How Can I Convert a String JSON to a JSON Object in Java?
In the world of software development, data interchange formats play a crucial role in enabling seamless communication between systems. Among these formats, JSON (JavaScript Object Notation) has emerged as a favorite due to its simplicity and readability. For Java developers, the ability to convert a string representation of JSON into a usable JSON object is an essential skill that opens the door to a myriad of applications, from web services to mobile apps. This article will guide you through the intricacies of transforming JSON strings into JSON objects in Java, empowering you to handle data more effectively in your projects.
Understanding how to convert a string containing JSON data into a JSON object in Java is fundamental for any developer working with APIs or data storage solutions. This process not only allows for easier manipulation of data but also ensures that applications can dynamically interact with various data sources. As we delve deeper into this topic, we’ll explore the various libraries available in Java, such as Jackson and Gson, which simplify this conversion process and enhance your coding efficiency.
Moreover, the conversion from a JSON string to a JSON object involves several considerations, including error handling and data validation. By mastering this conversion, developers can ensure that their applications are robust and capable of processing data in real-time. Join us as we unpack the methods and best practices for converting
Converting JSON String to JSON Object in Java
In Java, converting a JSON string to a JSON object is a common task, especially when dealing with web APIs or configuration files. The most widely used libraries for this conversion are Jackson and Gson. Below are the steps to achieve this using both libraries.
Using Jackson Library
Jackson is a powerful library for processing JSON data in Java. To convert a JSON string to a JSON object, you typically use the `ObjectMapper` class.
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
MyObject obj = objectMapper.readValue(jsonString, MyObject.class);
System.out.println(obj);
} catch (Exception e) {
e.printStackTrace();
}
}
}
class MyObject {
private String name;
private int age;
// Getters and setters
}
“`
- Key Classes:
- `ObjectMapper`: Main class for reading and writing JSON.
- `MyObject`: Class that defines the structure of the JSON data.
Using Gson Library
Gson is another popular library developed by Google for working with JSON. The process for converting a JSON string to a JSON object is similar to that of Jackson.
“`java
import com.google.gson.Gson;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
Gson gson = new Gson();
MyObject obj = gson.fromJson(jsonString, MyObject.class);
System.out.println(obj);
}
}
class MyObject {
private String name;
private int age;
// Getters and setters
}
“`
- Key Classes:
- `Gson`: Main class for converting JSON to Java objects and vice versa.
- `MyObject`: Class representing the structure of the JSON data.
Comparison of Jackson and Gson
When choosing between Jackson and Gson, consider the following factors:
Feature | Jackson | Gson |
---|---|---|
Performance | Generally faster for large datasets | Good performance, but slower than Jackson |
Data Binding | Supports advanced data binding | Simple data binding |
Annotations | Rich support for annotations | Limited support for annotations |
Ease of Use | More complex, but powerful | Simple and easy to use |
Both libraries are capable of effectively converting JSON strings to JSON objects. The choice between them may depend on specific project requirements, performance needs, and personal preference.
Converting JSON String to JSON Object in Java
In Java, converting a JSON string to a JSON object can be efficiently accomplished using libraries such as Jackson or Gson. Below are methods using both libraries.
Using Jackson Library
Jackson is a popular library for handling JSON in Java. To use Jackson for converting a JSON string to a JSON object, follow these steps:
- Add Jackson Dependency: If you’re using Maven, include the following dependency in your `pom.xml`:
“`xml
“`
- Convert JSON String to Object:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Using Gson Library
Gson is another widely used library for JSON manipulation in Java. To convert a JSON string to a JSON object using Gson, follow these steps:
- Add Gson Dependency: For Maven, include the following in your `pom.xml`:
“`xml
“`
- Convert JSON String to Object:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Comparison of Jackson and Gson
Feature | Jackson | Gson |
---|---|---|
Performance | Generally faster for large data | Slower for large data |
Data Binding | More flexible data binding | Easy to use, but less flexible |
Annotations | Supports more annotations | Limited support for annotations |
JSON Streaming | Yes | No |
Default Value Types | Supports various data types | Limited to JSON-compatible types |
Both libraries are capable of parsing JSON strings efficiently, but your choice may depend on specific project requirements, such as performance needs or ease of use.
