How Can You Compute the R² Score on a Test Set Using Statsmodels?
In the realm of statistical modeling and machine learning, the R² score, or coefficient of determination, serves as a vital metric for evaluating the performance of regression models. As practitioners delve into the intricacies of model evaluation, understanding how to compute the R² score on a test set using libraries like Statsmodels becomes essential. This powerful Python library not only facilitates the creation of robust statistical models but also provides tools to assess their predictive accuracy. In this article, we will explore the significance of the R² score, the role of test sets in model validation, and how Statsmodels can streamline the process of calculating this critical metric.
The R² score quantifies how well a regression model explains the variability of the target variable, offering insights into its predictive power. A high R² value indicates that a significant portion of the variance is captured by the model, while a low value suggests that the model may not be effectively capturing the underlying patterns in the data. When evaluating a model, it is crucial to compute the R² score on a test set, which consists of data that was not used during the training phase. This helps ensure that the model’s performance is assessed on unseen data, providing a more accurate reflection of its generalizability.
Statsmodels, a comprehensive library for statistical modeling
Computing R² Score with Statsmodels
To compute the R² score for a model using the Statsmodels library, it is essential to understand the context in which R² is used. The R² score, or coefficient of determination, measures the proportion of variance in the dependent variable that can be predicted from the independent variables. A higher R² value indicates a better fit of the model to the data.
After fitting a model, you can assess its performance on a test set by calculating the R² score. This process typically involves the following steps:
- Prepare your dataset: Split your data into training and test sets. This allows for model evaluation on unseen data.
- Fit the model: Use the training data to fit your statistical model.
- Make predictions: Use the fitted model to predict the outcomes on the test set.
- Calculate R² score: Compare the predicted outcomes to the actual outcomes to compute the R² score.
Here is a code snippet demonstrating this process using Statsmodels:
“`python
import statsmodels.api as sm
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score
Sample data
X = np.random.rand(100, 2)
y = X @ np.array([1.5, -2]) + np.random.normal(size=100)
Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Add a constant to the model (intercept)
X_train_sm = sm.add_constant(X_train)
X_test_sm = sm.add_constant(X_test)
Fit the model
model = sm.OLS(y_train, X_train_sm).fit()
Predict on test set
y_pred = model.predict(X_test_sm)
Calculate R² score
r2 = r2_score(y_test, y_pred)
print(“R² score:”, r2)
“`
Understanding R² Score Interpretation
Interpreting the R² score can provide insights into the model’s performance. Below are some common interpretations:
- R² = 1: Perfect fit, all variance explained.
- R² = 0: Model does not explain any variance.
- 0 < R² < 1: Indicates the proportion of variance explained by the model; closer to 1 is better.
It is important to consider the context of the data and the model, as a high R² score does not always imply a good model, especially if overfitting occurs.
Summary of R² Score Calculation Steps
The following table summarizes the steps to compute the R² score using Statsmodels:
Step | Description |
---|---|
1 | Prepare the dataset (train/test split) |
2 | Fit the model using training data |
3 | Generate predictions on the test set |
4 | Compute R² score using actual vs predicted values |
By following these procedures, you can effectively assess the performance of your regression model with the R² score using Statsmodels, ensuring a robust evaluation of model accuracy.
Computing R² Score Using Statsmodels
To compute the R² score on a test set using the `statsmodels` library in Python, you first need to fit your model to the training data and then use it to make predictions on the test set. The R² score quantifies how well the model explains the variance in the dependent variable.
Steps to Compute R² Score
- Import Necessary Libraries: Ensure you have `statsmodels` and `sklearn` installed and imported.
“`python
import statsmodels.api as sm
from sklearn.metrics import r2_score
“`
- Fit the Model: Use the training data to fit your regression model.
“`python
X_train = sm.add_constant(X_train) Add a constant to the model (intercept)
model = sm.OLS(y_train, X_train).fit()
“`
- Make Predictions: Generate predictions on the test set.
“`python
X_test = sm.add_constant(X_test) Add a constant to the test set
y_pred = model.predict(X_test)
“`
- Calculate R² Score: Use the `r2_score` function from `sklearn` to compute the R² value.
“`python
r2 = r2_score(y_test, y_pred)
print(“R² Score:”, r2)
“`
Understanding R² Score
The R² score provides insight into how well your model fits the data:
- Values Range:
- 1 indicates perfect prediction.
- 0 indicates that the model does not explain any variance.
- Negative values indicate worse predictions than the mean.
