How Can I Use SQL to Search Stored Procedures for Specific Text?
In the world of database management, stored procedures play a pivotal role in encapsulating complex logic and streamlining operations. However, as databases grow in size and complexity, so does the challenge of managing and navigating through these stored procedures. Whether you’re troubleshooting an issue, optimizing performance, or simply trying to understand the code better, the ability to efficiently search for specific text within stored procedures becomes an invaluable skill. This article will guide you through the process of using SQL to uncover the hidden gems within your database, allowing you to harness the full potential of your stored procedures.
Searching for text in stored procedures can seem daunting, especially when dealing with large databases that contain numerous scripts and functions. Fortunately, SQL provides powerful tools that enable developers and database administrators to quickly locate specific keywords or phrases within their stored procedure definitions. By leveraging system catalog views and dynamic management views, you can create queries that sift through the metadata of your database, pinpointing the exact locations where your desired text appears.
In this exploration, we will delve into various techniques and best practices for crafting effective SQL queries that can streamline your search process. From understanding the underlying structure of your database to utilizing specific functions designed for text searching, you’ll gain insights that will not only enhance your efficiency but also empower you to maintain and optimize your database
Understanding the Need for Searching Stored Procedures
Stored procedures are essential components of database management systems, encapsulating business logic and database operations. As applications evolve, the requirement to search through these procedures for specific text or keywords becomes increasingly important. This need arises from various scenarios, such as debugging, refactoring, or assessing impact during changes in business requirements.
SQL Query to Search Stored Procedures
To search for specific text within stored procedures in SQL Server, you can utilize the `INFORMATION_SCHEMA.ROUTINES` view or the `sys.sql_modules` system view. Below are examples of SQL queries that can be employed for this purpose.
Using `INFORMATION_SCHEMA.ROUTINES`:
“`sql
SELECT ROUTINE_NAME, ROUTINE_DEFINITION
FROM INFORMATION_SCHEMA.ROUTINES
WHERE ROUTINE_DEFINITION LIKE ‘%YourSearchText%’
AND ROUTINE_TYPE=’PROCEDURE’;
“`
Using `sys.sql_modules`:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName, definition
FROM sys.sql_modules
WHERE definition LIKE ‘%YourSearchText%’;
“`
Both methods will return the names of stored procedures containing the specified text along with their definitions.
Considerations When Searching
When performing searches through stored procedures, consider the following:
- Case Sensitivity: Depending on your database collation settings, searches might be case-sensitive.
- Wildcards: Utilize the `%` wildcard in your `LIKE` clauses to broaden your search.
- Permissions: Ensure you have the necessary permissions to access the metadata of the stored procedures.
Examples of Use Cases
The following table summarizes typical scenarios where searching through stored procedures is beneficial:
Use Case | Description |
---|---|
Debugging | Identifying problematic code or logic errors within stored procedures. |
Impact Analysis | Determining how changes in one procedure affect others or the overall application. |
Documentation | Updating or creating documentation based on existing stored procedures. |
Refactoring | Finding text for refactoring or optimizing code within stored procedures. |
Advanced Search Techniques
For more complex searches, consider the following advanced techniques:
- Regular Expressions: Some SQL databases support regex for more sophisticated text searches.
- Full-Text Search: Implementing full-text search capabilities can enhance the ability to search large bodies of text efficiently.
- Third-Party Tools: Various database management tools offer graphical interfaces and enhanced search capabilities for stored procedures.
By applying these methods, you can effectively manage and maintain your stored procedures, ensuring that your database remains efficient and aligned with business needs.
Searching for Text in Stored Procedures
Stored procedures often contain critical business logic within a database. Searching for specific text within these procedures can be essential for maintenance, debugging, or refactoring tasks. Various SQL Server systems provide methods to query metadata for this purpose.
Using SQL Server System Views
SQL Server maintains several system views that can be utilized to search for text in stored procedures. The most commonly used views include `sys.sql_modules` and `sys.objects`. The following SQL query demonstrates how to search for a specific text string within all stored procedures:
“`sql
SELECT
o.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ AND
m.definition LIKE ‘%your_search_text%’
ORDER BY
o.name;
“`
This query provides:
- ProcedureName: The name of the stored procedure.
- ProcedureDefinition: The complete definition of the stored procedure.
Replace `your_search_text` with the text you are looking for.
