Why Am I Getting SQLSTATE[HY000]: General Error: 1 No Such Column: Test?
In the world of database management, encountering errors can be a frustrating yet enlightening experience. One such error that developers and database administrators often face is the notorious `SQLSTATE[HY000]: General error: 1 no such column: test`. This cryptic message can halt your progress and leave you scratching your head, questioning the integrity of your database schema or the accuracy of your SQL queries. Understanding this error is crucial for anyone working with SQL databases, as it not only highlights potential pitfalls in your code but also serves as a gateway to mastering effective database management practices.
When you see the “no such column” error, it typically indicates that your SQL query is attempting to access a column that doesn’t exist in the specified table. This could be due to a simple typo, a misunderstanding of the database structure, or even an oversight in the migration process. As databases evolve, maintaining awareness of schema changes is essential to prevent such errors from derailing your work. Moreover, this error can serve as a valuable teaching moment, prompting developers to refine their debugging skills and improve their understanding of SQL syntax and database design.
As we delve deeper into this topic, we will explore the common causes of the `SQLSTATE[HY000]: General error: 1 no such column:
Understanding the Error
The error message `sqlstate[hy000]: general error: 1 no such column: test` typically indicates that the SQL query you are executing is referencing a column named “test,” which does not exist in the specified database table. This can occur for several reasons, including:
- Typographical Errors: A simple misspelling of the column name.
- Incorrect Table References: The query may be targeting the wrong table where the column is not present.
- Schema Changes: If the database schema has been altered (e.g., columns added or removed), your query may need to be updated accordingly.
Troubleshooting Steps
To resolve this error, consider the following troubleshooting steps:
- Check Column Names: Verify the column name in your SQL statement. Ensure it matches the column names in the database schema exactly, including case sensitivity.
- Inspect the Table Structure: Use a query to list the columns of the table to confirm their existence. For example:
“`sql
PRAGMA table_info(your_table_name);
“`
- Review Query Logic: Ensure that your SQL query is logically sound and correctly references the intended table.
- Examine Database Schema: If you recently modified the database schema, double-check that the changes were applied correctly.
Common Scenarios Leading to the Error
The following scenarios frequently lead to the “no such column” error:
Scenario | Description |
---|---|
Misspelled Column Name | Using a typo in the column name in your SQL query. |
Wrong Table Name | Referencing a different table that does not contain the specified column. |
Database Migration Issues | Failing to update queries after migrating to a new database version. |
Case Sensitivity | Some databases are case-sensitive, leading to issues if not matched properly. |
Preventive Measures
To avoid encountering this error in the future, consider the following best practices:
- Maintain Consistency: Use consistent naming conventions for columns and tables across your database.
- Version Control: Keep track of database schema changes with version control systems.
- Documentation: Maintain clear documentation of your database schema to reference during query development.
- Testing: Test your SQL queries in a development environment before executing them in production.
By adhering to these guidelines, you can significantly reduce the likelihood of encountering the `sqlstate[hy000]: general error: 1 no such column: test` error in your SQL operations.
Understanding the Error Message
The error message `sqlstate[hy000]: general error: 1 no such column: test` indicates that a SQL query is attempting to reference a column named `test`, which does not exist in the specified database table. This situation can arise due to various reasons, and understanding these can help in troubleshooting.
Common Causes of the Error
- Misspelled Column Name:
- The most frequent cause is a typo in the column name. Ensure that the column name `test` is spelled correctly in the query.
- Column Does Not Exist:
- The column might not have been created. Check the table structure to confirm the existence of the column.
- Database Context Issues:
- If multiple databases are in use, ensure that the query is directed to the correct database where the column is defined.
- Case Sensitivity:
- Some database systems are case-sensitive. Verify whether the column name should be in upper or lower case.
- Schema Changes:
- If the database schema has changed recently (e.g., columns added or removed), the current query might be outdated.
Troubleshooting Steps
To resolve the error effectively, follow these troubleshooting steps:
- Check Column Names:
- Run a query to retrieve the schema of the table:
“`sql
PRAGMA table_info(table_name);
“`
- Validate Database Context:
- Confirm that you are connected to the correct database. You can check the current database with:
“`sql
SELECT name FROM sqlite_master WHERE type=’table’;
“`
- Inspect Query Syntax:
- Review the SQL query for any incorrect syntax or misplaced punctuation.
