How Can I Filter SQL Queries by Date Effectively?
In the world of data management, SQL (Structured Query Language) stands as a powerful tool for extracting, manipulating, and analyzing information stored in relational databases. One of the most common tasks that database professionals encounter is filtering data by date. Whether you’re analyzing sales trends, tracking user activity, or generating reports, the ability to efficiently query data based on specific time frames is essential. Understanding how to craft effective SQL queries that incorporate date filters can significantly enhance your data analysis capabilities and provide deeper insights into your datasets.
When working with SQL, filtering data by date involves leveraging various date functions and operators to narrow down results to a specific timeframe. This can include filtering for records that fall within a particular date range, identifying entries from a specific day, or even querying data from the past week or month. The flexibility of SQL allows users to tailor their queries to meet specific analytical needs, making it a crucial skill for anyone looking to harness the full potential of their database.
Moreover, the nuances of date filtering can vary depending on the database system in use, such as MySQL, PostgreSQL, or SQL Server. Each platform may have its own syntax and functions, which can affect how date queries are constructed. As we delve deeper into the intricacies of SQL date filtering
Filtering Dates in SQL Queries
Filtering by date in SQL is a common task that allows users to retrieve records from a database based on specific date criteria. This functionality is essential for generating reports, analyzing trends, or managing data over time. SQL provides various methods to filter records based on date fields, and understanding these methods can significantly enhance the effectiveness of your queries.
When filtering by date, you typically use the `WHERE` clause in conjunction with date functions and operators. The basic syntax for filtering by a date looks like this:
“`sql
SELECT column1, column2
FROM table_name
WHERE date_column = ‘YYYY-MM-DD’;
“`
However, filtering by date can be more complex, depending on your requirements. Here are some common techniques:
– **Filtering for a Specific Date**: Use the `=` operator to retrieve records that match a specific date.
– **Using Comparison Operators**: To find records within a range, utilize operators like `>`, `<`, `>=`, and `<=`. For example, to find records from a particular year, you can write:
```sql
SELECT *
FROM orders
WHERE order_date >= ‘2023-01-01’ AND order_date < '2024-01-01';
```
- **Using BETWEEN**: This operator simplifies range queries. For example:
```sql
SELECT *
FROM sales
WHERE sale_date BETWEEN '2023-01-01' AND '2023-12-31';
```
- **Filtering by Date Functions**: SQL also allows the use of date functions for more advanced filtering. For instance, to get records from the last 30 days, you can use:
```sql
SELECT *
FROM events
WHERE event_date >= CURDATE() – INTERVAL 30 DAY;
“`
Common Date Functions in SQL
Several SQL database systems provide built-in functions to manipulate date values. Here are a few widely used functions:
- CURDATE(): Returns the current date.
- NOW(): Returns the current date and time.
- DATEDIFF(date1, date2): Returns the difference in days between two dates.
- DATE_FORMAT(date, format): Formats the date according to the specified format.
The following table outlines examples of these functions:
Function | Description | Example |
---|---|---|
CURDATE() | Current date | SELECT CURDATE(); |
NOW() | Current date and time | SELECT NOW(); |
DATEDIFF() | Difference in days | SELECT DATEDIFF(‘2023-12-31’, ‘2023-01-01’); |
DATE_FORMAT() | Format a date | SELECT DATE_FORMAT(order_date, ‘%Y-%m-%d’); |
Timezone Considerations
When working with dates and times, especially in global applications, it’s crucial to account for timezones. Different SQL databases offer various ways to handle timezone data.
- Storing Timezone Information: Use `TIMESTAMP WITH TIME ZONE` to store timezone-aware timestamps.
- Converting Timezones: Use functions like `AT TIME ZONE` in SQL Server to convert between timezones.
Be mindful of how dates are stored in your database. Using UTC is a common practice for consistency, especially when applications are accessed from multiple regions.
By understanding these SQL date filtering techniques and considerations, you can effectively manage and analyze your data based on time-related criteria.
Filtering Data by Date in SQL
To filter data by date in SQL, you typically use the `WHERE` clause in conjunction with date functions and operators. Below are the common approaches for filtering records based on date criteria.
Basic Date Filtering
When filtering records by a specific date, you can use the `=` operator. For example:
“`sql
SELECT *
FROM orders
WHERE order_date = ‘2023-10-01’;
“`
This query retrieves all records from the `orders` table where the `order_date` matches October 1, 2023.
Filtering by Date Range
To select records within a specific date range, use the `BETWEEN` operator or a combination of `>=` and `<=` operators. Here’s how both can be applied: Using `BETWEEN`: ```sql SELECT * FROM orders WHERE order_date BETWEEN '2023-10-01' AND '2023-10-31'; ``` Using `>=` and `<=`: ```sql SELECT * FROM orders WHERE order_date >= ‘2023-10-01’ AND order_date <= '2023-10-31'; ``` Both queries will return all orders placed during October 2023.
Filtering with Date Functions
SQL provides various date functions that can enhance filtering capabilities. Here are some commonly used functions:
- YEAR(): Extracts the year from a date.
