How Can You Search for Text in Stored Procedures Using SQL?
In the realm of database management, stored procedures are invaluable tools that streamline complex operations and enhance performance. However, as systems evolve and applications grow, the need to maintain and audit these stored procedures becomes increasingly critical. One common challenge developers and database administrators face is searching for specific text or keywords within these procedures. Whether it’s for debugging, optimization, or ensuring compliance with coding standards, efficiently locating text in stored procedures can save time and prevent potential errors. In this article, we will explore effective strategies and techniques for conducting thorough searches within stored procedures, empowering you to manage your database with confidence.
Navigating the intricacies of stored procedures can be daunting, especially when dealing with large codebases. The ability to search for specific text not only aids in troubleshooting but also enhances the overall maintainability of your database architecture. Various SQL Server tools and built-in functions can facilitate this process, allowing users to pinpoint exact locations of interest within the code. Understanding how to leverage these tools effectively can transform the way you interact with your database, making it easier to implement changes and improvements.
Moreover, the search process is not just about finding text; it also involves understanding the context in which that text appears. This article will delve into methods for refining your search criteria, ensuring that you can quickly and accurately identify
Searching for Text in Stored Procedures
To effectively search for specific text within stored procedures in SQL Server, you can utilize a combination of system views and built-in functions. This method allows you to identify where certain keywords, phrases, or code patterns exist within your procedural code.
The primary system view that holds the definition of stored procedures is `sys.sql_modules`, which contains the SQL text for all objects. Additionally, the `sys.objects` view can provide context about the type of object you are dealing with.
Using SQL Queries to Search
To perform a search, you can execute a SQL query that joins these two views. Here’s a sample query that demonstrates how to find specific text, such as “YourSearchText”, in the definitions of stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — ‘P’ indicates a stored procedure
AND m.definition LIKE ‘%YourSearchText%’
“`
This query retrieves the names of stored procedures and their definitions where the specified text is found. You can replace “YourSearchText” with any string you are searching for.
Additional Considerations
When searching through stored procedures, keep the following points in mind:
- Case Sensitivity: Depending on the collation settings of your database, the search may be case-sensitive or case-insensitive.
- Performance: If your database contains a large number of stored procedures, this operation could take some time. Consider running it during off-peak hours.
- Regular Expressions: SQL Server does not natively support regular expressions. If you require more complex pattern matching, consider using a CLR function or processing the results in an external application.
Example Output
When executing the above query, you may receive results similar to the following table:
ProcedureName | ProcedureDefinition |
---|---|
usp_GetCustomerData | CREATE PROCEDURE usp_GetCustomerData AS … |
usp_UpdateOrderStatus | CREATE PROCEDURE usp_UpdateOrderStatus AS … |
This output clearly shows the stored procedures containing your search term, along with their definitions. Using this method will facilitate quick identification and review of stored procedures that may require updates or refactoring based on the text you are searching for.
Searching for Text in Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize the system catalog views. The `sys.sql_modules` view contains the definition of all SQL Server objects, including stored procedures, while the `sys.objects` view provides additional information about the objects.
Basic Query to Find Text
You can execute the following SQL query to search for a specific text string within the definitions of stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — ‘P’ stands for stored procedures
AND m.definition LIKE ‘%your_search_text%’
“`
Replace `your_search_text` with the text you want to search for. This query retrieves the names and definitions of all stored procedures containing the specified text.
Filtering by Schema
If you need to filter the results by schema, you can enhance your query as follows:
“`sql
SELECT
SCHEMA_NAME(o.schema_id) AS SchemaName,
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%your_search_text%’
AND SCHEMA_NAME(o.schema_id) = ‘your_schema_name’
“`
Replace `your_schema_name` with the appropriate schema to narrow your search.
Searching Across All Object Types
If you want to search not only in stored procedures but also in other object types such as functions, views, or triggers, you can modify the `WHERE` clause:
“`sql
SELECT
o.type_desc AS ObjectType,
o.name AS ObjectName,
m.definition AS ObjectDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
m.definition LIKE ‘%your_search_text%’
“`
This query retrieves the type, name, and definition of all objects containing the specified text.
