How Can I Swiftly Pass a Struct to a Function in Swift?
In the world of Swift programming, structuring your data efficiently is crucial for building robust applications. One common task that developers encounter is the need to pass structs to functions. Whether you’re working on a small project or a large-scale application, understanding how to effectively manipulate and transfer data using structs can significantly enhance your coding experience. This article will delve into the intricacies of passing structs to functions in Swift, providing you with the knowledge to streamline your code and improve performance.
At its core, a struct in Swift is a powerful tool for encapsulating related data and behaviors. When you pass a struct to a function, you are essentially allowing that function to operate on a specific set of data, which can lead to cleaner and more maintainable code. However, Swift’s unique handling of value types, such as structs, means that you need to be mindful of how data is copied and modified during this process. This can impact performance and memory usage, making it essential to grasp the nuances of passing structs effectively.
Throughout this article, we will explore the various methods of passing structs to functions, including the implications of using inout parameters and the benefits of immutability. By the end, you will have a comprehensive understanding of how to leverage structs in your Swift functions, empowering you to write
Understanding Structs in Swift
In Swift, structs are value types that encapsulate related data and behaviors. They are commonly used to model simple data structures. Structs can contain properties, methods, initializers, and can even conform to protocols. When passing structs to functions, it is essential to understand how value types behave in Swift.
When you pass a struct to a function, a copy of the struct is made. This is because structs are passed by value, meaning the function receives a duplicate of the original instance. Any modifications made to the struct within the function do not affect the original instance outside the function.
Passing Structs to Functions
To pass a struct to a function, you simply declare the struct type in the function’s parameter list. Here is an example:
“`swift
struct User {
var name: String
var age: Int
}
func printUserInfo(user: User) {
print(“Name: \(user.name), Age: \(user.age)”)
}
let user1 = User(name: “Alice”, age: 30)
printUserInfo(user: user1)
“`
In this example, `user1` is passed to the `printUserInfo` function. The function prints the user’s name and age without modifying `user1`.
Modifying Structs Inside Functions
If you need to modify the struct within the function, you can create a mutable copy of the struct. However, any changes will still be limited to that copy. Here is an illustration:
“`swift
func updateUserAge(user: inout User, newAge: Int) {
user.age = newAge
}
var user2 = User(name: “Bob”, age: 25)
updateUserAge(user: &user2, newAge: 26)
printUserInfo(user: user2) // Outputs: Name: Bob, Age: 26
“`
In this case, the `updateUserAge` function uses the `inout` keyword, which allows the function to modify the original `user2` instance. The `&` symbol is required to indicate that we are passing a reference to the variable.
Benefits and Considerations
When passing structs to functions, consider the following benefits and considerations:
- Benefits:
- Encapsulation of related data and functions.
- Safety in concurrent environments due to value semantics.
- Simplified memory management since structs are stack-allocated.
- Considerations:
- Performance overhead due to copying large structs.
- Need to use `inout` for modifications, which can introduce complexity.
Here’s a comparison table summarizing the characteristics of structs and classes in Swift:
Feature | Structs | Classes |
---|---|---|
Type | Value Type | Reference Type |
Memory Allocation | Stack | Heap |
Inheritance | No | Yes |
Mutability | Immutable by default | Mutable |
By understanding these aspects, developers can effectively manage how structs are passed to functions and leverage their benefits in Swift programming.
Passing Structs to Functions in Swift
In Swift, passing a struct to a function can be done by value or reference depending on the context. Structs are value types, which means that when you pass a struct to a function, a copy of the original struct is created. This behavior is crucial to understand when working with functions that manipulate data.
Defining a Struct
Before passing a struct to a function, you must define it. Here’s a simple example of defining a struct:
“`swift
struct Person {
var name: String
var age: Int
}
“`
In this example, `Person` is a struct with two properties: `name` and `age`.
Passing a Struct to a Function
To pass a struct to a function, you define the function with a parameter of the struct type. Below is an example of a function that accepts a `Person` struct:
“`swift
func greet(person: Person) {
print(“Hello, \(person.name)! You are \(person.age) years old.”)
}
“`
In this function, `greet` takes a `Person` instance as an argument and prints a greeting message.
Calling the Function
You can call the function by creating an instance of the struct and passing it as follows:
“`swift
let john = Person(name: “John”, age: 30)
greet(person: john)
“`
This will output: `Hello, John! You are 30 years old.`
Modifying Structs Inside Functions
Since structs are value types, any modifications made to the struct inside the function will not affect the original instance. Here’s an example:
“`swift
func celebrateBirthday(person: Person) {
var updatedPerson = person
updatedPerson.age += 1
print(“Happy Birthday, \(updatedPerson.name)! You are now \(updatedPerson.age) years old.”)
