How Can I Search for Text in All Stored Procedures in SQL Server?
In the world of database management, SQL Server stands as a powerful tool that enables organizations to store, retrieve, and manipulate data efficiently. As applications grow in complexity, so do the number of stored procedures that help streamline operations and encapsulate business logic. However, with this growth comes the challenge of maintaining clarity and organization within the database. One common issue developers face is the need to search for specific text or keywords within these stored procedures. Whether you’re troubleshooting an issue, optimizing performance, or simply trying to understand the existing code, knowing how to effectively search through your stored procedures can save you time and frustration.
Searching for text in all stored procedures in SQL Server is not just a matter of convenience; it’s an essential skill for any database administrator or developer. The ability to quickly locate references to a particular function, variable, or business rule can significantly enhance your workflow and improve collaboration among team members. While SQL Server Management Studio (SSMS) provides some tools for this purpose, understanding the underlying methods and queries can empower you to perform more efficient searches tailored to your specific needs.
In this article, we will explore various techniques for searching text within stored procedures in SQL Server. From leveraging built-in system views to crafting custom scripts, we will uncover the strategies that can help you navigate your database
Searching for Text in Stored Procedures
To search for specific text within all stored procedures in SQL Server, you can utilize the system views that store metadata about your database objects. The most common approach is to query the `sys.sql_modules` and `sys.objects` views.
The `sys.sql_modules` view contains the definition of all SQL Server objects, including stored procedures, while `sys.objects` provides additional information about each object, including its type. By joining these two views, you can effectively filter and find stored procedures containing the specified text.
SQL Query Example
Here is an example SQL query that searches for a specific text string within all stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules AS m
JOIN
sys.objects AS o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%YourSearchText%’
ORDER BY
o.name;
“`
In this query:
- Replace `YourSearchText` with the text you are searching for.
- The `o.type = ‘P’` condition ensures that only stored procedures are included in the results.
Understanding the Results
The query returns a result set with the following columns:
- ProcedureName: The name of the stored procedure.
- ProcedureDefinition: The actual SQL code of the stored procedure that contains the search text.
This can be useful for auditing or refactoring tasks where you need to locate specific functionalities within your stored procedures.
Alternative Methods
In addition to the SQL query approach, you can also use SQL Server Management Studio (SSMS) to perform a search:
- Open SSMS and connect to your database.
- Use the “Object Explorer” to navigate to the “Programmability” section under your database.
- Right-click on “Stored Procedures” and select “View Code” for individual procedures.
- Alternatively, you can use the “Find” feature (Ctrl + F) to search through the code of open scripts.
Considerations
When performing text searches in stored procedures, keep the following points in mind:
- Performance: Searching through a large number of stored procedures can impact performance, especially on large databases. It’s advisable to run such queries during off-peak hours.
- Exact Matches vs. Wildcards: Using the `LIKE` clause allows for flexible searches, but can return positives. Consider using more specific patterns if needed.
- Security: Ensure that you have the appropriate permissions to access the metadata of the stored procedures.
Example Result Set
Here’s an example of what the result set might look like when executing the search query:
ProcedureName | ProcedureDefinition |
---|---|
usp_GetEmployeeData | CREATE PROCEDURE usp_GetEmployeeData AS BEGIN … WHERE EmployeeID = @EmployeeID |
usp_UpdateEmployee | CREATE PROCEDURE usp_UpdateEmployee AS BEGIN … SET EmployeeName = @NewName |
This structured approach to searching stored procedures will assist developers and database administrators in maintaining and managing SQL Server databases efficiently.
Searching for Text in All Stored Procedures
In SQL Server, you can efficiently search for specific text within all stored procedures using system catalog views. The most commonly used views for this purpose are `sys.sql_modules` and `sys.procedures`. These views contain the definitions of your SQL Server objects, including stored procedures.
Using T-SQL to Search
You can execute the following T-SQL query to find stored procedures containing a specific text string:
“`sql
SELECT
p.name AS ProcedureName,
m.definition AS Definition
FROM
sys.procedures AS p
JOIN
sys.sql_modules AS m ON p.object_id = m.object_id
WHERE
m.definition LIKE ‘%YourSearchText%’
ORDER BY
p.name;
“`
Replace `YourSearchText` with the term you wish to search for. This query retrieves the names and definitions of all stored procedures that include the specified text.
Understanding the Query Components
- `sys.procedures`: This system view contains a row for each stored procedure in the database.
- `sys.sql_modules`: This view holds the definitions of SQL objects, such as stored procedures and functions.
- `JOIN` Clause: This combines the two views based on the `object_id`, allowing you to access both the name and the definition of the stored procedures.
- `LIKE` Operator: This is used to search for a specified pattern in the definition text.
