How Can I Convert Swift URLSession Errors to String for Easier Debugging?
In the world of iOS development, managing network requests efficiently is crucial for creating responsive and user-friendly applications. Swift’s URLSession provides a powerful framework for handling these requests, but as any developer knows, the road to seamless networking is often fraught with challenges. One of the most common hurdles encountered is dealing with errors that arise during network operations. Understanding how to convert these errors into meaningful strings can significantly enhance debugging efforts and improve the overall user experience. In this article, we will explore effective strategies for transforming URLSession errors into human-readable strings, empowering developers to tackle issues with confidence.
When working with URLSession, developers frequently encounter various error types that can arise from network failures, invalid responses, or even server issues. Each of these errors comes with its own set of codes and descriptions, which can be daunting to interpret at first glance. However, by leveraging Swift’s error handling capabilities, developers can create a systematic approach to convert these errors into strings that provide clarity and context. This transformation not only aids in debugging but also allows developers to present more informative messages to users, enhancing the overall user experience.
As we delve deeper into the intricacies of error handling in URLSession, we will uncover best practices for capturing and converting errors into strings. By the end of this discussion
Understanding URLSession Errors
When working with URLSession in Swift, developers often encounter various errors that can arise during network requests. These errors can stem from a multitude of issues, such as connectivity problems, server unavailability, or incorrect URL formatting. Understanding how to convert these errors into a human-readable string format is vital for effective debugging and user feedback.
Common URLSession Error Types
Here are some common error types you might encounter when using URLSession:
- NSURLErrorNotConnectedToInternet: Indicates that the device is not connected to the internet.
- NSURLErrorTimedOut: Signifies that the request timed out.
- NSURLErrorBadURL: Represents a malformed URL string.
- NSURLErrorCannotFindHost: Implies that the host could not be found.
- NSURLErrorCannotConnectToHost: Denotes that the connection to the host failed.
Converting Errors to Strings
To convert URLSession errors into a string format, you can create a utility function that captures these errors and maps them to user-friendly messages. Below is an example of how to implement such a function in Swift:
“`swift
func errorToString(error: Error) -> String {
let nsError = error as NSError
let errorCode = nsError.code
switch errorCode {
case NSURLErrorNotConnectedToInternet:
return “No internet connection.”
case NSURLErrorTimedOut:
return “The request timed out.”
case NSURLErrorBadURL:
return “The URL provided was malformed.”
case NSURLErrorCannotFindHost:
return “Cannot find the specified host.”
case NSURLErrorCannotConnectToHost:
return “Cannot connect to the host.”
default:
return “An unknown error occurred: \(nsError.localizedDescription)”
}
}
“`
This function uses a switch statement to match the error codes with appropriate messages. If the error code does not match any known cases, it returns a generic error message along with the localized description.
Example Usage
Here’s how you might use the `errorToString` function in a URLSession data task:
“`swift
let url = URL(string: “https://example.com”)!
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error {
let errorMessage = errorToString(error: error)
print(“Error: \(errorMessage)”)
return
}
// Handle successful response
}
task.resume()
“`
This implementation ensures that any errors encountered during the network request are captured and displayed in a user-friendly manner.
Error Handling Best Practices
When dealing with URLSession errors, consider the following best practices:
- User Feedback: Always provide clear and actionable feedback to users when an error occurs.
- Logging: Log errors for further analysis to help identify recurring issues.
- Retry Logic: Implement retry logic for transient errors, such as timeouts or connectivity issues.
- Graceful Degradation: Ensure your app can still function to some extent even when certain requests fail.
Summary of Error Types and Messages
Error Type | User-Friendly Message |
---|---|
NSURLErrorNotConnectedToInternet | No internet connection. |
NSURLErrorTimedOut | The request timed out. |
NSURLErrorBadURL | The URL provided was malformed. |
NSURLErrorCannotFindHost | Cannot find the specified host. |
NSURLErrorCannotConnectToHost | Cannot connect to the host. |
By implementing these practices and utilizing the error handling function, developers can create more robust applications that enhance user experience even in the face of network issues.
Handling URLSession Errors in Swift
When working with `URLSession` in Swift, managing errors effectively is crucial to building robust applications. Errors can arise from network issues, server responses, or data parsing problems. Understanding how to convert these errors into user-friendly strings can enhance the user experience.
Converting URLSession Errors to Strings
To convert `URLSession` errors to strings, you can utilize Swift’s `NSError` class. The `error` object returned in the completion handler is typically of type `Error`, which can be cast to `NSError` for detailed description.
