Is SQLite’s LAG Function Usable in the WHERE Clause?
In the realm of data analysis and manipulation, SQL has long stood as a powerful tool, and SQLite is no exception. Among its many features, the ability to use window functions like `LAG()` has revolutionized how we handle time-series data and sequential records. However, as users delve deeper into the intricacies of SQLite, a common question arises: can you utilize the `LAG()` function within a `WHERE` clause? This query opens the door to a broader discussion about the capabilities and limitations of SQLite, as well as the creative solutions that can be employed to achieve desired results.
The `LAG()` function, which allows users to access data from a previous row in a result set, can be a game-changer for tasks involving comparisons and trend analysis. However, its placement within SQL queries often leads to confusion, particularly regarding filtering conditions. While traditional SQL practices might suggest that window functions are best suited for the `SELECT` clause, the challenge of incorporating them into the `WHERE` clause presents a unique puzzle for developers and analysts alike.
Understanding the nuances of how `LAG()` interacts with other SQL components not only enhances one’s proficiency in SQLite but also broadens the scope of analytical possibilities. By exploring the theoretical underpinnings and practical applications
Understanding LAG Functionality in SQLite
The `LAG` function in SQLite is a powerful analytical tool that allows users to access data from a previous row in the result set without the need for a self-join. It is commonly used in scenarios where comparisons between current and previous entries are essential, such as time-series data analysis. However, utilizing `LAG` within a `WHERE` clause presents certain limitations due to its nature.
Limitations of Using LAG in WHERE Clauses
One of the primary restrictions of using the `LAG` function is that it cannot be directly incorporated into a `WHERE` clause. The `WHERE` clause is evaluated before the window functions, including `LAG`, are processed. Consequently, any attempt to filter rows based on the output of `LAG` will result in an error. Instead, alternatives must be employed to achieve the desired filtering.
Alternative Approaches
To work around the limitation of `LAG` in the `WHERE` clause, consider the following strategies:
- Common Table Expressions (CTEs): Utilize a CTE to first compute the `LAG` values, then filter the results in the main query.
- Subqueries: Similar to CTEs, subqueries can be used to calculate the lagged values and subsequently apply conditions in the outer query.
Example Using a Common Table Expression
Here is an example illustrating how to effectively use a CTE to filter results based on a `LAG` calculation:
“`sql
WITH lagged_data AS (
SELECT
id,
value,
LAG(value) OVER (ORDER BY id) AS previous_value
FROM
my_table
)
SELECT
id,
value,
previous_value
FROM
lagged_data
WHERE
previous_value > 10; — Filter condition on lagged value
“`
In this example, the `LAG` function calculates the previous value for each row, and the outer query applies the filtering condition.
Performance Considerations
When using `LAG` in combination with CTEs or subqueries, it is essential to consider the potential performance implications:
- Execution Time: CTEs and subqueries can increase execution time if the dataset is large, as the database must compute the `LAG` values before filtering.
- Indexes: Ensure that appropriate indexes are in place on the columns involved in the `ORDER BY` clause to enhance performance.
Method | Description | Performance Impact |
---|---|---|
CTE | Calculates lagged values first, then filters. | Can be slower with large datasets. |
Subquery | Similar to CTE but within a nested query. | May increase complexity and execution time. |
By understanding these limitations and approaches, users can effectively leverage the `LAG` function within their SQLite queries while ensuring that they adhere to best practices for performance and clarity.
Understanding the LAG Function in SQLite
The `LAG` function in SQLite is a window function that allows users to access data from a previous row in the same result set without the need for a self-join. It is particularly useful for comparing values in sequential rows. The basic syntax of the `LAG` function is as follows:
“`sql
LAG(column_name, offset, default_value) OVER (PARTITION BY partition_column ORDER BY order_column)
“`
- column_name: The column from which you want to retrieve the value.
- offset: The number of rows back from the current row to fetch the value (default is 1).
- default_value: The value to return if the offset goes beyond the window (optional).
- PARTITION BY: Divides the result set into partitions to which the function is applied.
- ORDER BY: Specifies the order of rows within each partition.
Limitations of Using LAG in the WHERE Clause
In SQLite, the `WHERE` clause is used to filter records before the window functions (like `LAG`) are applied. This limitation means you cannot directly use the results of a `LAG` function within the same `WHERE` clause. Instead, you can use a common table expression (CTE) or a subquery to first compute the `LAG` values and then filter based on those computed values.