Using INFORMATION_SCHEMA Views
Another method involves utilizing the `INFORMATION_SCHEMA.ROUTINES` view. This approach is more ANSI-compliant and can be beneficial when working across different database systems. Here’s an example of how to implement this:
“`sql
SELECT
ROUTINE_NAME,
ROUTINE_DEFINITION
FROM
INFORMATION_SCHEMA.ROUTINES
WHERE
ROUTINE_TYPE=’PROCEDURE’ AND
ROUTINE_DEFINITION LIKE ‘%your_search_text%’
ORDER BY
ROUTINE_NAME;
“`
This will yield similar results but may vary in performance depending on the database schema.
Performance Considerations
Searching through large stored procedures can be resource-intensive. Consider the following tips to optimize performance:
- Limit Scope: Narrow down your search to specific schemas if possible.
- Use Full-Text Indexing: For extensive textual searches, consider implementing full-text indexing if supported by your SQL Server version.
- Batch Searches: If searching across multiple databases, batch your queries to avoid overwhelming the server.
Alternative Methods
For databases that may not support the above methods or for more complex scenarios, consider:
- Scripting Tools: Use SQL Server Management Studio (SSMS) scripting capabilities to export definitions and perform text searches using external tools like text editors or IDEs.
- Third-Party Tools: There are various third-party database management tools that offer advanced search functionalities, which can simplify the process.
By employing the appropriate SQL queries and techniques, one can efficiently search for text within stored procedures, ensuring effective database management and maintenance.
Searching Stored Procedures for Text: Expert Insights
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “To effectively search stored procedures for specific text, utilizing system catalog views such as INFORMATION_SCHEMA.ROUTINES or sys.sql_modules can be invaluable. These views allow for querying the definition of stored procedures, enabling developers to pinpoint exact matches for the desired text.”
Michael Tran (Senior SQL Developer, Data Solutions Group). “Implementing a script that leverages the OBJECT_DEFINITION function can streamline the process of searching through stored procedures. This method not only enhances performance but also simplifies the retrieval of relevant code snippets containing the specified text.”
Linda Zhao (Data Analyst, Analytics Hub). “For teams managing extensive databases, creating a dedicated search tool that indexes stored procedure definitions can significantly reduce search time. By integrating full-text search capabilities, users can quickly locate procedures containing specific keywords or phrases.”
Frequently Asked Questions (FAQs)
How can I search for specific text within stored procedures in SQL?
You can search for specific text within stored procedures by querying the `sys.sql_modules` and `sys.objects` system views. Use a SQL query like:
“`sql
SELECT OBJECT_NAME(object_id), definition
FROM sys.sql_modules
WHERE definition LIKE ‘%your_search_text%’;
“`
Is there a way to search for text in stored procedures using SQL Server Management Studio (SSMS)?
Yes, in SSMS, you can use the “Object Explorer” to find stored procedures. Right-click on the database, select “Find,” and then choose “Find in Object Explorer.” Enter the text you want to search for.
Can I search for text in stored procedures across multiple databases?
Yes, you can search across multiple databases by using a cursor to iterate through the databases and execute the search query for each database context. Alternatively, you can use a script that dynamically constructs and executes the search query.
What permissions are required to search stored procedures in SQL Server?
To search stored procedures, you typically need at least `VIEW DEFINITION` permission on the database. Without this permission, you may not be able to access the definitions of the stored procedures.
Are there any tools available to search for text in stored procedures more efficiently?
Yes, there are several third-party tools available, such as Redgate SQL Search and ApexSQL Search, which provide advanced searching capabilities for SQL Server objects, including stored procedures.
What should I do if the search text returns no results?
If the search text returns no results, ensure that the text is correctly spelled and formatted. Additionally, check if you are searching in the correct database and that the stored procedures exist.
In summary, searching for specific text within stored procedures in SQL databases is a crucial task for database administrators and developers. This process allows for the identification of procedures that contain particular keywords or phrases, which can be essential for debugging, updating, or understanding the database schema. Various methods exist to accomplish this, including querying system catalog views or using built-in functions that provide metadata about stored procedures.
One effective approach is to utilize the `INFORMATION_SCHEMA.ROUTINES` or `sys.sql_modules` views, which store definitions of stored procedures. By executing a SQL query that filters these views based on the `definition` column, users can pinpoint the procedures that include the desired text. Additionally, leveraging tools like SQL Server Management Studio (SSMS) can simplify this process through its search functionality, allowing for a more user-friendly experience.
Key takeaways from this discussion include the importance of maintaining clear documentation and understanding the structure of stored procedures for efficient searching. Furthermore, being familiar with the SQL syntax and available system views can significantly enhance one’s ability to navigate and manage complex databases. Ultimately, mastering these techniques contributes to better database management practices and improved overall efficiency in development workflows.
Author Profile
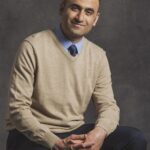
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?