How to Handle a Single-Row Subquery That Returns More Than One Row?
In the world of SQL and database management, subqueries play a pivotal role in crafting efficient and powerful queries. However, one common pitfall that developers encounter is the infamous “single-row subquery returns more than one row” error. This seemingly innocuous message can halt your progress and leave you scratching your head, especially when you’re trying to extract precise data from a complex database. Understanding the nuances of subqueries and the implications of this error is crucial for anyone looking to master SQL and optimize their data retrieval strategies.
At its core, a single-row subquery is designed to return only one row and one column of data. When it unexpectedly returns multiple rows, it triggers an error that can disrupt your entire query. This situation often arises from misunderstandings about the underlying data or the logic applied in the subquery itself. By examining the reasons behind this error and the contexts in which it occurs, developers can gain valuable insights into their database structure and improve their query-writing skills.
Navigating the intricacies of SQL requires not only technical knowledge but also an understanding of how to effectively structure queries to avoid common mistakes. In this article, we will explore the causes of the “single-row subquery returns more than one row” error, its implications for database operations, and best practices for
Understanding Single-Row Subqueries
Single-row subqueries are a type of nested query that is designed to return a single value from the database. When you use a single-row subquery in SQL, it is essential to ensure that the subquery returns only one row; otherwise, an error will occur.
In SQL, a common mistake is using a single-row subquery that inadvertently returns multiple rows. This can lead to runtime errors, which can disrupt the execution of your query. To avoid this, it’s crucial to understand how to structure your subqueries correctly.
Common Causes of Multiple Rows in Subqueries
Several factors can lead to a single-row subquery returning more than one row:
- Lack of Unique Constraints: If the condition specified in the subquery does not uniquely identify a single row, multiple rows will be returned.
- Incorrect Use of Aggregation Functions: Not employing aggregation functions (like `MAX`, `MIN`, `COUNT`) when necessary can result in the subquery fetching multiple records.
- Inadequate Filtering Conditions: Failing to apply stringent filtering conditions can cause the subquery to return more data than intended.
Strategies to Prevent Errors
To ensure that your single-row subquery returns exactly one row, consider implementing the following strategies:
- Use of Aggregation Functions: If you expect to retrieve a specific value, use aggregation functions to condense multiple rows into a single result.
- Adding Conditions: Enhance your filtering conditions by utilizing `WHERE` clauses to restrict the results to a single row.
- Implementing `LIMIT`: In some SQL dialects, you can append a `LIMIT 1` clause to your subquery to forcibly limit the result set to one row.
Example Scenarios
Here are some examples illustrating how to construct single-row subqueries properly:
“`sql
SELECT employee_id,
(SELECT MAX(salary)
FROM employees
WHERE department_id = 10) AS max_salary
FROM employees
WHERE employee_id = 1;
“`
In this example, the subquery is safe because it uses the `MAX` function to ensure that only one value is returned.
When to Use Single-Row Subqueries
Single-row subqueries are most useful in scenarios where you need to compare each row of a main query against a specific value. They can be effectively used in:
- Filter Conditions: To filter results based on a single value derived from another table.
- Calculations: To compute derived metrics that are unique to a specific context.
Table: Common SQL Errors with Subqueries
Error Type | Cause | Solution |
---|---|---|
Subquery returns more than one row | Lack of unique filtering | Refine the WHERE clause |
Aggregation error | Missing aggregation function | Add MAX, MIN, etc. |
Performance issues | Complex subqueries | Consider using joins |
By following these guidelines, you can minimize the risk of encountering errors associated with single-row subqueries in SQL.
Understanding Single-Row Subqueries
A single-row subquery is designed to return a single value or a single row of data. When a subquery returns more than one row, it can lead to errors in SQL statements, particularly in scenarios where a single value is expected.
Common Scenarios Causing Multiple Row Returns
Several factors can contribute to a single-row subquery returning multiple rows:
- Inadequate Filtering: The WHERE clause may not be specific enough, allowing multiple records to meet the criteria.
- Non-Unique Values: The subquery is referencing a column that has duplicate values.
- Join Misconfiguration: Incorrect joins may inadvertently pull in multiple records.
Error Handling in SQL
When a single-row subquery inadvertently returns multiple rows, SQL engines typically raise an error. This error can take various forms, depending on the specific database system being used. Common error messages include:
Database System | Error Message |
---|---|
Oracle | “ORA-01427: single-row subquery returns more than one row” |
MySQL | “Subquery returns more than 1 row” |
SQL Server | “Subquery returned more than 1 value” |
PostgreSQL | “ERROR: more than one row returned by a subquery used as an expression” |
Best Practices to Avoid Errors
To prevent a single-row subquery from returning more than one row, consider the following best practices:
- Use Aggregation Functions: Functions like `MAX()`, `MIN()`, or `COUNT()` can condense multiple rows into a single value.
