking a non-existent method on a range object.
- Examine Data Types: Ensure you are operating on the correct data types. For example:
- If using a list or another iterable, ensure the correct methods are being called.
- Test in Isolation: Run the code snippet that generates the error independently to see if it persists. This may help isolate the issue.
- Memory Usage: If working with large ranges, try reducing the size or use generators that yield values on demand.
- Consult Documentation: Ensure that the methods and classes being used are supported in your version of Python.
Example Code for Correct Usage
Here’s an example demonstrating the correct usage of the `range` class in a loop without invoking any unsupported methods:
“`python
for i in range(1, 10):
print(i) Correctly iterates through numbers 1 to 9
“`
If you need to select items from a range or perform operations, consider converting the range to a list:
“`python
my_list = list(range(1, 10)) Converts range to a list
selected_items = [x for x in my_list if x % 2 == 0] Selects even numbers
print(selected_items) Output: [2, 4, 6, 8]
“`
By adhering to these guidelines and understanding the limitations and proper usage of the `range` class, you can avoid common pitfalls and ensure smooth execution of your Python code.
Understanding the “Select Method of Range Class Failed” Error
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “The ‘Select method of Range class failed’ error typically arises in Excel VBA when attempting to select a range that is not currently active or visible. This often occurs when the code attempts to select a range on a worksheet that is not currently displayed to the user. Ensuring that the correct worksheet is activated before making selections can help mitigate this issue.”
Michael Chen (VBA Programming Specialist, Code Masters). “This error can also stem from attempting to select a range that has been deleted or is out of bounds. It is crucial to validate the range before selection and to handle potential exceptions gracefully to prevent runtime errors. Implementing error handling routines can enhance the robustness of your VBA scripts.”
Sarah Johnson (Excel Consultant, Data Insights Group). “Users often overlook that the ‘Select’ method is not always necessary in VBA. Instead of selecting a range, directly manipulating the range object can lead to more efficient code. For instance, instead of using ‘Range(“A1”).Select’, it is more effective to directly assign values or formats to ‘Range(“A1″)’ without selecting it first.”
Frequently Asked Questions (FAQs)
What does the error “select method of range class failed” indicate?
This error typically indicates that there is an issue with the specified range in a spreadsheet application, often due to incorrect referencing or an attempt to access a range that does not exist.
What are common causes for the “select method of range class failed” error?
Common causes include attempting to select a range on a worksheet that is not active, referencing a range that is outside the bounds of the worksheet, or using an invalid range address.
How can I resolve the “select method of range class failed” error in Excel VBA?
To resolve this error, ensure that the worksheet containing the range is activated before selecting it. Additionally, verify that the range address is correct and falls within the valid limits of the worksheet.
Is there a way to avoid the “select method of range class failed” error in my code?
Yes, you can avoid this error by directly manipulating the range object without using the Select method. For example, instead of selecting a range, you can directly set values or properties on the range object.
Can this error occur in other programming environments besides Excel VBA?
Yes, similar errors can occur in other programming environments that involve manipulating ranges or collections, such as Google Sheets scripts or other spreadsheet automation tools.
What should I do if the error persists despite troubleshooting?
If the error persists, consider reviewing your code for logical errors, checking for any hidden sheets or ranges, and ensuring that your application is updated to the latest version to avoid bugs related to range handling.
The error message “select method of range class failed” typically arises in Microsoft Excel when a user attempts to reference a range of cells that is not valid or accessible. This can occur due to various reasons, including the specified range being outside the current worksheet, the worksheet being protected, or the range being improperly defined in the code. Understanding the context in which this error occurs is crucial for troubleshooting and resolving the issue effectively.
One of the primary takeaways from the discussion surrounding this error is the importance of validating the range before attempting to select it programmatically. Users should ensure that the range exists within the active worksheet and that there are no typographical errors in the range definition. Additionally, checking for any worksheet protection settings that may prevent selection is essential in avoiding this error.
Furthermore, implementing error handling in the code can significantly improve the user experience by providing informative feedback when an error occurs. This proactive approach allows users to diagnose issues more efficiently and avoid disruptions in their workflow. Overall, a thorough understanding of range references and proper coding practices can help mitigate the occurrence of the “select method of range class failed” error in Excel.
Author Profile
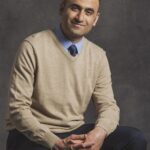
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?