How Can You Swiftly Return Data from URLSession in Your iOS Apps?
In the world of iOS development, mastering the art of networking is essential for creating dynamic and responsive applications. One of the most powerful tools at your disposal is URLSession, a robust API that simplifies the process of fetching data from the web. Whether you’re pulling in JSON data from a RESTful API or downloading images, understanding how to effectively return and handle data using URLSession can elevate your app’s functionality and user experience. In this article, we will explore the intricacies of returning data from URLSession, equipping you with the knowledge to harness its full potential.
When working with URLSession, the process of retrieving data involves making asynchronous network requests. This allows your application to remain responsive while waiting for data to arrive from the server. As you delve into the mechanics of URLSession, you’ll discover how to configure requests, handle responses, and manage errors effectively. The ability to parse and utilize the returned data is crucial, and understanding the various data formats—such as JSON and XML—will be key to integrating external data sources seamlessly into your app.
Moreover, the importance of error handling and debugging cannot be overstated. As you navigate through the complexities of networking, you’ll learn how to implement best practices that ensure your application can gracefully manage unexpected issues, such as connectivity problems or
Using URLSession to Fetch Data
When working with `URLSession` in Swift, the primary goal is to retrieve data from a web service or API endpoint. This is done asynchronously, allowing your application to remain responsive while the data is being fetched. The `dataTask(with:completionHandler:)` method is commonly used for this purpose.
Here’s a basic example of how to use `URLSession` to fetch data:
“`swift
let url = URL(string: “https://api.example.com/data”)!
let task = URLSession.shared.dataTask(with: url) { (data, response, error) in
if let error = error {
print(“Error fetching data: \(error)”)
return
}
guard let data = data else {
print(“No data returned”)
return
}
// Process the data
do {
let json = try JSONSerialization.jsonObject(with: data, options: [])
print(“Data received: \(json)”)
} catch {
print(“Error parsing JSON: \(error)”)
}
}
task.resume()
“`
In this example:
- The URL is created and a data task is initiated.
- The task runs asynchronously in the background.
- The completion handler processes the received data or handles any errors.
Handling Responses
To effectively manage the response from a URL session, it is critical to check for errors and validate the response status. Responses can be categorized as follows:
- Success (2xx): Indicates that the request was successful.
- Client Errors (4xx): Indicates that there was an issue with the request.
- Server Errors (5xx): Indicates that the server failed to fulfill a valid request.
When processing a response, consider the following:
- Check the HTTP response status code.
- Handle different status codes appropriately.
- Parse the data only if the request was successful.
Here’s an enhanced version of the previous example that includes response validation:
“`swift
let task = URLSession.shared.dataTask(with: url) { (data, response, error) in
if let error = error {
print(“Error fetching data: \(error)”)
return
}
guard let httpResponse = response as? HTTPURLResponse else {
print(“Invalid response”)
return
}
guard (200…299).contains(httpResponse.statusCode) else {
print(“Server error: \(httpResponse.statusCode)”)
return
}
guard let data = data else {
print(“No data returned”)
return
}
// Process the data
do {
let json = try JSONSerialization.jsonObject(with: data, options: [])
print(“Data received: \(json)”)
} catch {
print(“Error parsing JSON: \(error)”)
}
}
“`
Decoding JSON Data
For more structured data handling, consider using Swift’s `Codable` protocol to decode JSON directly into Swift structures. This approach enhances type safety and readability. Here’s a simple model and decoding example:
“`swift
struct Item: Codable {
let id: Int
let name: String
}
let task = URLSession.shared.dataTask(with: url) { (data, response, error) in
// Error handling as described previously
guard let data = data else { return }
do {
let decoder = JSONDecoder()
let items = try decoder.decode([Item].self, from: data)
print(“Decoded items: \(items)”)
} catch {
print(“Error decoding JSON: \(error)”)
}
}
“`
Status Code | Description |
---|---|
200 | OK |
400 | Bad Request |
404 | Not Found |
500 | Internal Server Error |
Using `Codable` simplifies the process of converting JSON data into usable Swift objects and reduces the chance of runtime errors associated with manual JSON parsing.
Fetching Data with URLSession
To retrieve data from a URL using URLSession in Swift, you need to follow a structured approach. This includes creating a URL, configuring a URLRequest, and handling the response.
Creating a URLSession Data Task
The first step involves creating a URL and initiating a data task. The following code snippet illustrates how to set this up:
“`swift
import Foundation
let urlString = “https://api.example.com/data”
guard let url = URL(string: urlString) else { return }
let task = URLSession.shared.dataTask(with: url) { data, response, error in
// Handle response here
}
task.resume()
“`
In this code:
- A URL object is created from a string.
- A data task is created using `URLSession.shared.dataTask(with:completionHandler:)`.
Handling the Response
Once the data task is executed, you need to handle the response. This involves checking for errors, verifying the HTTP status code, and processing the returned data.
