How Can You Find Text Within a Stored Procedure in SQL?
In the world of database management, SQL (Structured Query Language) is a powerful tool that allows developers and data analysts to interact with relational databases. Among the myriad tasks that SQL can perform, one of the more nuanced challenges is searching for specific text within stored procedures. Stored procedures are essential for encapsulating complex logic and enhancing the efficiency of database operations, but as they grow in size and complexity, locating particular pieces of text or code can become a daunting task. Whether you’re troubleshooting, optimizing, or simply trying to understand existing code, knowing how to effectively search through stored procedures is an invaluable skill.
Finding text in stored procedures involves utilizing SQL’s querying capabilities to sift through the definitions of these procedures stored in the database. This process can be crucial for identifying specific functions, parameters, or even comments that might hold the key to understanding the logic behind a procedure. It not only aids in debugging but also enhances maintainability by allowing developers to ensure that changes are made thoughtfully and with a full understanding of the implications.
As we delve deeper into this topic, we will explore various methods and techniques for locating text within stored procedures, including the use of system catalog views and specialized queries. Understanding these methods will empower you to navigate your database with greater confidence and efficiency, ensuring that you can manage your
Using SQL to Find Text in Stored Procedures
To locate specific text within stored procedures in SQL Server, one can leverage system catalog views. These views store metadata about the database objects, including stored procedures. The `sys.sql_modules` view is particularly useful as it contains a column named `definition`, which holds the text of the stored procedures.
You can execute a query to search for a specific string within the stored procedure definitions. The following example demonstrates how to find stored procedures that contain a particular text string:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%your_search_text%’
“`
In this query, replace `your_search_text` with the desired string you want to find. The result will list all stored procedures where the specified text appears, along with the full text of each procedure.
Understanding Key SQL Components
When searching through stored procedures, it’s essential to understand some key components of the query:
- OBJECT_NAME(object_id): This function retrieves the name of the stored procedure based on its object ID.
- sys.sql_modules: This system view contains the definitions of SQL Server objects, making it ideal for text searches.
- LIKE Operator: This operator is utilized for pattern matching in SQL. The `%` wildcard represents any sequence of characters, allowing for flexible searches.
Example Query Output
The output of the above query might resemble the following table:
ProcedureName | Definition |
---|---|
usp_GetCustomerData | CREATE PROCEDURE usp_GetCustomerData AS BEGIN … WHERE customer_name LIKE ‘%search_text%’ |
usp_UpdateOrderStatus | CREATE PROCEDURE usp_UpdateOrderStatus AS BEGIN … SET status = ‘Processed’ WHERE order_id = @orderId |
This output provides clear visibility into which stored procedures contain the specified text and shows snippets of their definitions for context.
Additional Considerations
While the above method is effective, there are additional considerations to keep in mind:
- Permissions: Ensure you have the necessary permissions to access the metadata views.
- Performance: Searching through large databases with numerous stored procedures might affect performance. It’s advisable to limit searches to specific schemas if possible.
- Text Variations: Consider variations in text, such as different casing or spacing, as these may affect your search results.
By utilizing these techniques, database administrators and developers can efficiently locate and analyze stored procedures containing specific text, thereby enhancing their ability to manage and maintain SQL Server databases effectively.
Finding Text in Stored Procedures
To locate specific text within stored procedures in SQL Server, you can utilize several methods. Each method varies in complexity and efficiency, depending on the database management tool and environment you are using.
Using SQL Server Management Studio (SSMS)
In SQL Server Management Studio, you can search for text in stored procedures through the following steps:
- Open SSMS and connect to your database.
- Navigate to the Object Explorer and expand the database containing the stored procedures.
- Right-click on the **Stored Procedures** folder and select **Filter > Filter Settings**.
- In the filter settings, you can specify the Name or Description to find relevant stored procedures. However, this method only filters names and descriptions.
To search the content of the stored procedures:
- Press `Ctrl + Shift + F` to open the Find in Files dialog.
- Enter the text you want to find in the Find what box.
- Set the Look in option to the specific database or the entire solution.
- Click Find All.
Using T-SQL Queries
You can run a T-SQL query to search for text directly in the definitions of stored procedures. Here’s a common query to accomplish this:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
“`
Replace `YourSearchText` with the text you need to find. This query searches through the definitions of all stored procedures within the database.
