Does Using Set Object Break Excel VBA Functionality?
Excel VBA (Visual Basic for Applications) is a powerful tool that allows users to automate tasks and enhance the functionality of their spreadsheets. However, even the most seasoned developers can encounter unexpected challenges, particularly when it comes to managing objects within their code. One common issue that arises is the infamous “set object breaks” error. This seemingly innocuous phrase can lead to frustration and confusion, leaving users wondering where they went wrong. In this article, we will explore the intricacies of object handling in Excel VBA, shedding light on the causes of these errors and offering practical solutions to prevent them.
When working with objects in Excel VBA, understanding the nuances of object references is crucial. A “set object breaks” error typically occurs when the code attempts to reference an object that has not been properly initialized or has been inadvertently altered. This can happen for a variety of reasons, including scope issues, improper object assignments, or even changes in the underlying data structure. As users delve deeper into the world of VBA, they may find themselves navigating a complex landscape of object manipulation, where a single misstep can lead to code failures.
Moreover, the implications of these errors extend beyond mere inconvenience; they can disrupt workflows and lead to significant time loss. By gaining a clearer understanding of how to
Understanding Set Objects in Excel VBA
In Excel VBA, the `Set` statement is essential for assigning object references to variables. This allows for efficient manipulation of Excel objects, such as workbooks, worksheets, ranges, and charts. However, improper use of the `Set` statement can lead to various issues, including runtime errors and unexpected behavior in macros.
When using the `Set` keyword, it is important to remember that it is only applicable to object variables. For example, you can use `Set` to assign a worksheet object to a variable, but not for primitive data types such as integers or strings.
“`vba
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets(“Sheet1”)
“`
If you attempt to use `Set` with a non-object variable, VBA will throw a “Type mismatch” error.
Common Issues with Set Objects
Several common problems can arise when working with `Set` objects in Excel VBA:
- Object Not Set: This error occurs when you try to access a property or method of an object that has not been assigned a reference. For example:
“`vba
Dim ws As Worksheet
Debug.Print ws.Name ‘ This will cause an error.
“`
- Incorrect Object Type: Assigning an object to a variable of the wrong type can lead to runtime errors. Always ensure the object you are assigning matches the variable’s declared type.
- Scope Issues: An object variable defined within a procedure is not accessible outside that procedure unless declared at a module level. This can cause confusion when trying to use the object elsewhere in your code.
Best Practices for Using Set Objects
To avoid issues when using `Set` objects in Excel VBA, consider the following best practices:
- Always declare object variables explicitly with the appropriate type.
- Use error handling to manage runtime errors gracefully.
- Ensure that the object is initialized before use.
- Clean up object references when they are no longer needed using `Set objectVariable = Nothing`.
Here is a simple illustration of these best practices:
“`vba
Dim ws As Worksheet
On Error Resume Next ‘ Enable error handling
Set ws = ThisWorkbook.Sheets(“Sheet1”)
If ws Is Nothing Then
MsgBox “Sheet1 does not exist.”
Else
‘ Proceed to use ws
End If
Set ws = Nothing ‘ Clean up
“`
Table of Common Object Types and Their Usages
Object Type | Usage |
---|---|
Workbook | Represents an Excel workbook. Used to open, close, or manipulate workbooks. |
Worksheet | Represents a single sheet within a workbook. Used to access data and methods related to that sheet. |
Range | Represents a cell or a collection of cells. Used to read or write data. |
Chart | Represents a chart object. Used for creating and manipulating charts. |
By adhering to these practices and understanding the intricacies of the `Set` statement, you can effectively leverage Excel VBA’s powerful object model while minimizing errors and maximizing efficiency.
Understanding Set Object in Excel VBA
In Excel VBA, the `Set` statement is used to assign an object reference to a variable. This is crucial for working with objects such as ranges, worksheets, or other Excel entities. Misusing the `Set` statement can lead to runtime errors or unexpected behavior in your VBA code.
Common Issues with Set Object
Errors related to the `Set` statement typically arise from:
- Incorrect Object Type: Assigning an object type that does not match the variable’s declared type.
- Uninitialized Objects: Attempting to use an object variable that has not been set to an instance of an object.
- Scope Conflicts: Using an object variable outside its defined scope.
Examples of Set Object Misuse
Consider the following examples that demonstrate common pitfalls when using the `Set` statement.
Example 1: Uninitialized Object
“`vba
Dim ws As Worksheet
ws.Name = “Sheet1” ‘ This will raise an error because ws is not set.
