How Can I Find Specific Text in a SQL Server Stored Procedure?
In the world of database management, SQL Server stands out as a powerful tool for organizing, retrieving, and manipulating data. However, as systems grow and evolve, maintaining clarity and efficiency in your stored procedures becomes increasingly challenging. One common issue developers face is the need to locate specific text or code snippets within these procedures. Whether you’re troubleshooting, optimizing performance, or just performing routine maintenance, knowing how to effectively search through stored procedures can save you time and frustration. In this article, we will explore various methods to find text within SQL Server stored procedures, empowering you to navigate your database with confidence and precision.
When working with SQL Server, stored procedures serve as essential building blocks for executing complex queries and operations. However, as these procedures accumulate over time, identifying specific pieces of code can become a daunting task. Developers often find themselves sifting through lengthy scripts, searching for particular keywords or logic. Understanding how to efficiently locate text within these stored procedures not only enhances productivity but also aids in ensuring code quality and consistency.
There are several techniques available for searching through stored procedures in SQL Server, ranging from built-in functions to more advanced querying methods. Each approach offers unique advantages, depending on the complexity of your database and the specific requirements of your search. By mastering these strategies,
Methods to Search for Text in Stored Procedures
To locate specific text within stored procedures in SQL Server, several methods can be employed. Each method has its advantages depending on the complexity of the search and the environment setup. The most common approaches include querying system tables, using built-in functions, and utilizing SQL Server Management Studio (SSMS) features.
Querying System Tables
SQL Server stores metadata about all its objects, including stored procedures, in system tables. You can execute a query on these tables to find specific text within the definition of stored procedures. Here’s a sample query:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%your_search_text%’;
“`
This query will return the names and definitions of all stored procedures that contain the specified text. Adjust `your_search_text` to the text you wish to find.
Using the OBJECT_DEFINITION Function
Another effective way to search for text in stored procedures is to utilize the `OBJECT_DEFINITION` function alongside a loop to iterate through all stored procedures. Here’s an example:
“`sql
DECLARE @procName NVARCHAR(255)
DECLARE @searchText NVARCHAR(255) = ‘your_search_text’
DECLARE proc_cursor CURSOR FOR
SELECT name
FROM sys.procedures;
OPEN proc_cursor
FETCH NEXT FROM proc_cursor INTO @procName
WHILE @@FETCH_STATUS = 0
BEGIN
IF OBJECT_DEFINITION(OBJECT_ID(@procName)) LIKE ‘%’ + @searchText + ‘%’
BEGIN
PRINT @procName
END
FETCH NEXT FROM proc_cursor INTO @procName
END
CLOSE proc_cursor
DEALLOCATE proc_cursor
“`
This script will print the names of all stored procedures containing the specified search text.
Utilizing SQL Server Management Studio
SQL Server Management Studio (SSMS) provides a user-friendly interface to search through stored procedures. You can use the “Find in Files” option:
- Open SSMS.
- Navigate to the “Edit” menu.
- Select “Find and Replace” and then “Find in Files”.
- In the “Find what” box, input your search text.
- Set the “Look in” option to the desired database or folder.
- Click “Find All”.
This method allows you to quickly see all occurrences of the specified text across all stored procedures, functions, and other SQL objects.
Performance Considerations
When searching for text in stored procedures, consider the following:
- Execution Time: The more procedures you have, the longer the search may take, especially if querying system tables.
- Indexing: Ensure that your database is properly indexed to improve search performance.
- Complex Queries: Avoid overly complex queries that may result in performance degradation.
Method | Pros | Cons |
---|---|---|
System Tables Query | Direct and customizable | Requires SQL knowledge |
OBJECT_DEFINITION Function | Dynamic and flexible | Can be slower for many procedures |
SSMS Find in Files | User-friendly interface | Less customizable |
By leveraging these methods, users can efficiently locate text within stored procedures, enhancing their ability to manage and maintain SQL Server databases effectively.
Finding Text in Stored Procedures
To locate specific text within stored procedures in SQL Server, you can utilize various methods. The following approaches will help you efficiently search and identify the stored procedures that contain the desired text.
Using SQL Server Management Studio (SSMS)
In SSMS, you can perform a search across all stored procedures by using the “Find” feature.
- Open SSMS.
- Connect to your database.
- In the Object Explorer, right-click on the database and select “Find.”
- Enter the text you want to search for in the “Find what” box.
- Choose “Entire Solution” to search through all objects, including stored procedures.
- Click “Find All.”
This method provides a straightforward way to see all occurrences of the text within the stored procedures.
Querying sys.sql_modules
You can also run a SQL query against the `sys.sql_modules` system view to find the text in stored procedures.
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%YourSearchText%’
AND OBJECTPROPERTY(object_id, ‘IsProcedure’) = 1;
“`
- Replace `YourSearchText` with the text you wish to find.