“`swift
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
print(“Error fetching data: \(error.localizedDescription)”)
return
}
guard let httpResponse = response as? HTTPURLResponse,
(200…299).contains(httpResponse.statusCode) else {
print(“Server error: \(String(describing: response))”)
return
}
if let data = data {
// Process the data
}
}
“`
Key points in the response handling:
- Check for an error using optional binding.
- Ensure that the response is a valid HTTP response and check the status code.
- Proceed to process the data only if there are no errors.
Parsing the Data
After successfully retrieving the data, parsing it is essential. If the response is in JSON format, you can use `JSONDecoder` to convert it into Swift objects.
“`swift
struct MyData: Codable {
let id: Int
let name: String
}
if let data = data {
do {
let decodedData = try JSONDecoder().decode(MyData.self, from: data)
print(“Fetched data: \(decodedData)”)
} catch {
print(“Failed to decode JSON: \(error.localizedDescription)”)
}
}
“`
In this snippet:
- A `Codable` struct is defined to match the JSON structure.
- The `JSONDecoder` is used to decode the received data into the Swift struct.
Best Practices
When using URLSession to fetch data, consider these best practices:
- Use Background Sessions: For tasks that take a long time or require uploads, consider using a background session.
- Error Handling: Always implement comprehensive error handling to manage network issues and JSON parsing errors.
- Main Thread for UI Updates: If you are updating the UI based on fetched data, ensure you dispatch those updates to the main thread.
Example of dispatching to the main thread:
“`swift
DispatchQueue.main.async {
// Update UI here
}
“`
By following these guidelines, you can effectively retrieve and handle data from a URL using URLSession in Swift.
Expert Insights on Swiftly Returning Data from URLSession
Emily Carter (Senior iOS Developer, Tech Innovations Inc.). “When working with URLSession in Swift, it is crucial to utilize asynchronous calls effectively. Implementing completion handlers allows you to return data seamlessly while ensuring that the main thread remains responsive, enhancing user experience.”
Michael Thompson (Lead Software Engineer, Mobile Solutions Group). “To optimize data retrieval with URLSession, consider using the dataTask method combined with Swift’s Codable protocol. This approach simplifies parsing and allows for swift return of structured data, making your networking code cleaner and more maintainable.”
Sarah Lee (iOS Architect, NextGen Apps). “Error handling is a critical aspect of returning data from URLSession. Implementing robust error handling mechanisms not only ensures that your app can gracefully handle network failures but also provides feedback to the user, which is essential for a polished application.”
Frequently Asked Questions (FAQs)
How do I perform a network request using URLSession in Swift?
To perform a network request using URLSession in Swift, create a URL object, then instantiate a URLSession data task with that URL. Call the `resume()` method on the task to start the request.
What is the best way to handle JSON data returned from a URLSession request?
The best way to handle JSON data is to use the `JSONDecoder` class. After receiving the data in the completion handler, decode it into your model objects using `decoder.decode(Model.self, from: data)`.
How can I handle errors when using URLSession?
You can handle errors by checking the `error` parameter in the completion handler of your data task. Implement appropriate error handling logic based on the error type, such as network connectivity issues or server errors.
What is the difference between dataTask and uploadTask in URLSession?
The `dataTask` is used for fetching data from a URL, while the `uploadTask` is specifically designed for uploading data to a server. Use `uploadTask` when you need to send data (e.g., files or form data) along with your request.
Can I cancel a URLSession data task after it has started?
Yes, you can cancel a URLSession data task at any time by calling the `cancel()` method on the task instance. This will stop the request and prevent any further processing of the response.
How do I ensure that my URLSession requests are performed on a background thread?
To perform URLSession requests on a background thread, use a background configuration when creating your URLSession instance. This can be done by calling `URLSession(configuration: .background(withIdentifier: “yourIdentifier”))`.
In Swift, using URLSession to return data from network requests is a fundamental aspect of developing applications that require internet connectivity. URLSession provides a robust API for making HTTP requests, handling responses, and processing data asynchronously. The key to effectively utilizing URLSession lies in understanding its configuration options, task types, and delegate methods, which allow developers to tailor their networking code to meet specific requirements.
When making network requests with URLSession, it is essential to handle the data returned properly. This involves parsing the response data, managing errors, and ensuring that the UI updates occur on the main thread. Utilizing completion handlers is a common practice, enabling developers to execute code after the data has been successfully fetched or an error has occurred. Additionally, employing Swift’s Codable protocol can simplify the process of converting JSON data into Swift objects, enhancing code readability and maintainability.
Another important aspect of working with URLSession is understanding its various task types, including data tasks, upload tasks, and download tasks. Each task type serves different use cases and can be optimized for performance based on the needs of the application. Moreover, leveraging features such as caching, background downloads, and authentication challenges can significantly improve the user experience and the efficiency of network operations.
Author Profile
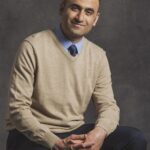
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?