Alternative Method: Using OBJECT_DEFINITION
Another method for retrieving stored procedure definitions is using the `OBJECT_DEFINITION` function. You can loop through each stored procedure with a cursor or use a set-based approach:
“`sql
DECLARE @ProcedureName NVARCHAR(255);
DECLARE @Definition NVARCHAR(MAX);
DECLARE proc_cursor CURSOR FOR
SELECT name
FROM sys.procedures;
OPEN proc_cursor;
FETCH NEXT FROM proc_cursor INTO @ProcedureName;
WHILE @@FETCH_STATUS = 0
BEGIN
SET @Definition = OBJECT_DEFINITION(OBJECT_ID(@ProcedureName));
IF @Definition LIKE ‘%YourSearchText%’
BEGIN
PRINT @ProcedureName;
END
FETCH NEXT FROM proc_cursor INTO @ProcedureName;
END
CLOSE proc_cursor;
DEALLOCATE proc_cursor;
“`
This script will print the names of the procedures that contain the specified text.
Performance Considerations
When searching through stored procedures, consider the following:
- Database Size: Large databases may take longer to search.
- Execution Plan: The execution plan can affect performance; avoid complex queries if possible.
- Regular Maintenance: Regularly update statistics and perform index maintenance to optimize query performance.
Using SQL Server Management Studio (SSMS)
For users who prefer a graphical interface, SQL Server Management Studio (SSMS) offers a built-in feature:
- Open SSMS and connect to your database instance.
- Expand the database and navigate to the “Programmability” section.
- Right-click on “Stored Procedures” and select “Filter” > “Filter Settings.”
- In the filter settings, you can specify the search criteria for the stored procedure names.
This method allows for a more user-friendly approach to searching through stored procedures without needing to write T-SQL queries.
Utilizing these methods enables efficient text searches within stored procedures in SQL Server, aiding developers and database administrators in managing and maintaining their SQL environment effectively.
Expert Insights on Searching Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “To effectively search for text within all stored procedures in SQL Server, utilizing the `sys.sql_modules` system view alongside `OBJECT_DEFINITION` can yield comprehensive results. This approach allows developers to retrieve the definition of stored procedures and apply pattern matching techniques such as `LIKE` or full-text search capabilities.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “When searching for specific text in stored procedures, I recommend creating a dedicated script that iterates through the `sys.procedures` and `sys.sql_modules` tables. This method not only enhances performance but also provides a clear pathway to identify dependencies and potential areas of refactoring.”
Lisa Patel (SQL Server Consultant, OptimizeDB). “Leveraging SQL Server Management Studio’s built-in search functionality can significantly streamline the process of locating text within stored procedures. Additionally, implementing regular expressions in combination with T-SQL can further refine search results, especially in large databases with numerous stored procedures.”
Frequently Asked Questions (FAQs)
How can I search for specific text in all stored procedures in SQL Server?
You can use the system views `sys.sql_modules` and `sys.objects` to search for specific text in all stored procedures. The following SQL query can be used:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to search for text in stored procedures using SQL Server Management Studio (SSMS)?
Yes, you can use the “Find in Files” feature in SSMS. Press `Ctrl + Shift + F`, enter your search text, select the “Project” or “Solution” to search, and execute the search to find occurrences in stored procedures.
Can I search for text in stored procedures using T-SQL?
Yes, T-SQL can be used to search for text within stored procedures by querying the `sys.sql_modules` or `INFORMATION_SCHEMA.ROUTINES` views, as shown in the previous example.
What permissions are required to search text in stored procedures?
You need at least `VIEW DEFINITION` permission on the database to access the definitions of stored procedures. Without this permission, you may not be able to see the procedure definitions.
Are there any tools available for searching text in stored procedures in SQL Server?
Yes, several third-party tools, such as Redgate SQL Search and ApexSQL Search, provide advanced features for searching and navigating through stored procedures and other database objects.
Can I automate the search for text in stored procedures?
Yes, you can create a SQL Server Agent job or a script that runs periodically to search for specific text in stored procedures and log the results for review.
Searching for text within all stored procedures in SQL Server is a common requirement for database administrators and developers. This task is essential for understanding dependencies, maintaining code quality, and ensuring that changes in business logic are consistently applied across the database. SQL Server provides several methods to achieve this, including querying system views and using built-in functions that can help locate specific text strings within the definitions of stored procedures.
One effective approach is to utilize the `sys.sql_modules` system view, which contains the definitions of all SQL Server objects, including stored procedures. By combining this view with the `sys.objects` view, users can filter results to target only stored procedures and search for specific keywords or phrases. This method allows for efficient identification of relevant stored procedures that may require updates or modifications based on the search criteria.
Additionally, employing tools such as SQL Server Management Studio (SSMS) can streamline the process. SSMS offers a built-in search feature that allows users to search through the entire database for specific text, including within stored procedures. This user-friendly interface can save time and enhance productivity, especially in larger databases with numerous stored procedures.
In summary, searching for text in all stored procedures in SQL Server is a vital task that can
Author Profile
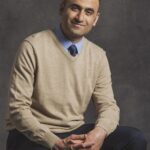
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?