How Can You Store and Read Data in Shadertoy?
In the ever-evolving world of computer graphics, Shadertoy stands out as a vibrant platform that empowers artists and developers to create stunning visual effects using shaders. One of the most intriguing aspects of Shadertoy is its ability to store and read data, enabling creators to push the boundaries of what’s possible in real-time rendering. Whether you’re a seasoned shader programmer or a curious newcomer, understanding how to manipulate data within Shadertoy can unlock a new realm of creativity and interactivity in your projects. In this article, we will explore the fundamental concepts of data storage and retrieval in Shadertoy, providing you with the tools to enhance your visual creations.
Shadertoy utilizes a unique architecture that allows shaders to not only render visuals but also manage and manipulate data efficiently. By leveraging textures and buffers, users can store information such as colors, coordinates, and even complex mathematical computations that can be accessed in subsequent frames. This capability transforms static images into dynamic experiences, where the state of the scene can evolve over time based on user input or pre-defined algorithms. As we delve deeper into the intricacies of data handling in Shadertoy, you’ll discover how these techniques can elevate your shader creations from simple graphics to immersive, interactive environments.
Moreover
Storing Data in Shadertoy
In Shadertoy, storing data can be achieved through the use of textures. Textures in Shadertoy serve as a means to hold and manipulate data across frames. By utilizing fragment shaders, users can write to textures and subsequently read from them in future frames, creating a dynamic feedback loop that can be leveraged for various visual effects and simulations.
To store data in a texture:
- Create a new texture in the Shadertoy interface.
- Use the `imageStore` function to write data into the texture.
- Specify the coordinates and the data you want to store.
For example, to store a color value in a texture:
“`glsl
vec4 colorValue = vec4(1.0, 0.0, 0.0, 1.0); // Red color
imageStore(iChannel0, ivec2(gl_FragCoord.xy), colorValue);
“`
This code snippet stores a red color at the current fragment’s coordinates in the texture bound to `iChannel0`.
Reading Data in Shadertoy
Reading data from textures in Shadertoy is performed using the `texture` function. This function retrieves the value stored in a texture at specified coordinates, allowing for dynamic visual effects based on previously stored data.
To read data from a texture:
- Use the `texture` function with the appropriate sampler and coordinates.
- The output will be a vector representing the color or value stored at that location.
For instance, to read the color value from a texture:
“`glsl
vec4 storedValue = texture(iChannel0, gl_FragCoord.xy / iResolution.xy);
“`
This will fetch the color value stored in the texture based on the current fragment’s coordinates.
Data Storage Techniques
When working with data in Shadertoy, consider the following techniques to optimize storage and retrieval:
- Multiple Channels: Utilize different channels (iChannel0, iChannel1, etc.) for storing various types of data.
- Mipmap Levels: Use mipmaps to store data at different resolutions, improving performance when sampling.
- Dynamic Resizing: Adjust texture sizes based on the requirements of your shader to manage GPU memory effectively.
Technique | Description |
---|---|
Multiple Channels | Store different datasets in separate textures to maintain organization. |
Mipmap Levels | Utilize mipmaps for better performance and quality when sampling textures. |
Dynamic Resizing | Modify texture dimensions according to the shader’s needs to optimize memory usage. |
By understanding the methods of storing and reading data in Shadertoy, users can create intricate visual effects and simulations that rely on dynamic data manipulation, enhancing the capabilities of their shaders.
Storing Data in Shadertoy
Shadertoy provides a unique environment for working with shaders where data can be stored and accessed effectively, primarily through the use of textures and buffers. Here are the main methods for data storage:
- Textures:
- Textures can be used to store pixel data. In Shadertoy, you can create textures using the `iChannel` inputs, which allows you to read from and write to textures.
- The data stored in textures can be manipulated in various ways, making them versatile for different applications, such as rendering or computational tasks.
- Buffers:
- Shadertoy supports buffer textures, which allow for storing and reading data in a structured way.
- Buffers can be utilized to maintain state information between frames, thus enabling more complex interactions and data handling.
- Uniforms:
- Uniforms are constants that remain unchanged for all processed fragments in a single draw call.
- They can be used to pass data from the host application to the shader, allowing for dynamic control over various shader parameters without needing to redraw.
Reading Data in Shadertoy
Reading data in Shadertoy is primarily accomplished through the fragment shader, where various inputs are available to access stored data. The following are key components for reading data:
- iChannel Inputs:
- `iChannel0`, `iChannel1`, etc., represent texture inputs that can be accessed within the shader.
- Each channel can be set to a different texture, allowing complex data retrieval.
- Texture Sampling:
- Use the `texture()` function to sample data from a texture. This function takes coordinates as parameters to retrieve pixel data.