- Interpretation:
- An R² of 0.75 means 75% of the variance in the target variable is explained by the model.
Example Code
Here is a complete example illustrating the entire process:
“`python
import numpy as np
import pandas as pd
import statsmodels.api as sm
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score
Sample data
data = pd.DataFrame({
‘feature1’: np.random.rand(100),
‘feature2’: np.random.rand(100),
‘target’: np.random.rand(100)
})
Splitting the data
X = data[[‘feature1’, ‘feature2’]]
y = data[‘target’]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Fitting the model
X_train = sm.add_constant(X_train)
model = sm.OLS(y_train, X_train).fit()
Making predictions
X_test = sm.add_constant(X_test)
y_pred = model.predict(X_test)
Calculating R² score
r2 = r2_score(y_test, y_pred)
print(“R² Score:”, r2)
“`
Key Considerations
- Ensure that the model assumptions are met (linearity, independence, homoscedasticity).
- R² can be misleading in the presence of outliers; consider using adjusted R² for more robust evaluation.
- Always visualize the residuals to check for patterns that might indicate model inadequacy.
By following these guidelines, one can effectively compute and interpret the R² score using the `statsmodels` library in Python.
Evaluating Model Performance with R² Score in Statsmodels
Dr. Emily Carter (Data Scientist, Predictive Analytics Institute). “To compute the R² score on a test set using Statsmodels, one must first ensure that the model is fitted to the training data. After making predictions on the test set, the R² score can be calculated using the `statsmodels` library’s built-in functions, which provide a comprehensive understanding of the model’s explanatory power.”
Michael Chen (Machine Learning Engineer, Tech Innovations Corp). “Using the R² score is essential for assessing the goodness of fit of a regression model. In Statsmodels, after fitting the model, the `rsquared` attribute can be accessed directly from the results object, which simplifies the process of evaluating performance on the test set.”
Lisa Patel (Quantitative Analyst, Financial Data Solutions). “When working with Statsmodels, it is crucial to interpret the R² score correctly. A high R² value indicates that the model explains a significant portion of the variance in the test set, but it is also important to consider other metrics to avoid overfitting and ensure robust model evaluation.”
Frequently Asked Questions (FAQs)
How can I compute the R² score using statsmodels?
To compute the R² score in statsmodels, you can use the `fit()` method of a model object and access the `rsquared` attribute from the results. This provides the R² value directly after fitting the model to your training data.
Can I calculate the R² score for a test set in statsmodels?
Yes, you can calculate the R² score for a test set by first making predictions on the test data using the fitted model and then comparing these predictions to the actual values using the `r2_score` function from the `sklearn.metrics` module.
What is the purpose of the R² score?
The R² score, or coefficient of determination, measures the proportion of variance in the dependent variable that can be explained by the independent variables in the model. It indicates how well the model fits the data.
Is R² the only metric to evaluate model performance?
No, R² is not the only metric. Other metrics such as Mean Absolute Error (MAE), Mean Squared Error (MSE), and Adjusted R² can provide additional insights into model performance and should be considered depending on the context.
What does an R² score of 1 indicate?
An R² score of 1 indicates that the model explains 100% of the variance in the dependent variable. This means that the predictions perfectly match the actual values, which can occur in overfitting scenarios.
How does one interpret negative R² values?
Negative R² values indicate that the model performs worse than a horizontal line representing the mean of the dependent variable. This suggests that the model does not capture the relationship between the variables effectively.
In the context of using the Statsmodels library in Python for statistical modeling, computing the R² score on a test set is a crucial step in evaluating the performance of regression models. The R² score, also known as the coefficient of determination, quantifies how well the independent variables explain the variability of the dependent variable. A higher R² value indicates a better fit of the model to the data, making it an essential metric for assessing model accuracy on unseen data.
To compute the R² score using Statsmodels, one typically fits a regression model to the training data and then makes predictions on the test set. The predicted values can be compared to the actual values from the test set to calculate the R² score. While Statsmodels itself does not provide a built-in function specifically for R² computation, users can easily derive it using the formula: R² = 1 – (SS_res / SS_tot), where SS_res is the sum of the squared residuals and SS_tot is the total sum of squares. This approach allows for a clear understanding of model performance.
Key takeaways from this discussion include the importance of validating model performance using a separate test set to avoid overfitting and to ensure generalizability. Additionally,
Author Profile
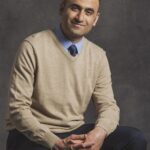
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?