Why Am I Seeing ‘SLF4J: No SLF4J Providers Were Found’ and How Can I Fix It?
In the world of Java development, logging is an essential aspect that can significantly influence the maintainability and debuggability of applications. However, developers often encounter the frustrating error message: “slf4j: no SLF4J providers were found.” This seemingly cryptic warning can halt progress and leave even seasoned programmers scratching their heads. Understanding the root causes of this error and how to resolve it is crucial for anyone looking to implement effective logging in their Java applications. In this article, we will delve into the intricacies of SLF4J (Simple Logging Facade for Java), explore why this error occurs, and provide practical solutions to ensure your logging framework functions seamlessly.
SLF4J serves as a simple facade or abstraction for various logging frameworks, allowing developers to plug in their preferred logging implementation without changing the codebase. However, the error indicating that no SLF4J providers were found typically arises when the application cannot locate an appropriate logging backend. This can occur due to missing dependencies, incorrect configurations, or even classpath issues. By understanding the components involved in SLF4J and how they interact with different logging frameworks, developers can better navigate these common pitfalls.
Moreover, resolving the “no SLF4J providers were found” error
Understanding SLF4J
SLF4J, or Simple Logging Facade for Java, serves as an abstraction layer for various logging frameworks. It allows developers to plug in their desired logging implementation without modifying the actual logging code. This flexibility is crucial in software development, particularly when dealing with multiple environments or frameworks that may require different logging strategies.
Common Causes of the “No SLF4J Providers Found” Warning
The warning message “no SLF4J providers were found” indicates that SLF4J cannot locate any binding implementations in the classpath. This typically occurs due to several reasons:
- Missing SLF4J Binding: The SLF4J API is present, but the specific logging backend (e.g., Logback, Log4j) is not included in the project.
- Incorrect Classpath Configuration: The binding might exist but is not correctly configured in the classpath, leading to SLF4J being unable to find it.
- Version Mismatch: There may be a compatibility issue between SLF4J and the logging implementation due to version differences.
- Multiple Bindings: Having multiple SLF4J bindings can also cause confusion, resulting in runtime errors.
Resolving the Issue
To resolve the “no SLF4J providers were found” warning, follow these steps:
- Add an SLF4J Binding: Ensure you include a compatible SLF4J binding in your project dependencies. Common bindings include:
- Logback: Recommended for modern applications.
- Log4j: Widely used but requires additional configuration.
- java.util.logging: Basic logging capabilities.
- Check Dependency Management: If using a build tool like Maven or Gradle, verify that the dependencies are correctly defined in your configuration files.
For Maven, your `pom.xml` might look like this:
“`xml
“`
For Gradle, your `build.gradle` might include:
“`groovy
implementation ‘org.slf4j:slf4j-api:1.7.30’
implementation ‘ch.qos.logback:logback-classic:1.2.3’
“`
- Review Classpath Settings: If you are running a Java application from the command line, ensure that the classpath includes the required SLF4J and its binding JAR files.
- Check for Conflicting Dependencies: Use tools like Maven’s `dependency:tree` or Gradle’s `dependencies` task to identify any conflicting versions of SLF4J or its bindings.
Example of Dependency Management
The following table summarizes common SLF4J bindings and their respective dependencies:
Binding | Group ID | Artifact ID | Version |
---|---|---|---|
Logback | ch.qos.logback | logback-classic | 1.2.3 |
Log4j 1.x | log4j | log4j | 1.2.17 |
Log4j 2.x | org.apache.logging.log4j | log4j-slf4j-impl | 2.14.1 |
JUL | org.slf4j | slf4j-jdk14 | 1.7.30 |
By following these guidelines and ensuring proper dependency inclusion, the warning regarding SLF4J providers should be effectively resolved, allowing for seamless logging in your applications.
Understanding the SLF4J Warning
The warning message `slf4j: no slf4j providers were found` indicates that the Simple Logging Facade for Java (SLF4J) is unable to locate a compatible logging implementation. This can result in logging functionality being disabled or limited, which might hinder debugging and monitoring efforts in your application.
Common Causes
Several factors can lead to this warning:
- Missing SLF4J Binding: The most common reason is the absence of an SLF4J binding in your classpath. SLF4J requires a specific binding (e.g., logback, log4j) to route logging calls.
- Incorrect Dependencies: If your project dependencies include SLF4J but not a compatible logging framework, the binding will not be available.
- Version Mismatch: Using incompatible versions of SLF4J and the logging implementations can cause this issue.
- Exclusions in Dependency Management: Sometimes, dependency management systems like Maven or Gradle may inadvertently exclude necessary logging implementations.
Resolutions
To resolve the warning, consider the following steps:
- Add a Binding: Ensure that a suitable SLF4J binding is included in your project dependencies. Common bindings include:
- Logback: Use `slf4j-logback` for a robust logging implementation.