- This query will return the names of stored procedures that contain the specified text along with the procedure definitions.
Using INFORMATION_SCHEMA.ROUTINES
Another option is to query the `INFORMATION_SCHEMA.ROUTINES` view, which provides information about stored procedures.
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
AND ROUTINE_TYPE = ‘PROCEDURE’;
“`
- This method is similar to the previous one but uses a different system view.
- It can also be used to check for functions by modifying the `ROUTINE_TYPE` filter.
Considerations for Searching
When searching for text within stored procedures, consider the following:
- Case Sensitivity: The search can be case-sensitive depending on the collation settings of your SQL Server.
- Performance: Searching large databases may take time. Use specific keywords to narrow down results.
- Text Length: The maximum length for `ROUTINE_DEFINITION` may truncate longer procedures, leading to incomplete results.
Using Third-Party Tools
Several third-party tools can enhance your ability to search through SQL Server objects more effectively:
Tool Name | Description |
---|---|
Redgate SQL Search | A powerful tool that integrates with SSMS for advanced search capabilities. |
ApexSQL Search | Allows searching for SQL objects, including stored procedures, with advanced filtering. |
dbForge Studio | Provides a comprehensive search feature for various SQL Server objects. |
Utilizing these tools can streamline the search process and offer additional functionality, such as syntax highlighting and context-sensitive search.
Finding text within stored procedures in SQL Server can be accomplished through SSMS, SQL queries, and third-party tools. By utilizing these methods, you can efficiently identify and analyze the stored procedures that contain specific text.
Expert Insights on Locating Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “To efficiently find text within stored procedures in SQL Server, developers should utilize the system views such as sys.sql_modules and sys.objects. This allows for a comprehensive search across all procedures in the database, ensuring that no relevant code is overlooked.”
Mark Thompson (Senior SQL Developer, Data Insights Group). “Using the OBJECT_DEFINITION function can be particularly effective for retrieving the definition of a stored procedure, which can then be searched for specific text. This method is both straightforward and efficient for pinpointing code segments that require attention.”
Lisa Chen (SQL Server Consultant, Database Dynamics). “For those looking to automate the process, creating a script that queries the sys.sql_modules and incorporates a WHERE clause to filter by the desired text string can save significant time. This approach not only identifies the stored procedures but also enhances overall code maintenance.”
Frequently Asked Questions (FAQs)
How can I search for text within stored procedures in SQL Server?
You can search for text within stored procedures by querying the `sys.sql_modules` system view or the `sys.objects` view. For example, you can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to find all stored procedures containing a specific keyword?
Yes, you can use the same query mentioned above, replacing `your_search_text` with the specific keyword you want to find. This will return all stored procedures that include that keyword in their definition.
Can I use SQL Server Management Studio (SSMS) to find text in stored procedures?
Yes, in SSMS, you can use the “Find” feature (Ctrl + F) to search for text across your database objects, including stored procedures. Ensure you select the appropriate scope for your search.
What is the difference between searching in sys.sql_modules and sys.objects?
`sys.sql_modules` contains the definitions of SQL objects, including stored procedures, while `sys.objects` provides metadata about all objects in the database. Searching in `sys.sql_modules` is more effective for finding specific text within the definitions.
Are there any performance considerations when searching for text in stored procedures?
Yes, searching through large definitions can impact performance, especially in databases with many objects. It is advisable to limit your search scope and use indexed views or full-text search features for more extensive searches.
Can I automate the process of finding text in stored procedures?
Yes, you can create a SQL Server Agent job or a script that runs periodically to search for specific text in stored procedures and log the results. This can help in monitoring and maintaining your database codebase effectively.
In SQL Server, finding specific text within stored procedures is a common task that can be efficiently accomplished using system catalog views. The primary views used for this purpose are `sys.sql_modules` and `sys.objects`. By querying these views, users can retrieve the definition of stored procedures and search for particular keywords or phrases within their text. This approach allows database administrators and developers to maintain code quality and ensure compliance with coding standards.
Utilizing the `OBJECT_DEFINITION` function in conjunction with `sys.objects` can also enhance the search process. This method provides a straightforward way to access the text of stored procedures directly. Additionally, using the `LIKE` operator in SQL queries enables flexible pattern matching, making it easier to locate specific strings within the procedure definitions. This capability is particularly useful for large databases with numerous stored procedures.
Overall, leveraging SQL Server’s built-in catalog views and functions streamlines the process of searching for text within stored procedures. This not only aids in code maintenance and refactoring but also assists in identifying potential security vulnerabilities or areas for optimization. By employing these techniques, database professionals can ensure their stored procedures are well-documented and align with best practices.
Author Profile
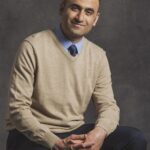
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?