Example of Using LAG with CTE
Here’s how to structure a query with a `CTE` to utilize the `LAG` function effectively:
“`sql
WITH lagged_data AS (
SELECT
id,
value,
LAG(value) OVER (ORDER BY id) AS previous_value
FROM
your_table
)
SELECT
id,
value,
previous_value
FROM
lagged_data
WHERE
previous_value > 100; — Filtering based on the lagged value
“`
In this example:
- The `CTE` named `lagged_data` computes the `previous_value` using `LAG`.
- The outer query then filters for rows where the `previous_value` exceeds 100.
Alternative Approaches to Filter with LAG
If you prefer not to use a CTE, you can also achieve similar results with a subquery. Here’s an alternative example:
“`sql
SELECT
id,
value,
previous_value
FROM (
SELECT
id,
value,
LAG(value) OVER (ORDER BY id) AS previous_value
FROM
your_table
) AS subquery
WHERE
previous_value > 100; — Applying filter after computing lag
“`
This structure ensures that the `LAG` calculation is complete before filtering, allowing you to use the computed values effectively.
Performance Considerations
When using `LAG` in conjunction with filtering operations, consider the following:
- Indexes: Ensure that the columns used in the `ORDER BY` clause are indexed to enhance performance.
- Partition Size: When using `PARTITION BY`, be mindful of the partition size as larger partitions may slow down performance.
- Data Size: For large datasets, consider testing performance with different query structures (CTE vs. subquery).
By employing these strategies, you can effectively work with the `LAG` function in SQLite while adhering to its limitations regarding the `WHERE` clause.
Understanding the Use of LAG in SQLite WHERE Clauses
Dr. Emily Carter (Database Systems Researcher, Tech Innovations Journal). “Using the LAG function within a WHERE clause in SQLite can lead to unexpected results, as LAG is designed to operate on the result set after filtering. It is essential to understand that LAG computes values based on the order of rows, and its application in a WHERE clause may not yield the intended filtering effect.”
Michael Chen (Senior Data Analyst, Data Insights Corp). “In SQLite, the LAG function is typically used in the SELECT statement to access data from previous rows. Attempting to use it in a WHERE clause is not standard practice and can cause confusion. Instead, consider using a subquery or a CTE to achieve similar results while maintaining clarity in your SQL logic.”
Sarah Patel (SQL Developer, CodeCraft Solutions). “While it is technically possible to use LAG in conjunction with a WHERE clause, it is generally not advisable. The LAG function is evaluated after the WHERE clause, which means it does not influence row filtering directly. To leverage previous row values effectively, one should restructure the query to ensure LAG is used appropriately in the SELECT context.”
Frequently Asked Questions (FAQs)
Can I use the LAG function in the WHERE clause of an SQLite query?
No, the LAG function cannot be directly used in the WHERE clause because it is a window function that operates on the result set after the WHERE clause is processed.
How can I filter results based on LAG values in SQLite?
To filter results based on LAG values, you can use a Common Table Expression (CTE) or a subquery. First, calculate the LAG values in the CTE or subquery, then apply the filtering in the outer query.
What is the purpose of the LAG function in SQLite?
The LAG function allows you to access data from a previous row in the result set, making it useful for comparing current row values with those of preceding rows.
Are there any performance considerations when using LAG in SQLite?
Yes, using LAG can impact performance, especially on large datasets, as it requires the database to compute values for each row. Proper indexing and query optimization can help mitigate performance issues.
Can I use LAG with ORDER BY in SQLite?
Yes, the LAG function must be used with an ORDER BY clause to define the order of rows. This ensures that the function retrieves the correct preceding row based on the specified criteria.
What versions of SQLite support the LAG function?
The LAG function is supported starting from SQLite version 3.25.0. Ensure your SQLite version is up to date to utilize this feature effectively.
In SQLite, the use of the LAG() function is primarily for analytical purposes, allowing users to access data from a previous row in a result set. However, it is important to note that the LAG() function cannot be directly utilized within a WHERE clause. The WHERE clause is executed before the window functions, such as LAG(), which means that any filtering based on the results of LAG() must be performed in a subsequent step, typically using a subquery or a Common Table Expression (CTE).
To effectively use LAG() in conjunction with filtering conditions, one can first create a derived table or a CTE that computes the LAG values. This intermediate result can then be filtered in an outer query. This approach allows for the necessary calculations to be performed while still enabling the application of conditional logic based on the results of those calculations.
In summary, while LAG() is a powerful tool for analyzing data trends and patterns, its limitations in the WHERE clause necessitate a more structured approach to querying. By leveraging subqueries or CTEs, users can effectively incorporate LAG() results into their data analysis workflows, ensuring that they can filter based on previous row values as needed.
Author Profile
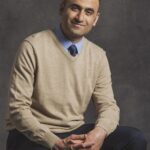
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?