How Can I Perform an SQL Lookup for a Key Value in a Specific Column?
In the world of data management, SQL (Structured Query Language) stands as a powerful tool for interacting with databases. Whether you’re a seasoned developer or a newcomer to the field, understanding how to efficiently look up key values within specific columns can significantly enhance your data retrieval capabilities. This skill not only streamlines your queries but also optimizes performance, enabling you to extract meaningful insights from vast datasets with ease.
At its core, the concept of looking up key values in SQL revolves around the ability to search for specific entries within a table based on defined criteria. This process is essential for tasks ranging from data analysis to application development, as it allows users to pinpoint the information they need without sifting through irrelevant data. By leveraging SQL functions and commands, you can perform lookups that are both precise and efficient, ensuring that your applications run smoothly and your analyses yield accurate results.
As we delve deeper into this topic, we will explore various techniques and best practices for executing key-value lookups in SQL. From simple queries to more complex joins and subqueries, you’ll discover how to harness the full potential of SQL to enhance your data manipulation skills. Whether you’re looking to improve your querying efficiency or simply want to expand your SQL knowledge, this guide will provide you with the insights you need to master
Understanding SQL Lookup Functions
SQL lookup functions are essential for retrieving specific values from a database based on key identifiers. These functions allow you to fetch data efficiently by matching a key value with corresponding data in a column. The most common SQL commands used for lookups include `JOIN`, `SUBQUERY`, and aggregate functions like `MAX`, `MIN`, or `GROUP BY`.
When using SQL for lookups, consider the following points:
- Key Columns: Ensure the columns used for lookups are indexed to improve performance.
- Data Types: Match the data types of the key value and column to avoid mismatches.
- NULL Values: Be aware of how NULL values are handled in your lookups, as they can affect results.
Using JOIN for Lookups
The `JOIN` clause is frequently used to combine rows from two or more tables based on a related column. This method is particularly useful when you need to retrieve related data from multiple tables.
Example syntax of a basic JOIN operation:
“`sql
SELECT a.key_column, b.value_column
FROM table_a a
JOIN table_b b ON a.key_column = b.key_column;
“`
In this example:
- `table_a` contains the primary data set.
- `table_b` contains the lookup data.
- `key_column` is the common identifier between the two tables.
Using Subqueries for Lookups
Subqueries can also be used to perform lookups by nesting a SELECT statement within another statement. This is beneficial when the lookup is conditional or requires aggregating data.
Example syntax of a subquery:
“`sql
SELECT key_column,
(SELECT value_column FROM table_b WHERE table_b.key_column = table_a.key_column) AS lookup_value
FROM table_a;
“`
This retrieves the `lookup_value` from `table_b` for each row in `table_a`.
Example Table Structure
To illustrate the concept of lookups, consider the following example of two tables:
table_a (Products) | table_b (Categories) |
---|---|
product_id | category_id |
1 | 101 |
2 | 102 |
3 | 101 |
Categories | |
---|---|
category_id | category_name |
101 | Electronics |
102 | Books |
To perform a lookup to find the category name for each product, you could use:
“`sql
SELECT p.product_id, c.category_name
FROM table_a p
JOIN table_b c ON p.category_id = c.category_id;
“`
This query will return the product ID along with its corresponding category name, effectively utilizing the lookup functionality within SQL.
Best Practices for SQL Lookups
To ensure efficient data retrieval during lookups, adhere to the following best practices:
- Indexing: Create indexes on columns frequently used in lookups to enhance performance.
- Avoid SELECT *: Specify only the columns needed in your queries to minimize data transfer.
- Consider Query Complexity: Simplify queries where possible to avoid performance bottlenecks.
By applying these techniques and principles, you can effectively perform lookups in SQL, leading to more efficient data handling and analysis.
Understanding SQL Lookup Functions
In SQL, lookup functions can be used to retrieve values from one table based on the matching values in another table. This is typically achieved through JOIN operations or subqueries. The primary objective is to enhance data retrieval efficiency and to maintain data integrity across related tables.
Using JOINs for Lookup Operations
JOIN statements allow for the combination of rows from two or more tables based on a related column. The most common types of JOINs are:
- INNER JOIN: Returns records that have matching values in both tables.
- LEFT JOIN: Returns all records from the left table and the matched records from the right table. If there is no match, NULL values are returned for columns of the right table.
- RIGHT JOIN: Returns all records from the right table and the matched records from the left table. If there is no match, NULL values are returned for columns of the left table.
- FULL OUTER JOIN: Returns all records when there is a match in either left or right table records.
Example of INNER JOIN:
“`sql
SELECT a.id, a.name, b.value
FROM TableA a
INNER JOIN TableB b ON a.id = b.a_id;
“`
In this example, `TableA` and `TableB` are joined on the `id` column of `TableA` and the `a_id` column of `TableB`. Only records with matching IDs will be returned.