“`
Example 2: Incorrect Object Type
“`vba
Dim rng As Range
Set rng = “A1” ‘ This will raise a type mismatch error.
“`
Best Practices for Using Set Object
To avoid issues with the `Set` statement, consider the following best practices:
- Always Initialize Objects: Ensure that you set your object variables before using them.
“`vba
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets(“Sheet1”)
“`
- Use the Correct Object Type: Always match the variable type with the object being assigned.
“`vba
Dim rng As Range
Set rng = ws.Range(“A1”)
“`
- Check for Nothing: Before using an object, check if it is set to `Nothing` to prevent errors.
“`vba
If Not ws Is Nothing Then
ws.Activate
End If
“`
Debugging Set Object Errors
When encountering errors with the `Set` statement, employ the following debugging techniques:
Technique | Description |
---|---|
Use Debug.Print | Print object values to the Immediate Window to trace issues. |
Step Through Code | Use the VBA debugger to step through your code line by line. |
Check Variable Types | Ensure your variables are declared with the correct types. |
Conclusion on Set Object Usage
Proper usage of the `Set` statement is vital for effective Excel VBA programming. By adhering to best practices and employing debugging techniques, you can minimize errors and enhance the reliability of your code.
Understanding the Challenges of Set Object Breaks in Excel VBA
Dr. Emily Carter (Senior Software Engineer, Excel Solutions Corp.). “The use of ‘Set’ in Excel VBA is crucial for object manipulation. When a set object breaks, it often indicates that the reference to the object is lost, which can lead to runtime errors. It is essential to ensure that the object is properly instantiated before using it in your code.”
Michael Chen (VBA Developer, Data Insights Group). “One common issue with set object breaks in Excel VBA arises from scope problems. If an object is declared in a procedure and then accessed outside of that procedure without proper handling, it can result in a broken reference. Developers should carefully manage object lifetimes to avoid such pitfalls.”
Sarah Thompson (Excel VBA Specialist, Tech Innovations Inc.). “Debugging set object breaks can be challenging, particularly in complex applications. It is advisable to utilize error handling techniques and to regularly check object states before performing operations on them. This proactive approach can significantly reduce the occurrence of runtime errors.”
Frequently Asked Questions (FAQs)
What does the “Set object breaks” error mean in Excel VBA?
The “Set object breaks” error in Excel VBA typically indicates that an attempt was made to assign an object to a variable without properly using the `Set` keyword. This error occurs when trying to assign an object reference incorrectly.
How can I resolve the “Set object breaks” error in my VBA code?
To resolve the error, ensure that you use the `Set` keyword when assigning object variables. For example, instead of `myObject = New Object`, use `Set myObject = New Object`.
Are there any common scenarios that lead to “Set object breaks” errors?
Common scenarios include forgetting to use `Set` when assigning objects, attempting to assign a non-object value to an object variable, or referencing an object that has not been properly instantiated.
Can “Set object breaks” errors occur with built-in Excel objects?
Yes, “Set object breaks” errors can occur with built-in Excel objects such as `Workbook`, `Worksheet`, or `Range` if the assignment does not include the `Set` keyword.
Is there a way to debug “Set object breaks” errors effectively?
To debug effectively, use the VBA debugger to step through your code line by line. Pay special attention to object assignments and ensure that the `Set` keyword is used appropriately.
What are the best practices to avoid “Set object breaks” errors in Excel VBA?
Best practices include always using `Set` for object assignments, checking object initialization before use, and employing error handling to manage unexpected issues.
In the realm of Excel VBA, the use of set objects is crucial for managing and manipulating collections of data effectively. However, improper handling of set objects can lead to various issues, including runtime errors and unexpected behavior in macros. Understanding how to properly declare, assign, and manipulate set objects is essential for any VBA developer to avoid breaking their code and to ensure smooth execution of their applications.
One of the key insights is that set objects in VBA, such as collections and dictionaries, require careful management of memory and references. When a set object is not appropriately initialized or is inadvertently altered, it can lead to errors that disrupt the flow of the program. Therefore, it is vital for developers to implement error handling and validation checks to safeguard against such occurrences.
Additionally, leveraging best practices in coding, such as using meaningful variable names and maintaining a clear structure in code, can significantly reduce the likelihood of errors related to set objects. By adhering to these principles, developers can create more robust and maintainable VBA applications that minimize the risk of breaking due to improper handling of set objects.
Author Profile
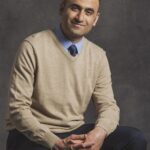
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?