How Can You Create a List from a Struct in Swift for iOS Development?
In the world of iOS development, Swift has emerged as a powerful and intuitive programming language that empowers developers to create dynamic and engaging applications. One common task that developers encounter is the need to display data in a structured format, particularly when working with custom data types. This is where the concept of creating a list from a struct becomes essential. Whether you’re building a simple app or a complex user interface, understanding how to efficiently manage and present data through lists can significantly enhance user experience and application performance.
When working with structs in Swift, developers can define custom data types that encapsulate related properties and behaviors. This approach not only promotes code reusability but also makes it easier to manage data. By leveraging Swift’s powerful features, such as type safety and optionals, you can create robust data models that seamlessly integrate with your application’s user interface. The ability to transform these structs into lists allows for a more organized presentation of information, making it easier for users to interact with the data.
In this article, we will explore the various techniques for creating lists from structs in Swift, focusing on best practices and common patterns that can streamline your development process. From utilizing SwiftUI to leveraging UIKit, we will delve into the methods that can help you display your data effectively. Whether you’re
Creating a List from a Struct in Swift
In Swift, you can create a list from a struct by defining a struct and then populating an array with instances of that struct. This approach allows you to manage collections of related data effectively.
To illustrate, consider the following example where we define a struct called `Person`:
“`swift
struct Person {
var name: String
var age: Int
}
“`
Once you have your struct defined, you can create an array of `Person` instances:
“`swift
var people: [Person] = [
Person(name: “Alice”, age: 30),
Person(name: “Bob”, age: 25),
Person(name: “Charlie”, age: 28)
]
“`
This array `people` can now be used to access and manipulate a list of `Person` objects.
Accessing and Displaying Data
You can access elements in the array using standard array indexing. To display the list of people, you might use a loop:
“`swift
for person in people {
print(“\(person.name) is \(person.age) years old.”)
}
“`
This loop will iterate over each `Person` in the `people` array and print their name and age.
Using Codable for JSON Representation
If you need to serialize your list of structs to JSON, you can make your struct conform to the `Codable` protocol. This allows easy encoding and decoding.
“`swift
struct Person: Codable {
var name: String
var age: Int
}
“`
You can then convert your list to JSON data as follows:
“`swift
let encoder = JSONEncoder()
if let jsonData = try? encoder.encode(people) {
if let jsonString = String(data: jsonData, encoding: .utf8) {
print(jsonString)
}
}
“`
This will output a JSON string representation of the `people` array.
Filtering the List
To filter the list based on specific criteria, you can use the `filter` method. For example, to find all people older than 26:
“`swift
let filteredPeople = people.filter { $0.age > 26 }
“`
You can then display the filtered results using the same loop as before.
Example Table of Struct Data
Below is a table representation of the `Person` struct data:
Name | Age |
---|---|
Alice | 30 |
Bob | 25 |
Charlie | 28 |
This table format can be useful for displaying structured data in a user interface or in documentation.
By structuring your data using Swift structs and leveraging arrays, you can efficiently manage and manipulate collections of related data. The use of `Codable` makes it easy to encode your data for storage or transmission, while filtering allows you to quickly access subsets of your data as needed.
Creating a List from a Struct in Swift
In Swift, you can easily create a list from a struct by defining a struct type and then instantiating an array that contains elements of that struct. This approach is useful for managing collections of related data efficiently.
Defining the Struct
To begin, define a struct that encapsulates the data you want to manage. For example, consider a `Book` struct that includes properties like title, author, and year published.
“`swift
struct Book {
var title: String
var author: String
var yearPublished: Int
}
“`
Creating an Array of Structs
Once the struct is defined, you can create an array of `Book` instances. Here’s how to instantiate and populate the array:
“`swift
var books: [Book] = [
Book(title: “1984”, author: “George Orwell”, yearPublished: 1949),
Book(title: “To Kill a Mockingbird”, author: “Harper Lee”, yearPublished: 1960),
Book(title: “The Great Gatsby”, author: “F. Scott Fitzgerald”, yearPublished: 1925)
]
“`
Accessing and Modifying the List
You can access and modify the list of structs using standard array methods.
- Accessing elements:
“`swift
let firstBook = books[0] // Access the first book
print(firstBook.title) // Output: 1984
“`
- Modifying elements:
“`swift
books[1].yearPublished = 1961 // Update the year of “To Kill a Mockingbird”
“`
- Adding new elements:
“`swift
books.append(Book(title: “Brave New World”, author: “Aldous Huxley”, yearPublished: 1932))
“`
Iterating Through the List
To perform operations on each element of the list, you can use a loop. For instance, you can print out the details of each book:
“`swift
for book in books {
print(“\(book.title) by \(book.author), published in \(book.yearPublished)”)
}
“`
Filtering the List
You might want to filter the list based on specific criteria, such as finding books published after a certain year. This can be accomplished using the `filter` method:
“`swift
let recentBooks = books.filter { $0.yearPublished > 1950 }
“`
This code snippet would yield books published after 1950.
