How Can You Resolve the Issue of Multiple SLF4J Bindings in Your Class Path?
In the world of Java development, logging is an essential aspect that can significantly impact the performance and maintainability of applications. Among the myriad of logging frameworks available, SLF4J (Simple Logging Facade for Java) stands out as a popular choice due to its versatility and ease of use. However, while integrating SLF4J into your project, you may encounter a common yet perplexing issue: the warning that your class path contains multiple SLF4J bindings. This situation can lead to confusion and unexpected behavior in your application, making it crucial for developers to understand the implications and resolutions surrounding this warning.
As you delve deeper into the intricacies of SLF4J, you’ll discover that the presence of multiple bindings can create conflicts that hinder your application’s logging capabilities. Each binding represents a different logging implementation, and when more than one is detected, it can result in unpredictable logging behavior or even runtime errors. Understanding the root causes of this issue is vital for maintaining a clean and efficient logging setup.
Moreover, resolving the multiple bindings issue not only enhances your application’s performance but also simplifies debugging and monitoring processes. By ensuring that only the desired SLF4J binding is present in your class path, you can streamline your logging framework and provide a more consistent experience
Understanding SLF4J Binding Conflicts
When using SLF4J (Simple Logging Facade for Java), you may encounter a warning indicating that your class path contains multiple SLF4J bindings. This situation arises when more than one logging implementation is present in your project, which can lead to unpredictable logging behavior and runtime exceptions.
The SLF4J library is designed to allow developers to plug in their desired logging framework. However, if multiple bindings are found, SLF4J will choose one of them arbitrarily, often leading to confusion and inconsistencies in log outputs.
Common Causes of Multiple Bindings
There are several reasons you might find multiple SLF4J bindings in your project:
- Transitive Dependencies: When you include a library that itself has SLF4J as a dependency, this can inadvertently introduce additional bindings.
- Multiple Logging Implementations: Including more than one logging backend (e.g., Logback, Log4j, java.util.logging) can result in conflicts.
- Incorrect Dependency Management: If you manually manage your dependencies without properly excluding unwanted bindings, you may accidentally include them.
Identifying and Resolving Binding Issues
To resolve issues related to multiple SLF4J bindings, follow these steps:
- Check the Warning Message: The SLF4J warning message typically provides details about the bindings found in the class path. Review this message to identify the conflicting bindings.
- Review Dependencies: Use tools like Maven or Gradle to analyze your project dependencies. For Maven, you can run:
“`bash
mvn dependency:tree
“`
This command will display all dependencies and their transitive dependencies, helping you spot conflicts.
- Exclude Unwanted Bindings: Once you identify the conflicting bindings, you can exclude them. For example, in Maven, you can exclude a dependency like this:
“`xml
“`
- Verify Single Binding: Ensure that only one SLF4J binding remains after resolving conflicts. The preferred binding can often be Logback, as it is the native implementation of SLF4J.
Recommended SLF4J Bindings
Choosing the right SLF4J binding is crucial for achieving optimal logging capabilities. Below is a comparison of some commonly used SLF4J bindings:
Binding | Description | Notes |
---|---|---|
Logback | The native implementation of SLF4J; highly configurable. | Recommended for most applications. |
Log4j | A popular logging library offering flexibility. | Requires additional setup for SLF4J integration. |
java.util.logging | Built-in Java logging framework. | Less recommended due to limited features. |
By carefully managing your dependencies and ensuring that only one SLF4J binding is present, you can avoid logging conflicts and maintain a clear and consistent logging framework within your application.
Understanding SLF4J Bindings
SLF4J (Simple Logging Facade for Java) is a popular logging abstraction in Java applications. It allows developers to plug in various logging frameworks such as Log4j, Logback, or java.util.logging. However, when multiple SLF4J bindings are present on the class path, it can lead to confusion and runtime issues. This section delves into the implications and best practices regarding SLF4J bindings.
Implications of Multiple Bindings
Having multiple SLF4J bindings on the class path can result in:
- Ambiguity: It becomes unclear which logging framework will be used for logging events, leading to unpredictable behavior.
- Performance Overhead: The presence of multiple bindings can introduce unnecessary overhead during application startup as SLF4J attempts to load each binding.
- Runtime Exceptions: In some cases, applications may throw exceptions due to conflicting bindings or incompatibilities.
Identifying Multiple Bindings
To identify multiple SLF4J bindings in your project, you can take the following steps:
- Check the Class Path: Review the libraries included in your build tool (e.g., Maven, Gradle) to see which logging frameworks are present.
- Run SLF4J’s Diagnostic Utility: SLF4J provides a diagnostic utility that can help identify the bindings:
- Include the SLF4J API in your project.
- Execute the application and observe the logs; SLF4J will typically log a warning message if multiple bindings are detected.
