How Can You Easily Get the Size of a SQL Server Database?
In the world of data management, understanding the size of your databases is crucial for optimizing performance, ensuring efficient storage, and planning for future growth. SQL Server, a robust relational database management system, provides a wealth of tools and techniques to help administrators assess the size of their databases effectively. Whether you’re a seasoned database administrator or a newcomer eager to learn, knowing how to retrieve and interpret database size information is an essential skill that can significantly impact your database management strategies.
When it comes to determining the size of a database in SQL Server, there are several methods available, each suited for different scenarios and levels of detail. From simple queries that provide a quick overview to more comprehensive reports that break down sizes by tables and indexes, SQL Server equips users with the necessary resources to gain insight into their database structures. Understanding these methods not only aids in capacity planning but also helps in identifying potential issues related to performance and storage.
As we delve deeper into the topic, we’ll explore various approaches to obtaining database size information, including built-in SQL Server functions, system views, and management tools. By the end of this article, you’ll be equipped with the knowledge to monitor and manage your database sizes effectively, ensuring that your SQL Server environment remains efficient and scalable. Whether you’re looking to
Methods to Retrieve Database Size in SQL Server
To determine the size of a database in SQL Server, several methods can be utilized, each providing varying levels of detail. The most common approaches include using system views, built-in stored procedures, and querying system functions.
Using System Views
One effective way to find the size of a database is by querying the `sys.master_files` system view. This view contains a row for each file of every database, which allows you to sum the sizes accordingly.
“`sql
SELECT
DB_NAME(database_id) AS DatabaseName,
SUM(size * 8 / 1024) AS SizeMB
FROM
sys.master_files
GROUP BY
database_id;
“`
This query will return the total size of each database in megabytes (MB). The multiplication by 8 converts the size from pages to kilobytes, and dividing by 1024 converts it to megabytes.
Using Built-in Stored Procedures
Another method to obtain the database size is through the use of the `sp_spaceused` stored procedure. This procedure returns the total size of the database, as well as the unallocated space.
To use it, first select the database:
“`sql
USE YourDatabaseName;
EXEC sp_spaceused;
“`
The output will provide details in the following format:
- Database size
- Unallocated space
- Reserved space
- Data space
- Index size
Querying System Functions
SQL Server also provides a function called `DBCC SHRINKFILE`, which can be used to retrieve information about the size of database files. While primarily used to shrink files, it can also return the current size of the specified file.
“`sql
DBCC SHRINKFILE (YourDatabaseFileName, 1);
“`
Note that this command is typically used for maintenance and should be executed with caution.
Size Overview Table
The following table summarizes the various methods discussed for retrieving database size information:
Method | Query/Command | Description |
---|---|---|
System Views |
SELECT DB_NAME(database_id) AS DatabaseName, SUM(size * 8 / 1024) AS SizeMB FROM sys.master_files GROUP BY database_id;
|
Summarizes the size of all files for each database. |
Stored Procedure |
EXEC sp_spaceused;
|
Returns detailed information about database size and space allocation. |
System Functions |
DBCC SHRINKFILE (YourDatabaseFileName, 1);
|
Retrieves the current size of database files. |
By leveraging these methods, database administrators can efficiently monitor and manage the size of their SQL Server databases.
Methods to Get Database Size in SQL Server
To determine the size of a database in SQL Server, there are several methods available. Each method can provide insights into the size of data files, log files, and overall database space usage.
Using SQL Server Management Studio (SSMS)
- Connect to the SQL Server instance.
- Navigate to the desired database.
- Right-click on the database name and select Properties.
- In the Database Properties window, click on the General page. The size of the database will be displayed in the Size section.
This method provides a graphical representation of the database size and allows for easy viewing of other properties.
Using T-SQL Commands
The following T-SQL queries can be executed to retrieve database size details:
- Get Database Size with sp_spaceused:
“`sql
USE [YourDatabaseName];
EXEC sp_spaceused;
“`
This command returns the total database size, unallocated space, and the number of rows in the database.
- Get Size of Data and Log Files:
“`sql
USE [YourDatabaseName];
SELECT
name AS FileName,
size * 8 / 1024 AS SizeMB,
max_size,
growth * 8 / 1024 AS GrowthMB
FROM sys.master_files
WHERE database_id = DB_ID(‘YourDatabaseName’);
“`
This query lists each data and log file, their sizes in megabytes, and their growth settings.
Using Dynamic Management Views (DMVs)
Dynamic Management Views can also be utilized to assess database size. The following query provides detailed size metrics:
“`sql
SELECT
DB_NAME(database_id) AS DatabaseName,
SUM(size) * 8 / 1024 AS SizeMB,
SUM(CAST(FILEPROPERTY(name, ‘SpaceUsed’) AS INT)) * 8 / 1024 AS UsedMB,
SUM(size) * 8 / 1024 – SUM(CAST(FILEPROPERTY(name, ‘SpaceUsed’) AS INT)) * 8 / 1024 AS FreeMB
FROM sys.master_files
GROUP BY database_id;
“`
This query outputs the database name, total size, used space, and free space in megabytes.
