How Can I Search for Text within Stored Procedures in SQL Server?
In the realm of database management, the ability to efficiently search for and retrieve information is paramount. SQL Server, a robust relational database management system, offers a variety of tools and techniques to streamline this process. Among these, the use of stored procedures stands out as a powerful method for encapsulating complex queries and operations. But what happens when you need to search through the text of these stored procedures? This article delves into the intricacies of searching stored procedure text in SQL Server, equipping you with the knowledge to enhance your database querying capabilities.
Understanding how to effectively search for stored procedure text can significantly improve your database management tasks. Whether you’re troubleshooting issues, performing audits, or simply trying to locate specific functionality within your database, knowing how to navigate and query stored procedures is essential. SQL Server provides several methods to access this information, allowing users to filter and retrieve relevant data quickly and efficiently.
As we explore the mechanisms available for searching stored procedure text, we will touch upon various system views and functions that SQL Server offers. This knowledge not only empowers database administrators and developers to maintain their systems more effectively but also enhances overall productivity by reducing the time spent on locating and analyzing stored procedures. Join us as we unravel the strategies and best practices for mastering stored procedure text searches in SQL Server.
Understanding the Need for Text Search in SQL Server
In many applications, the ability to search for specific text within stored procedures is crucial for maintenance and development. Developers often need to locate procedures that contain particular keywords, comments, or logic that may require updates or reviews. SQL Server provides several methods to facilitate text searches within stored procedures.
Using System Catalog Views
SQL Server includes system catalog views that can be queried to find stored procedures containing specific text. The most commonly used views for this purpose are `sys.sql_modules` and `sys.objects`. The `sys.sql_modules` view contains the definitions of SQL objects, while `sys.objects` contains metadata about those objects.
To search for a specific text in stored procedure definitions, you can use the following query:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — ‘P’ stands for stored procedures
AND m.definition LIKE ‘%your_search_text%’;
“`
This query returns the names and definitions of stored procedures that include the specified text.
Creating a Stored Procedure for Text Search
To streamline the process of searching for text within stored procedures, you can create a stored procedure that encapsulates the search logic. Below is an example of such a stored procedure:
“`sql
CREATE PROCEDURE SearchStoredProcedures
@SearchText NVARCHAR(100)
AS
BEGIN
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%’ + @SearchText + ‘%’;
END
“`
To execute this stored procedure, simply call it with the desired search text:
“`sql
EXEC SearchStoredProcedures ‘your_search_text’;
“`
Key Considerations
When implementing a text search functionality in SQL Server, consider the following aspects:
- Performance: Text searches can be resource-intensive, especially in databases with numerous stored procedures. Indexing and optimizing queries can help mitigate performance issues.
- Security: Ensure that access to metadata is controlled, as revealing stored procedure definitions could expose sensitive logic.
- Case Sensitivity: SQL Server’s text search is case-insensitive by default, but this behavior can vary based on the collation settings of your database.
Example Results Table
The results from the stored procedure search can be presented in a structured format. Below is an example of how the output may look:
Procedure Name | Procedure Definition |
---|---|
usp_GetEmployeeDetails | CREATE PROCEDURE usp_GetEmployeeDetails AS … |
usp_UpdateEmployeeSalary | CREATE PROCEDURE usp_UpdateEmployeeSalary AS … |
By utilizing these methods, developers can efficiently search and manage stored procedures in SQL Server, ensuring that code remains maintainable and up-to-date.
Understanding SQL Server Stored Procedures
Stored procedures in SQL Server are precompiled collections of SQL statements and optional control-of-flow statements that are stored in the database. They can be executed as a single unit, which improves performance and security.
Key features of stored procedures include:
- Reusability: Once created, stored procedures can be executed multiple times without needing to rewrite the SQL code.
- Security: Permissions can be granted to execute a stored procedure without exposing the underlying table structures.
- Performance: Stored procedures are precompiled, which can lead to improved execution speed.
Searching for Text Within Stored Procedures
To search for text within stored procedures in SQL Server, you can query the system catalog views. A common approach is to use the `sys.sql_modules` view in combination with `sys.objects`.
Here’s a sample query to find stored procedures containing specific text:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
INNER JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — P indicates stored procedures
AND m.definition LIKE ‘%search_text%’ — Replace with your search text
ORDER BY
o.name;
“`
This SQL query retrieves the names and definitions of stored procedures that contain the specified text.
Using Full-Text Search on Stored Procedure Definitions
For more advanced searches, especially when dealing with large databases, consider using SQL Server’s Full-Text Search feature. This allows for more sophisticated querying capabilities.
To set up Full-Text Search:
- Ensure that the Full-Text Search feature is installed on your SQL Server instance.
