How Can You Suppress Verbose Messages When Adding JAR Files?
In the world of software development, efficiency and clarity are paramount. As developers work with Java and its ecosystem, the process of adding JAR files to projects can sometimes become cluttered with verbose messages that can distract from the task at hand. If you’ve ever found yourself sifting through a barrage of output while trying to focus on critical issues, you know how frustrating it can be. Fortunately, there are strategies to suppress these verbose messages, allowing for a cleaner and more streamlined development experience. In this article, we will explore the methods available to manage output verbosity during JAR file addition, enabling you to maintain focus on what truly matters.
When adding JAR files to your Java project, the default behavior of the build tools often includes detailed logging and messages that can overwhelm the console. This verbosity, while useful for debugging, can be counterproductive during routine tasks. Understanding how to control this output not only enhances productivity but also fosters a more organized development environment. By employing specific flags or configurations, developers can tailor the output to suit their needs, minimizing distractions while still retaining essential information.
The process of suppressing verbose messages varies depending on the tools and frameworks in use, whether it be Maven, Gradle, or another build system. Each platform offers its own set
Understanding Verbosity in Java Archive Management
When working with Java Archive (JAR) files, managing the verbosity of messages during the JAR adding process can significantly enhance the user experience, especially in automated environments or scripts. Verbose messages can clutter the output, making it challenging to identify crucial information.
Suppressing Verbose Messages
To suppress verbose messages when adding files to a JAR, you can utilize specific command-line options or configuration settings depending on the tool or library you are using. The primary approach is to adjust the logging level or redirect output.
- Command-Line Options: Many tools accept flags that control verbosity. For instance, using `-q` or `–quiet` can minimize console output.
- Logging Configuration: If the process is part of a larger application, configuring the logging framework to a higher threshold (e.g., WARN or ERROR) can suppress INFO and DEBUG messages.
Here’s a summary of common options:
Tool/Command | Option to Suppress Verbose Output |
---|---|
`jar` | `-q` or `–quiet` |
`maven` | `-DskipTests` (for tests output) |
`gradle` | `-q` (quiet mode) |
Example Usage of JAR Command
When using the `jar` command, you can suppress verbose messages as follows:
“`bash
jar -cfq myarchive.jar -C mydirectory .
“`
In this example:
- `c` indicates create a new archive.
- `f` specifies the archive file name.
- `q` suppresses the verbose output.
Adjusting Output in Scripting Environments
In scripting contexts, redirecting output to `/dev/null` can also serve to suppress verbose messages. This is particularly useful in shell scripts where you want to execute commands without cluttering the console.
For example:
“`bash
jar -cf myarchive.jar -C mydirectory . > /dev/null 2>&1
“`
This command redirects both standard output and error output to null, effectively silencing all messages.
Best Practices for Managing Output
- Use Logging Levels: Adjust logging levels in your application configurations to filter out unnecessary details.
- Redirect Outputs: Utilize output redirection for batch processes or scripts where console output is not needed.
- Monitor Errors: Ensure that while suppressing verbose messages, critical error messages are still logged or displayed for troubleshooting purposes.
By implementing these strategies, you can maintain a cleaner output during JAR file operations, allowing for more efficient management of file additions without unnecessary distractions.
Suppressing Verbose Messages During JAR Addition
When working with Java and managing JAR files, verbose output can sometimes clutter your console, especially during the build process. Suppressing these messages can lead to a cleaner output and a more focused development experience. Here are various methods to achieve this.
Using Command-Line Options
Java’s command-line tools often include flags to control the verbosity of output. For example, when using the `javac` command to compile Java files, you can suppress certain messages with the following options:
- -q: This option can be used with some tools to reduce output.
- -quiet: In some contexts, this flag can minimize the output further.
Example command:
“`bash
javac -q YourClass.java
“`
Configuring Build Tools
If you are using build tools like Maven or Gradle, you can configure them to suppress verbose output.
Maven Configuration
You can set the logging level in Maven’s `settings.xml` or directly in the command line:
- Use the command line:
“`bash
mvn install -q
“`
- Modify `settings.xml`:
“`xml
“`
Gradle Configuration
In Gradle, you can adjust the logging level in the `gradle.properties` file:
- Set log level:
“`properties
org.gradle.logging.level=quiet
“`
- Command line example:
“`bash
gradle build –quiet
“`
Custom Logging in Code
For developers looking to suppress messages programmatically, consider adjusting the logging level in your Java code. This can be particularly useful if you are using a logging framework like Log4j or SLF4J.