Understanding Database Size Metrics
When evaluating database size, it is essential to understand the different components:
- Data File Size: The size allocated for storing data.
- Log File Size: The size allocated for transaction logs.
- Used Space: The space currently utilized by data and logs.
- Free Space: The remaining space available in the allocated files.
Metric | Description |
---|---|
Total Size | Combined size of data and log files |
Used Space | Space actively used for data storage |
Free Space | Unused space available for future data growth |
By using these methods and understanding the metrics involved, database administrators can effectively monitor and manage SQL Server database sizes.
Expert Insights on Determining SQL Server Database Size
Dr. Emily Chen (Database Administrator, Tech Solutions Inc.). “To accurately retrieve the size of a SQL Server database, one can utilize the built-in stored procedure ‘sp_spaceused’. This procedure provides a comprehensive overview of the database size, including both data and log file sizes, which is crucial for effective database management and optimization.”
Mark Johnson (Senior Data Analyst, Data Insights Group). “Understanding the size of your SQL Server database is essential for capacity planning and performance tuning. Using the query ‘SELECT DB_NAME() AS DatabaseName, SUM(size) * 8 / 1024 AS SizeMB FROM sys.master_files WHERE database_id = DB_ID() GROUP BY DB_NAME()’ is an efficient way to get a quick overview of the database size in megabytes.”
Lisa Patel (SQL Server Consultant, OptimizeDB). “For ongoing monitoring of database size, I recommend implementing a scheduled job that runs the ‘sp_spaceused’ procedure periodically. This proactive approach not only helps in tracking growth trends but also aids in making informed decisions regarding resource allocation and scaling.”
Frequently Asked Questions (FAQs)
How can I check the size of a specific database in SQL Server?
You can check the size of a specific database by executing the following SQL query:
“`sql
SELECT
DB_NAME(database_id) AS DatabaseName,
SUM(size * 8 / 1024) AS SizeMB
FROM
sys.master_files
WHERE
database_id = DB_ID(‘YourDatabaseName’)
GROUP BY
database_id;
“`
Replace ‘YourDatabaseName’ with the name of your database.
What SQL command shows the total size of all databases in SQL Server?
You can use the following SQL command to retrieve the total size of all databases:
“`sql
EXEC sp_spaceused;
“`
This command provides information about the total database size and the space used.
Is there a way to get the size of each database along with their names?
Yes, you can use this SQL query to get the size of each database along with their names:
“`sql
SELECT
name AS DatabaseName,
CONVERT(VARCHAR(20), (size * 8 / 1024)) AS SizeMB
FROM
sys.master_files
GROUP BY
name;
“`
How can I find the size of the transaction log for a database?
To find the size of the transaction log for a specific database, use the following SQL query:
“`sql
SELECT
DB_NAME(database_id) AS DatabaseName,
SUM(size * 8 / 1024) AS LogSizeMB
FROM
sys.master_files
WHERE
type_desc = ‘LOG’
AND database_id = DB_ID(‘YourDatabaseName’)
GROUP BY
database_id;
“`
What tools can I use to monitor database size in SQL Server?
You can use SQL Server Management Studio (SSMS) for graphical monitoring, or third-party tools like Redgate SQL Monitor or SolarWinds Database Performance Analyzer for more advanced features.
Can I automate the process of checking database size in SQL Server?
Yes, you can automate the process by creating a SQL Server Agent job that runs a script to check database sizes at scheduled intervals and logs the results for review.
In SQL Server, determining the size of a database is a crucial task for database administrators and developers alike. Understanding the size of a database helps in resource management, performance tuning, and capacity planning. SQL Server provides multiple methods to retrieve this information, including system stored procedures, dynamic management views, and SQL queries that can be executed directly in SQL Server Management Studio (SSMS).
One of the most common methods to get the database size is by using the `sp_spaceused` stored procedure. This procedure provides a quick overview of the total size of the database, including both data and log files. Additionally, querying the `sys.master_files` system catalog view can yield detailed information about each file associated with the database, including their sizes and growth patterns. This allows for a more granular understanding of how storage is utilized across different files.
Another valuable approach is utilizing the `DBCC SHOWFILESTATS` command, which provides statistics about the files in the database. Furthermore, SQL Server Management Studio offers a graphical representation of database sizes, making it easier to visualize and compare the sizes of multiple databases. These various methods ensure that users can choose the most appropriate one based on their specific needs and preferences.
In summary,
Author Profile
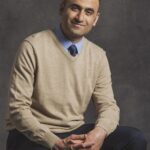
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?