How Can I Search for Specific Text Within SQL Server Stored Procedures?
In the realm of database management, SQL Server stands as a powerful tool for handling vast amounts of data. However, as applications evolve and grow, so do the complexities of the underlying code, particularly stored procedures. These essential components of SQL Server encapsulate business logic and data manipulation, making them crucial for efficient database operations. Yet, as developers and database administrators navigate through layers of stored procedures, the need to search for specific text or keywords becomes paramount. Whether you’re troubleshooting an issue, optimizing performance, or simply trying to understand the codebase better, knowing how to effectively search through stored procedures can save you time and enhance your productivity.
Searching for text within stored procedures in SQL Server is not merely a matter of convenience; it’s a vital skill for anyone working with databases. As stored procedures can often be lengthy and complex, pinpointing the exact location of a particular function or command can feel like searching for a needle in a haystack. Fortunately, SQL Server provides several methods and tools that enable users to efficiently locate text within these procedures, streamlining the process of code review and maintenance.
Understanding the various techniques for searching through stored procedures can empower developers and DBAs alike. From using built-in SQL Server functions to leveraging third-party tools, the ability to quickly find and analyze code
Searching for Text in Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize the system catalog views or the `INFORMATION_SCHEMA` views. These methods enable you to find occurrences of text strings, such as variable names, keywords, or comments, within your stored procedures.
One of the most common approaches is to query the `sys.sql_modules` view, which contains the definition of all SQL Server objects, including stored procedures. You can combine this with the `sys.objects` view to filter only stored procedures.
Here is a basic query that demonstrates how to search for a specific text string within stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — P indicates stored procedures
AND m.definition LIKE ‘%YourSearchText%’
“`
Replace `YourSearchText` with the actual text you want to search for. This query will return the names of the stored procedures and their definitions containing the specified text.
Using INFORMATION_SCHEMA Views
Another method involves using the `INFORMATION_SCHEMA.ROUTINES` view, which provides a standard way to access metadata about routines, including stored procedures. Here’s how to perform a similar search using this approach:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
“`
This query also returns the names and definitions of the procedures matching the search term.
Limitations and Considerations
When searching through stored procedures, it is essential to consider the following:
- Definition Length: The `ROUTINE_DEFINITION` column in `INFORMATION_SCHEMA.ROUTINES` has a maximum length of 4000 characters. For larger procedures, you might not get the full definition.
- Case Sensitivity: The search is case-sensitive or case-insensitive based on the collation settings of the database.
- Performance: Searching through a large number of stored procedures can be resource-intensive. It’s advisable to limit searches to specific schemas if possible.
Example Table of Search Results
To visualize the results of your search, consider the following example table structure:
Procedure Name | Procedure Definition Snippet |
---|---|
usp_GetOrders | SELECT * FROM Orders WHERE CustomerID = @CustomerID |
usp_UpdateStock | UPDATE Products SET Stock = Stock – @Quantity WHERE ProductID = @ProductID |
This table format provides a clear view of the stored procedures that contain the searched text, along with relevant snippets from their definitions.
By utilizing these queries and considerations, you can effectively search for text within stored procedures in SQL Server, aiding in code maintenance and analysis.
Searching for Text in Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize system catalog views or the built-in `sys.sql_modules` function. This enables you to identify procedures containing a particular keyword or phrase.
Using `sys.sql_modules`
The `sys.sql_modules` view contains information about the definitions of SQL Server objects, including stored procedures. You can query this view to find procedures that include a specific text fragment.
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%YourSearchText%’
“`
- OBJECT_NAME(object_id): Returns the name of the procedure.
- definition: Contains the SQL text of the procedure.
- LIKE ‘%YourSearchText%’: Use this to specify the text you are searching for. The percent signs (%) are wildcards that allow for any characters before or after the search text.
Using `INFORMATION_SCHEMA.ROUTINES`
Another option is to query the `INFORMATION_SCHEMA.ROUTINES` view. This view provides a more standardized approach and can include user-defined functions as well.
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
“`
- ROUTINE_NAME: The name of the stored procedure.
- ROUTINE_DEFINITION: The SQL text of the procedure.
- ROUTINE_TYPE: Filtering for ‘PROCEDURE’ ensures only stored procedures are considered.
Example Scenario
Consider a scenario where you want to find all stored procedures that reference the term “CustomerID”. You can execute the following SQL commands:
“`sql
SELECT
OBJECT_NAME(object_id) AS ProcedureName,
definition
FROM
sys.sql_modules
WHERE
definition LIKE ‘%CustomerID%’
“`
You may also use:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%CustomerID%’
“`
Both queries will return a list of stored procedures that reference “CustomerID”.