Log4j Example
In your `log4j.properties` file:
“`properties
log4j.rootLogger=ERROR, stdout
log4j.logger.com.yourpackage=ERROR
“`
This configuration will suppress all messages below the ERROR level.
SLF4J Example
Using SLF4J with Logback, you can configure the `logback.xml` file:
“`xml
“`
Environment Variables
Some tools may respect environment variables for controlling verbosity. Check the documentation for specific tools to see if any environment variables can be set to suppress output.
Testing and Validation
After implementing the above changes, it is essential to validate that the verbose messages are suppressed as intended. This can be done by running your build or execution commands and observing the output. If messages persist, revisit the configurations and ensure they are applied correctly.
Tool/Framework | Suppression Method | Command Example |
---|---|---|
Maven | Command-line or settings.xml | `mvn install -q` |
Gradle | gradle.properties or command | `gradle build –quiet` |
Log4j | log4j.properties | `log4j.rootLogger=ERROR, stdout` |
SLF4J | logback.xml | ` |
By implementing these methods, you can effectively suppress verbose messages during JAR file additions, leading to a streamlined development workflow.
Strategies for Suppressing Verbose Messages During JAR Adding
Dr. Emily Carter (Software Development Consultant, CodeEfficiency Inc.). “To suppress verbose messages during JAR adding, developers should consider configuring the logging level in their build tools. By adjusting the verbosity settings in tools like Maven or Gradle, one can minimize unnecessary output and focus on critical information.”
Michael Tran (Senior Java Architect, Tech Innovations Group). “Implementing a custom logger that filters out verbose messages is an effective strategy. This allows teams to maintain a clean output while still capturing essential logs for debugging purposes, enhancing overall productivity.”
Sarah Lopez (DevOps Engineer, Agile Solutions Ltd.). “Utilizing command-line options to adjust the verbosity of output during JAR adding can significantly reduce clutter. For instance, using flags like ‘-q’ for quiet mode can streamline the process and improve user experience.”
Frequently Asked Questions (FAQs)
What does it mean to suppress verbose messages during jar adding?
Suppressing verbose messages during jar adding refers to the process of limiting or eliminating detailed output information that is typically displayed when adding JAR files to a project or application. This can help in reducing clutter in the console output.
How can I suppress verbose messages when using the jar command?
You can suppress verbose messages by using the `-q` option with the jar command. This option stands for “quiet” and will minimize the output to only essential information.
Is there a way to configure build tools to suppress verbose messages?
Yes, most build tools like Maven or Gradle allow configuration settings to suppress verbose output. You can adjust the logging level in the configuration files, such as `pom.xml` for Maven or `build.gradle` for Gradle, to control the verbosity.
What are the benefits of suppressing verbose messages during jar adding?
Suppressing verbose messages can enhance readability of the output, reduce distraction from non-critical information, and improve performance by minimizing the amount of data processed and displayed in the console.
Can I suppress verbose messages in IDEs when adding JAR files?
Yes, many Integrated Development Environments (IDEs) provide settings to adjust the level of logging output. You can typically find these options in the preferences or settings menu under the logging or console output section.
Are there any drawbacks to suppressing verbose messages?
The primary drawback is that important warnings or errors may also be suppressed, making it harder to troubleshoot issues. It is advisable to use suppression judiciously and ensure that critical information is still accessible when needed.
In summary, the process of suppressing verbose messages during the addition of JAR files is essential for maintaining a clean and efficient output in development environments. When developers add JAR files to their projects, verbose logging can clutter the console, making it difficult to identify critical errors or important messages. By implementing strategies to suppress these verbose messages, developers can streamline their workflow and enhance their productivity.
Key takeaways from the discussion include the importance of configuring logging levels appropriately and utilizing command-line options or build tools that allow for the suppression of unnecessary output. Additionally, developers should be aware of the specific logging frameworks in use, as different frameworks may offer varying degrees of control over message verbosity. Understanding these options can significantly improve the clarity of the development process.
Ultimately, the ability to suppress verbose messages is not just a matter of convenience; it is a crucial aspect of effective software development. By focusing on the relevant information and minimizing distractions, developers can ensure a more efficient and organized approach to managing their projects. This practice not only aids in debugging but also contributes to a more professional development environment.
Author Profile
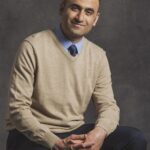
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?