How Can You Use SQL CASE in a WHERE Clause with an IN Statement?
When working with SQL, the power of the `CASE` statement can transform the way you query your data, allowing for dynamic decision-making within your queries. However, combining `CASE` with the `WHERE` clause and the `IN` statement can be a bit tricky. This combination opens up a world of possibilities for filtering data based on complex conditions, enabling you to write more efficient and expressive queries. Whether you’re a seasoned SQL developer or a newcomer eager to enhance your skills, understanding how to effectively utilize `CASE` within a `WHERE` clause can significantly elevate your database querying capabilities.
In SQL, the `CASE` statement acts as a conditional expression, allowing you to evaluate different conditions and return specific values based on the results. When integrated into a `WHERE` clause, it can help refine your query results by applying conditional logic to filter records. The `IN` statement, on the other hand, provides a straightforward way to check if a value exists within a specified list. By combining these two elements, you can create nuanced queries that adapt to various scenarios, making your data retrieval more precise and relevant.
As you delve deeper into this topic, you’ll discover practical examples and best practices for implementing `CASE` in conjunction with the `IN` statement. This exploration will
Using SQL CASE in WHERE Clause with IN Statement
The SQL `CASE` statement can be effectively utilized within a `WHERE` clause, especially in conjunction with the `IN` statement. This combination allows for dynamic filtering based on multiple conditions. The `CASE` statement evaluates conditions and returns specific values, making it powerful for conditional logic.
When using `CASE` within a `WHERE` clause, you can create complex queries that adjust the filtering criteria based on certain values. Here’s the general syntax for using `CASE` with `IN`:
“`sql
SELECT column1, column2
FROM table_name
WHERE column_name IN (
CASE
WHEN condition1 THEN value1
WHEN condition2 THEN value2
ELSE value_default
END
);
“`
This setup allows you to specify different sets of values based on conditions evaluated at runtime.
Example Scenario
Consider a scenario where you have a table named `employees` with the following structure:
employee_id | name | department | status |
---|---|---|---|
1 | John | Sales | Active |
2 | Jane | Marketing | Inactive |
3 | Bob | Sales | Active |
4 | Alice | HR | Active |
5 | Tom | IT | Inactive |
Suppose you want to select employees based on their status, but you want to change the criteria based on the department. For instance, for the `Sales` department, you want to include only `Active` employees, while for `IT`, you want to include both `Active` and `Inactive` employees.
The SQL query using `CASE` could look like this:
“`sql
SELECT employee_id, name
FROM employees
WHERE department = ‘Sales’ AND status IN (
CASE
WHEN department = ‘Sales’ THEN ‘Active’
WHEN department = ‘IT’ THEN ‘Active’
ELSE ‘Inactive’
END
);
“`
In this example, only employees from the `Sales` department who are `Active` will be returned.
Important Considerations
- Performance: Using `CASE` statements can lead to performance issues if not used judiciously, especially on large datasets.
- Readability: Complex `CASE` statements can make your SQL queries less readable. It’s important to maintain clarity.
- Compatibility: Ensure that your SQL database supports the use of `CASE` in the `WHERE` clause, as syntax and behavior may vary.
Alternative Approaches
In scenarios where readability and maintainability are priorities, consider using common table expressions (CTEs) or subqueries. These approaches can simplify complex logic and provide clearer structure.
For instance, you might rewrite the previous query using a CTE:
“`sql
WITH FilteredEmployees AS (
SELECT employee_id, name, department, status
FROM employees
WHERE (department = ‘Sales’ AND status = ‘Active’)
OR (department = ‘IT’ AND status IN (‘Active’, ‘Inactive’))
)
SELECT employee_id, name
FROM FilteredEmployees;
“`
This method enhances clarity by separating the logic into a distinct part of the query.
Using the `CASE` statement in the `WHERE` clause with `IN` offers powerful conditional filtering capabilities, but it requires careful implementation to ensure efficiency and clarity.
Using SQL CASE in WHERE Clause with IN Statement
In SQL, the `CASE` statement allows for conditional logic in queries. When combined with the `IN` statement, it can enhance the filtering capabilities of the `WHERE` clause. This approach is particularly useful when you want to apply different criteria based on certain conditions.
Basic Syntax
The syntax for using `CASE` within a `WHERE` clause alongside the `IN` statement is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE
(CASE
WHEN condition1 THEN value1
WHEN condition2 THEN value2
ELSE default_value
END) IN (list_of_values);
“`
Explanation of Components
- CASE Statement: Evaluates conditions and returns specific values.
- value1, value2: These represent the outcomes based on the evaluated conditions.
- default_value: This is returned if none of the conditions are met.
- list_of_values: This is a list of acceptable values for comparison.
Example Scenario
Consider a scenario where you have an `employees` table with columns `employee_id`, `department_id`, and `salary`. You want to filter employees based on their department and salary conditions.