Resolving Binding Conflicts
To resolve conflicts arising from multiple SLF4J bindings, consider the following strategies:
- Exclude Unwanted Dependencies: Use dependency management features of your build tool to exclude unnecessary logging dependencies. For example, in Maven, you can use:
“`xml
“`
- Choose a Single Binding: Select one logging framework to use with SLF4J and ensure all other bindings are removed from your project.
Common SLF4J Bindings
Here is a table summarizing popular SLF4J bindings and their corresponding logging frameworks:
SLF4J Binding | Logging Framework | Description |
---|---|---|
slf4j-log4j12 | Log4j | Integrates SLF4J with Log4j 1.x. |
slf4j-log4j2 | Log4j 2.x | Integrates SLF4J with Log4j 2.x. |
slf4j-simple | Simple Logger | A simple implementation for testing purposes. |
slf4j-jdk14 | java.util.logging | Integrates SLF4J with Java’s built-in logging. |
slf4j-nop | No Operation | Ignores all logging calls (useful for disabling logging). |
Best Practices
To maintain a clean logging configuration, adhere to these best practices:
- Regularly audit your dependencies for unnecessary logging frameworks.
- Utilize a dependency tree visualization tool (e.g., Maven’s `dependency:tree` command) to inspect the structure of your project’s dependencies.
- Document the chosen logging framework and its configuration to ensure consistency across development teams.
By following these guidelines, you can effectively manage SLF4J bindings and ensure that your logging setup remains robust and predictable.
Understanding SLF4J Binding Conflicts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The presence of multiple SLF4J bindings in the class path often leads to unpredictable logging behavior. It is crucial to ensure that only one binding is present to maintain a consistent logging framework across applications.”
Michael Chen (Lead Java Developer, Open Source Solutions). “When encountering the ‘multiple SLF4J bindings’ warning, developers should prioritize identifying and resolving the conflicting bindings. This not only enhances application performance but also simplifies the debugging process.”
Sarah Patel (Java Framework Specialist, CodeCraft Labs). “Managing SLF4J bindings is essential for effective logging. Utilizing dependency management tools like Maven or Gradle can help streamline this process and prevent binding conflicts from occurring.”
Frequently Asked Questions (FAQs)
What does it mean when the SLF4J class path contains multiple SLF4J bindings?
When the SLF4J class path contains multiple bindings, it indicates that multiple implementations of the SLF4J API are present in the class path, which can lead to unpredictable behavior. SLF4J allows only one binding to be active at a time.
How can I identify which SLF4J bindings are present in my class path?
You can identify the SLF4J bindings by examining the class path entries in your build configuration or by using tools like Maven or Gradle to list dependencies. Additionally, running your application will typically produce a warning message detailing the bindings found.
What are the potential issues caused by multiple SLF4J bindings?
Multiple SLF4J bindings can cause logging inconsistencies, unexpected behavior, and performance issues. The application may not log messages as intended, leading to difficulties in debugging and monitoring.
How can I resolve the issue of multiple SLF4J bindings?
To resolve this issue, you should remove all but one SLF4J binding from your class path. This can be done by modifying your build configuration files (e.g., pom.xml for Maven or build.gradle for Gradle) to exclude unnecessary dependencies.
What is the recommended binding for SLF4J?
The recommended binding for SLF4J depends on your logging framework preference. Common bindings include Logback, Log4j, and java.util.logging. Logback is often recommended due to its performance and feature set.
Can I use SLF4J with multiple logging frameworks simultaneously?
No, SLF4J is designed to work with one binding at a time. If you need to use multiple logging frameworks, consider using a logging facade or bridge that can route logging calls appropriately without causing conflicts.
The presence of multiple SLF4J bindings in the class path can lead to significant issues in Java applications that utilize the Simple Logging Facade for Java (SLF4J). When multiple bindings are detected, SLF4J will log a warning message indicating the conflict, which can result in unpredictable logging behavior. This situation arises when different libraries or dependencies include their own SLF4J bindings, creating ambiguity about which binding should be used for logging. Consequently, it is crucial to ensure that only one SLF4J binding is present in the class path at any given time to maintain consistent logging functionality.
To resolve the issue of multiple SLF4J bindings, developers should conduct a thorough audit of their project dependencies. This involves identifying all included libraries and their respective SLF4J bindings. Once the conflicting bindings are identified, developers can either exclude the unnecessary bindings from their dependencies or choose a single binding that meets their requirements. Utilizing build tools such as Maven or Gradle can facilitate this process by providing dependency management features that help to avoid such conflicts.
In summary, managing SLF4J bindings effectively is essential for ensuring reliable logging in Java applications. By maintaining a clean class path with a single SLF4J binding,
Author Profile
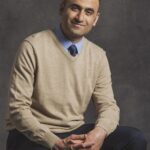
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?