}
“`
If you call this function:
“`swift
celebrateBirthday(person: john)
“`
The output will be: `Happy Birthday, John! You are now 31 years old.` However, the original `john` instance remains unchanged.
Using In-Out Parameters
If you need to modify a struct and have those changes reflect outside the function, you can use in-out parameters. Here’s how:
“`swift
func haveBirthday(person: inout Person) {
person.age += 1
}
“`
You must use the `&` operator when calling the function to indicate that you are passing a reference:
“`swift
var jane = Person(name: “Jane”, age: 28)
haveBirthday(person: &jane)
print(jane.age) // Output: 29
“`
Comparison: Structs vs. Classes
Structs and classes are both used to create custom data types, but they exhibit different behaviors:
Feature | Structs | Classes |
---|---|---|
Type | Value type | Reference type |
Memory Management | Stack allocation | Heap allocation |
Inheritance | No | Yes |
Mutability | Immutable unless marked as `var` | Mutable by default |
Understanding these differences is vital for selecting the appropriate type for your needs in Swift development.
Expert Insights on Passing Structs in Swift Functions
Dr. Emily Carter (Senior Software Engineer, Swift Innovations Inc.). In Swift, passing a struct to a function is straightforward due to the language’s value semantics. When you pass a struct, you are passing a copy of the data, which ensures that the original struct remains unchanged. This behavior is particularly beneficial for maintaining data integrity in concurrent programming scenarios.
Michael Tran (iOS Development Specialist, TechForward). It is essential to understand that when you pass a struct to a function, you can also use inout parameters if you need to modify the struct within the function. This allows for efficient memory usage while still enabling changes to the struct’s properties without creating a new instance.
Lisa Huang (Swift Language Advocate, CodeCraft Magazine). One of the key advantages of using structs in Swift is their ability to encapsulate related data and functionality. When passing structs to functions, developers should leverage Swift’s powerful type system to create clear and maintainable code, which enhances readability and reduces the likelihood of errors in larger codebases.
Frequently Asked Questions (FAQs)
How do I define a struct in Swift?
To define a struct in Swift, use the `struct` keyword followed by the name of the struct and a set of curly braces containing its properties and methods. For example:
“`swift
struct Person {
var name: String
var age: Int
}
“`
What is the syntax to pass a struct to a function in Swift?
To pass a struct to a function, define the function with a parameter of the struct type. For example:
“`swift
func greet(person: Person) {
print(“Hello, \(person.name)”)
}
“`
Can I modify a struct inside a function in Swift?
Structs are value types in Swift, meaning they are copied when passed to a function. To modify a struct inside a function, you must declare the parameter as `inout`, allowing you to change its properties directly. For example:
“`swift
func updateAge(person: inout Person, newAge: Int) {
person.age = newAge
}
“`
What happens if I pass a struct to a function without using `inout`?
If you pass a struct to a function without using `inout`, the function receives a copy of the struct. Any modifications made to the struct inside the function will not affect the original instance outside the function.
Can I return a struct from a function in Swift?
Yes, you can return a struct from a function in Swift. Simply specify the struct type as the return type of the function. For example:
“`swift
func createPerson(name: String, age: Int) -> Person {
return Person(name: name, age: age)
}
“`
Are there any performance considerations when passing large structs to functions?
Yes, passing large structs can lead to performance overhead due to copying. In such cases, consider using classes (reference types) or passing the struct as an `inout` parameter to avoid unnecessary copies.
In Swift, passing a struct to a function is a common practice that leverages the language’s value type semantics. Structs in Swift are defined as value types, meaning that when they are passed to a function, a copy of the original instance is created. This behavior is crucial to understand, as it impacts how data is manipulated and maintained within different scopes of a program. When a struct is passed to a function, any modifications made to the struct within that function do not affect the original instance outside of the function.
Moreover, Swift provides a straightforward syntax for passing structs to functions, which can include parameters of various types, including other structs, arrays, or dictionaries. This flexibility allows developers to create more modular and reusable code. It is also important to note that Swift’s use of structs promotes immutability and encourages safer programming practices, as developers can work with copies of data rather than references, reducing the risk of unintended side effects.
understanding how to pass structs to functions in Swift is essential for effective programming in the language. By recognizing the implications of value types and the mechanics of function parameters, developers can write cleaner, more efficient code. This knowledge not only enhances code maintainability but also fosters a deeper understanding
Author Profile
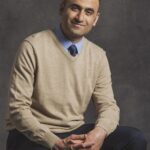
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?