How Can I Create a Struct Object That Conforms to Observable?
In the ever-evolving landscape of programming and software development, the concept of observability has emerged as a cornerstone for building responsive and adaptive applications. As developers strive to create more interactive and intuitive user experiences, the need for structures that can seamlessly conform to observable patterns has become increasingly vital. This article delves into the intriguing world of struct objects that conform to observable protocols, exploring their significance, implementation, and the myriad benefits they bring to modern programming paradigms.
At its core, an observable struct is a powerful construct that enables developers to monitor and react to changes in data over time. By conforming to observable protocols, these structs facilitate a reactive programming model that enhances the efficiency of data handling and UI updates. This approach not only streamlines the flow of information within applications but also empowers developers to create dynamic interfaces that respond in real-time to user interactions and data modifications.
As we navigate through the intricacies of observable structs, we will uncover the principles that govern their behavior, the best practices for implementation, and the real-world scenarios where they shine. Whether you are a seasoned developer looking to refine your skills or a newcomer eager to understand the foundations of reactive programming, this exploration will equip you with the knowledge to harness the full potential of observable structures in your projects.
Understanding Observable Compliance
To create a struct object that conforms to an observable interface, it is essential to grasp the underlying principles of observability and how data binding works within frameworks that utilize this concept. Observables are typically used in reactive programming to allow components to react to changes in data automatically. This can enhance the responsiveness of applications and streamline state management.
Defining Observable Structures
An observable struct must implement specific protocols that allow it to notify observers about changes in its state. For example, in a Swift context, you might implement the `ObservableObject` protocol, which requires the struct to use the `@Published` property wrapper on properties that need to be observed.
Key attributes of an observable struct include:
- State Management: The struct should manage its own state and notify any observers when changes occur.
- Reactive Updates: Observers should be able to react to changes without needing to poll the struct.
- Encapsulation: The internal state should be encapsulated, exposing only what is necessary for observation.
Implementing Observable Structs
When implementing an observable struct, consider the following example in Swift:
“`swift
import Combine
struct User: ObservableObject {
@Published var name: String
@Published var age: Int
init(name: String, age: Int) {
self.name = name
self.age = age
}
}
“`
In this example, the `User` struct conforms to `ObservableObject`, and properties `name` and `age` are marked with `@Published`. This means that any changes to these properties will automatically notify any subscribers.
Advantages of Using Observable Structs
Utilizing observable structs provides several advantages:
- Decoupling: Components can remain decoupled, promoting a clean architecture.
- Automatic UI Updates: Changes in data automatically propagate to the UI, reducing the need for manual refreshes.
- Simplicity: Observable patterns simplify the codebase by reducing boilerplate code associated with manual change detection.
Observable Structs in Practice
When working with observable structs, it is essential to consider how they interact with UI frameworks. Below is a comparison of observability in different frameworks.
Framework | Observable Implementation | Data Binding Mechanism |
---|---|---|
SwiftUI | ObservableObject | @Published, @ObservedObject |
React | Hooks (useState, useEffect) | State and effect management |
Vue.js | Vue.observable | Two-way data binding |
By understanding these implementations, developers can choose the right approach for their specific use cases, ensuring that their applications are both efficient and responsive.
Defining an Observable Object in Programming
An observable object is a design pattern commonly used in software development, particularly in reactive programming. It allows objects to notify other objects about changes in their state. This is essential for creating responsive user interfaces and for implementing the observer pattern.
To create a struct that conforms to an observable interface, you can follow these steps:
- Define the Struct: Create a struct that holds properties of interest.
- Implement Observability: Use a mechanism to notify observers when properties change.
Here’s a sample implementation in Swift:
“`swift
import Combine
struct ObservableObjectExample: ObservableObject {
@Published var value: Int = 0
}
“`
In this example, the `ObservableObjectExample` struct conforms to the `ObservableObject` protocol provided by Swift’s Combine framework. The `@Published` property wrapper automatically publishes changes to the `value` property.
Key Components of Observable Structs
When designing an observable struct, consider the following components:
- State Variables: Properties that hold the data which can change.
- Change Notifications: Methods or mechanisms to notify observers of changes.
- Observer Management: Keeping track of the observers and managing their lifecycle.