Utilizing Subqueries for Value Lookup
Subqueries, or nested queries, can also be employed to fetch values from a column based on conditions set in another query. This is particularly useful when needing to retrieve a single value or when working with complex filtering.
**Example of a Subquery:**
“`sql
SELECT name
FROM TableA
WHERE id IN (SELECT a_id FROM TableB WHERE value > 100);
“`
In this case, names from `TableA` are selected based on the IDs that meet the criteria defined in the subquery.
Using CASE for Conditional Lookup
The CASE statement can be used within a SELECT statement to perform conditional lookups directly in the query. It evaluates conditions and returns specific values based on those conditions.
**Example of CASE Statement:**
“`sql
SELECT id,
name,
CASE
WHEN value > 100 THEN ‘High’
WHEN value BETWEEN 50 AND 100 THEN ‘Medium’
ELSE ‘Low’
END AS value_category
FROM TableB;
“`
This query categorizes values from `TableB` into ‘High’, ‘Medium’, or ‘Low’ based on their numerical value.
Performance Considerations
When performing lookups in SQL, consider the following to optimize query performance:
- Indexes: Ensure that the columns used in JOINs and WHERE clauses are indexed to speed up retrieval times.
- Selectivity: Use WHERE clauses to filter results as early as possible to minimize the number of records processed.
- Database Design: Normalize tables appropriately to reduce redundancy while ensuring efficient data retrieval.
- Analyze Execution Plans: Use tools to analyze query execution plans for potential bottlenecks.
By understanding these methods and strategies, you can effectively perform lookups in SQL, enhancing both the performance and clarity of your database queries.
Expert Insights on SQL Lookup Key Value Techniques
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Utilizing SQL for key-value lookups is essential for efficient data retrieval. By leveraging indexing strategies, one can significantly reduce query execution time, particularly in large datasets. It is crucial to understand the underlying database architecture to optimize these lookups effectively.”
James Patel (Database Administrator, Cloud Solutions Group). “When performing key-value lookups in SQL, employing JOIN operations can enhance data integrity and retrieval accuracy. However, one must be cautious about performance implications, especially with multiple joins, as they can lead to increased query complexity and execution time.”
Lisa Tran (SQL Developer, Data Dynamics). “A well-structured database schema is vital for efficient key-value lookups. Normalization can help eliminate data redundancy, but denormalization may be beneficial in specific scenarios where read performance is prioritized. Understanding the trade-offs is key to effective database design.”
Frequently Asked Questions (FAQs)
What is an SQL lookup key?
An SQL lookup key is a unique identifier used to retrieve specific records from a database table. It typically corresponds to a primary key or a unique field within the table.
How can I perform a lookup for a key value in SQL?
To perform a lookup for a key value in SQL, you can use the `SELECT` statement combined with a `WHERE` clause. For example: `SELECT * FROM table_name WHERE key_column = ‘key_value’;`.
What is the difference between a primary key and a foreign key in SQL?
A primary key uniquely identifies each record in a table, ensuring no duplicate values. A foreign key, on the other hand, is a field in one table that links to the primary key of another table, establishing a relationship between the two.
Can I use multiple columns as a lookup key in SQL?
Yes, you can use multiple columns as a composite key for lookups. This is done by specifying multiple columns in the `WHERE` clause, such as: `SELECT * FROM table_name WHERE column1 = ‘value1’ AND column2 = ‘value2’;`.
What are some common functions to assist with lookups in SQL?
Common functions for lookups include `JOIN` for combining tables based on keys, `EXISTS` to check for the existence of a key, and `IN` for checking if a key value exists within a specified set of values.
How can I optimize SQL lookups for performance?
To optimize SQL lookups, ensure that appropriate indexes are created on the lookup columns, avoid using wildcard characters at the beginning of search strings, and limit the number of returned rows by using `LIMIT` or `TOP` clauses when applicable.
In the realm of SQL, performing a lookup for a key value within a specific column is a fundamental operation that allows users to retrieve relevant data efficiently. This process typically involves using SQL queries such as SELECT, JOIN, or subqueries to filter and extract information based on certain criteria. By understanding how to effectively implement these queries, users can enhance their data retrieval capabilities and streamline their database interactions.
Moreover, utilizing functions such as CASE or COALESCE can further refine the lookup process, enabling users to handle null values or provide conditional outputs. Additionally, indexing the columns involved in lookups can significantly improve query performance, especially in large datasets. It is crucial to consider the structure of the database and the relationships between tables to optimize these lookups effectively.
In summary, mastering SQL lookup techniques for key values in columns is essential for efficient data management. By leveraging appropriate SQL functions and optimizing database design, users can achieve more accurate and faster results. Continuous practice and exploration of advanced SQL features will further enhance one’s ability to perform complex queries and data analysis.
Author Profile
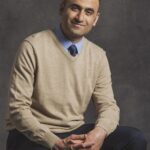
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?