- Ensure Uniqueness: Use DISTINCT to filter out duplicate results or ensure the primary key or unique constraint is applied.
- Limit Results: Use the `LIMIT` clause to restrict the number of rows returned.
- Validate Conditions: Confirm that the conditions in the WHERE clause are precise enough to yield a single result.
Example of a Single-Row Subquery
Consider the following SQL statement:
“`sql
SELECT employee_id,
(SELECT department_id
FROM departments
WHERE department_name = ‘Sales’) AS dept_id
FROM employees;
“`
If there are multiple departments named ‘Sales’, this query will cause an error. To fix it, ensure the subquery returns only one row:
“`sql
SELECT employee_id,
(SELECT department_id
FROM departments
WHERE department_name = ‘Sales’
LIMIT 1) AS dept_id
FROM employees;
“`
Conclusion on Managing Single-Row Subquery Errors
Understanding how to manage single-row subqueries is crucial for writing efficient SQL queries. By employing the appropriate strategies and best practices outlined above, you can minimize the risk of encountering multiple-row returns and ensure your queries function as intended.
Understanding the Implications of Single-Row Subqueries Returning Multiple Rows
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When a single-row subquery unexpectedly returns multiple rows, it indicates a fundamental issue in the query design. This often leads to runtime errors, as the SQL engine cannot process multiple results where a single value is expected. It is crucial to review the logic of the subquery and ensure that it is structured to return only one row, typically by using aggregation functions or refining the WHERE clause.”
Michael Thompson (Senior Data Analyst, Analytics Solutions Group). “In practice, encountering a situation where a single-row subquery returns more than one row can disrupt data integrity and application performance. Developers should implement error handling to manage such scenarios gracefully. Additionally, employing techniques like EXISTS or IN can help avoid these pitfalls by ensuring the subquery aligns with the intended logic of the main query.”
Linda Garcia (SQL Consultant, Database Dynamics). “The occurrence of a single-row subquery returning multiple rows often suggests a misunderstanding of the dataset or the relationships between tables. To mitigate this, it is essential to conduct thorough data analysis and testing. Utilizing tools like EXPLAIN can help visualize the query execution plan and identify where the logic may be failing, allowing for more effective troubleshooting and optimization.”
Frequently Asked Questions (FAQs)
What does it mean when a single-row subquery returns more than one row?
A single-row subquery is designed to return exactly one row. If it returns more than one row, it indicates a logical error in the query, as the context requires a single value for comparison or assignment.
What are common causes for a single-row subquery returning multiple rows?
Common causes include insufficient filtering criteria, incorrect joins, or using aggregate functions improperly. These issues can lead to multiple records being selected when only one is expected.
How can I troubleshoot a single-row subquery that returns more than one row?
To troubleshoot, review the subquery’s WHERE clause and ensure it includes sufficient conditions to limit results. Additionally, consider using aggregate functions or modifying the logic to ensure a single row is returned.
What are the consequences of using a single-row subquery that returns multiple rows in a SQL statement?
Using a single-row subquery that returns multiple rows will result in an error, typically stating that the subquery returned more than one value. This prevents the main query from executing successfully.
Can I convert a single-row subquery that returns multiple rows into a valid query?
Yes, you can convert it by either modifying the subquery to ensure it returns a single row, or by using a different approach, such as using an `IN` clause or a `JOIN`, depending on the desired outcome.
What are some best practices to avoid single-row subqueries returning multiple rows?
Best practices include using explicit filtering conditions, validating the expected output of subqueries, employing aggregate functions when necessary, and testing queries incrementally to ensure they produce the intended results.
A single-row subquery is designed to return only one row of results. When a subquery returns more than one row, it leads to an error in the main query, as the database engine cannot process multiple values where a single value is expected. This situation often arises in SQL statements that use operators such as ‘=’, ‘<', or '>‘. Understanding the implications of this behavior is crucial for database management and query optimization.
To prevent errors associated with single-row subqueries, developers can utilize various strategies. One common approach is to ensure that the subquery is appropriately structured to return only a single row, often by using aggregate functions like MAX() or MIN(), or by employing filtering conditions that narrow down the results. Alternatively, using multi-row subqueries with operators like IN or EXISTS can effectively handle scenarios where multiple results are anticipated.
Moreover, proper error handling and testing are essential when working with subqueries. Developers should validate the expected output of subqueries during the development phase to identify potential issues early. Additionally, leveraging database tools that provide insights into query performance can help in diagnosing and resolving problems related to subquery results.
Author Profile
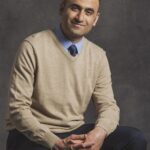
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?