How Do You Format Dates in SQL to DD MM YYYY?
When it comes to managing and manipulating data in SQL, understanding date formats is crucial for effective database operations. Among the various formats available, the `dd mm yyyy` style stands out for its clarity and ease of use, particularly in regions where this format is the standard. Whether you’re working with date inputs, querying data, or formatting outputs, mastering the `dd mm yyyy` format can enhance your SQL skills and streamline your data management processes.
In SQL, dates can be represented in multiple formats, which can sometimes lead to confusion, especially when dealing with international datasets. The `dd mm yyyy` format is not only user-friendly but also aligns with the way many users naturally interpret dates. This format can be particularly beneficial when it comes to ensuring that data is correctly entered and retrieved, thereby minimizing errors and improving the overall integrity of your database.
Understanding how to implement and manipulate the `dd mm yyyy` date format in SQL involves a few key concepts, including date functions, formatting options, and regional settings. By diving into these aspects, you can unlock the full potential of SQL’s date handling capabilities, making your queries more efficient and your data analysis more intuitive. As we explore this topic further, you’ll discover practical tips and techniques to confidently work with dates in your SQL projects.
SQL Date Format: Understanding dd mm yyyy
In SQL, handling date formats is crucial for effective data manipulation and retrieval. The `dd mm yyyy` format is commonly used in many regions, particularly in Europe and Asia, to represent dates. To achieve this format in SQL, various functions and methods can be employed depending on the database management system (DBMS) in use.
Formatting Dates in SQL Server
In SQL Server, the `FORMAT()` function can be utilized to convert dates into specific string formats. To display a date in the `dd mm yyyy` format, you can use the following syntax:
“`sql
SELECT FORMAT(GETDATE(), ‘dd MM yyyy’) AS FormattedDate;
“`
In this example, `GETDATE()` retrieves the current date and time, and `FORMAT()` converts it to the desired format.
For older versions of SQL Server, you might use `CONVERT()`:
“`sql
SELECT CONVERT(VARCHAR(10), GETDATE(), 105) AS FormattedDate;
“`
The `105` style code corresponds to the `dd-mm-yyyy` format.
Formatting Dates in MySQL
In MySQL, the `DATE_FORMAT()` function allows you to format date values easily. To convert a date to `dd mm yyyy`, you would use:
“`sql
SELECT DATE_FORMAT(NOW(), ‘%d %m %Y’) AS FormattedDate;
“`
In this function:
- `%d` represents the day of the month (01 to 31).
- `%m` represents the month (01 to 12).
- `%Y` represents the year in four digits.
Formatting Dates in PostgreSQL
PostgreSQL uses the `TO_CHAR()` function for date formatting. The syntax to format a date in `dd mm yyyy` is as follows:
“`sql
SELECT TO_CHAR(NOW(), ‘DD MM YYYY’) AS FormattedDate;
“`
This will yield a result in the required format directly from the current timestamp.
Common Use Cases for Date Formatting
When working with dates in SQL, it is important to format them appropriately for various applications:
- Data Presentation: Displaying dates in reports or user interfaces.
- Data Export: Preparing data for spreadsheets or external systems that require specific formats.
- Data Comparison: Ensuring consistency in date comparisons across different tables or databases.
DBMS | Function | Example |
---|---|---|
SQL Server | FORMAT() | FORMAT(GETDATE(), ‘dd MM yyyy’) |
MySQL | DATE_FORMAT() | DATE_FORMAT(NOW(), ‘%d %m %Y’) |
PostgreSQL | TO_CHAR() | TO_CHAR(NOW(), ‘DD MM YYYY’) |
Understanding how to format dates correctly in SQL is essential for effective database management. By utilizing the appropriate functions for each DBMS, you can ensure that your date representations meet the needs of your applications and users.
Understanding SQL Date Formats
SQL databases can vary in how they handle date formats, but the common format for representing dates is `YYYY-MM-DD`. However, when working with SQL, you may need to convert or display dates in the `DD MM YYYY` format for specific requirements or readability.
Converting Dates to DD MM YYYY Format
To convert dates into the `DD MM YYYY` format in SQL, you can use various functions depending on the SQL database being used. Below are examples for some of the most popular SQL databases:
MySQL
In MySQL, you can use the `DATE_FORMAT()` function:
“`sql
SELECT DATE_FORMAT(your_date_column, ‘%d %m %Y’) AS formatted_date
FROM your_table;
“`
This will return the date in the desired format.
SQL Server
In SQL Server, you can achieve the `DD MM YYYY` format using the `FORMAT()` function or `CONVERT()` function:
“`sql
SELECT FORMAT(your_date_column, ‘dd MM yyyy’) AS formatted_date
FROM your_table;
— Alternatively using CONVERT
SELECT CONVERT(VARCHAR, your_date_column, 105) AS formatted_date
FROM your_table;
“`
The `105` style in the `CONVERT()` function formats the date as `DD-MM-YYYY`, so you will need to replace the dashes with spaces afterward if necessary.
PostgreSQL
In PostgreSQL, you can use the `TO_CHAR()` function:
“`sql
SELECT TO_CHAR(your_date_column, ‘DD MM YYYY’) AS formatted_date
FROM your_table;
“`
This will produce the output in the desired format.
