How Can I Search for Text in SQL Server Stored Procedures?
In the world of database management, SQL Server stands out as a powerful tool for handling vast amounts of data with precision and efficiency. One of its most valuable features is the ability to create stored procedures—predefined SQL code that can be executed with a single command. As organizations increasingly rely on data-driven decisions, the need to search for specific text within these stored procedures becomes paramount. Whether you’re troubleshooting, optimizing performance, or simply trying to understand the logic behind your database operations, knowing how to effectively search for text in SQL Server stored procedures can save you time and enhance your productivity.
Searching for text within stored procedures may seem straightforward, but it involves a nuanced understanding of SQL Server’s architecture and querying capabilities. From identifying the appropriate system views to leveraging dynamic SQL, there are various techniques that can be employed to locate the exact snippets of code you need. This process not only aids in maintaining clean and efficient code but also empowers developers and database administrators to manage their environments more effectively.
As we delve deeper into this topic, we will explore the methods and best practices for searching text within SQL Server stored procedures. Whether you’re a seasoned SQL developer or just starting your journey, mastering these techniques will equip you with the tools necessary to navigate the complexities of your database landscape with confidence. Get ready to
Using LIKE for Text Search
The `LIKE` operator in SQL Server is a common method for performing text searches within stored procedures. It allows you to search for a specified pattern in a column, making it particularly useful for finding partial matches. The basic syntax is as follows:
“`sql
SELECT column_name
FROM table_name
WHERE column_name LIKE ‘pattern’;
“`
The pattern can include wildcard characters:
- `%` represents zero or more characters.
- `_` represents a single character.
For example, to find any entries that contain the word “data,” you could use:
“`sql
SELECT *
FROM your_table
WHERE your_column LIKE ‘%data%’;
“`
This query retrieves all records where `your_column` contains “data” anywhere within the text.
Implementing Full-Text Search
For more advanced text searching capabilities, SQL Server provides full-text search functionality. This feature is especially beneficial when dealing with large volumes of text data. Full-text indexing allows for more sophisticated searching methods, including searching for words and phrases, as well as ignoring certain common words (stopwords).
To use full-text search, you must first create a full-text index on the table. Here’s a brief overview of the steps involved:
- Create a Full-Text Catalog: This is a container for full-text indexes.
“`sql
CREATE FULLTEXT CATALOG catalog_name AS DEFAULT;
“`
- Create a Full-Text Index: This index is created on the columns you want to search.
“`sql
CREATE FULLTEXT INDEX ON your_table(your_column)
KEY INDEX your_primary_key_index
WITH STOPLIST = SYSTEM;
“`
- Searching with CONTAINS or FREETEXT: You can now perform searches using these functions.
- `CONTAINS` searches for specific words or phrases.
- `FREETEXT` searches for words that are similar to those in the given text.
Example using `CONTAINS`:
“`sql
SELECT *
FROM your_table
WHERE CONTAINS(your_column, ‘data’);
“`
Example using `FREETEXT`:
“`sql
SELECT *
FROM your_table
WHERE FREETEXT(your_column, ‘data analysis’);
“`
Performance Considerations
When implementing text searches in SQL Server, it is essential to consider performance implications. Using `LIKE` with leading wildcards (e.g., `%data`) can lead to full table scans, which may significantly degrade performance. In contrast, full-text indexes are designed for efficient querying of large text fields.
Here are some performance tips:
- Use full-text search for large text fields or when searching for multiple words.
- Avoid using leading wildcards with `LIKE` to improve performance.
- Regularly maintain your indexes to ensure optimal search performance.
Example Stored Procedure
The following example illustrates a stored procedure that utilizes the `LIKE` operator and full-text search:
“`sql
CREATE PROCEDURE SearchRecords
@searchTerm NVARCHAR(100)
AS
BEGIN
SELECT *
FROM your_table
WHERE your_column LIKE ‘%’ + @searchTerm + ‘%’
OR CONTAINS(your_column, @searchTerm);
END
“`
This stored procedure allows users to pass a search term and retrieves records matching that term using both `LIKE` and full-text search for comprehensive results.
Search Method | Use Case | Performance |
---|---|---|
LIKE | Simple pattern matching | Can degrade with leading wildcards |
Full-Text Search | Complex searches across large text | Optimized for performance with indexing |
Searching for Text in SQL Server Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize system views and built-in functions. This method allows you to identify procedures that contain particular keywords or phrases.
Using `sys.sql_modules` and `sys.objects`
SQL Server provides system views that can be queried to find text in stored procedures. The `sys.sql_modules` view contains the definitions of all SQL Server objects, including stored procedures. The `sys.objects` view includes metadata about the objects.
The following SQL query retrieves the names of stored procedures that contain a specified keyword:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
INNER JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%YourSearchText%’
ORDER BY
o.name;
“`
- Replace `YourSearchText` with the text you are looking for.