- Create a full-text catalog:
“`sql
CREATE FULLTEXT CATALOG YourCatalogName AS DEFAULT;
“`
- Create a full-text index on the stored procedure definitions:
“`sql
CREATE FULLTEXT INDEX ON sys.sql_modules(definition)
KEY INDEX YourUniqueIndexName
ON YourCatalogName
WITH CHANGE_TRACKING AUTO;
“`
Once configured, you can run queries using the `FREETEXT` or `CONTAINS` functions to search for specific terms or phrases.
Considerations When Searching
When searching through stored procedures, keep the following in mind:
- Performance: Frequent searches across large databases can impact performance; consider indexing strategies.
- Permissions: Ensure that the user executing the search has appropriate permissions to access the system catalog views.
- Text Variability: Be aware of potential variations in text (e.g., comments, whitespace) that may affect search results.
Common Use Cases for Searching Stored Procedures
Searching for text in stored procedures can be useful in various scenarios:
- Code Maintenance: Identify dependencies or references to specific tables or columns when refactoring code.
- Security Audits: Find stored procedures that may expose sensitive data or have security implications.
- Documentation: Assist in generating documentation by identifying all procedures related to specific functionalities.
By leveraging the above techniques, you can effectively search and manage SQL Server stored procedures to maintain code quality and security.
Expert Insights on SQL Server Search Stored Procedure Text
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “When implementing a search stored procedure in SQL Server, it is crucial to optimize the indexing strategy to enhance performance. Proper indexing not only speeds up search queries but also reduces the load on the server, ensuring that the application remains responsive.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “Utilizing full-text search capabilities in SQL Server can significantly improve the efficiency of stored procedures designed for text searches. By leveraging these features, developers can execute complex queries that return relevant results faster than traditional methods.”
Sarah Thompson (Data Analyst, Analytics Experts LLC). “It is essential to monitor the performance of stored procedures that handle text searches in SQL Server. Regularly reviewing execution plans and query statistics allows for timely optimizations, ensuring that the stored procedures continue to meet performance expectations as data grows.”
Frequently Asked Questions (FAQs)
How can I search for stored procedure text in SQL Server?
You can search for stored procedure text in SQL Server by querying the `sys.sql_modules` or `sys.procedures` system catalog views. For example, you can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%search_term%’;
“`
Is there a way to find all stored procedures containing a specific keyword?
Yes, you can find all stored procedures containing a specific keyword by using the `LIKE` operator in your query. For instance:
“`sql
SELECT name
FROM sys.procedures
WHERE OBJECT_DEFINITION(object_id) LIKE ‘%keyword%’;
“`
Can I search for stored procedures in multiple databases at once?
You cannot directly search across multiple databases with a single query. However, you can write a script that iterates through each database and executes the search query within each context.
What permissions are required to search for stored procedure text?
To search for stored procedure text, you need at least `VIEW DEFINITION` permission on the database where the stored procedures reside. Without this permission, you will not be able to access the definitions.
Are there any tools available to assist in searching stored procedure text?
Yes, several third-party tools and SQL Server Management Studio (SSMS) features can assist in searching stored procedure text. SSMS has a built-in “Object Explorer” that allows you to view and search through stored procedures easily.
How can I search for stored procedures based on their parameters?
To search for stored procedures based on their parameters, you can query the `sys.parameters` catalog view. For example:
“`sql
SELECT p.object_id, p.name
FROM sys.parameters p
JOIN sys.procedures pr ON p.object_id = pr.object_id
WHERE p.name LIKE ‘%parameter_name%’;
“`
In SQL Server, searching for stored procedure text is a crucial task for database administrators and developers who need to manage and optimize their database systems effectively. Stored procedures are essential for encapsulating business logic and database operations, and being able to search through their definitions can significantly aid in understanding and maintaining the database schema. SQL Server provides various methods to locate stored procedure text, including querying system catalog views such as `sys.sql_modules` and `sys.objects`, utilizing the `OBJECT_DEFINITION` function, and employing full-text search capabilities for more complex queries.
One of the primary methods to search for specific text within stored procedures is to utilize the `LIKE` operator in conjunction with the `sys.sql_modules` view. This allows users to filter stored procedures based on specific keywords or phrases contained within their definitions. Additionally, understanding the structure of the system catalog views can enhance the efficiency of these searches, enabling users to obtain the necessary information with minimal overhead.
Moreover, leveraging tools such as SQL Server Management Studio (SSMS) can simplify the search process. SSMS provides built-in features that allow users to search for stored procedures by name or text, making it more user-friendly. Additionally, implementing best practices for naming conventions and documentation can streamline the
Author Profile
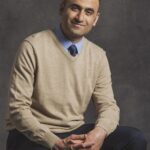
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?