- MONTH(): Extracts the month from a date.
- DAY(): Extracts the day from a date.
Example using `YEAR()`:
“`sql
SELECT *
FROM orders
WHERE YEAR(order_date) = 2023;
“`
This retrieves all orders from the year 2023.
Using Current Date
You can filter records based on the current date using the `CURRENT_DATE` or `GETDATE()` function, depending on the SQL dialect. Here’s how to get records from the current month:
“`sql
SELECT *
FROM orders
WHERE order_date >= DATE_TRUNC(‘month’, CURRENT_DATE);
“`
This query will return all orders placed from the start of the current month to today.
Handling Time Components in Dates
If your date field includes time, it is essential to consider that in your filtering. For instance, if you want to filter records for a specific day regardless of the time, you can use the `::date` cast in PostgreSQL or `CAST` in SQL Server:
PostgreSQL example:
“`sql
SELECT *
FROM orders
WHERE order_date::date = ‘2023-10-01’;
“`
SQL Server example:
“`sql
SELECT *
FROM orders
WHERE CAST(order_date AS DATE) = ‘2023-10-01’;
“`
This ensures that you are only comparing the date part of the datetime field.
Example: Combining Multiple Conditions
You can combine date filters with other conditions using `AND` or `OR`. For example, to find orders from a specific customer within a date range:
“`sql
SELECT *
FROM orders
WHERE customer_id = 123
AND order_date BETWEEN ‘2023-10-01’ AND ‘2023-10-31’;
“`
This query retrieves orders for customer ID 123 made in October 2023.
By applying the methods outlined above, you can effectively filter data by date in your SQL queries. Understanding how to manipulate date data types and functions will enhance your ability to retrieve relevant information from your databases.
Expert Insights on Filtering SQL Queries by Date
Dr. Emily Carter (Data Analyst, Tech Insights Group). “When filtering SQL queries by date, it is crucial to use the correct date format that matches your database’s configuration. Utilizing functions like `DATE()` or `STR_TO_DATE()` can ensure accurate comparisons, especially when dealing with different time zones.”
Michael Chen (Database Administrator, Cloud Solutions Inc.). “Incorporating indexed date columns can significantly enhance the performance of SQL queries that filter by date. This optimization minimizes the search space and speeds up data retrieval, which is vital for applications requiring real-time analytics.”
Sarah Patel (SQL Developer, Data Dynamics). “It is essential to consider edge cases when filtering by date, such as inclusive versus exclusive boundaries. Using the `BETWEEN` operator can simplify this process, but always verify that your date ranges align with your business logic to avoid data discrepancies.”
Frequently Asked Questions (FAQs)
What is an SQL query filter by date?
An SQL query filter by date allows users to retrieve records from a database that match specific date criteria, using the WHERE clause to specify the desired date range or exact date.
How do I filter records by a specific date in SQL?
To filter records by a specific date, use the WHERE clause with the date column and the desired date in single quotes. For example: `SELECT * FROM table_name WHERE date_column = ‘YYYY-MM-DD’;`.
Can I filter by a date range in SQL?
Yes, you can filter by a date range using the BETWEEN operator. For example: `SELECT * FROM table_name WHERE date_column BETWEEN ‘start_date’ AND ‘end_date’;`.
What date formats are accepted in SQL queries?
SQL typically accepts date formats such as ‘YYYY-MM-DD’, ‘MM/DD/YYYY’, or ‘DD-MM-YYYY’, depending on the database system and its configuration.
How do I filter by the current date in SQL?
To filter by the current date, you can use the CURDATE() function in MySQL or GETDATE() in SQL Server. For example: `SELECT * FROM table_name WHERE date_column = CURDATE();`.
Is it possible to filter by date and time in SQL?
Yes, you can filter by both date and time by including the time component in your query. For example: `SELECT * FROM table_name WHERE datetime_column = ‘YYYY-MM-DD HH:MM:SS’;`.
In SQL, filtering data by date is a fundamental operation that allows users to retrieve specific records based on temporal criteria. This process typically involves using the `WHERE` clause in conjunction with date functions or operators to specify the desired date range. Commonly used date functions include `BETWEEN`, `>=`, and `<=`, which help define the start and end dates for the query. Additionally, SQL databases often support various date formats, so it is crucial to ensure that the date literals are correctly formatted to match the database's requirements.
Understanding how to effectively filter by date enhances data analysis capabilities and improves the accuracy of reports generated from the database. By applying date filters, users can isolate records from specific periods, such as daily, monthly, or yearly data, which is essential for trend analysis and performance evaluation. Furthermore, incorporating date filtering into SQL queries can significantly optimize query performance, as it reduces the amount of data processed and returned, leading to faster response times.
mastering date filtering in SQL queries is vital for anyone working with databases. It not only streamlines data retrieval processes but also empowers users to derive meaningful insights from their data. By leveraging the appropriate SQL syntax and functions, users can enhance their analytical capabilities and make
Author Profile
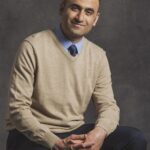
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?