- Example:
“`glsl
vec4 color = texture(iChannel0, uv);
“`
- Buffer Access:
- Buffers can be accessed similarly to textures but often require specific setup in Shadertoy.
- The use of buffer textures allows for fetching and writing data efficiently over multiple frames.
- Using Coordinates:
- The coordinates used for accessing data should be appropriately normalized (e.g., from 0 to 1) to correspond with texture dimensions.
- For example, using `gl_FragCoord.xy / resolution.xy` for normalized coordinates.
Example Workflow for Data Storage and Retrieval
The following table outlines a basic workflow for storing and reading data in Shadertoy using textures and buffers:
Step | Description |
---|---|
1. Setup Texture/Buffer | Initialize a texture or buffer to store data. Use `iChannel` for textures. |
2. Write Data | In the fragment shader, write data to the texture using the `gl_FragCoord` values. |
3. Read Data | Use the `texture()` function to read from the texture in subsequent frames. |
4. Update and Render | Update the texture or buffer as needed and render the output. |
By following these steps, users can effectively manage data flow in Shadertoy, creating dynamic and interactive visual experiences.
Expert Insights on Storing and Reading Data in Shadertoy
Dr. Elena Martinez (Computer Graphics Researcher, Visual Computing Lab). “In Shadertoy, storing and reading data efficiently is crucial for creating complex visual effects. Utilizing textures as buffers allows developers to maintain state across frames, enabling dynamic interactions and more intricate visualizations.”
James T. Hargrove (Senior Graphics Engineer, GameDev Innovations). “The key to effective data management in Shadertoy lies in the use of uniform variables and textures. By leveraging these elements, developers can store intermediate results and retrieve them seamlessly, which enhances performance and visual fidelity.”
Linda Chen (Shader Development Specialist, Creative Technologies Inc.). “Understanding how to manipulate framebuffers in Shadertoy is essential for advanced data storage techniques. This allows for the accumulation of data over time, enabling effects such as trails and motion blur that are both visually appealing and computationally efficient.”
Frequently Asked Questions (FAQs)
How can I store data in Shadertoy?
Data can be stored in Shadertoy using the built-in `iChannel` variables, which allow you to read from and write to textures. By rendering to a texture in one pass and then using that texture in subsequent passes, you can effectively store and manipulate data over multiple frames.
What types of data can I store in Shadertoy?
Shadertoy primarily supports texture data, which can represent various types of information such as colors, depth, or custom data formats. You can encode numerical data into texture channels, allowing for flexible data storage and retrieval.
How do I read data from a texture in Shadertoy?
To read data from a texture in Shadertoy, you can use the `texture()` function, providing the texture sampler and UV coordinates. This function retrieves the color value stored at the specified coordinates, allowing you to access the data for further processing.
Can I store and read data between different shaders in Shadertoy?
No, data cannot be directly shared between different shaders in Shadertoy. Each shader operates in isolation. However, you can use the same texture as an input across multiple shaders by passing it through the `iChannel` variables, effectively sharing data indirectly.
What is the maximum texture size I can use for data storage in Shadertoy?
The maximum texture size in Shadertoy is typically 4096×4096 pixels, depending on the user’s GPU capabilities. It is advisable to optimize texture usage to ensure performance and avoid exceeding limits, especially when storing large amounts of data.
Are there any performance considerations when storing and reading data in Shadertoy?
Yes, performance can be affected by the frequency of texture reads and writes, as well as the complexity of the shader calculations. Minimizing the number of texture accesses and optimizing shader code can significantly enhance performance while working with data storage in Shadertoy.
In the realm of Shadertoy, storing and reading data is a crucial aspect that allows developers to create complex visual effects and interactive experiences. Shadertoy provides various methods for managing data, including the use of textures and buffers. These methods enable users to store information such as colors, positions, and other parameters that can be accessed and manipulated in real-time within shaders. The ability to read and write data efficiently is fundamental for achieving dynamic visual outputs and enhancing the overall performance of shader programs.
One of the key insights from the discussion on data management in Shadertoy is the importance of utilizing textures as a means of storing intermediate results. By leveraging framebuffers and textures, developers can create a feedback loop that allows for intricate animations and effects. This technique is particularly beneficial for effects that require multiple passes, such as procedural generation or complex simulations. Moreover, understanding how to effectively read from and write to these textures can significantly optimize shader performance.
Additionally, the use of uniform variables and global variables plays a pivotal role in data management within Shadertoy. Uniforms allow for the passing of data from the host application to the shader, while global variables can store state information that persists across frames. This dual approach enables developers
Author Profile
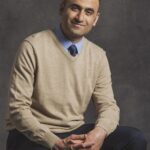
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?