- Review Recent Changes:
- If the database structure has been modified recently, ensure that all queries are updated accordingly.
Example of Correcting the Error
Consider the following example that generates the error:
“`sql
SELECT name, test FROM users;
“`
If the column `test` does not exist, you can correct it by:
- Checking the correct column name:
“`sql
SELECT name, age FROM users; — Assuming ‘age’ is a valid column
“`
- If the column should exist, create it using:
“`sql
ALTER TABLE users ADD COLUMN test TEXT; — Adds the column ‘test’
“`
Preventive Measures
To avoid encountering the `no such column` error in the future, consider implementing the following practices:
- Maintain Documentation: Keep detailed documentation of the database schema, including column names and types.
- Use ORM Tools: Employ Object-Relational Mapping (ORM) tools that can abstract and manage the database schema, reducing direct SQL queries.
- Regular Schema Reviews: Conduct regular reviews of the database schema to ensure that all queries are in alignment with the current structure.
- Testing Queries: Before deploying changes, test all SQL queries in a development environment to catch errors early.
By adhering to these guidelines, developers can minimize the likelihood of running into the `no such column` error, enhancing the overall efficiency and reliability of database interactions.
Understanding SQL Errors: Insights on ‘no such column’
Dr. Lisa Chen (Database Architect, Tech Innovations Inc.). “The error ‘no such column: test’ typically indicates that the SQL query is referencing a column that does not exist in the specified table. This can occur due to typos in the column name or changes in the database schema that have not been accounted for in the query.”
Mark Thompson (Senior Data Analyst, Data Solutions Group). “When encountering the SQLSTATE[HY000]: general error: 1 no such column: test, it is crucial to verify the database structure. Ensure that the column name is spelled correctly and that it exists in the context of the query being executed. Additionally, consider checking if the correct database is being accessed.”
Sarah Patel (Lead Software Engineer, CodeCraft Labs). “This error often arises in scenarios involving migrations or schema updates. Developers should implement rigorous testing to ensure that all queries align with the current database schema, as discrepancies can lead to runtime errors like this one.”
Frequently Asked Questions (FAQs)
What does the error message “sqlstate[hy000]: general error: 1 no such column: test” indicate?
This error indicates that the SQL query is attempting to reference a column named “test” that does not exist in the specified table within the database.
How can I resolve the “no such column” error in SQL?
To resolve this error, verify the column name in your query against the actual table schema. Ensure that the column exists and is correctly spelled, including case sensitivity.
What steps should I take to check the database schema for the correct column names?
You can use SQL commands such as `PRAGMA table_info(table_name);` in SQLite or `DESCRIBE table_name;` in MySQL to list all columns in a specific table and confirm their names and data types.
Could this error occur due to a typo in the SQL query?
Yes, a typo in the SQL query, such as a misspelled column name, can lead to this error. Always double-check your SQL syntax for accuracy.
Is it possible that the column was deleted or renamed in the database?
Yes, if the column was deleted or renamed after the last successful query execution, it could lead to this error. Review recent changes to the database schema to confirm.
What should I do if the column exists but I still encounter this error?
If the column exists but the error persists, check for issues such as incorrect table references, database connection problems, or the use of the wrong database context in your query.
The error message “SQLSTATE[HY000]: general error: 1 no such column: test” indicates a problem encountered when executing an SQL query. This specific error arises when the database engine cannot locate a column named “test” in the specified table. This situation often occurs due to typographical errors in the column name, changes in the database schema, or querying a table that does not contain the expected structure.
It is essential to verify the database schema to ensure that the column exists and is correctly spelled. Additionally, checking the context in which the query is executed can provide insights into whether the correct table is being referenced. In some cases, this error may also stem from using an outdated database version or a migration that did not execute as intended, leading to discrepancies in the expected database structure.
To resolve this issue, developers should review their SQL queries and confirm the existence of the column in the database. Utilizing database management tools or executing schema inspection queries can aid in identifying the correct structure. Furthermore, maintaining thorough documentation of database changes and schema updates can prevent similar errors in the future, ensuring a smoother development process.
Author Profile
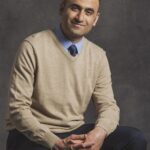
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?