Using the System Catalog Views
SQL Server also provides catalog views that can be queried for additional details. You can use `sys.sql_modules` along with `sys.procedures` for a more comprehensive search:
“`sql
SELECT
p.name AS ProcedureName,
m.definition
FROM
sys.procedures AS p
INNER JOIN
sys.sql_modules AS m ON p.object_id = m.object_id
WHERE
m.definition LIKE ‘%YourSearchText%’
“`
This approach gives you the names of stored procedures along with their definitions that contain the specified text.
Considerations
- Permissions: Ensure you have the necessary permissions to access the stored procedure definitions.
- Performance: Searching through large numbers of stored procedures can be resource-intensive. It may impact performance, especially in production environments.
- Text Encoding: Be mindful of text encoding issues that may affect how the text is stored and searched.
Alternative Tools
Several third-party tools and extensions can assist in searching through stored procedures, providing more advanced features:
- Redgate SQL Search: A free add-in for SSMS that allows for fast searches across various SQL objects, including stored procedures.
- ApexSQL Search: Another tool that provides comprehensive search capabilities within SQL Server databases.
Utilizing these methods and tools can streamline the process of locating specific text within stored procedures, enhancing your database management efficiency.
Expert Insights on Finding Text in SQL Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “To efficiently find text within stored procedures, one can leverage system views such as sys.sql_modules or sys.procedures. Utilizing the OBJECT_DEFINITION function allows for a targeted search within the procedure’s definition, making it easier to pinpoint specific keywords or phrases.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “Employing the INFORMATION_SCHEMA.ROUTINES view is a practical approach for searching through stored procedures. By filtering on the ROUTINE_DEFINITION column, developers can quickly locate the text they need, ensuring that their code remains maintainable and efficient.”
Linda Nguyen (SQL Performance Analyst, OptimizeDB). “In addition to using built-in views, I recommend creating a custom script that utilizes regular expressions for more complex searches. This method can enhance the search capabilities, allowing for more nuanced text retrieval within stored procedures.”
Frequently Asked Questions (FAQs)
How can I search for a specific text within a stored procedure in SQL?
You can use the `INFORMATION_SCHEMA.ROUTINES` view to search for specific text within stored procedures. Execute a query like:
“`sql
SELECT ROUTINE_NAME, ROUTINE_DEFINITION
FROM INFORMATION_SCHEMA.ROUTINES
WHERE ROUTINE_DEFINITION LIKE ‘%your_text_here%’
AND ROUTINE_TYPE=’PROCEDURE’;
“`
Is there a way to find all stored procedures that contain a specific keyword?
Yes, you can utilize the `sys.sql_modules` system view in SQL Server. The following query can be used:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%your_keyword%’;
“`
Can I use regular expressions to find text in stored procedures?
SQL Server does not support regular expressions natively for searching text in stored procedures. However, you can use `LIKE` or `CHARINDEX` functions for pattern matching.
What permissions are required to search for text in stored procedures?
You need at least `VIEW DEFINITION` permission on the stored procedures you are querying. This permission allows you to see the definition of the procedures.
Are there any tools available to help find text in stored procedures?
Yes, various database management tools like SQL Server Management Studio (SSMS), Redgate SQL Search, and ApexSQL Search provide functionality to search for text within stored procedures efficiently.
Can I automate the process of finding text in stored procedures?
Yes, you can create a script or stored procedure that encapsulates the search logic, allowing you to automate the process. This can be scheduled to run periodically or triggered based on specific events.
Finding specific text within stored procedures in SQL is a common task for database administrators and developers. This process typically involves querying the system catalog or information schema to locate the definitions of stored procedures that contain the desired text. Different database management systems (DBMS) provide various methods to achieve this, including using system views or built-in functions. For example, in SQL Server, the `sys.sql_modules` view can be utilized to search for text within the definitions of stored procedures.
Utilizing SQL queries to search for text can significantly enhance the efficiency of code maintenance and debugging. By leveraging the appropriate system views or functions, users can quickly identify stored procedures that require updates or modifications. This capability is particularly beneficial in large databases where manual searching would be time-consuming and prone to error. Moreover, understanding how to effectively search through stored procedures can lead to improved code quality and better database performance.
mastering the techniques for finding text within stored procedures is essential for effective database management. By employing the right queries and tools, users can streamline their workflows, enhance productivity, and maintain high standards of code integrity. As databases continue to grow in complexity, these skills will remain invaluable for anyone working in the field of database development and administration.
Author Profile
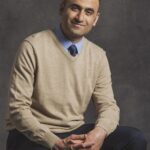
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?