Using Maps to Transform Data
If you need to create a list of titles from your `books` array, you can utilize the `map` function:
“`swift
let titles = books.map { $0.title }
“`
This will produce an array containing only the titles of the books.
Example: Complete Implementation
Here is a complete example that combines all the elements discussed:
“`swift
struct Book {
var title: String
var author: String
var yearPublished: Int
}
var books: [Book] = [
Book(title: “1984”, author: “George Orwell”, yearPublished: 1949),
Book(title: “To Kill a Mockingbird”, author: “Harper Lee”, yearPublished: 1960),
Book(title: “The Great Gatsby”, author: “F. Scott Fitzgerald”, yearPublished: 1925)
]
for book in books {
print(“\(book.title) by \(book.author), published in \(book.yearPublished)”)
}
let recentBooks = books.filter { $0.yearPublished > 1950 }
let titles = books.map { $0.title }
“`
This example provides a robust understanding of how to manage a list of structs in Swift, allowing for effective data handling and manipulation.
Expert Insights on Creating Lists from Structs in Swift for iOS Development
Emily Chen (Senior iOS Developer, Tech Innovations Inc.). “When working with structs in Swift, utilizing the `map` function is an efficient way to create a list from an array of structs. This approach not only enhances code readability but also leverages Swift’s functional programming capabilities, making your code more concise and expressive.”
James Patel (Lead Software Engineer, Mobile Solutions Group). “To generate a list from a struct in Swift, it’s crucial to ensure that your struct conforms to the `Identifiable` protocol if you’re planning to use it in a SwiftUI List. This allows for better performance and smoother updates when the data changes, which is essential for creating a responsive user interface.”
Linda Garcia (Swift Programming Instructor, Code Academy). “A common best practice when creating lists from structs is to encapsulate the logic within a dedicated function. This not only promotes code reusability but also adheres to the single responsibility principle, making your codebase easier to maintain and test over time.”
Frequently Asked Questions (FAQs)
How can I create a list from a struct in Swift?
You can create a list from a struct in Swift by defining an array of your struct type. For example, if you have a struct named `Person`, you can create a list like this: `var people: [Person] = []`.
What is the best way to populate a list from a struct in Swift?
To populate a list from a struct, you can initialize instances of the struct and append them to the array. For instance:
“`swift
var people: [Person] = []
let person1 = Person(name: “Alice”, age: 30)
people.append(person1)
“`
Can I use Codable structs to create a list in Swift?
Yes, you can use Codable structs to create a list in Swift. By conforming your struct to the Codable protocol, you can easily decode JSON data into an array of your struct type.
How do I iterate over a list of structs in Swift?
You can iterate over a list of structs using a for-in loop. For example:
“`swift
for person in people {
print(person.name)
}
“`
Is it possible to filter a list of structs in Swift?
Yes, you can filter a list of structs using the `filter` method. For example, to filter people by age:
“`swift
let adults = people.filter { $0.age >= 18 }
“`
How can I sort a list of structs in Swift?
You can sort a list of structs using the `sorted` method. For example, to sort people by name:
“`swift
let sortedPeople = people.sorted { $0.name < $1.name }
```
In Swift for iOS development, creating a list from a struct is a fundamental practice that enhances data management and user interface design. Structs in Swift are value types that allow developers to define custom data models, which can then be utilized to populate lists or collections. By leveraging Swift's powerful features, such as generics and protocols, developers can efficiently manage and display structured data in their applications.
One of the key advantages of using structs to create lists is the clarity and organization they bring to the codebase. Structs enable developers to encapsulate related properties and methods, promoting better code readability and maintainability. Furthermore, Swift's type safety ensures that the data used within these lists is consistent and reliable, reducing the likelihood of runtime errors.
Additionally, Swift provides various tools and frameworks, such as SwiftUI and UIKit, that facilitate the creation of dynamic lists from structs. Developers can easily bind data to UI components, allowing for seamless updates and interactions. This integration not only enhances user experience but also streamlines the development process, making it easier to implement complex functionalities with minimal effort.
utilizing structs to create lists in Swift for iOS development is a best practice that promotes organized code, enhances data integrity
Author Profile
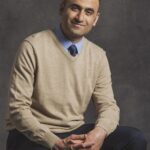
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?