Common Errors and Troubleshooting
When converting JSON strings to objects, several common issues may arise:
- Malformed JSON: Ensure the JSON string is well-formed. Use tools like JSONLint to validate.
- Incorrect Data Types: Ensure that the JSON fields match expected Java types (e.g., converting strings to integers).
- Dependencies Missing: Verify that the necessary library dependencies are correctly added to your project.
By following these guidelines and examples, you can effectively convert JSON strings into JSON objects in Java using either the Jackson or Gson library.
Converting String JSON to JSON Object in Java: Expert Insights
Dr. Emily Carter (Lead Software Engineer, Tech Innovations Inc.). The conversion of a string JSON to a JSON object in Java is primarily achieved using libraries such as Jackson or Gson. These libraries provide efficient methods to parse JSON strings and create corresponding Java objects, which simplifies data handling in Java applications.
Michael Chen (Senior Java Developer, CodeCraft Solutions). When dealing with string JSON in Java, it is crucial to ensure that the JSON format is valid before parsing. Using tools like JSONLint can help validate the JSON structure, thereby preventing runtime errors during conversion. Once validated, libraries like Gson offer straightforward APIs to convert strings into JSON objects seamlessly.
Sarah Thompson (Java Architect, Enterprise Systems Group). It is important to choose the right library based on project requirements. For instance, while Gson is lightweight and easy to use, Jackson provides more advanced features for complex data binding. Understanding these differences can significantly impact performance and maintainability when converting string JSON to JSON objects in Java.
Frequently Asked Questions (FAQs)
What is the process to convert a string JSON to a JSON object in Java?
To convert a string JSON to a JSON object in Java, you can use libraries such as `org.json`, `Gson`, or `Jackson`. For example, using `org.json`, you can create a `JSONObject` by passing the string to its constructor: `JSONObject jsonObject = new JSONObject(jsonString);`.
Which libraries are commonly used for JSON handling in Java?
Common libraries for JSON handling in Java include `org.json`, `Gson` by Google, and `Jackson`. Each library offers various features for parsing, generating, and manipulating JSON data.
How do I handle exceptions when converting a string JSON to a JSON object?
When converting a string JSON to a JSON object, you should handle exceptions such as `JSONException` (for `org.json`) or `JsonParseException` (for `Gson` and `Jackson`). Use try-catch blocks to manage these exceptions effectively.
Can I convert a JSON array string to a JSON array object in Java?
Yes, you can convert a JSON array string to a JSON array object in Java. Using `org.json`, you can create a `JSONArray` by passing the string to its constructor: `JSONArray jsonArray = new JSONArray(jsonArrayString);`.
Is it possible to convert a JSON object back to a string in Java?
Yes, you can convert a JSON object back to a string in Java. For instance, using `org.json`, you can call `jsonObject.toString()` to obtain the string representation of the JSON object.
What are the performance considerations when parsing large JSON strings in Java?
When parsing large JSON strings, consider using streaming parsers like Jackson’s `JsonParser` for better performance and lower memory consumption. Additionally, ensure proper error handling and validation to avoid processing malformed JSON data.
In Java, converting a string in JSON format to a JSON object is a common task that can be accomplished using various libraries. The most popular libraries for handling JSON in Java include Jackson, Gson, and org.json. Each of these libraries provides straightforward methods to parse a JSON string and create a corresponding JSON object, enabling developers to manipulate and access the data efficiently.
One of the key takeaways is that the choice of library can significantly affect the ease of implementation and performance. For instance, Jackson is known for its high performance and ability to handle large datasets, while Gson offers a simpler API that is easier for beginners to use. The org.json library is lightweight and straightforward but may lack some advanced features found in the other two libraries.
Additionally, it is important to handle exceptions that may arise during the parsing process, such as malformed JSON strings. Proper error handling ensures that applications remain robust and can gracefully manage unexpected input. Overall, understanding how to convert a JSON string to a JSON object in Java is essential for developers working with web services, APIs, and data interchange formats.
Author Profile
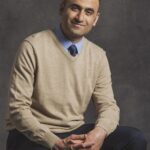
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?