- The query returns the procedure name and its definition for easy identification.
Using `INFORMATION_SCHEMA.ROUTINES`
Alternatively, you can query the `INFORMATION_SCHEMA.ROUTINES` view, which also provides information about stored procedures. Here’s how to search for text:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE = ‘PROCEDURE’
AND ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
ORDER BY
ROUTINE_NAME;
“`
This method is straightforward and returns similar results as the previous query.
Considerations for Text Search
- Case Sensitivity: The search may be case-sensitive depending on the collation settings of your database. Ensure that your search text matches the case used in the stored procedure definitions.
- Text Length Limitation: The `ROUTINE_DEFINITION` field in `INFORMATION_SCHEMA.ROUTINES` has a maximum length of 4000 characters. If stored procedures are longer, consider using `sys.sql_modules` for complete definitions.
Automating the Search Process
For frequent searches, you can create a stored procedure that takes a search term as a parameter. Here’s an example:
“`sql
CREATE PROCEDURE SearchStoredProcedures
@SearchText NVARCHAR(100)
AS
BEGIN
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
INNER JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’
AND m.definition LIKE ‘%’ + @SearchText + ‘%’
ORDER BY
o.name;
END;
“`
- Execute this stored procedure by passing the search term to streamline the search process.
Utilizing the `sys.sql_modules` and `INFORMATION_SCHEMA.ROUTINES` views allows for effective searching of text within stored procedures in SQL Server. Custom stored procedures can further enhance usability and efficiency in performing these searches.
Expert Insights on Searching Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, TechSolutions Inc.). “When searching for text within SQL Server stored procedures, it is crucial to utilize the system catalog views, particularly `sys.sql_modules`, which contain the definitions of stored procedures. This allows for effective querying of procedure definitions for specific text patterns.”
Michael Tran (Senior SQL Developer, Data Dynamics). “Using the `LIKE` operator in combination with `OBJECT_DEFINITION` can be an efficient method to search for text in stored procedures. This approach enables developers to pinpoint specific keywords or phrases that may be critical for maintenance and debugging.”
Linda Patel (SQL Server Consultant, OptimizeDB). “For comprehensive text searches, consider implementing a script that iterates through all stored procedures and utilizes regular expressions. This method enhances the search capabilities beyond simple substring matches, making it easier to find complex patterns in your SQL code.”
Frequently Asked Questions (FAQs)
How can I search for specific text within a SQL Server stored procedure?
You can search for specific text within a SQL Server stored procedure by querying the `sys.sql_modules` system view or using the `OBJECT_DEFINITION` function. For example, you can execute:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to find all stored procedures that contain a specific keyword?
Yes, you can find all stored procedures containing a specific keyword by using the same `sys.sql_modules` view. Use the `LIKE` operator to filter results based on the keyword you are searching for.
Can I search for text in stored procedures using SQL Server Management Studio (SSMS)?
Yes, you can search for text in stored procedures using the “Find in Files” feature in SSMS. Press `Ctrl + Shift + F`, enter your search text, and specify the search scope to include stored procedures.
What SQL command can I use to list all stored procedures in a database?
You can list all stored procedures in a database using the following SQL command:
“`sql
SELECT name
FROM sys.procedures;
“`
How do I identify the stored procedure that needs modification based on a text search?
After performing a text search using `sys.sql_modules`, you can review the results to identify the specific stored procedure(s) that contain the text. Analyze the definition to determine if modifications are necessary.
Can I automate the search for text within stored procedures?
Yes, you can automate the search by creating a SQL script or stored procedure that encapsulates the search logic. This script can be executed periodically or triggered based on specific events.
In summary, searching for text within SQL Server using stored procedures is a powerful technique that enhances data retrieval capabilities. By leveraging SQL’s built-in functions such as `CHARINDEX`, `PATINDEX`, and `LIKE`, developers can create stored procedures that efficiently locate specific text patterns within columns of a database. This functionality is particularly useful for applications that require dynamic querying based on user input or for auditing and reporting purposes.
Moreover, implementing full-text search capabilities can significantly improve performance and accuracy when dealing with large datasets. Full-text indexes allow for more complex queries, including searching for words or phrases, and can handle linguistic variations, making them ideal for applications that require advanced search functionalities. Understanding how to create and manage these indexes is crucial for optimizing search operations in SQL Server.
Key takeaways from the discussion include the importance of choosing the right search method based on the specific requirements of the application. While simple text searches can be accomplished using basic SQL functions, more sophisticated needs may necessitate the use of full-text search features. Additionally, careful consideration of indexing strategies can lead to improved performance and user experience when retrieving data.
Author Profile
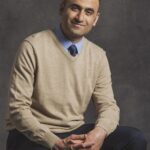
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?