Implementation Example
Here’s a basic implementation of how to convert a `URLSession` error into a string:
“`swift
func fetchData(from url: URL) {
let task = URLSession.shared.dataTask(with: url) { data, response, error in
if let error = error as NSError? {
let errorMessage = error.localizedDescription
print(“Error fetching data: \(errorMessage)”)
return
}
// Handle response and data
}
task.resume()
}
“`
Error Handling Strategies
When handling errors in URLSession, consider the following strategies:
- Check for Network Connectivity: Use `Network` framework to determine if the device is connected to the internet.
- Analyze Status Codes: Inspect the HTTP status codes in the response to identify client or server errors.
Status Code | Description |
---|---|
200 | Success |
400 | Bad Request |
401 | Unauthorized |
404 | Not Found |
500 | Internal Server Error |
Custom Error Messages
For a better user experience, consider creating custom error messages based on the error type. Here’s a simple function to map errors to user-friendly messages:
“`swift
func userFriendlyErrorMessage(for error: NSError) -> String {
switch error.code {
case NSURLErrorNotConnectedToInternet:
return “Please check your internet connection.”
case NSURLErrorTimedOut:
return “The request timed out. Please try again.”
default:
return “An unexpected error occurred: \(error.localizedDescription)”
}
}
“`
This function can be integrated into your error handling logic to provide clearer feedback to users.
Logging Errors for Debugging
In addition to user-facing error messages, logging errors is vital for debugging. Use the following code snippet to log errors:
“`swift
func logError(_ error: NSError) {
let logMessage = “Error Code: \(error.code), Description: \(error.localizedDescription)”
print(logMessage)
}
“`
Integrate logging with your error handling to ensure that developers can track and resolve issues effectively.
Expert Insights on Converting Swift URLSession Errors to Strings
Jordan Lee (Senior iOS Developer, Tech Innovations Inc.). “When handling errors in URLSession, it is crucial to convert them to a user-friendly string format. This not only aids in debugging but also enhances the user experience by providing clear feedback on what went wrong during network requests.”
Alexandra Kim (Software Engineer, Mobile Development Team Lead at App Solutions). “Utilizing Swift’s error handling capabilities, one can create a function that maps URLSession errors to descriptive strings. This approach allows developers to easily log and display meaningful error messages, improving overall app reliability.”
Michael Tran (Lead iOS Architect, Future Tech Labs). “To effectively convert URLSession errors to strings, I recommend implementing a centralized error handling strategy. By categorizing errors and providing specific messages, developers can streamline the troubleshooting process and enhance the maintainability of their code.”
Frequently Asked Questions (FAQs)
What is URLSession in Swift?
URLSession is a class in Swift that provides an API for downloading and uploading data to and from web services. It supports various tasks such as data transfer, file downloads, and background downloads.
How can I convert a URLSession error to a string?
To convert a URLSession error to a string, you can use the `localizedDescription` property of the error object. This property provides a human-readable description of the error.
What types of errors can occur with URLSession?
Common errors include network connectivity issues, timeouts, invalid URLs, and server response errors. Each error type can be identified using the error code and domain.
How do I handle URLSession errors in Swift?
You can handle URLSession errors by implementing the `completionHandler` in your data task. Check for errors in the response and use the error object to determine the type of error and respond accordingly.
Can I create custom error messages for URLSession errors?
Yes, you can create custom error messages by checking the error code and domain, then mapping them to user-friendly messages. This can enhance the user experience by providing clearer feedback.
Is it possible to log URLSession errors for debugging?
Yes, logging URLSession errors is a good practice for debugging. You can log the error’s localized description and any relevant information from the response to help identify issues during development.
In Swift, handling errors generated by URLSession is a crucial aspect of network programming. When working with URLSession, developers often encounter various types of errors, such as connectivity issues, timeouts, or invalid responses. Converting these errors into a user-friendly string format is essential for effective debugging and user feedback. By utilizing Swift’s error handling mechanisms, developers can extract meaningful information from the error objects and present it in a clear, understandable manner.
To convert a URLSession error to a string, developers can leverage the localizedDescription property of the NSError object associated with the error. This property provides a human-readable description of the error, which can be displayed to users or logged for further analysis. Additionally, it is beneficial to implement custom error handling strategies that categorize errors and provide context-specific messages, enhancing the overall user experience.
understanding how to effectively handle and convert URLSession errors to strings is vital for Swift developers. By focusing on proper error management, developers can improve their applications’ robustness and provide clearer communication regarding network issues. This not only aids in debugging during development but also enhances user satisfaction by delivering informative feedback when errors occur.
Author Profile
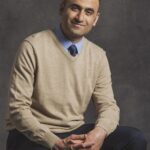
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?