- Log4j: Include `slf4j-log4j12` if you prefer Log4j.
- Java Util Logging: Use `slf4j-jdk14` for integration with Java’s built-in logging.
- Check Dependency Management: Verify your dependency management files (like `pom.xml` for Maven or `build.gradle` for Gradle) to ensure that required logging frameworks are not excluded.
- Update Versions: Ensure that you are using compatible versions of SLF4J and any bindings. You can check the SLF4J website or repository for compatibility matrices.
- Verify Classpath: Ensure that the logging library is in the classpath during runtime. You can check this through your IDE’s project settings or the build configuration.
Example Maven Configuration
Below is an example of how to include SLF4J with Logback in a Maven project:
“`xml
“`
Example Gradle Configuration
For a Gradle project, you can include SLF4J with Logback as follows:
“`groovy
dependencies {
implementation ‘org.slf4j:slf4j-api:1.7.32’
implementation ‘ch.qos.logback:logback-classic:1.2.6’
}
“`
Testing the Configuration
After modifying the dependencies, it is essential to validate that the logging framework is correctly set up. You can do this by:
- Adding Test Logging: Implement a simple logging statement in your application code, such as:
“`java
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApplication {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public static void main(String[] args) {
logger.info(“Logging is set up correctly.”);
}
}
“`
- Running the Application: Execute your application and check the output. If the logging statement is printed without any warnings, the configuration is successful.
Understanding the SLF4J Provider Issue
Dr. Emily Carter (Senior Software Engineer, JavaTech Solutions). “The error message ‘no SLF4J providers were found’ typically indicates that the SLF4J API is not properly linked to a concrete logging implementation. It is crucial to ensure that the correct SLF4J binding, such as slf4j-log4j12 or slf4j-simple, is included in your project dependencies.”
Michael Thompson (Lead Developer, Open Source Logging Initiative). “This issue often arises in environments where multiple logging frameworks are in use. Developers should verify their classpath to ensure that only one SLF4J binding is present to avoid conflicts and ensure that logging works as intended.”
Sarah Jenkins (Technical Consultant, Enterprise Java Solutions). “To resolve the ‘no SLF4J providers were found’ error, I recommend checking your build configuration files, such as Maven’s pom.xml or Gradle’s build.gradle, to confirm that the necessary dependencies for SLF4J and its implementations are correctly defined and up to date.”
Frequently Asked Questions (FAQs)
What does the error “slf4j: no slf4j providers were found” mean?
This error indicates that the SLF4J (Simple Logging Facade for Java) framework cannot find a suitable logging implementation in your classpath. SLF4J serves as a facade for various logging frameworks, and without a provider, logging calls will not function.
How can I resolve the “no slf4j providers were found” error?
To resolve this error, you need to include a compatible SLF4J binding in your project dependencies. Common bindings include SLF4J with Logback, Log4j, or java.util.logging. Ensure the appropriate library is added to your classpath.
What are some common SLF4J providers I can use?
Common SLF4J providers include Logback, Log4j 2, and JDK logging. Each of these logging frameworks can be used as a backend for SLF4J, providing the necessary implementation for logging functionality.
Can I use multiple SLF4J bindings in my project?
No, you should not include multiple SLF4J bindings in your project. Doing so can lead to conflicts and unpredictable behavior. Always ensure that only one logging implementation is present in your classpath.
How do I check if SLF4J is correctly set up in my project?
You can check your project’s dependencies to ensure that an SLF4J binding is included. Additionally, running your application and observing the logs for any SLF4J-related errors can help confirm proper setup.
What should I do if I have added a provider but still see the error?
If you have added a provider and still encounter the error, verify that the provider is compatible with the version of SLF4J you are using. Also, check for any classpath issues that may prevent the logging implementation from being recognized.
The message “SLF4J: No SLF4J providers were found” indicates that the Simple Logging Facade for Java (SLF4J) is unable to locate a suitable logging implementation in the classpath. This typically occurs when the SLF4J API is included in a project without a corresponding logging backend, such as Logback or Log4j. As a result, any logging calls made using the SLF4J API will not function, leading to potential difficulties in debugging and monitoring applications.
To resolve this issue, developers should ensure that a compatible SLF4J binding is included in their project dependencies. This can be achieved by adding the appropriate logging framework to the build configuration, whether using Maven, Gradle, or another dependency management tool. It is essential to verify that the versions of SLF4J and the chosen logging implementation are compatible to avoid further conflicts.
Additionally, understanding the logging requirements of the application is crucial. Different environments may necessitate different logging configurations, and developers should consider the implications of logging levels, output formats, and performance impacts. By addressing these factors, developers can effectively utilize SLF4J to enhance their application’s logging capabilities, ensuring that they have the necessary tools for effective
Author Profile
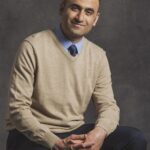
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?