“`sql
SELECT employee_id, department_id, salary
FROM employees
WHERE
(CASE
WHEN department_id = 1 THEN ‘High’
WHEN department_id = 2 THEN ‘Medium’
ELSE ‘Low’
END) IN (‘High’, ‘Medium’);
“`
Breakdown of the Example
- This query selects `employee_id`, `department_id`, and `salary`.
- The `CASE` statement checks the `department_id` and categorizes it as ‘High’, ‘Medium’, or ‘Low’.
- The `WHERE` clause filters results to include only those employees in ‘High’ or ‘Medium’ salary categories.
Multiple Conditions
You can also use multiple conditions within the `CASE` statement to refine your filtering. For instance, if you want to apply different salary ranges based on department:
“`sql
SELECT employee_id, department_id, salary
FROM employees
WHERE
(CASE
WHEN department_id = 1 AND salary > 70000 THEN ‘High’
WHEN department_id = 2 AND salary BETWEEN 50000 AND 70000 THEN ‘Medium’
ELSE ‘Low’
END) IN (‘High’, ‘Medium’);
“`
Key Points
- Flexibility: Using `CASE` allows for complex conditions.
- Clarity: This method can make SQL queries easier to read by consolidating logic into a single expression.
- Performance: While powerful, excessive use of `CASE` in a `WHERE` clause may impact query performance, especially on large datasets.
Considerations
When implementing `CASE` in a `WHERE` clause:
- Ensure that the `CASE` statement does not introduce ambiguity in your query.
- Be mindful of performance implications when using complex logic in large tables.
- Testing the query with sample data can help confirm expected behavior and outcomes.
This method of combining `CASE` with `IN` in SQL queries provides a robust way to conditionally filter data based on multiple criteria.
Expert Insights on Using SQL CASE in WHERE Clauses with IN Statements
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “Utilizing the SQL CASE statement within a WHERE clause alongside the IN statement can enhance query flexibility. It allows for conditional logic to determine which values should be included in the filtering process, making complex data retrieval more intuitive.”
Michael Chen (Senior Data Analyst, Insight Analytics). “Incorporating a CASE statement in a WHERE clause can streamline data analysis by enabling dynamic filtering based on varying conditions. This approach is particularly useful when dealing with datasets that require nuanced selection criteria, improving both performance and clarity.”
Lisa Patel (SQL Developer, DataTech Solutions). “The combination of CASE and IN in WHERE clauses is a powerful technique for conditional filtering. It allows developers to specify multiple conditions succinctly, which can significantly reduce the complexity of SQL queries while maintaining readability.”
Frequently Asked Questions (FAQs)
What is the purpose of using a CASE statement in a WHERE clause?
The CASE statement in a WHERE clause allows for conditional logic to filter records based on varying criteria, enabling more complex queries beyond simple comparisons.
Can I use a CASE statement with an IN clause in SQL?
Yes, you can use a CASE statement in conjunction with an IN clause, but you need to ensure that the CASE statement evaluates to a single value that can be checked against the list of values in the IN clause.
How does the syntax for a CASE statement in a WHERE clause look?
The syntax typically follows this structure: `WHERE column_name = CASE WHEN condition1 THEN value1 WHEN condition2 THEN value2 END`. Ensure the CASE statement returns a value that can be compared.
What is an example of using CASE in a WHERE clause with IN?
An example would be: `WHERE column_name IN (CASE WHEN condition THEN value1 ELSE value2 END)`. This allows filtering based on the evaluated condition’s result.
Are there performance considerations when using CASE in WHERE clauses?
Yes, using CASE statements can impact performance, especially in large datasets, as they may complicate query execution plans. It’s advisable to test performance on large tables.
Can I use multiple CASE statements in a single WHERE clause?
Yes, you can use multiple CASE statements within a single WHERE clause, but ensure that the logic remains clear and does not overly complicate the query.
The use of the SQL CASE statement within a WHERE clause, particularly in conjunction with the IN statement, is a powerful technique for enhancing query flexibility and control. This approach allows developers to create dynamic filtering conditions based on various criteria. By leveraging CASE, users can evaluate multiple conditions and return specific values that can be utilized in the IN clause, thus enabling more complex and nuanced queries that adapt based on the data being processed.
One of the key advantages of employing a CASE statement in this context is the ability to simplify complex logical expressions. Instead of writing multiple OR conditions, a CASE statement can condense the logic into a more readable format. This not only improves the maintainability of the SQL code but also enhances its performance by reducing the number of comparisons the database engine must perform.
Moreover, using CASE with IN can facilitate scenarios where the filtering criteria depend on the values of other columns or parameters. This dynamic capability allows for more sophisticated data retrieval strategies, making it easier to implement business rules directly within SQL queries. As a result, developers can ensure that their applications respond appropriately to varying data conditions without requiring excessive code or additional processing steps.
Author Profile
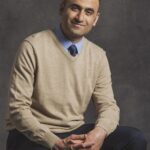
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?