How Does Spring JPA Hibernate DDL Auto Work and What Should You Know?
In the world of Java development, the combination of Spring, JPA, and Hibernate has become a powerful trio for building robust, data-driven applications. As developers strive for efficiency and simplicity, understanding how to manage database schemas effectively is crucial. One of the key features that can streamline this process is the `ddl-auto` setting in Spring JPA, which allows for automatic schema generation and management. This feature not only simplifies development but also enhances productivity, making it a topic worth exploring in depth.
At its core, the `ddl-auto` setting in Spring JPA and Hibernate offers various configurations that dictate how the underlying database schema is handled. From creating tables automatically to validating existing schemas, these options empower developers to choose the level of control they want over their database structure. Understanding the implications of each setting can significantly impact the development workflow, especially in environments where rapid iterations and testing are essential.
Moreover, the interplay between Spring, JPA, and Hibernate creates a seamless experience for developers, allowing them to focus on writing business logic rather than getting bogged down in database management. By leveraging the `ddl-auto` feature effectively, teams can ensure that their applications are not only functional but also maintainable and scalable. As we dive deeper into this topic, we will explore the various configurations
Understanding `ddl-auto` Settings in Spring JPA
In Spring JPA, the `ddl-auto` setting determines how the persistence provider (like Hibernate) handles the database schema. This configuration is crucial for managing the lifecycle of your database and ensuring that it aligns with your entity definitions. The values you can assign to `ddl-auto` include:
- none: No action will be performed on the database schema. This is suitable for production environments where the schema is managed manually.
- update: Hibernate will update the database schema to match the entity definitions. This can be useful during development but may lead to data loss if not handled carefully.
- create: This setting will drop the existing schema and create a new one each time the application starts. It is useful for testing but not recommended for production due to the potential loss of data.
- create-drop: Similar to `create`, but the schema will be dropped when the application shuts down. This is beneficial for integration tests where you want a fresh schema for each run.
- validate: Hibernate will validate the existing schema against the entity definitions but will not make any changes. This is useful for ensuring that your schema is in sync with your entities.
Configuring `ddl-auto` in Spring Boot
Setting the `ddl-auto` property is straightforward in a Spring Boot application. You can configure it in the `application.properties` or `application.yml` file. Below is an example of how to set the property in both formats.
application.properties:
“`properties
spring.jpa.hibernate.ddl-auto=update
“`
application.yml:
“`yaml
spring:
jpa:
hibernate:
ddl-auto: update
“`
Considerations When Using `ddl-auto`
When choosing a `ddl-auto` setting, it is vital to consider your environment and workflow:
- Development: Using `create` or `update` can speed up the development process by allowing rapid changes to the schema.
- Testing: `create-drop` is ideal for tests to ensure a clean state for each run.
- Production: Always opt for `none` or `validate` to prevent accidental data loss and ensure schema integrity.
Common Issues with `ddl-auto` Settings
Misconfigurations in the `ddl-auto` settings can lead to various issues, such as:
Issue | Description |
---|---|
Data Loss | Using `create` or `create-drop` in production can lead to loss of critical data. |
Schema Mismatches | An `update` setting may lead to inconsistencies if manual changes are made to the database. |
Application Startup Failures | Incorrect configurations can prevent the application from starting, especially with `validate` if mismatches are detected. |
By understanding these configurations and their implications, developers can effectively manage their database schemas in Spring JPA applications, ensuring both efficiency during development and safety in production environments.
Understanding DDL Auto in Spring JPA and Hibernate
The `ddl-auto` property in Spring JPA and Hibernate is crucial for defining how your entity model is managed in relation to the database schema. It simplifies database schema management in development and production environments.
Possible Values for DDL Auto
The `spring.jpa.hibernate.ddl-auto` property accepts several values, each influencing the schema management process in different ways:
- none: No action will be performed. The application will not change the database schema.
- validate: Hibernate validates the schema, ensuring that it matches the entity definitions. If discrepancies are found, an error is thrown.
- update: Hibernate updates the existing database schema without dropping any data. It modifies the schema based on the entity changes.
- create: Hibernate creates a new schema every time the application starts, dropping any existing data.
- create-drop: Similar to `create`, but also drops the schema when the session factory is closed, typically when the application shuts down.