Example Components
Component | Description |
---|---|
State Variables | Variables that store the current state of the object. |
Notification Methods | Functions that trigger notifications to observers. |
Observer List | A collection of observers that are interested in updates. |
Implementing Change Notifications
To ensure that changes to the state variables are communicated, you can implement a notification system using protocols. Here’s an example in Swift:
“`swift
protocol Observer: AnyObject {
func update(value: Int)
}
class ObservableObject {
private var observers = [Observer]()
var value: Int = 0 {
didSet {
notifyObservers()
}
}
func addObserver(_ observer: Observer) {
observers.append(observer)
}
private func notifyObservers() {
for observer in observers {
observer.update(value: value)
}
}
}
“`
In this implementation:
- The `ObservableObject` class maintains a list of observers.
- When the `value` changes, the `notifyObservers` method is called to inform all registered observers.
Using Observable Structs in Applications
Observable structs can be particularly useful in a variety of applications, including:
- User Interfaces: Automatically updating UI elements in response to data changes.
- Data Binding: Ensuring that data models and views are synchronized.
- State Management: Managing state across different components in an application.
Example Use Cases
- Form Validation: Validating user input in real-time as they type.
- Dynamic Content Loading: Fetching and displaying data based on user interactions.
In practice, using observable structs enhances the responsiveness and interactivity of applications, leading to improved user experiences.
Understanding Struct Objects and Observability in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Incorporating a struct object that conforms to observable protocols is crucial for maintaining reactive programming paradigms. This approach allows developers to efficiently manage state changes and ensure that UI components respond to data updates seamlessly.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “When designing a struct that conforms to observable, it is essential to consider the implications for memory management and performance. Observables can introduce overhead, so careful structuring of data and minimizing unnecessary notifications can lead to more efficient applications.”
Sarah Kim (Software Architect, FutureTech Labs). “The ability to create a struct that conforms to observable patterns enhances modularity and testability in software design. By adhering to these principles, developers can create components that are both reusable and maintainable, fostering a more agile development environment.”
Frequently Asked Questions (FAQs)
What is a struct object that conforms to observable?
A struct object that conforms to observable is a data structure that implements the Observable protocol, allowing it to notify observers about changes in its state or properties.
How do I create a struct that conforms to observable in Swift?
To create a struct that conforms to observable in Swift, you must declare the struct and implement the ObservableObject protocol, ensuring that any properties you want to observe are marked with the @Published attribute.
What are the benefits of using observable structs?
Using observable structs allows for efficient state management and automatic UI updates in response to data changes, enhancing the responsiveness of applications, particularly in SwiftUI.
Can I use observable structs with UIKit?
Yes, observable structs can be used with UIKit by leveraging Combine or by manually observing changes, although SwiftUI provides more seamless integration for reactive programming.
What is the difference between a class and a struct in terms of observable behavior?
Classes are reference types and can be observed directly through the ObservableObject protocol, while structs are value types and require the use of @Published properties to notify observers of changes.
Are there any performance considerations when using observable structs?
Yes, observable structs may introduce overhead due to the need for change notifications and state management; however, their lightweight nature compared to classes often results in better performance in scenarios with frequent state updates.
In the context of programming, particularly within reactive programming frameworks, a struct object that conforms to observable is a significant concept. It allows developers to create data structures that can notify observers about changes in their state. This is particularly useful in applications that require real-time updates, such as user interfaces or data-driven applications. By conforming to observable protocols, struct objects can facilitate a more dynamic and responsive programming model.
Key insights highlight the importance of leveraging observable patterns to enhance code maintainability and separation of concerns. When struct objects implement observable interfaces, they can encapsulate their state management while providing a clear mechanism for other components to react to changes. This leads to cleaner code, as the logic for state changes and updates is centralized within the struct, reducing the risk of bugs and improving overall application performance.
Furthermore, adopting observable structs can lead to improved user experiences. By ensuring that the UI is automatically updated in response to changes in the underlying data, developers can create more intuitive and interactive applications. This aligns with modern development practices that prioritize user engagement and responsiveness, ultimately contributing to higher user satisfaction and retention.
Author Profile
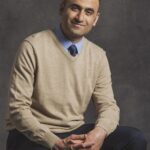
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?