Practical Examples
Below are practical examples illustrating how to convert a date from a standard format to `DD MM YYYY`:
MySQL Example
“`sql
CREATE TABLE events (event_date DATE);
INSERT INTO events (event_date) VALUES (‘2023-10-05’);
SELECT DATE_FORMAT(event_date, ‘%d %m %Y’) AS formatted_date
FROM events;
“`
Output:
“`
formatted_date
—————
05 10 2023
“`
SQL Server Example
“`sql
CREATE TABLE events (event_date DATE);
INSERT INTO events (event_date) VALUES (‘2023-10-05’);
SELECT FORMAT(event_date, ‘dd MM yyyy’) AS formatted_date
FROM events;
“`
Output:
“`
formatted_date
—————
05 10 2023
“`
PostgreSQL Example
“`sql
CREATE TABLE events (event_date DATE);
INSERT INTO events (event_date) VALUES (‘2023-10-05’);
SELECT TO_CHAR(event_date, ‘DD MM YYYY’) AS formatted_date
FROM events;
“`
Output:
“`
formatted_date
—————
05 10 2023
“`
Considerations for Date Formatting
When working with date formats in SQL, consider the following:
- Locale Settings: Different regions may have different default date formats. Always check the locale settings of your database.
- Data Type Consistency: Ensure that the date column is of the correct data type (e.g., `DATE`, `DATETIME`) to prevent conversion errors.
- Performance: Frequent conversion of date formats in queries can affect performance. Store dates in the standard format when possible and only convert for display purposes.
By understanding how to format dates in SQL, you can effectively manage and present date information according to your specific needs.
Understanding SQL Date Formats: Expert Insights
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When working with SQL, understanding the date format is crucial for ensuring data integrity. The ‘dd mm yyyy’ format is not universally supported across all SQL databases, so it’s essential to verify the specific requirements of the system in use, whether it’s MySQL, SQL Server, or PostgreSQL.”
Michael Zhang (Senior Data Analyst, Data Insights Corp.). “In my experience, converting dates into the ‘dd mm yyyy’ format often involves using the appropriate functions like DATE_FORMAT in MySQL or FORMAT in SQL Server. This ensures that the data is both human-readable and compatible with various reporting tools.”
Lisa Thompson (SQL Trainer and Consultant, Code Academy). “Many developers overlook the importance of consistent date formatting in SQL queries. Adopting a standard format such as ‘dd mm yyyy’ can significantly reduce errors and improve the clarity of the data being processed, especially when collaborating with international teams.”
Frequently Asked Questions (FAQs)
What is the standard SQL date format?
The standard SQL date format is ‘YYYY-MM-DD’. This format is recognized by most SQL databases, ensuring consistency in date storage and retrieval.
How can I convert a date to the format DD-MM-YYYY in SQL?
To convert a date to the format DD-MM-YYYY, you can use the `FORMAT()` function in SQL Server or `DATE_FORMAT()` in MySQL. For example, in MySQL: `SELECT DATE_FORMAT(your_date_column, ‘%d-%m-%Y’)`.
Is it possible to store dates in DD-MM-YYYY format in SQL databases?
Yes, you can store dates in DD-MM-YYYY format as strings or text. However, it is recommended to store dates in the standard ‘YYYY-MM-DD’ format for better performance and compatibility.
What are the implications of using non-standard date formats in SQL?
Using non-standard date formats can lead to data inconsistencies, difficulties in date comparisons, and potential errors in date calculations. It is advisable to adhere to the standard format for optimal database operations.
How can I retrieve dates in DD-MM-YYYY format from a SQL database?
You can retrieve dates in DD-MM-YYYY format by using the appropriate date formatting function in your SQL dialect. For example, in PostgreSQL, you can use: `SELECT TO_CHAR(your_date_column, ‘DD-MM-YYYY’)`.
Are there any SQL databases that require a specific date format?
Most SQL databases support the standard ‘YYYY-MM-DD’ format, but some may have specific requirements or functions for date formatting. Always refer to the documentation of the specific SQL database you are using for details.
In SQL, the standard date format can vary depending on the database management system (DBMS) being used. However, many systems support the format of ‘dd mm yyyy’ for representing dates. Understanding how to manipulate and format dates is crucial for effective data management and retrieval. Different SQL dialects provide various functions to convert and format dates, which can be essential when dealing with user input or displaying dates in a user-friendly manner.
One of the key takeaways is that while the ‘dd mm yyyy’ format is widely recognized, it is not universally adopted across all SQL databases. For instance, SQL Server, MySQL, and PostgreSQL may require specific functions or settings to properly display or interpret dates in this format. It is important for users to familiarize themselves with the date handling capabilities of their specific SQL environment to ensure accurate data processing.
Additionally, when formatting dates, developers should consider localization and user preferences. Different regions may have varying conventions for date formats, which can lead to confusion if not handled properly. Implementing robust date formatting practices can enhance the usability of applications and improve data integrity, making it essential for developers to prioritize this aspect in their SQL queries and applications.
Author Profile
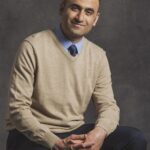
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?