Configuration Example
In a Spring Boot application, you can configure the `ddl-auto` setting in the `application.properties` or `application.yml` file as follows:
“`properties
application.properties
spring.jpa.hibernate.ddl-auto=update
“`
“`yaml
application.yml
spring:
jpa:
hibernate:
ddl-auto: update
“`
Impact on Development and Production
Choosing the right `ddl-auto` setting can significantly impact your development workflow and production stability. Here’s a brief overview of implications:
Value | Development Impact | Production Impact |
---|---|---|
none | No schema management. Manual intervention required. | Stable, but requires careful schema management. |
validate | Useful for ensuring model integrity during development. | Ensures schema matches entities before runtime. |
update | Convenient for iterative development and testing. | Risk of unintentional changes; use with caution. |
create | Good for starting fresh but leads to data loss. | Not recommended; can result in downtime and data loss. |
create-drop | Ideal for testing; cleans up after execution. | Not suitable for production; data loss on shutdown. |
Best Practices
To effectively utilize the `ddl-auto` property, consider the following best practices:
- Development: Use `update` or `create-drop` during development for flexibility.
- Testing: Employ `create-drop` to ensure a clean state before tests run.
- Production: Always use `none` or `validate` to avoid unintentional data loss or schema changes.
- Backup: Regularly back up your database, especially when using `create` or `update`.
By adhering to these practices, you can ensure that your use of Spring JPA and Hibernate aligns with both development efficiency and production stability.
Expert Insights on Spring JPA Hibernate DDL Auto Configuration
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The `ddl-auto` setting in Spring JPA Hibernate is crucial for managing database schema evolution. It allows developers to automate schema generation, which can significantly speed up the development process. However, it is essential to choose the right strategy—whether `create`, `update`, or `validate`—to avoid unintended data loss or schema inconsistencies in production environments.”
Michael Thompson (Lead Database Engineer, Cloud Solutions Group). “Using `ddl-auto` can be a double-edged sword. While it simplifies the initial setup and testing phases, relying on it in production can lead to complications. It is advisable to use version control for database migrations instead of automatic schema updates to ensure data integrity and maintainability.”
Sarah Jenkins (Java Developer and Open Source Contributor). “In my experience, setting `ddl-auto` to `update` during development is a practical approach. It allows for quick iterations without the overhead of manual migrations. However, once the application is ready for production, transitioning to a more controlled migration strategy using tools like Flyway or Liquibase is imperative for robust database management.”
Frequently Asked Questions (FAQs)
What is the purpose of the `spring.jpa.hibernate.ddl-auto` property?
The `spring.jpa.hibernate.ddl-auto` property configures the behavior of Hibernate’s schema generation. It determines how the database schema should be created, updated, validated, or dropped based on the entity classes defined in the application.
What are the possible values for `spring.jpa.hibernate.ddl-auto`?
The possible values include `none`, `update`, `create`, `create-drop`, and `validate`. Each value dictates a different approach to schema management, such as no action, updating existing schemas, creating new schemas, dropping schemas on shutdown, or validating existing schemas against the entity definitions.
What happens when `ddl-auto` is set to `create`?
When set to `create`, Hibernate will drop the existing schema and create a new one based on the current entity definitions each time the application starts. This is useful for development but not recommended for production environments due to data loss.
Is it safe to use `update` in a production environment?
Using `update` in production can be relatively safe as it attempts to alter the existing schema without dropping data. However, it is essential to thoroughly test schema changes and have backups, as complex changes may still lead to unexpected issues.
What is the effect of setting `ddl-auto` to `validate`?
Setting `ddl-auto` to `validate` instructs Hibernate to validate the existing database schema against the entity mappings at startup. If discrepancies are found, an exception is thrown, ensuring that the application only runs with a compatible schema.
Can I change the `ddl-auto` setting without restarting the application?
No, changes to the `spring.jpa.hibernate.ddl-auto` setting typically require a restart of the application for the new configuration to take effect. This is because the schema generation process occurs during the application startup phase.
In the context of Spring JPA and Hibernate, the term “DDL auto” refers to the automatic generation and management of database schema based on the entity classes defined in the application. This feature is controlled by the `spring.jpa.hibernate.ddl-auto` property in the application configuration, which can take several values such as `create`, `update`, `validate`, and `none`. Each of these options dictates how Hibernate interacts with the database schema during application startup, allowing developers to streamline the database setup process in development and testing environments.
One of the primary benefits of using DDL auto is the convenience it offers in managing database schemas without requiring manual SQL scripts. This is particularly useful in agile development scenarios where changes to the data model are frequent. The `update` option is commonly used in development, as it allows for incremental changes to the schema without dropping existing data, thus facilitating a smoother development workflow. However, it is essential to understand the implications of each setting, especially in production environments where data integrity and schema stability are critical.
It is also important to consider the potential downsides of relying on DDL auto. While it simplifies development, it can lead to unexpected behaviors if not managed carefully. For instance, using the `create
Author Profile
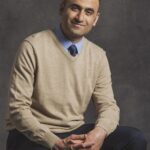
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?