Using Full-Text Search
For more complex searches, consider enabling full-text search on your SQL Server. This allows you to perform more advanced text searches. Here’s a basic setup:
- Create a Full-Text Catalog:
“`sql
CREATE FULLTEXT CATALOG ftCatalog AS DEFAULT;
“`
- Create a Full-Text Index on your stored procedure:
“`sql
CREATE FULLTEXT INDEX ON sys.sql_modules(definition)
KEY INDEX your_primary_key_index_name
WITH CHANGE_TRACKING AUTO;
“`
- Query Using Full-Text Search:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
CONTAINS(m.definition, ‘your_search_text’);
“`
This method offers more flexibility in search capabilities, allowing for phrase searches and proximity searches.
Performance Considerations
When searching through large numbers of stored procedures, consider the following:
- Indexing: Ensure that you have appropriate indexes on your objects to improve search performance.
- Execution Plan: Review the execution plan of your queries to identify potential bottlenecks.
- Search Scope: Limit the scope of your searches to specific schemas or object types when possible to enhance performance.
Utilizing these approaches will facilitate effective searching for text in stored procedures and other SQL objects within your database environment.
Expert Insights on SQL Text Search in Stored Procedures
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Searching for text within stored procedures can be quite challenging due to the nature of how SQL Server stores these objects. Utilizing the `sys.sql_modules` system view along with the `OBJECT_DEFINITION` function allows developers to extract the definition of stored procedures, making it easier to perform text searches effectively.”
Michael Thompson (Senior SQL Developer, Data Solutions Group). “To efficiently search for specific text in stored procedures, I recommend writing a custom script that leverages the `LIKE` operator in conjunction with the `sys.procedures` catalog view. This approach provides a straightforward way to filter procedures based on the presence of specific keywords.”
Lisa Patel (Data Analyst, Analytics Hub). “Incorporating full-text search capabilities can significantly enhance the process of searching for text in stored procedures. By enabling full-text indexing on the relevant database objects, users can perform more complex queries that yield faster and more accurate results.”
Frequently Asked Questions (FAQs)
How can I search for specific text within stored procedures in SQL Server?
You can use the `sys.sql_modules` system view along with the `OBJECT_DEFINITION` function to search for text within stored procedures. The query can be structured as follows:
“`sql
SELECT OBJECT_NAME(object_id)
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to search for text in stored procedures across multiple databases?
Yes, you can create a script that iterates through all databases and executes a similar query for each one. Utilizing dynamic SQL and `sp_msforeachdb` can facilitate this process.
What SQL command can I use to find stored procedures containing a specific keyword?
You can use the following SQL command:
“`sql
SELECT name
FROM sys.procedures
WHERE OBJECT_DEFINITION(object_id) LIKE ‘%keyword%’;
“`
Can I search for text in stored procedures in Oracle databases?
In Oracle, you can query the `USER_SOURCE` or `ALL_SOURCE` views to find specific text in stored procedures. For example:
“`sql
SELECT name
FROM user_source
WHERE UPPER(text) LIKE ‘%YOUR_SEARCH_TEXT%’;
“`
Are there any tools that can help search for text in stored procedures?
Yes, various SQL management tools such as SQL Server Management Studio (SSMS), Toad, and Oracle SQL Developer provide features to search for text within stored procedures, enhancing the search process.
What should I consider when searching for text in stored procedures?
Consider the potential performance impact of searching large definitions, the possibility of positives due to similar text in comments, and ensuring that you have appropriate permissions to access the definitions of stored procedures.
Searching for text within stored procedures in SQL is a crucial task for database administrators and developers. This process allows users to identify specific logic, business rules, or references within the stored procedures, which can be essential for debugging, optimizing performance, or understanding legacy code. Various methods can be employed to perform these searches, including querying system catalog views or using specialized tools and scripts designed for this purpose.
One effective method involves querying the `sys.sql_modules` system view, which contains the definitions of all SQL objects, including stored procedures. By filtering the results with a `LIKE` clause, users can locate stored procedures that contain specific keywords or phrases. Additionally, using the `OBJECT_DEFINITION` function can also aid in retrieving the text of a stored procedure for further analysis.
It is important to note that while manual searches can be effective, utilizing automated scripts or third-party tools can significantly enhance efficiency, especially in large databases with numerous stored procedures. Regularly auditing stored procedures for specific text can help maintain code quality and ensure compliance with organizational standards.
In summary, searching for text in stored procedures is a vital aspect of SQL database management. Employing the right techniques and tools can streamline the process, improve code maintainability,
Author Profile
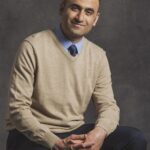
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?