Considerations
When performing text searches in stored procedures, consider the following:
- Performance: Searching large databases can be resource-intensive. Limit your search scope if possible.
- Permissions: Ensure you have the necessary permissions to access the system views or the stored procedure definitions.
- Text Length: Be aware of the maximum length of the `ROUTINE_DEFINITION` column, which can affect results if stored procedures are very large.
Alternative Methods
If the built-in methods do not meet your needs, consider:
- Third-party tools: Various SQL Server management tools allow for enhanced searching capabilities.
- Scripting: Create scripts that iterate through stored procedures and output definitions to a text file for manual searching.
By applying these methods, you can effectively locate text within stored procedures in SQL Server, aiding in maintenance and debugging processes.
Expert Insights on Searching for Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “When searching for text within stored procedures in SQL Server, utilizing the system catalog views such as sys.sql_modules and sys.objects can be incredibly effective. By querying these views, you can locate specific text strings within the definitions of your stored procedures, which is crucial for maintaining and auditing your database.”
James Liu (Senior SQL Developer, Data Dynamics). “A common approach to find text in stored procedures is to use the OBJECT_DEFINITION function along with a LIKE clause. This method allows developers to filter through the definitions efficiently. It is essential to ensure that you are searching across all relevant schemas to avoid missing any instances of the text.”
Maria Gonzalez (Database Administrator, Cloud Data Solutions). “For large databases, performance can be an issue when searching for text in stored procedures. I recommend implementing a full-text search solution or utilizing SQL Server Management Studio’s built-in search capabilities. This can significantly speed up the process and provide more accurate results.”
Frequently Asked Questions (FAQs)
How can I search for specific text within stored procedures in SQL Server?
You can search for specific text in stored procedures by querying the `sys.sql_modules` system view or using the `INFORMATION_SCHEMA.ROUTINES` view. For example, you can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to search for text in all database objects, including stored procedures?
Yes, you can search for text across all database objects by querying the `sys.sql_modules` view along with `sys.objects`. Use a query like this:
“`sql
SELECT o.name, m.definition
FROM sys.objects o
JOIN sys.sql_modules m ON o.object_id = m.object_id
WHERE m.definition LIKE ‘%your_search_text%’;
“`
Can I search for text in stored procedures using SQL Server Management Studio (SSMS)?
Yes, you can use the “Find” feature in SSMS. Right-click on the database in the Object Explorer, select “Find,” and then choose “Find in Object Explorer.” Enter your search text and select “Stored Procedures” as the object type.
What permissions do I need to search for text in stored procedures?
You need at least `VIEW DEFINITION` permission on the stored procedures you wish to search. This permission allows you to view the metadata and definitions of the stored procedures.
Are there any third-party tools that can help with searching text in stored procedures?
Yes, several third-party tools, such as Redgate SQL Search and ApexSQL Search, provide enhanced functionality for searching text within stored procedures and other database objects in SQL Server.
Can I automate the process of searching for text in stored procedures?
Yes, you can automate the process by creating a SQL Server Agent job that runs a script to search for text in stored procedures at scheduled intervals. You can also use PowerShell scripts to perform this task programmatically.
Searching for text within stored procedures in SQL Server is a crucial task for database administrators and developers. It allows users to identify specific code segments, understand dependencies, and maintain code quality. SQL Server provides various methods to perform these searches, including system views, built-in functions, and third-party tools. Utilizing these methods effectively can enhance code management and streamline troubleshooting processes.
One of the most common techniques involves querying the `sys.sql_modules` system view, which contains the definition of all SQL Server objects, including stored procedures. By using the `OBJECT_DEFINITION` function or the `LIKE` operator, users can filter the results to find procedures that contain specific keywords or phrases. This approach is efficient and leverages SQL Server’s built-in capabilities, making it accessible for users with varying levels of expertise.
Additionally, leveraging tools such as SQL Server Management Studio (SSMS) can simplify the search process. SSMS offers a user-friendly interface for searching through stored procedures, enabling users to quickly locate relevant code snippets. Furthermore, employing third-party tools can provide advanced search functionalities, such as regular expression support and comprehensive reporting features, which can significantly enhance productivity.
effectively searching for text in stored procedures within SQL Server
Author Profile
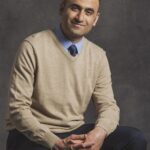
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?